The `git commit` command is used to save changes to the local repository, while `git commit --amend` allows you to modify the most recent commit, effectively replacing it with new content.
Here's a code snippet demonstrating both commands:
# Standard commit
git commit -m "Add new feature"
# Amend the last commit with a new message
git commit --amend -m "Update feature to improve performance"
What is Git Commit?
Definition and Purpose
The `git commit` command serves as a fundamental component of Git and is pivotal for tracking changes in your project. A commit encapsulates a snapshot of your project at a specific point in time, allowing you to revisit previous states, understand your project's history, and collaborate effectively with others.
Syntax and Basic Usage
The basic syntax for the `git commit` command is straightforward. At its simplest, you provide a message that describes the change you're making:
git commit -m "Your commit message here"
Here, the `-m` flag allows you to provide a short, meaningful message directly in the command line. This can significantly streamline the commit process, especially when working on a tight deadline.
Commit Best Practices
Crafting effective commit messages is crucial for maintaining a clean and comprehensible project history. A good commit message should briefly summarize the changes made, providing context for future collaborators (or yourself) who will revisit the project later.
Consider following conventional commit message formats, which categorize commits using prefixes such as `feat` (for features), `fix` (for bug fixes), and others.
Examples of Good and Bad Commit Messages:
- Bad: "Fixed stuff"
- Good: "fix: correct typos in the documentation"
This clarity helps to maintain the project in the long run and facilitates easier code reviews.
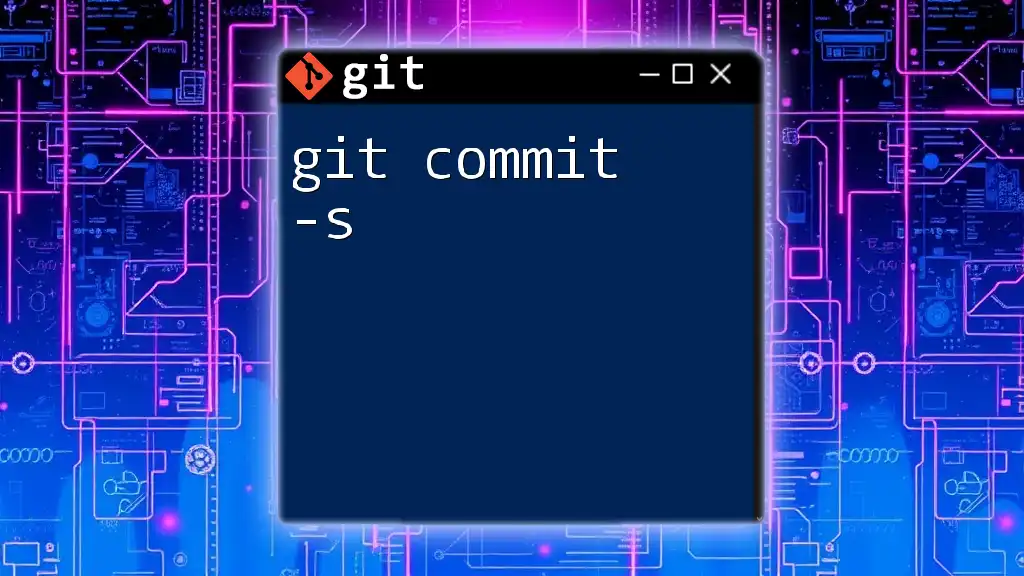
Git Commit Options and Flags
Common Flags
Beyond the basic command, `git commit` has a variety of options and flags that can enhance its functionality. Here are some commonly used flags:
-
`-a` or `--all`: Automatically stages all modified tracked files before creating a commit. This is particularly useful when you want to skip the staging step for changes.
-
`--amend`: Use this option to modify the last commit instead of creating a new one. It allows you to update the commit message or include additional changes.
-
`--no-edit`: When amending a commit, this flag keeps the previous commit message intact.
Examples
Using the `-a` flag can be seen in the following command:
git commit -a -m "Updated styles"
Here, modified files are automatically staged for commit.
To modify the last commit with `--amend`, you can use:
git commit --amend -m "Updated README with better instructions"
This command effectively replaces the last commit with the new changes, allowing for corrections or enhancements.
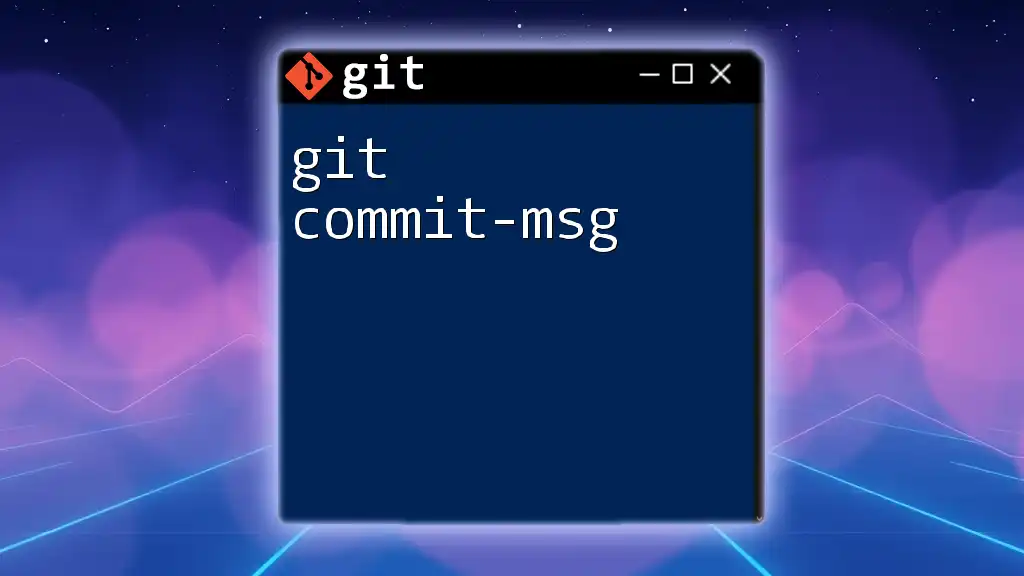
Alternatives to Git Commit
Staging States
Understanding the Staging Area
The staging area, often referred to as the index, is a space where you prepare changes before committing them to your repository. Understanding this concept is essential because it allows you to create commits that only reflect specific changes rather than all modified files.
Using `git commit --amend`
Use Cases for Modifying Previous Commits
Sometimes, you may realize you've made a mistake immediately after committing. Instead of cluttering your project history with correction commits, you can use the `--amend` option to seamlessly update your last commit.
Squashing Commits
What is Squashing?
Squashing commits is the process of combining multiple commits into a single commit. This is particularly valuable when you want to keep your project history clean and focused, especially after completing a feature.
How to Squash Commits
You can utilize interactive rebase to squash commits. The syntax is as follows:
git rebase -i HEAD~n
In this command, replace `n` with the number of commits you wish to squash. When prompted, you can mark the commits you want to combine. The result is a single, consolidated commit that retains the summary of changes.
Cherry-Picking Commits
What is Cherry-Picking?
Cherry-picking allows you to apply specific commits from one branch to another, bypassing the need for a complete merge.
How to Cherry-Pick
To cherry-pick a commit, use the command:
git cherry-pick COMMIT_HASH
Replace `COMMIT_HASH` with the actual hash of the commit you want to apply. This command is beneficial when working on several feature branches but only want to integrate selected changes.
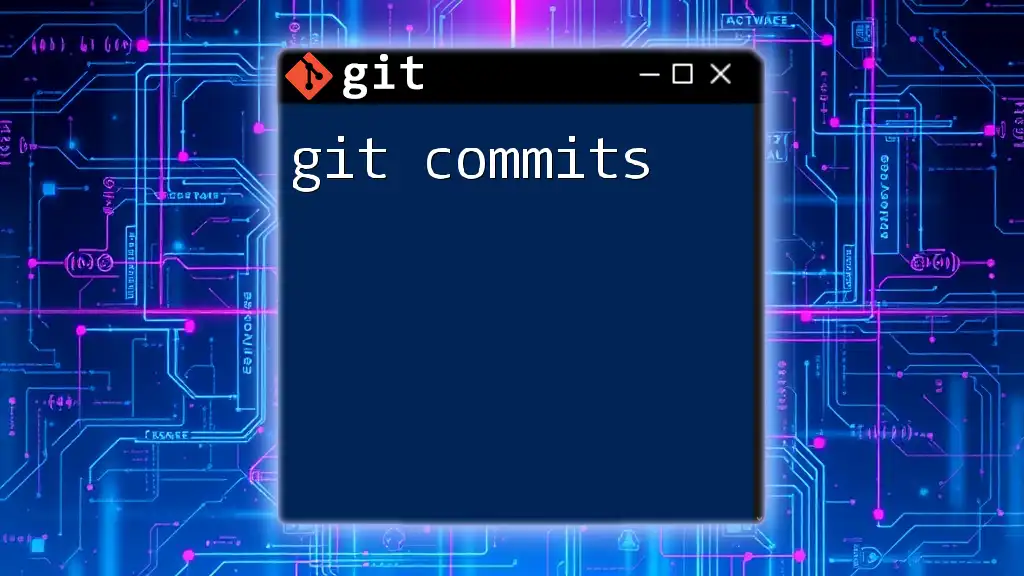
Advanced Commit Techniques
Commit Hooks
What are Commit Hooks?
Commit hooks are scripts that Git executes before or after events such as commits or merges. They can help automate checks or enforce coding standards.
Configuring a Pre-commit Hook
For example, to set up a pre-commit hook that runs ESLint (a popular JavaScript linter), you can create a script in your `.git/hooks` directory:
#!/bin/sh
eslint .
This approach ensures that your code maintains a certain quality before being added to the version history.
Signed Commits
What are Signed Commits?
Signed commits provide an additional level of verification. They ensure that the commit was truly made by the author it claims to represent, thereby enhancing your project’s security.
How to Create Signed Commits
You can sign your commits using the `-S` flag:
git commit -S -m "This is a signed commit"
This requires an available GPG key and signifies that you take responsibility for the changes being committed.
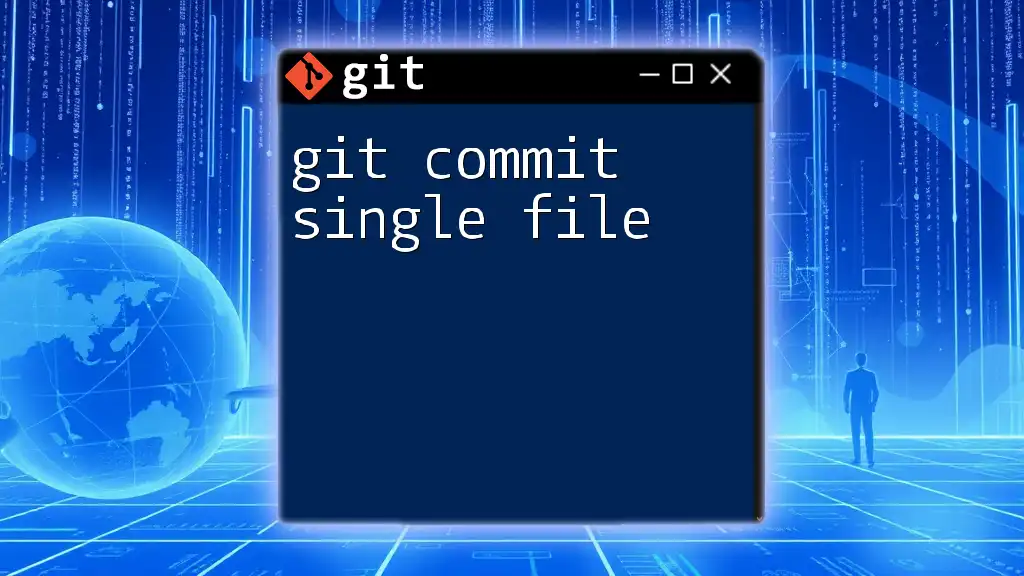
Conclusion
Understanding the `git commit` command and its various options and alternatives is essential for managing your project's version control effectively. The importance of clear commit messages, the utility of the staging area, and the advanced techniques like squashing and cherry-picking all contribute to a streamlined workflow. With these practices, you can ensure that your project history is clean, efficient, and easy to navigate. Dive deeper into Git commands and expand your knowledge to enhance your productivity further!
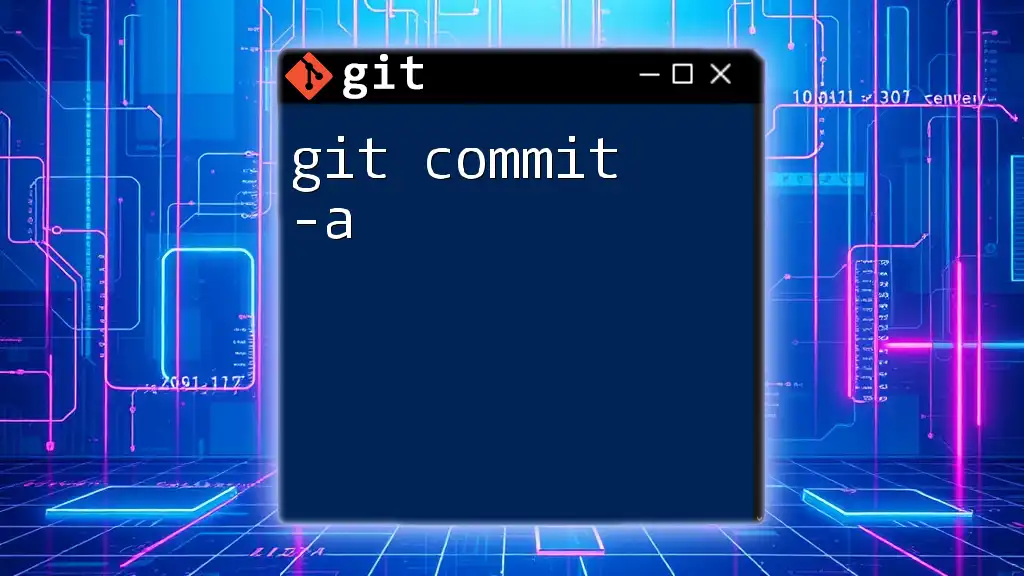
Additional Resources
For more in-depth information, please refer to the official Git documentation, and consider resources like books and tutorials that explore Git in greater detail.
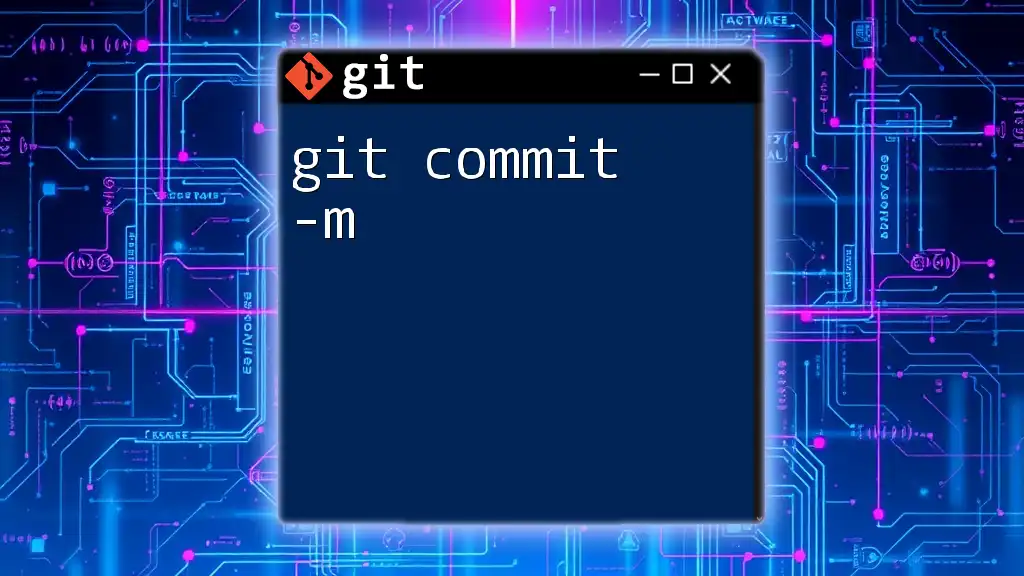
Frequently Asked Questions (FAQs)
What is the difference between `git commit` and `git push`?
While `git commit` records changes in the local repository, `git push` is the command used to transfer those committed changes from your local repository to a remote repository, thereby sharing your work with others.
Can I recover a lost commit?
Yes, you can use the `git reflog` command to recover lost commits. This command allows you to view all actions taken in the repository, making it easier to find and restore previous commits.
How do I revert a commit?
If you need to undo changes made by a specific commit, you can use the `git revert` command, which creates a new commit that effectively cancels out the changes made by the commit you specify.