The Git commit history is a record of all the changes made to a repository, allowing users to track modifications, revert to previous states, and understand project evolution.
You can view the commit history using the following command:
git log
Understanding Git Commits
To fully grasp git commit history, it's crucial to understand what a commit is. A commit in Git represents a snapshot of the project's current state at a specific point in time. Each commit is like a save point in a video game, allowing you to go back and restore previous versions or changes.
Components of a Git Commit
Each commit has several vital components:
- Commit Hash: This is a unique identifier generated by Git for every commit, allowing you to reference it precisely.
- Author Information: This includes the name and email of the person who made the changes, adding clarity and accountability.
- Commit Message: A clear description that outlines what changes were made. Slightly like a book's title, it gives context to the changes.
- Timestamp: Indicates when the changes were made, providing a timeline for project evolution.
Understanding these components is essential when looking at git commit history because they give significant insights into the development process.
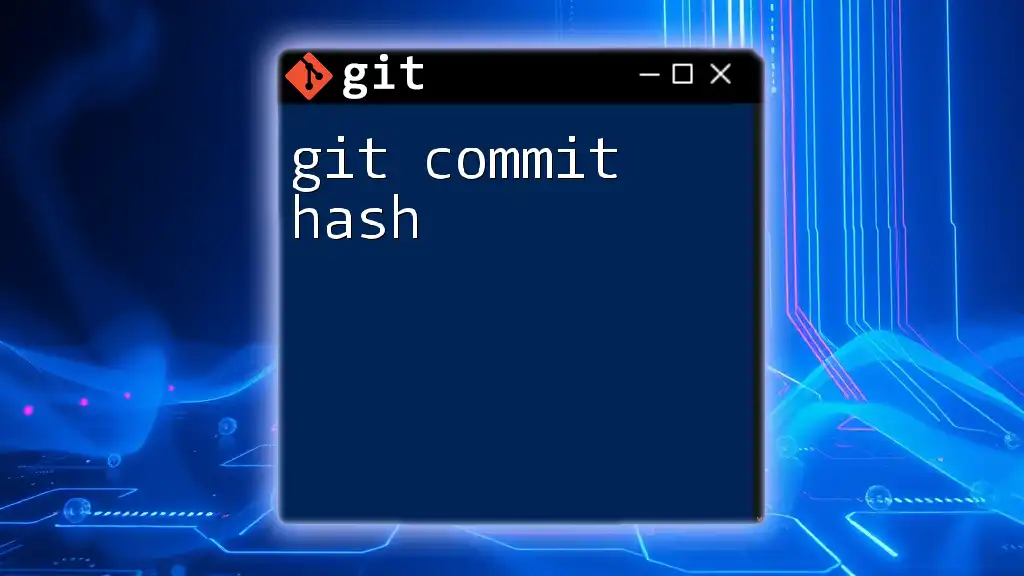
Viewing Commit History
Using the `git log` Command
One of the simplest ways to view your git commit history is through the `git log` command. By running the command:
git log
you'll see a chronologically ordered list of commits, with the most recent entries appearing first. Each entry includes the commit hash, author, date, and commit message.
Formatting the Output
To make the output more readable, you can customize it using various options. For example:
git log --oneline --graph --decorate
This command formats the log to display each commit on one line, presents a visual graph of branches, and decorates it with references to branches and tags.
How to View Specific Commits
You can filter your log to display only certain commits:
- To limit the number of commits shown, use:
git log -n 5
- To view commits made by a specific author, employ:
git log --author="John Doe"
Understanding the Output
Understanding the output of `git log` is crucial for navigating your project's history. Each commit hash represents a unique commit, the author field shows who made the changes, the date provides context for when the changes were made, and the commit message summarizes what was altered.
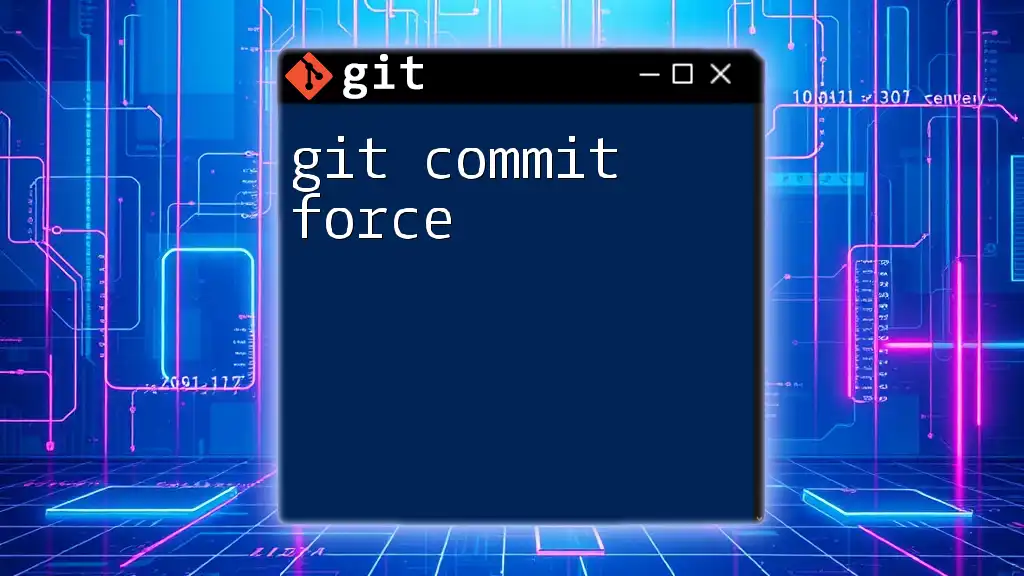
Navigating the Commit History
Checking Out Previous Commits
If you wish to view the state of your repository at a specific commit, you can use the `git checkout` command followed by the commit hash:
git checkout <commit-hash>
This will take you to a "detached HEAD" state, meaning you are not on any branch. Be cautious with this state to avoid making unintentional changes.
Reverting to a Previous Commit
Sometimes, you might need to undo changes without altering the commit history. You can achieve this through the `git revert` command, which creates a new commit that undoes changes made in a specified commit. To do this, use:
git revert <commit-hash>
This method preserves the commit history while effectively negating changes you wish to remove.
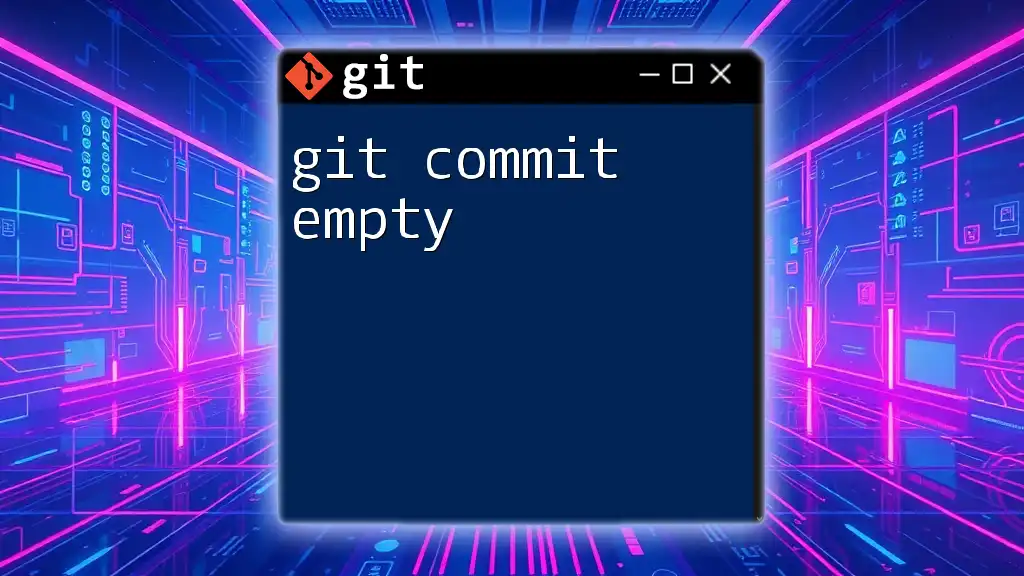
Analyzing Commit History
Finding Changes using `git diff`
To analyze the specific differences between commits, you can use the `git diff` command. This powerful tool enables you to compare two different commit states:
git diff <commit-hash-1> <commit-hash-2>
This command provides a line-by-line breakdown of what changed between the two commits, allowing you to track modifications effectively.
Understanding Commit History with `git blame`
Another valuable command is `git blame`, which lets you identify who changed a particular line of code within a file:
git blame <file>
This command displays each line of the specified file along with the last commit hash that modified that line, giving insight into the contribution of each collaborator.
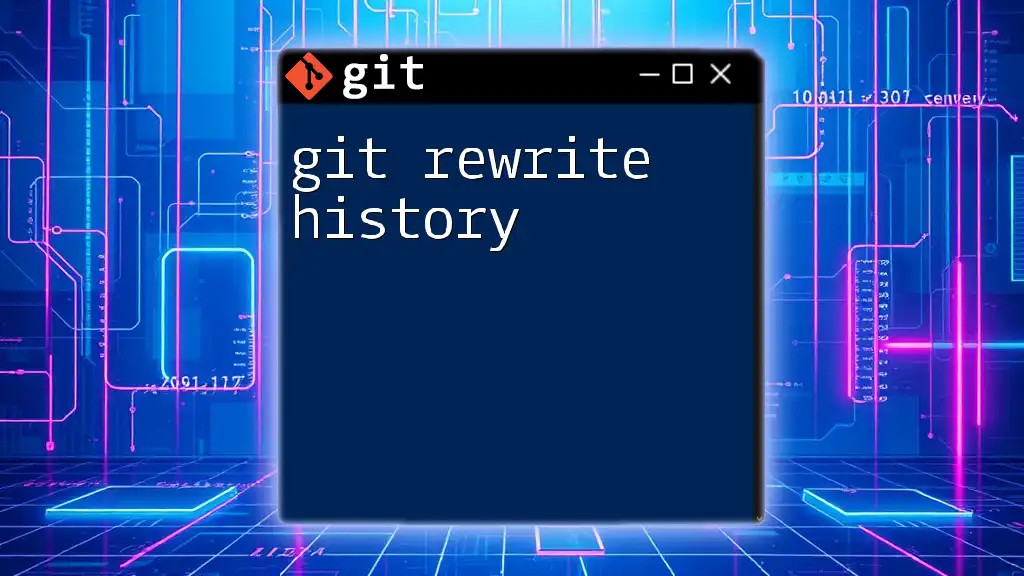
Best Practices for Commit Messages
Writing Clear and Concise Commit Messages
Well-structured commit messages are crucial for understanding project history. Avoid vague messages; instead, aim for clarity and context. For reference, here’s a good example of a commit message format:
Fix bug in user login function
- Corrected the input validation for user credentials.
- Improved error messaging for invalid inputs.
Structuring Commit Messages
A great commit message typically includes two parts:
- Subject Line: A brief, one-line summary of the changes (ideally 50 characters or less).
- Body: A more detailed description of the changes, if necessary.
This format helps future contributors understand the purpose of changes without needing to dig into the code itself.
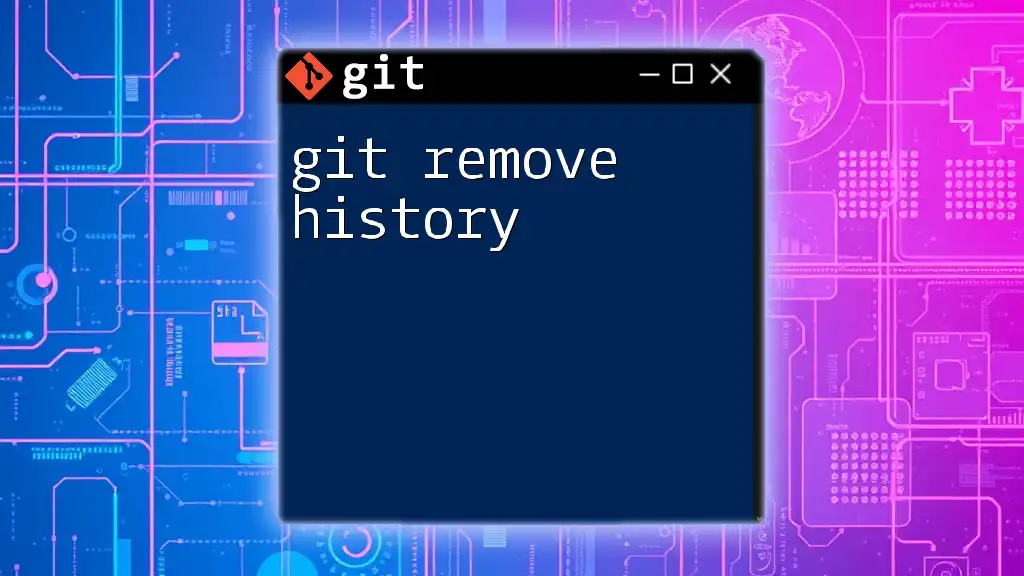
Advanced Techniques with Commit History
Interactive Rebase with `git rebase`
For those looking to modify multiple commits or streamline commit history, interactive rebasing is a powerful tool. It allows you to select commits for editing, squashing, or reordering. You can begin an interactive rebase for the last few commits with:
git rebase -i HEAD~3
This command opens an editor where you can change the order or content of your commits.
Squashing Commits
Squashing commits is another method for cleaning up commit history. It consolidates several commits into one, making your log clearer. To do this during an interactive rebase, you would choose "squash" for the commits you want to combine.
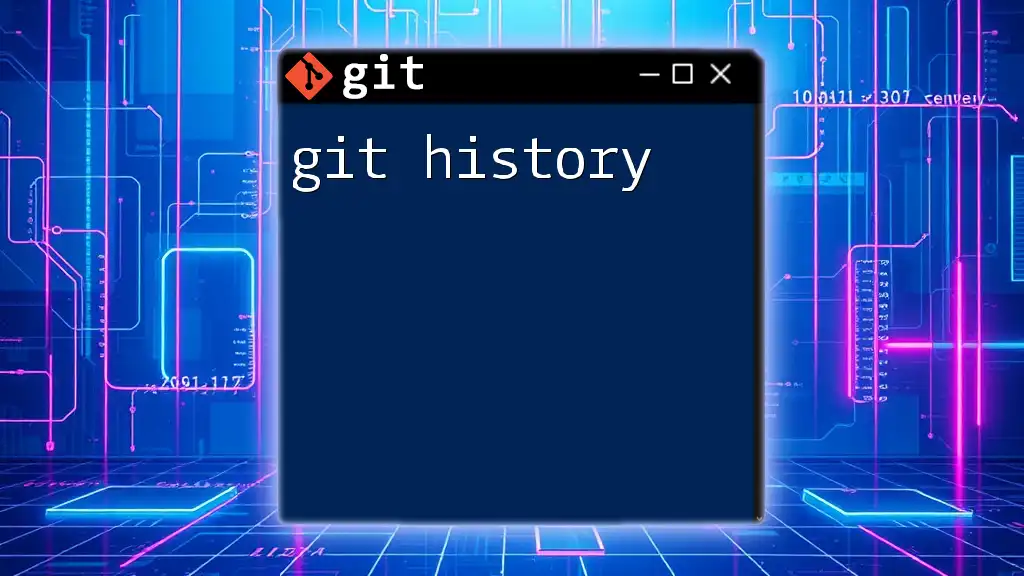
Conclusion
Understanding git commit history is foundational for effective collaboration and version control in software development. By mastering commands like `git log`, `git checkout`, and `git revert`, as well as employing best practices for commit messages, you'll not only enhance your own workflow but also contribute to a more comprehensible project history. Practice using these commands, and as you do, you'll become more proficient in navigating and managing your Git repositories efficiently.
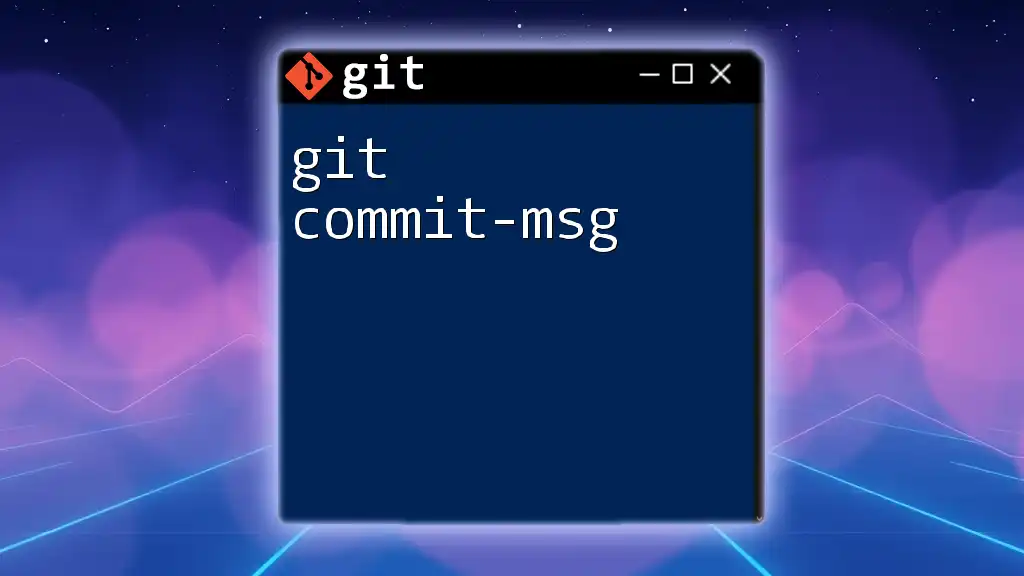
Additional Resources
For further learning, check out the official Git documentation or recommended tutorials that can deepen your understanding and skills regarding Git and git commit history.