To remove the history of your Git repository while keeping the current state of your files, you can create a new branch from the current state and remove all previous commits by using the following commands:
git checkout --orphan new-branch
git add -A
git commit -m "Initial commit with no history"
git branch -D master
git branch -m master
Understanding Git History
What is Git History?
Git history refers to the chronological series of commits that record the changes made to a project within a Git repository. Each commit contains important metadata including the author, timestamp, and a unique hash identifier, along with the actual changes made to the codebase. Understanding the commit log is crucial, as it allows developers to track collaboration effectively, debug issues, and revert to previous states if necessary.
Why Remove Git History?
Several reasons motivate users to remove history in a Git repository:
-
Security Concerns: Sensitive information, such as passwords or API keys, accidentally committed to a repository can pose security threats. Removing the history that contains this data is essential.
-
Repository Size: Over time, repositories can grow significantly in size due to unnecessary files and commits. Cleaning up history can reduce this footprint and enhance performance.
-
Mistaken Commits: Developers may sometimes commit changes that are incorrect, irrelevant, or should not have been included. Removing these commits will help maintain a cleaner and more relevant project history.
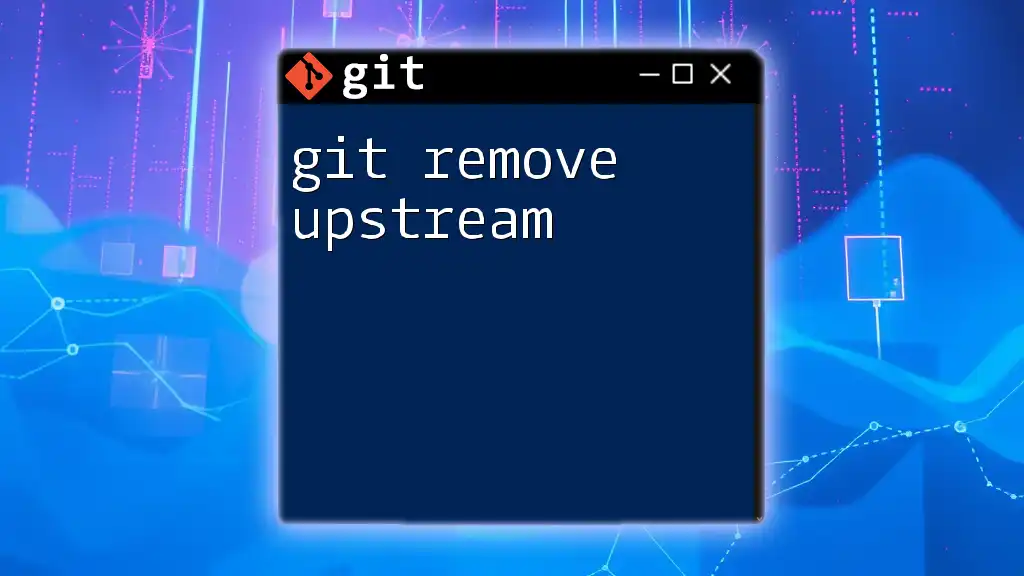
Methods to Remove History
Using `git rebase`
Rebasing is a powerful Git feature that allows you to rewrite commit history. By using `git rebase -i` (interactive rebase), you can edit, squash, or even delete specific commits.
To start an interactive rebase, run the command:
git rebase -i HEAD~n
Replace `n` with the number of commits you wish to review. This will open an editor listing the commits, allowing you to modify their actions (e.g., squashing them together).
For instance, if you decide to squash multiple commits into one, change `pick` to `squash` for the relevant commits. After saving and exiting, Git will merge those commits, creating a cleaner commit history.
Pros:
- Great for making small adjustments to the recent commit history.
- Keeps the branch history linear.
Cons:
- Can be dangerous if other collaborators have based their work on the commits you're changing, as it can create confusion.
Using `git reset`
The `git reset` command helps you manipulate your recent commits in various ways. It’s crucial to understand the differences between soft, mixed, and hard resets:
-
Soft Reset: Moves the HEAD pointer to a previous commit while leaving changes in the staging area. It is useful for reworking commits without losing changes.
git reset --soft HEAD~n
-
Mixed Reset: Moves the HEAD to a previous commit and puts all changes in your working directory, untracked by Git.
git reset HEAD~n
-
Hard Reset: Discards all changes and resets the repository to a previous state, effectively erasing history and any staged changes.
git reset --hard HEAD~n
Use Case Scenarios:
- Use a soft reset when you want to amend your last commit and keep your work.
- Use mixed reset for reorganizing changes.
- Apply hard reset with caution for a total rollback to a previous state.
Potential Pitfalls: Note that a hard reset is irreversible—the deleted data will be lost unless you have backups or you can access the reflog.
Using `git filter-branch`
`git filter-branch` is a powerful command used for rewriting older commits across the entire repository history. It allows for more comprehensive changes, such as removing files from past commits.
When to use `git filter-branch`: It's particularly effective for large rewrites or purging sensitive information from the entire commit history.
For example, to remove a specific file from all commits, you would execute:
git filter-branch --force --index-filter \
"git rm --cached --ignore-unmatch <file>" \
--prune-empty --tag-name-filter cat -- --all
Implications: Be cautious when using this command, as it can significantly change the history and will probably require that you force-push your changes to any shared repositories.
Using `BFG Repo-Cleaner`
Introduction to BFG Repo-Cleaner
BFG Repo-Cleaner is an efficient alternative to `git filter-branch`, specifically designed for repository cleaning tasks. It operates faster and is more user-friendly, making it an excellent choice for beginners and experienced users alike.
How to Use BFG for Removing History
To get started with BFG:
- Install BFG according to your platform's guidelines.
- For example, to remove all occurrences of a file across your history, you can execute:
bfg --delete-files <filename>
After running BFG, clean up your repository with:
git reflog expire --expire=now --all
git gc --prune=now --aggressive
Advantages: BFG significantly streamlines the process of removing large files or sensitive data, ensuring your repository is efficiently cleaned without undue complexity.
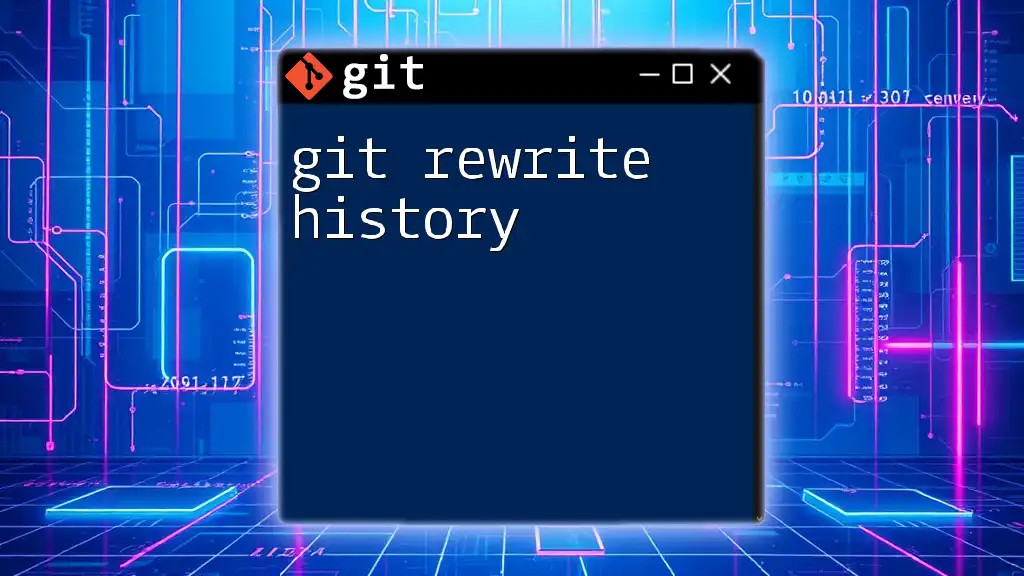
Best Practices to Follow
Backup Your Repository
Before any operation that alters Git history, always ensure you create backups. This safeguard is paramount, as it allows you to restore your work if anything goes wrong. You can clone your repository or simply archive your `.git` directory.
Understanding Implications on Remote Repositories
When you decide to remove history, remember that this change affects all collaborators. Communicate your intentions clearly, and be prepared for the possibility that they may need to align their own local branches with the updated history.
To synchronize changes with the remote repository, you may need to force-push your changes by using:
git push origin --force
Note that force pushing can overwrite changes made by others, so utilize this command judiciously.
Communicating with Your Team
Notify your team or collaborators about the changes you plan to make to the repository history. Effective communication ensures that they can adjust their workflows, minimizing disruption. Consider providing instructions or a guide to help your team align their local repositories with the new state of the project.
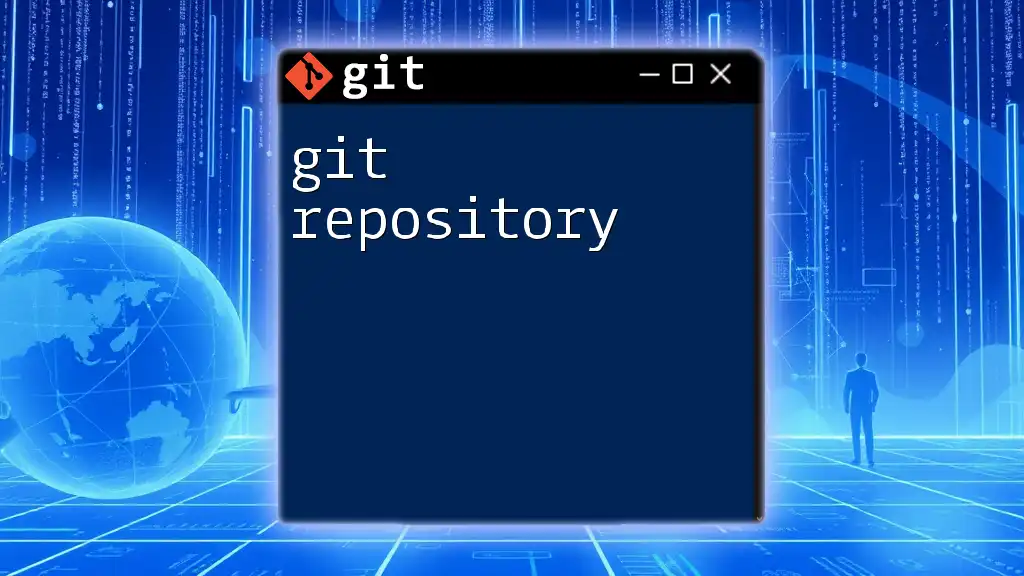
Conclusion
Removing history in Git is a powerful yet sensitive operation. Understanding various methods like `git rebase`, `git reset`, `git filter-branch`, and BFG Repo-Cleaner equips you to make informed decisions that best serve your project's needs. Always remember to back up your repository before making significant changes and communicate effectively with your team to maintain a collaborative and supportive environment. By practicing careful management of your repository history, you can maintain a clean, efficient, and secure codebase for your projects.
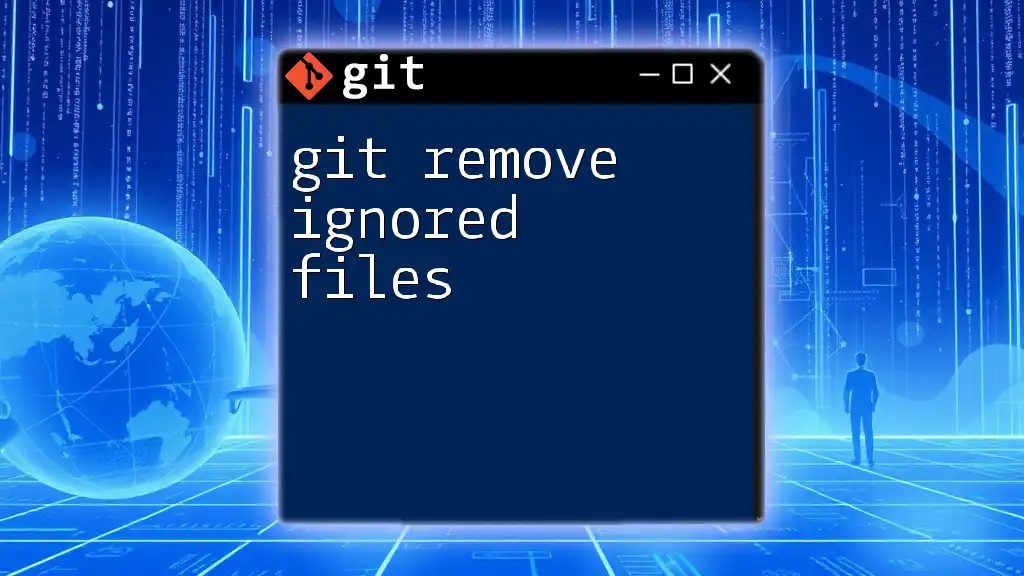
Additional Resources
For further learning, consider exploring the official Git documentation or engaging with community forums dedicated to Git. Recommended platforms for deepening your Git knowledge include online courses, video tutorials, and collaborative coding environments that focus on version control best practices.