In Git, the command to view the commit history of a repository is `git log`, which displays a chronological list of commits along with their unique identifiers, authors, dates, and messages.
git log
What is Git History?
Git history refers to the record of all commits made in a Git repository, tracking each change over time. As a powerful version control system, Git allows you to maintain a detailed history of your project, capturing all modifications, additions, and deletions made by contributors. This chronological timeline is not only essential for understanding how a project has evolved, but it also plays a crucial role in collaborative software development.
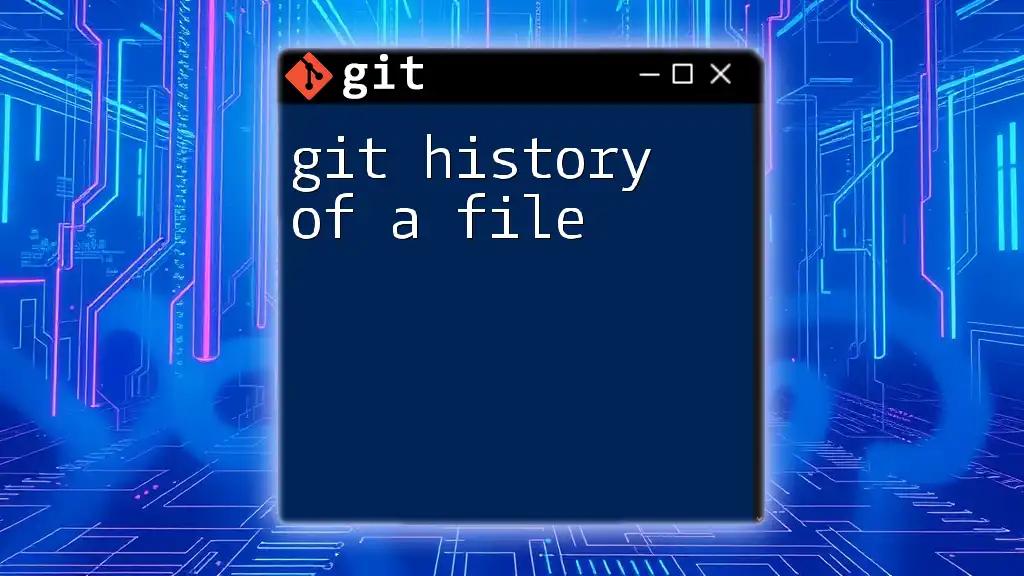
Key Concepts of Git History
Understanding Commits
A commit in Git is like a snapshot of changes made to the files in your repository at a particular point in time. Each commit consists of several components:
- Commit Hash: A unique identifier (typically a long string of alphanumeric characters) generated for each commit. This serves as a reference that can be used to retrieve specific commits.
- Author: The name and email of the person who made the changes.
- Date: The timestamp when the commit was created.
- Commit Message: A brief description detailing the purpose and content of the changes.
To create a commit, use the following command:
git commit -m "Your commit message here"
Writing clear and concise messages is vital as it helps maintainers and collaborators understand what changes were made and why.
The Commit Tree Structure
Git organizes commits in a tree structure that allows for effective tracking of changes. Each commit points to its parent commit, creating a lineage that illustrates the project's evolution. It's important to recognize that the most recent commit has no parent if it is the first in the branch, while merges produce commits with multiple parents.
You can visualize the commit tree by running:
git log --graph --oneline --all
This command presents a simplified view of the repository's history, showing a graphical representation of the commit relationships.
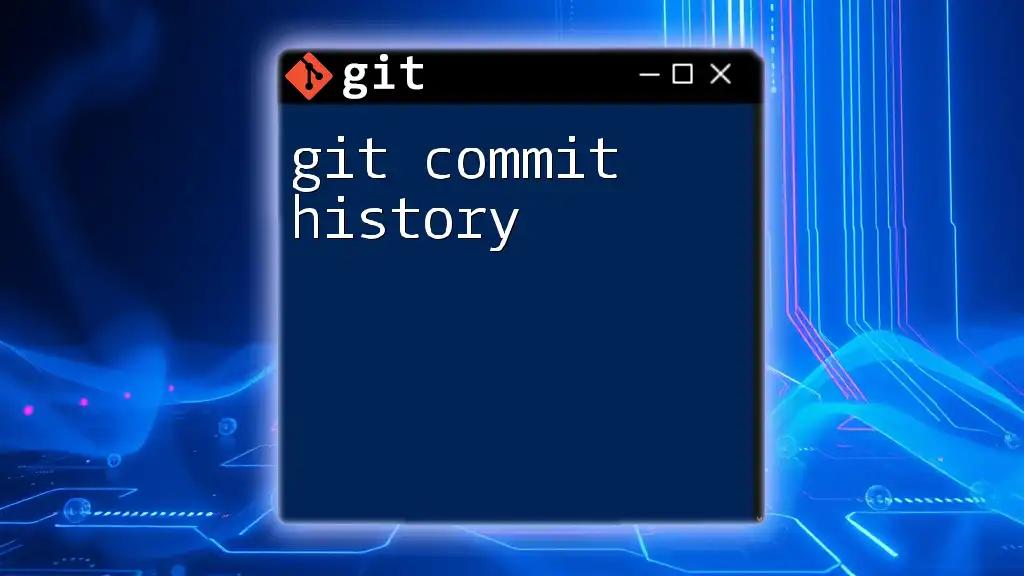
Viewing Git History
The Git Log Command
The `git log` command is fundamental for viewing the git history of a repository. When executed without any options, it shows a list of commits in reverse chronological order:
git log
You can customize this command to get more specific information. For instance, the `--oneline` option gives you a compact summary of each commit:
git log --oneline
Additional formatting options include:
- `--stat`: Shows the number of files changed and lines added or deleted in each commit.
- `--name-only`: Displays only the names of the files that were modified.
Use this command for a more detailed overview:
git log --oneline --stat
Commit History Options
The git log command also allows for filtering commits based on specific criteria. You can filter by author using the `--author` option, or by date using the `--since` and `--until` options:
git log --author="Name" --since="2 weeks ago"
These filtering capabilities are crucial for locating specific changes, especially in a project with an extensive commit history.
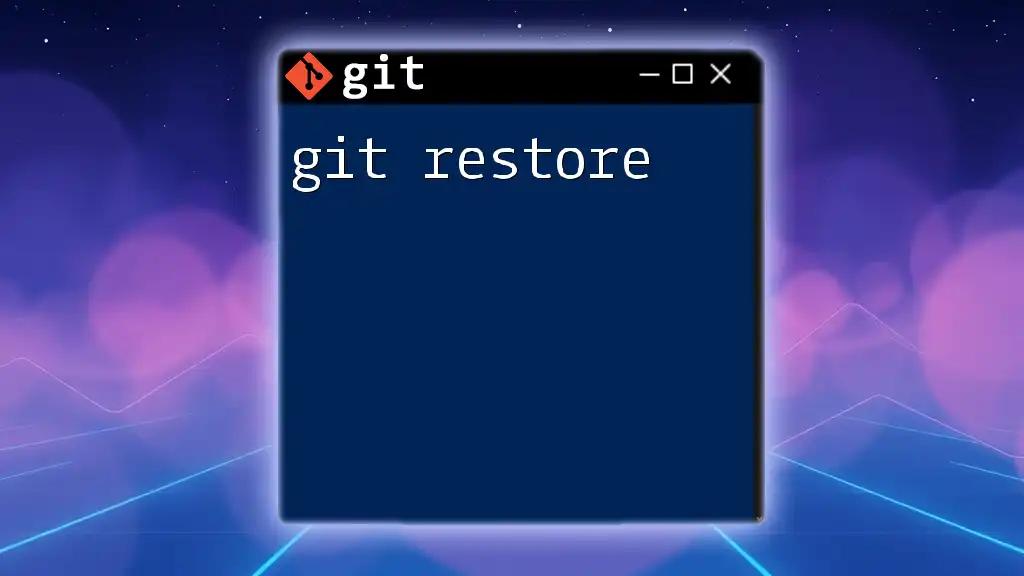
Navigating Through Commit History
Checking Out Previous Commits
To explore the repository at different points in time, you can check out previous commits using the `git checkout` command. This command allows you to switch your working directory to the state of a specific commit:
git checkout <commit-hash>
Note that checking out a commit will place you in a "detached HEAD" state, which means you'll not be on a branch. It's essential to work on a new branch or return to your previous branch afterward.
Reverting to a Previous State
When you need to undo changes, understanding the difference between `git revert` and `git reset` is crucial.
-
git revert creates a new commit that undoes the changes made by a specified commit. It's a safe way to remove changes while preserving history.
git revert <commit-hash>
-
git reset, on the other hand, moves the current branch to a specified commit and can change your working directory to match that commit. Use caution here, as it can rewrite history.
Understanding when to use these commands is vital for maintaining a clean and comprehensible project history.
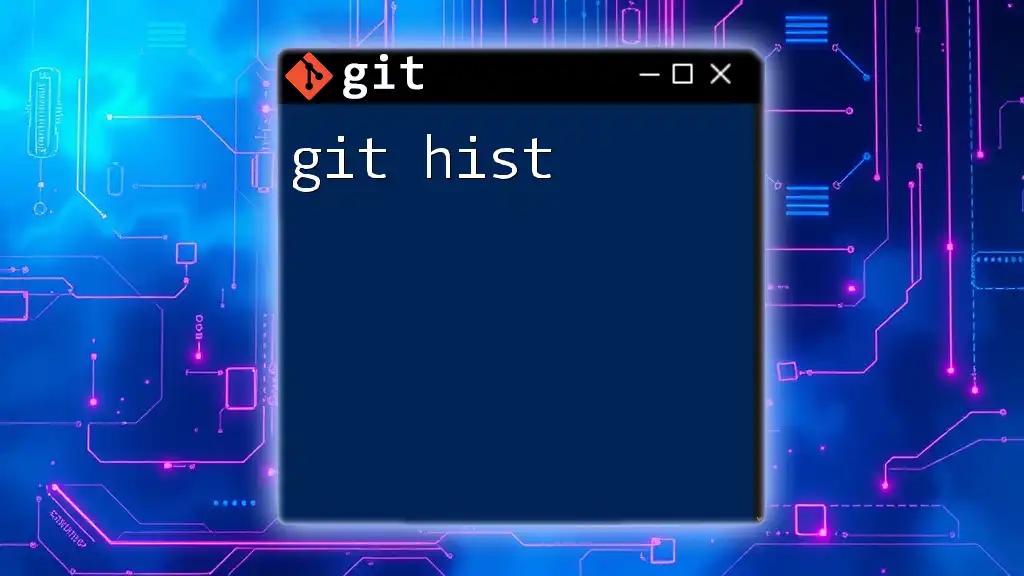
Annotated vs. Unannotated Tags
Understanding Tags
Tags are references to specific points in Git history, commonly used to mark release versions. There are two types of tags:
-
Annotated Tags: Created with the `-a` flag, these tags include information like the tagger's name, email, and date, along with a message. They are also stored as full objects in the Git database.
git tag -a v1.0 -m "Version 1.0"
-
Lightweight Tags: These are simply pointers to existing commits and do not have any additional metadata.
Using tags helps in easily accessing and managing specific versions of your project, aiding in deployment and versioning strategies.
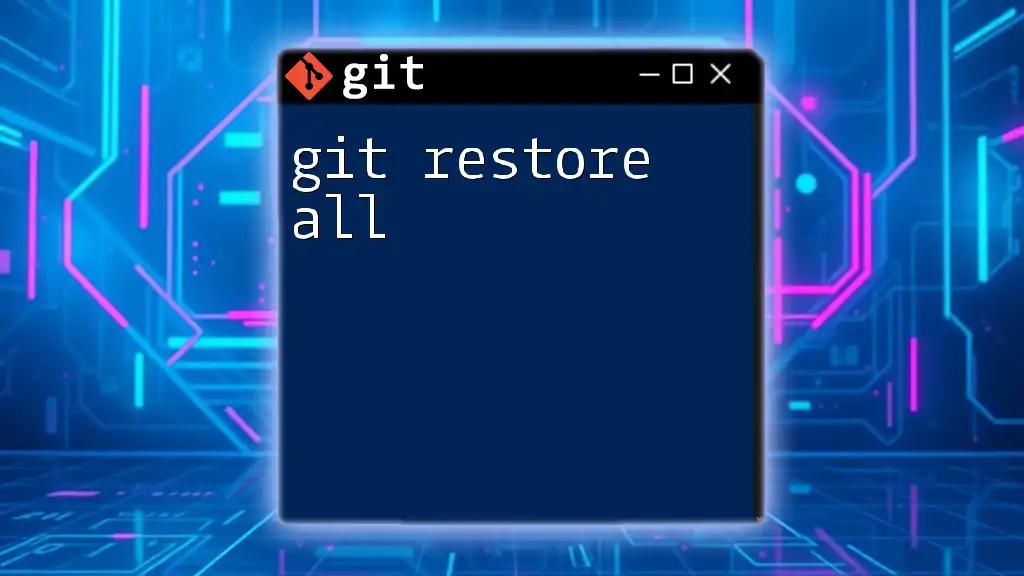
Practical Applications of Git History
Analyzing Code Changes
Understanding Git history allows you to analyze changes over time. The ability to view diffs between commits helps in assessing what modifications were made and their impact. You can view these differences using:
git diff <commit-hash1> <commit-hash2>
This command displays changes made between two different commits, allowing for thorough review and understanding.
Collaboration in Teams
In a team setting, comprehension of git history is crucial for effective collaboration. It aids in understanding who made specific changes and the rationale behind them. It also plays an essential role in resolving merge conflicts, as you can trace back to the last common commit between branches.
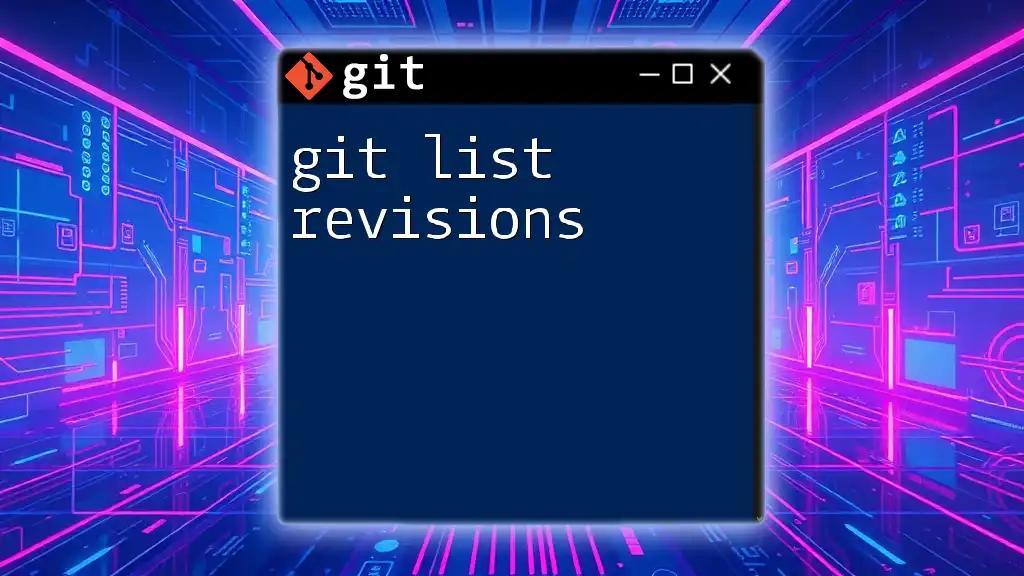
Best Practices for Managing Git History
Writing Quality Commit Messages
Crafting quality commit messages is vital for maintaining an understandable repository. Here are some best practices:
- Use imperative mood—describe what the commit will do, not just what it did (e.g., "Fix bug" instead of "Fixed bug").
- Provide context in the message for why the changes were necessary.
- Keep the summary concise but informative, ideally under 50 characters.
Using Branches Wisely
Branching effectively allows for isolated development, leading to a cleaner commit history. Each feature or bug fix should be worked on in its branch, leading to more manageable pull requests and a clearer history upon merging.
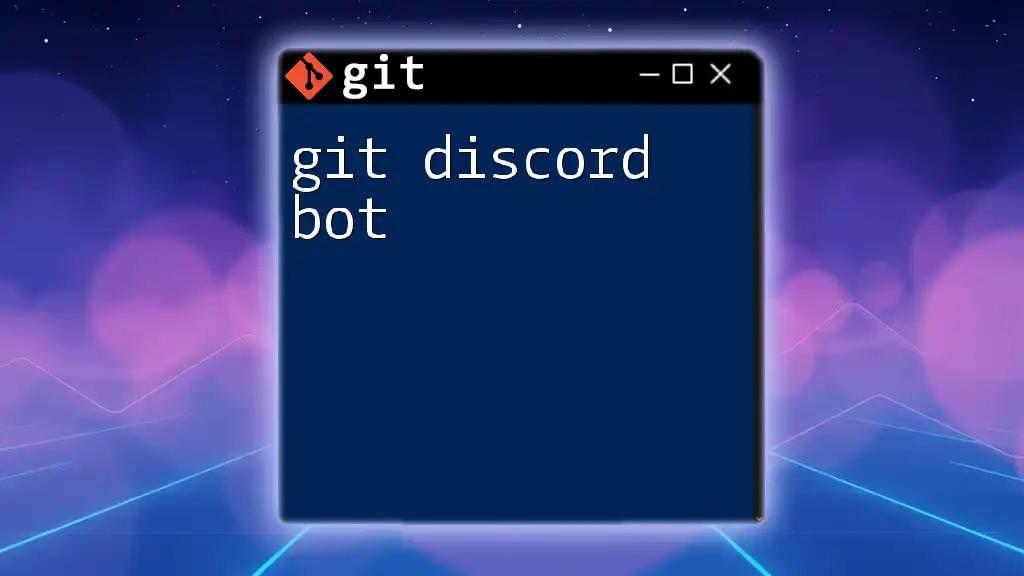
Conclusion
Understanding git history is fundamental for anyone working with version control, particularly in collaborative projects. By leveraging the tools Git provides to view, navigate, and manage commit history effectively, developers can enhance their workflows and maintain a clear understanding of their project's evolution. Practicing the commands and concepts discussed will lead to a more proficient use of Git, ultimately benefiting both individual projects and team collaborations.
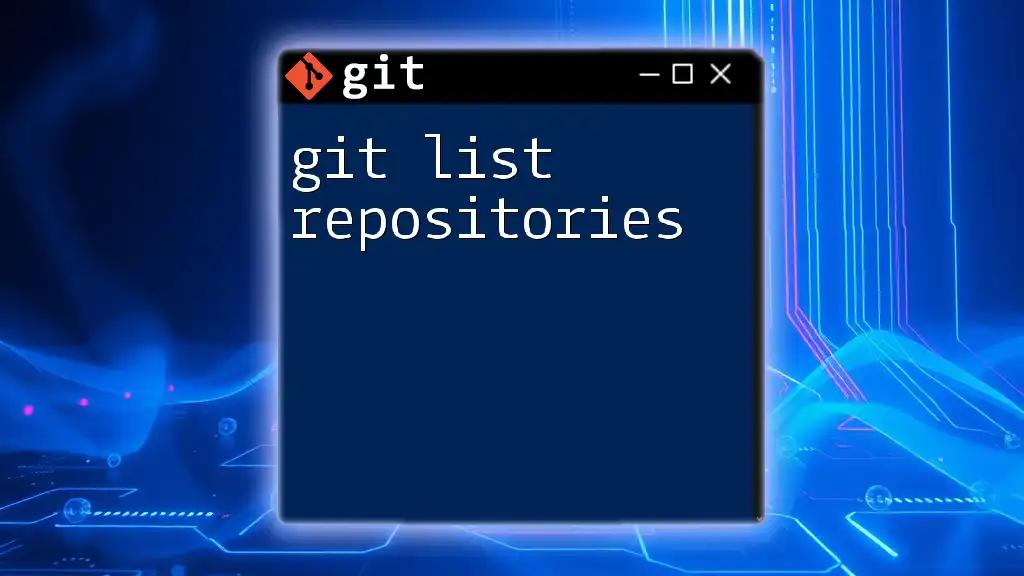
Additional Resources
For further reading, consider exploring the official Git documentation and other resources that focus on advanced Git techniques and best practices. Learning how to utilize Git efficiently will elevate your version control skills, allowing for smoother workflows and enhanced productivity.