A Git Discord bot is an automated tool integrated into a Discord server that can execute Git commands and provide responses to user queries related to version control.
Here's a basic example of how to use a Git command within a Discord bot made with JavaScript:
const { Client, GatewayIntentBits } = require('discord.js');
const { exec } = require('child_process');
const client = new Client({ intents: [GatewayIntentBits.Guilds, GatewayIntentBits.GuildMessages] });
client.on('messageCreate', message => {
if (message.content.startsWith('!git')) {
const command = message.content.slice(5); // Get the command after !git
exec(command, (error, stdout, stderr) => {
if (error) {
message.channel.send(`Error: ${stderr}`);
return;
}
message.channel.send(`Output: ${stdout}`);
});
}
});
client.login('YOUR_BOT_TOKEN');
This example listens for messages in Discord, checks if the message starts with `!git`, and processes the command using the `exec` function to interact with Git. Make sure to replace `'YOUR_BOT_TOKEN'` with your actual bot token.
Understanding Discord Bots
What is a Discord Bot?
A Discord bot is a programmable agent that automates tasks on Discord servers. These bots can execute a variety of functions, such as responding to user commands, managing communities, sending alerts, and integrating with other applications.
Discord bots extend the capabilities of Discord, making them indispensable for numerous use cases—whether it's moderating chat, providing game stats, or serving as a music player.
Why Use Git with Discord Bots?
Integrating Git into your Discord bot development provides a collaborative environment where multiple developers can work together seamlessly. Git’s version control means you can keep your code organized and track changes over time. You can easily revert to previous versions if something goes wrong and maintain a clear history of your modifications. This practice is vital for any serious development project.
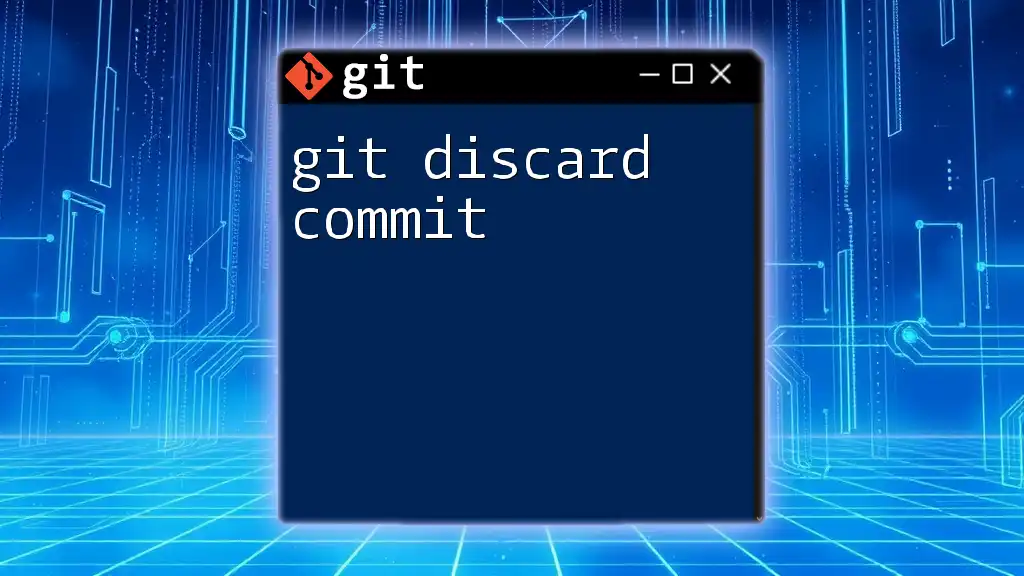
Setting Up Your Discord Bot
Creating a Discord Application
To get started, you need to create a Discord application. This is the first step in building your bot.
- Go to the [Discord Developer Portal](https://discord.com/developers/applications).
- Click on New Application.
- Name your application and hit Create.
This action generates an application that will hold your bot.
Adding a Bot to Your Application
Once your application is created, you can add a bot to it.
- Navigate to the Bot tab on the left side of the application settings.
- Click on Add Bot.
Now your bot is ready to be invited to your Discord server, which you can do by creating an invite link under the OAuth2 tab.
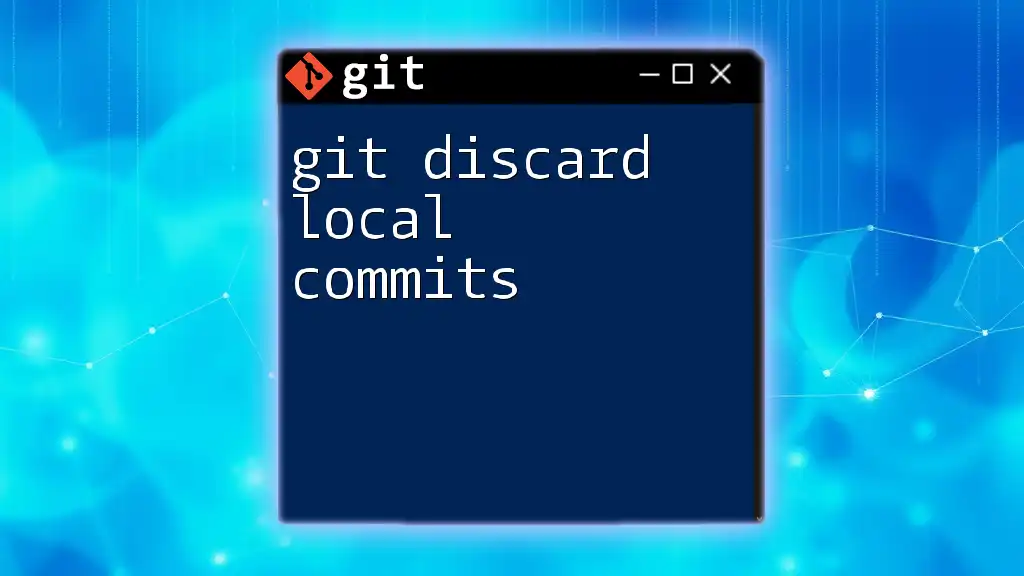
Initializing Your Git Repository
Installing Git
Before diving into your bot’s code, ensure you have Git installed on your computer. The installation steps will differ depending on your operating system:
# For Ubuntu
sudo apt-get install git
# For MacOS
brew install git
# For Windows
Download the installer from [Git's official website](https://git-scm.com/download/win)
Creating a New Repository
Once Git is installed, you can create a new Git repository for your Discord bot.
mkdir discord-bot
cd discord-bot
git init
This command initializes a new Git repository in your `discord-bot` folder.
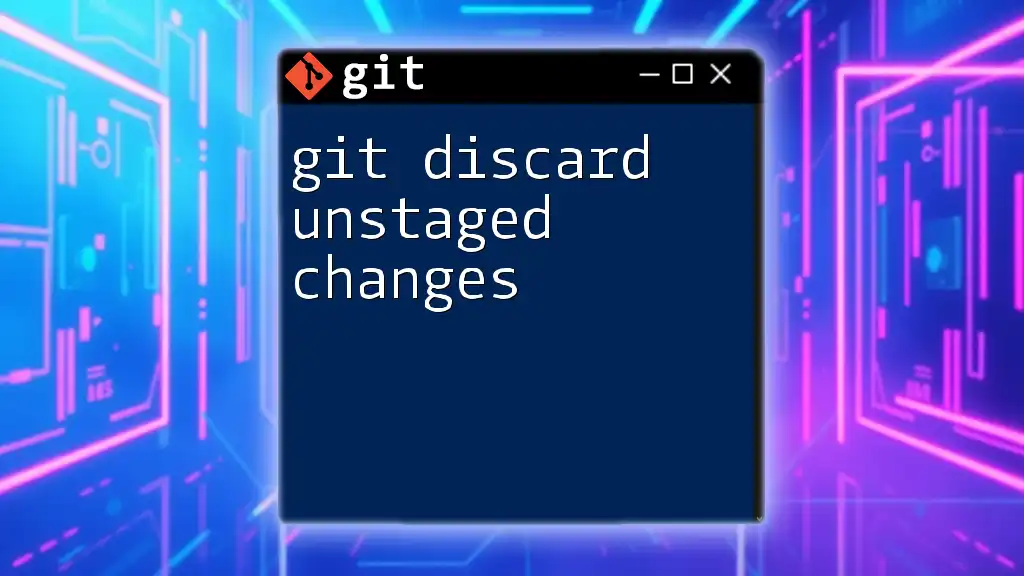
Coding Your Discord Bot
Setting Up Your Development Environment
To develop your Discord bot, you need to set up your development environment. Using Visual Studio Code is highly recommended due to its extensive features and support. Additionally, you will need Node.js installed on your system, as Discord bots are primarily written with JavaScript and Node.js.
Install the necessary packages using npm:
npm init -y
npm install discord.js dotenv
Writing Your First Bot Command
Now it’s time to write some code! Let’s create a simple Hello World bot that responds to a greeting command.
Here’s a basic example:
const Discord = require('discord.js');
const client = new Discord.Client();
client.on('message', message => {
if (message.content === '!hello') {
message.reply('Hello there!');
}
});
client.login('YOUR_BOT_TOKEN');
This code sets up a client that listens for messages on the server. When a user sends `!hello`, the bot replies with Hello there!. Ensure to replace `'YOUR_BOT_TOKEN'` with your actual bot token from the Discord Developer Portal.
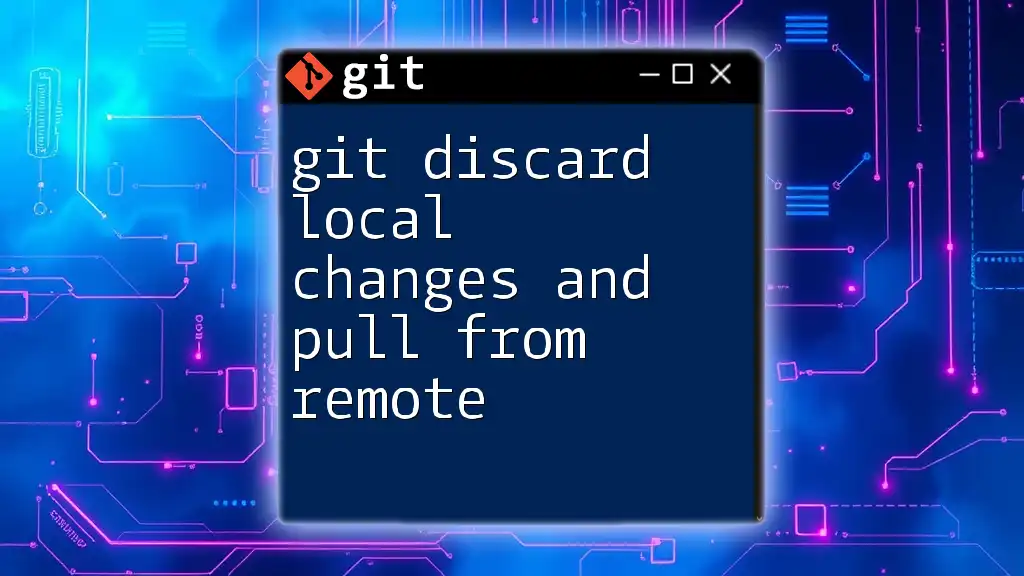
Version Control with Git
Committing Your Code
As you make changes to your bot, it’s essential to commit those changes in Git. This allows you to keep a history of your modifications.
To stage your changes, use the following commands:
git add .
git commit -m "Initial commit: Added hello world command"
The `git add .` command stages all the changes in your directory, while `git commit -m "message"` saves those changes with a meaningful message.
Creating Branches for Development
Branching is a vital aspect of using Git effectively. It allows you to work on features or fixes independently without affecting the main codebase.
To create and switch to a new branch, use:
git checkout -b feature/add-goodbye-command
Merging Branches
Once you've tested your feature on the new branch and are ready to incorporate it into the main codebase, you can merge the branches. Switch back to the main branch and execute:
git checkout main
git merge feature/add-goodbye-command
This way, you'll have a clean, organized Git history, making collaboration simpler and more efficient.
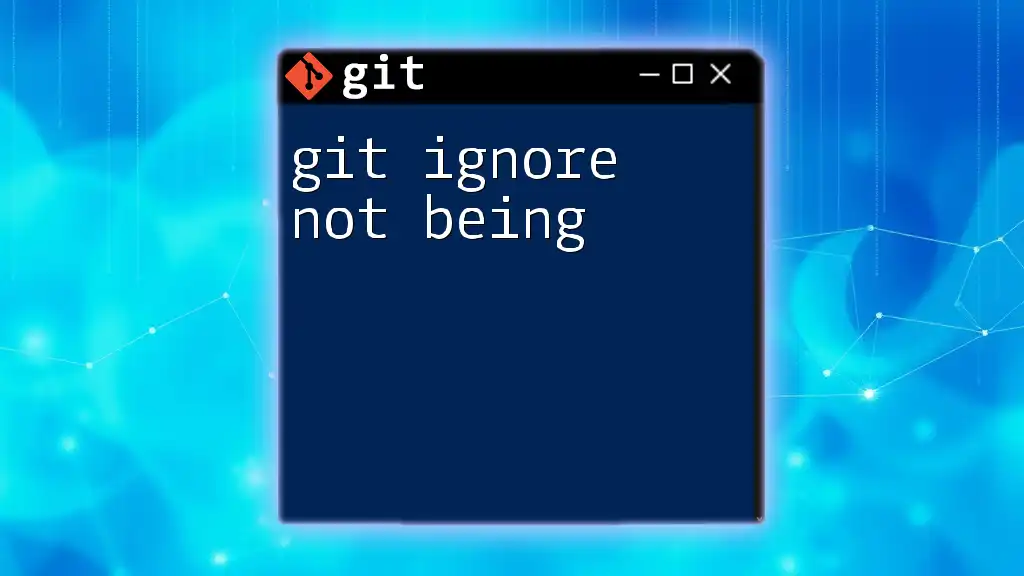
Hosting Your Discord Bot
Choosing a Hosting Service
After writing your bot, the next step is to host it. Several platforms are available, such as Heroku, DigitalOcean, and Repl.it. Each has its own benefits and drawbacks:
- Heroku is easy to use for beginners and offers generous free-tier hosting.
- DigitalOcean provides more control over your environment but requires a bit more configuration.
- Repl.it simplifies the process with online coding but may have limitations in terms of longer-running processes.
Deploying Your Bot
Let’s say you choose Heroku for hosting. Here’s how to deploy your bot:
- First, install the Heroku CLI and log in.
- In your bot directory, create a new Heroku app:
heroku create my-discord-bot
- Push your code to Heroku:
git push heroku main
Your bot is now live on Discord!
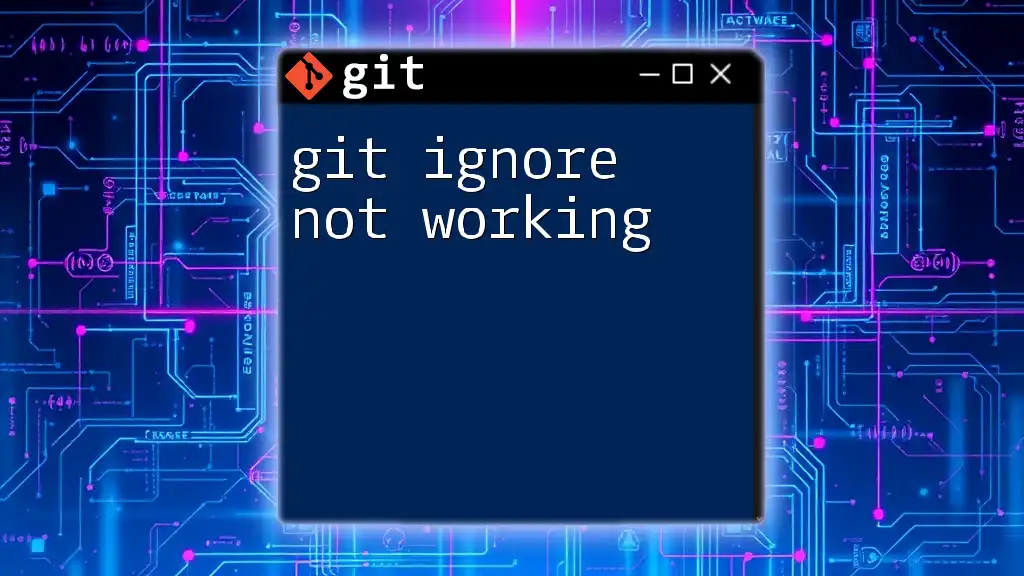
Conclusion
Creating a git discord bot is a rewarding experience that combines programming skills with the power of Git for version control. As you familiarize yourself with these tools, don’t hesitate to experiment with more advanced commands and features. Each step you take not only enhances your skills but also contributes to a larger community of developers.
Remember, the journey of developing a bot is filled with opportunities to learn, create, and innovate. Share your experiences, and continue exploring the exciting world of Discord bots!