If your `.gitignore` file is not ignoring the `node_modules` directory, make sure that the directory is correctly specified and that it is not already tracked by Git; you can add the following line to your `.gitignore` file to ignore it, and use the command to remove it from tracking if it was previously added:
echo 'node_modules/' >> .gitignore
git rm -r --cached node_modules/
Understanding .gitignore
What is .gitignore?
The `.gitignore` file is a crucial component of any Git repository. It specifies files and directories that Git should ignore—meaning they won’t be tracked or included in commits. This can help keep your repository clean and focused by excluding unnecessary files, especially in programming projects where large directories like `node_modules` can be created.
Structure of .gitignore
The `.gitignore` file consists of simple newline-separated entries. Each line represents a pattern that git will use to determine which files to ignore. Here’s a very basic example of a `.gitignore` file:
# Ignore node_modules folder
node_modules/
In this example, the `node_modules/` entry tells Git to ignore the entire `node_modules` directory, which is where Node.js packages are stored.
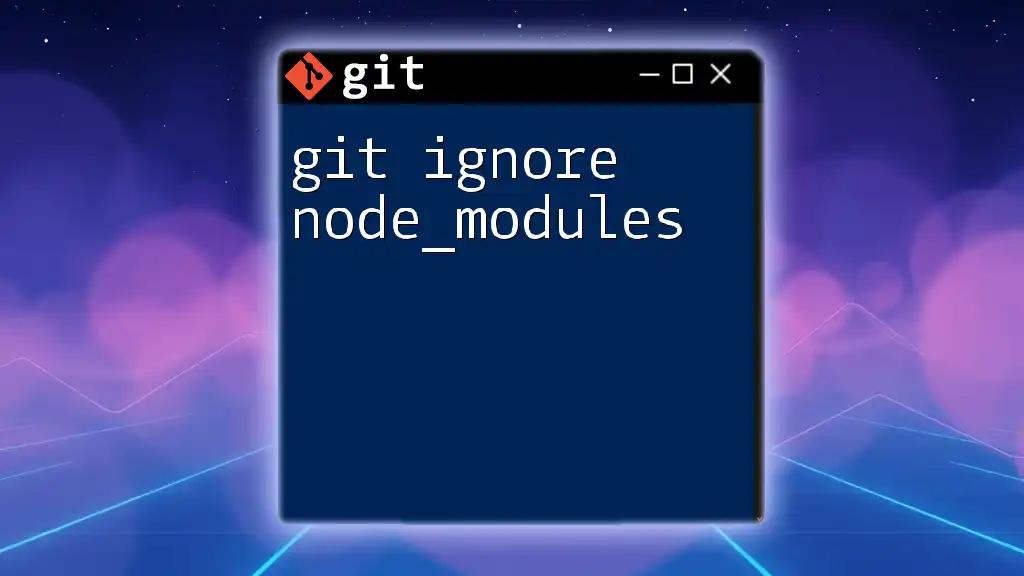
Why Node_Modules is Not Ignored
Common Reasons for Node_Modules Remaining in Git
Incorrect .gitignore syntax
One of the most frequent issues with `.gitignore` is incorrect syntax. For instance, if the file doesn’t contain the proper path or has typos, Git will continue tracking those files.
A common mistake might look like this:
node_modules
Without the trailing slash, this entry will not effectively ignore the directory. Always remember to include the trailing slash when you want to ignore a directory.
Node_Modules already tracked
Even if your `.gitignore` is correctly set up, it’s possible that the `node_modules` folder was already tracked in your repository. Once tracked, a file or folder will remain in the Git index unless explicitly removed.
You can check if `node_modules` is tracked by running the following command:
git ls-files | grep node_modules
If you see output that includes files from the `node_modules` directory, it means those files are currently being tracked.
How to Identify if Node_Modules is Tracked
To confirm that `node_modules` is indeed being tracked, use the `git status` command to see the status of your working tree:
git status
Additionally, you can use `git check-ignore` to identify specific files that should have been ignored but are not:
git check-ignore -v node_modules/somefile.js
This command reveals why a particular file isn’t being ignored, providing valuable context for debugging.
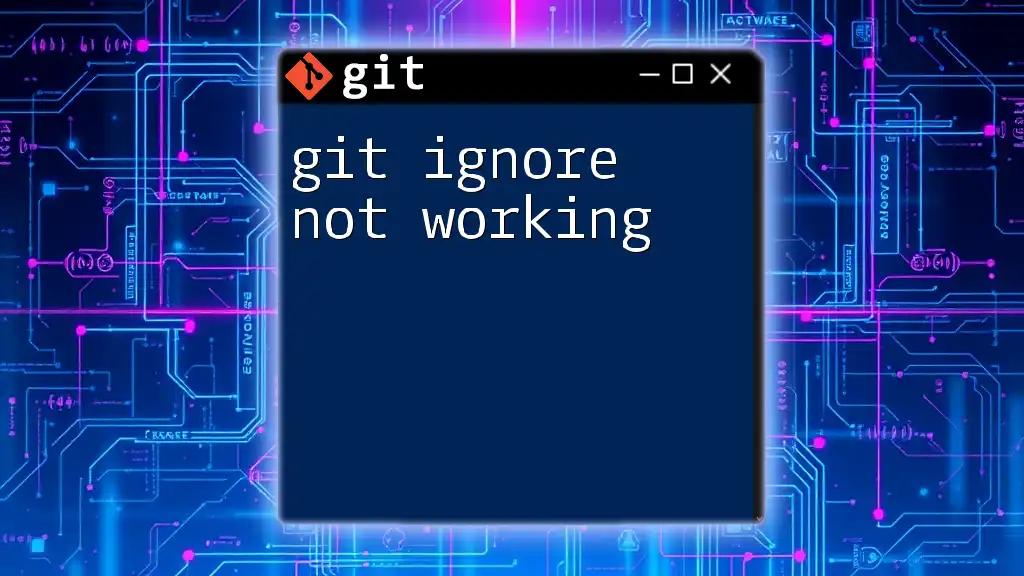
Configuring .gitignore Properly
Correctly Ignoring Node_Modules
To ensure that your `node_modules` folder is correctly ignored, you should include an entry in your `.gitignore` file that looks like this:
node_modules/
This properly ignores the entire directory and any files it contains.
Common Mistakes to Avoid
Several key oversights can lead to issues when trying to ignore the `node_modules` folder:
-
Not including a trailing slash: As previously mentioned, forgetting the trailing slash will prevent the folder from being ignored completely.
-
Multiple .gitignore files: If your project has multiple `.gitignore` files, ensure you know which one is in effect. Each file applies to its directory and its subdirectories, potentially causing confusion. For clarity, consider consolidating your ignore rules into a single file at the root of your repository.
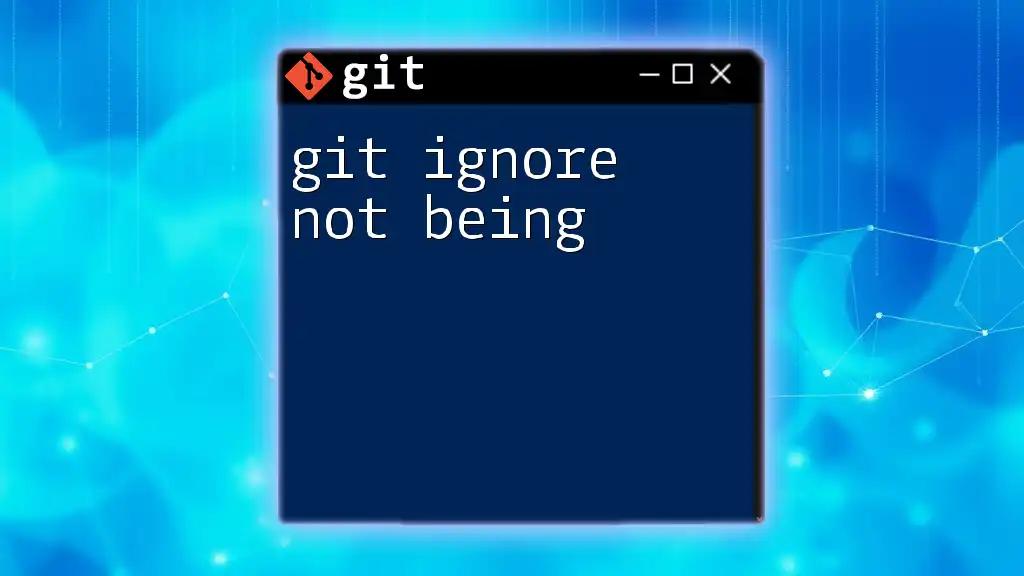
Removing Node_Modules from Git Tracking
Steps to Untrack Node_Modules
If your `node_modules` folder is already being tracked, you need to stop tracking it. You can do this using the following command:
git rm -r --cached node_modules
This command removes the folder from the Git index without deleting it from your filesystem, allowing you to keep your installed packages intact.
Committing the Changes
After untracking `node_modules`, you will need to commit these changes to finalize the process. Use a clear commit message to reflect the update:
git commit -m "Remove node_modules from tracking"
This makes it evident to anyone reviewing the repository history what changes were made.
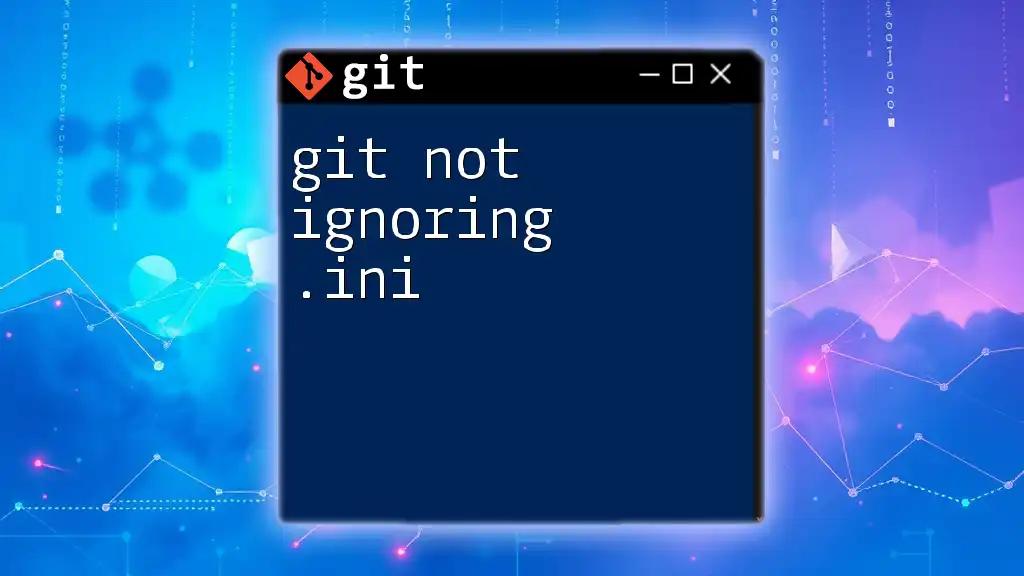
Ensuring Node_Modules is Ignored in Future Commits
Verifying .gitignore Functionality
After you’ve configured the `.gitignore` file and untracked `node_modules`, it’s essential to verify that everything is functioning as intended. Run:
git status
You should not see `node_modules` listed anymore. Additionally, running `git check-ignore` can provide further assurance:
git check-ignore -v node_modules/somefile.js
If everything is set up correctly, you should see output confirming that these files are, in fact, being ignored.
A Pro Tip for Node.js Projects
Consider automating the ignore process when setting up new Node.js projects. You can use templates containing a pre-configured `.gitignore` file, which includes defaults for ignoring `node_modules` along with other common entries.
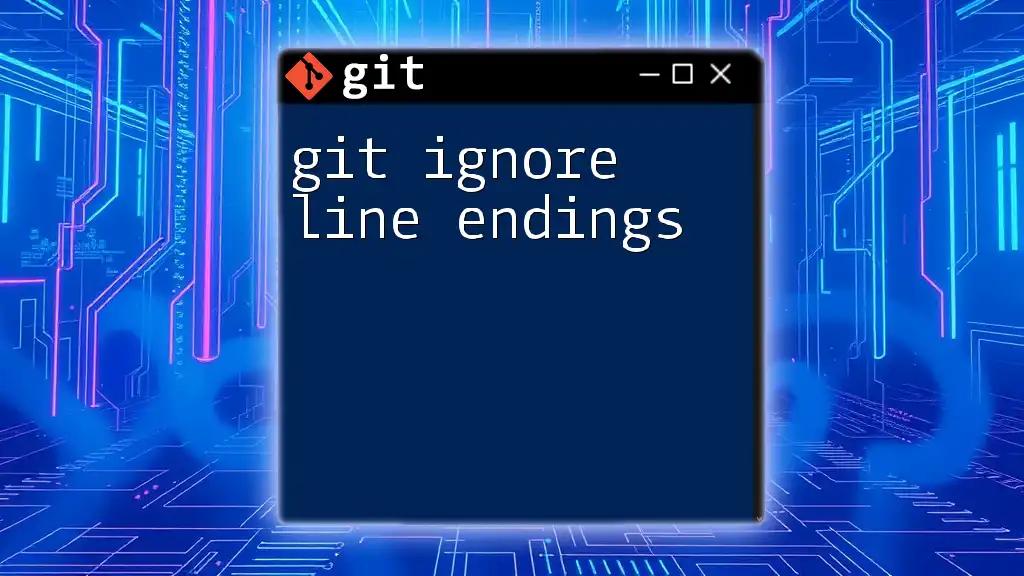
Troubleshooting .gitignore Issues
Debugging .gitignore
If you still find that `node_modules` isn’t being ignored, utilize verbose output to pinpoint the problem. Running the command:
git check-ignore -v node_modules/somefile.js
will detail exactly why the file is being tracked, helping you identify if there's a conflicting rule or path issue in your `.gitignore` file.
Resources for Further Learning
For those looking to dive deeper into `.gitignore` usage, refer to the official Git documentation. Additionally, there are several online tools and plugins designed to create and manage `.gitignore` files easily, which can streamline the process.
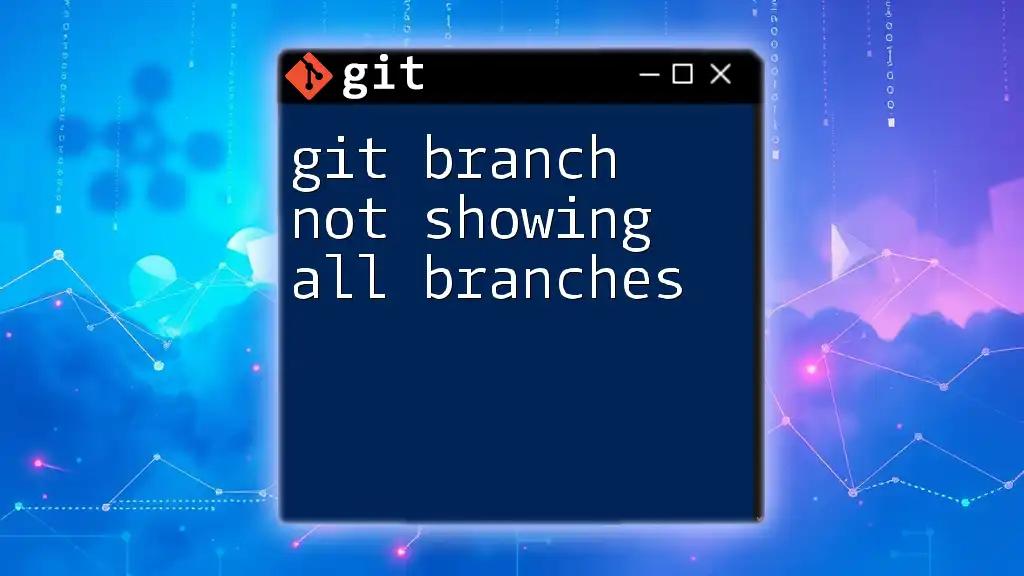
Conclusion
Summary of Key Points
To recap, ensuring that `node_modules` is ignored by Git requires proper syntax in your `.gitignore`, untracking any already-tracked files, and verifying that your ignore settings are functioning correctly.
Call to Action
Implement these strategies in your projects to avoid unnecessary clutter in your repositories. If you have any questions or would like to share your experiences, feel free to leave a comment below!