When using Git, you can prevent issues related to inconsistent line endings across different operating systems by configuring a `.gitattributes` file to specify how line endings are handled for text files.
Here's the code snippet you can include in your post:
# Create or edit the .gitattributes file to manage line endings
echo "* text=auto" > .gitattributes
Understanding Git and Line Endings
What Are Line Endings?
Line endings refer to the characters or sequences of characters used to signify the end of a line of text in files. The two most commonly used types of line endings are:
- LF (Line Feed): Used mainly in UNIX and UNIX-like systems, including Linux and macOS. Represented as `\n`.
- CRLF (Carriage Return + Line Feed): Used primarily in Windows environments. Represented as `\r\n`.
Understanding the distinction between these line endings is crucial because different programming environments or editors might handle these characters differently. Even a small discrepancy can lead to errors during version control operations, particularly when collaborating across different operating systems.
Why Line Endings Matter in Git
In the context of Git, line endings play a vital role in ensuring seamless collaboration and version control. When files are shared among developers using different operating systems, inconsistent line endings can lead to several issues:
- Merge Conflicts: If two developers working on different platforms modify the same file, the line endings may differ, causing Git to perceive the changes as conflicts.
- Polluted Version History: Different line endings can clutter the commit history with unnecessary changes, making it harder to track actual code revisions.
- Code Quality Assurance: Inconsistent line endings can lead to unexpected behavior in applications, especially if the code relies on specific formatting.
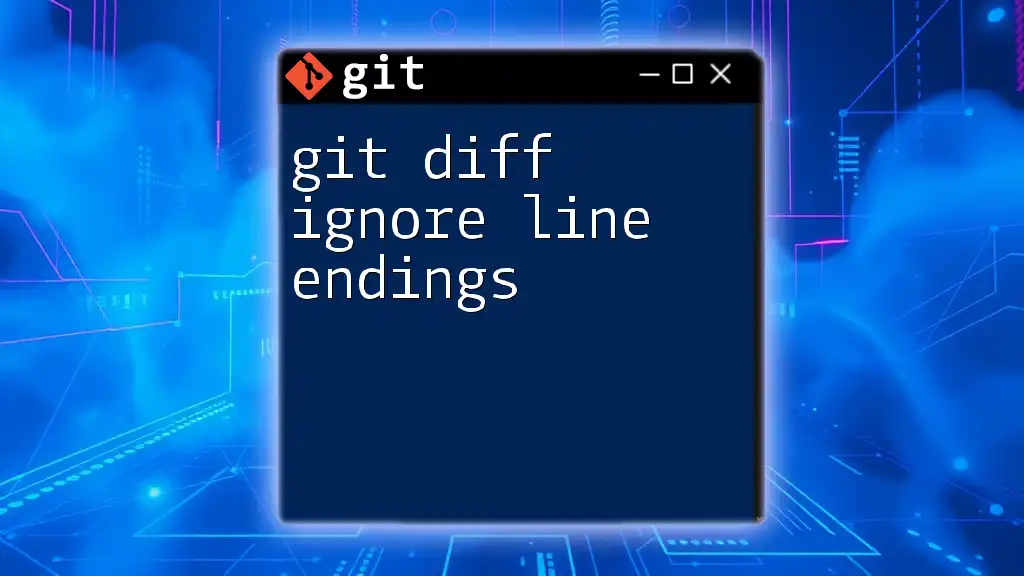
Setting Up Your .gitignore for Line Endings
The Purpose of a .gitignore File
A `.gitignore` file serves as a vital tool for specifying files and directories that Git should ignore. This is particularly useful for files that do not need to be tracked, such as temporary files, build artifacts, and files generated by the editor.
Configuring Line Endings in Your .gitignore
When configuring line endings in your project, it's essential to ensure that files with problematic line endings are ignored. To set this up, you can create or modify your `.gitignore` file:
# Ignore all files with CRLF line endings
*.log
*.tmp
*.bak
This will ensure that any files with CRLF line endings (which might be introduced unintentionally) do not contaminate your repository.
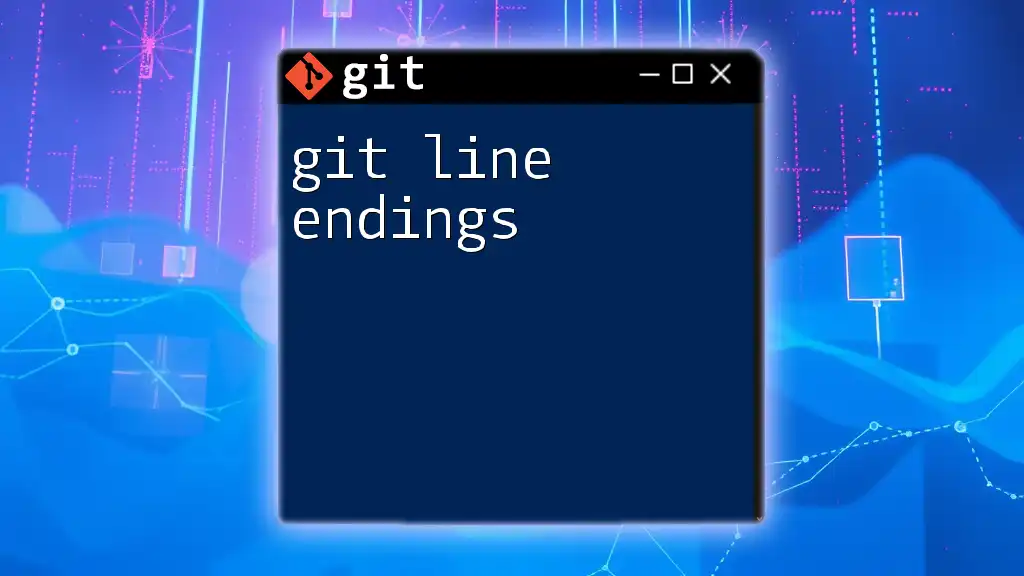
Managing Line Endings with Git Configuration
Core Autocrlf Setting
Git offers a feature known as `core.autocrlf` that allows you to manage line endings automatically depending on your operating system. This setting converts line endings as files are checked in and out of the repository.
- For Windows users, set it to `true`:
git config --global core.autocrlf true
- For macOS and Linux users, use:
git config --global core.autocrlf input
Setting `core.autocrlf` to true on Windows converts LF to CRLF upon checkout and CRLF back to LF upon commit, allowing for seamless transitions between different environments.
Checking Current Configuration
To verify your current `core.autocrlf` setting, you can run:
git config --get core.autocrlf
This command helps ensure that your settings are correct, ultimately preventing line ending issues in your codebase.
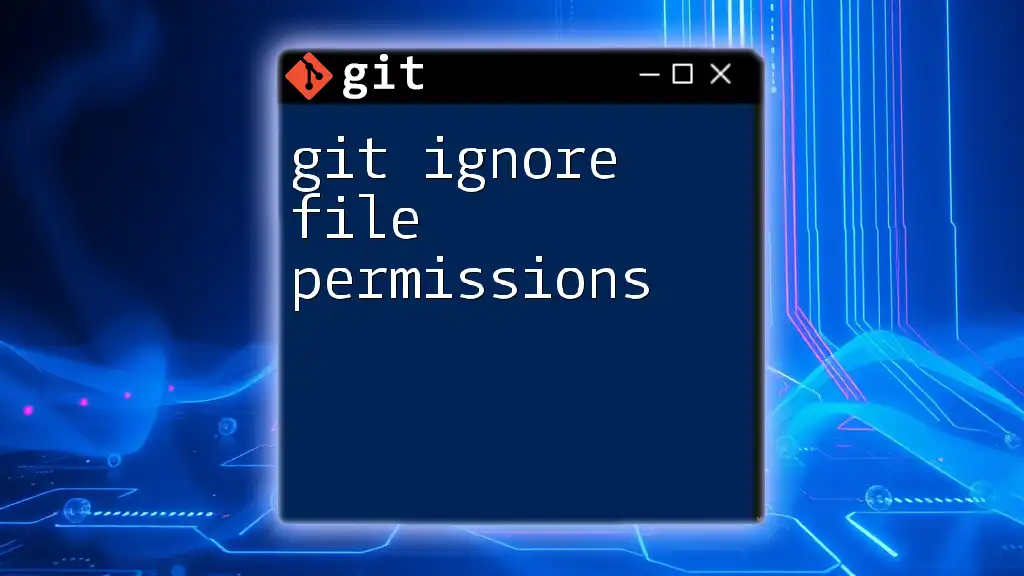
Normalizing Line Endings in Repositories
The Importance of Normalization
Normalization involves standardizing line endings across your project files to prevent inconsistencies and potential issues. Mixed line endings can cause confusion for both developers and the tools they use, leading to unnecessary troubleshooting.
Steps to Normalize Line Endings
One effective way to enforce normalization is through the `.gitattributes` file, which specifies how files are treated in terms of line endings. Including a `.gitattributes` file in your project can be a game-changer.
A simple configuration would be as follows:
* text=auto
Adding this line tells Git to treat all text files as having uniform line endings, ultimately converting CRLF to LF on commit.
Running the Normalization Process
Once you've set up the `.gitattributes` file, you may need to run the normalization process to enforce it across your repository. You can do this by executing:
git add --renormalize .
git commit -m "Normalize line endings"
This command will reapply the normalization and update your commit history to reflect consistent line endings.
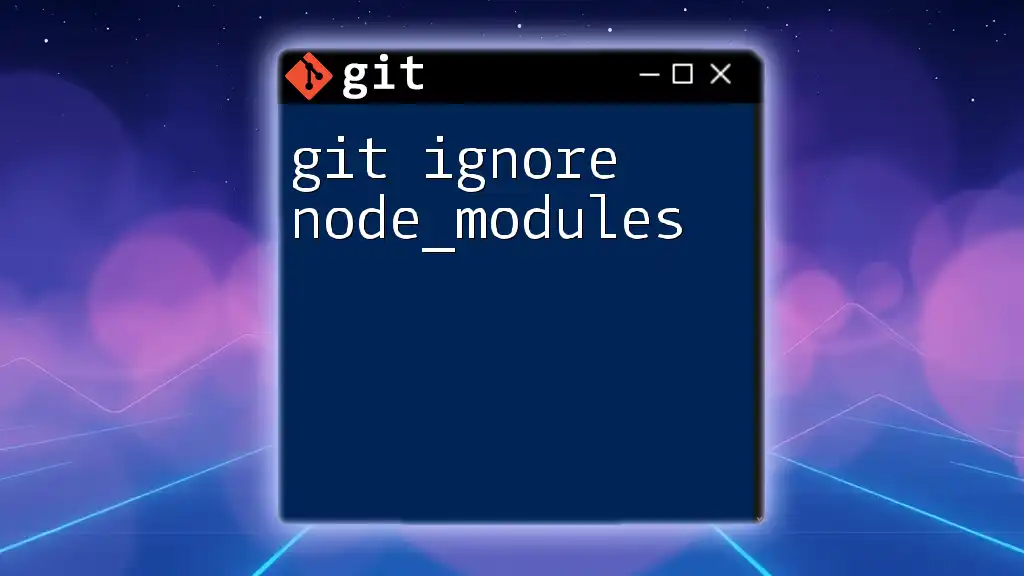
Common Pitfalls and Troubleshooting
Common Issues with Line Endings in Git
Despite taking all precautions, line ending problems may still arise, including:
- Merge Conflicts due to inconsistent line endings between different developers.
- Files Showing Differences that actually have no substantive changes other than varied line endings.
Troubleshooting Tips
If you encounter line ending issues, diagnosing the problem is the first step. You can resolve conflicts or restore line endings with commands like:
git checkout -- <file>
This command retrieves the last committed version of the file, helping to fix unintended changes related to line endings.
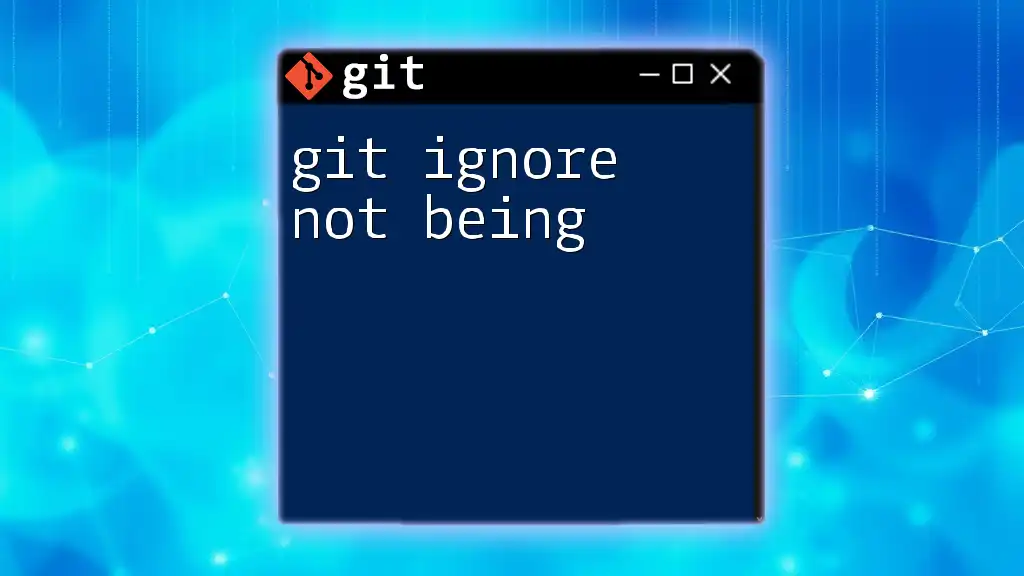
Best Practices for Managing Line Endings with Git
Recommended Practices
To ensure consistent line endings within your project, adopt the following best practices:
- Always Use a .gitattributes File: Consistently track line ending configurations per file type.
- Collaborate with Team: Make it a standard practice among all team members to configure Git settings regarding line endings correctly.
- Regular Reviews: Periodically check line ending configurations to maintain consistency, especially before major commits or releases.
Regular Maintenance and Verification
Establish a routine to check line endings in your repository, especially as new files are added. Commands such as `git diff` can help identify issues before they escalate into conflicts or errors, keeping your project clean and functional.
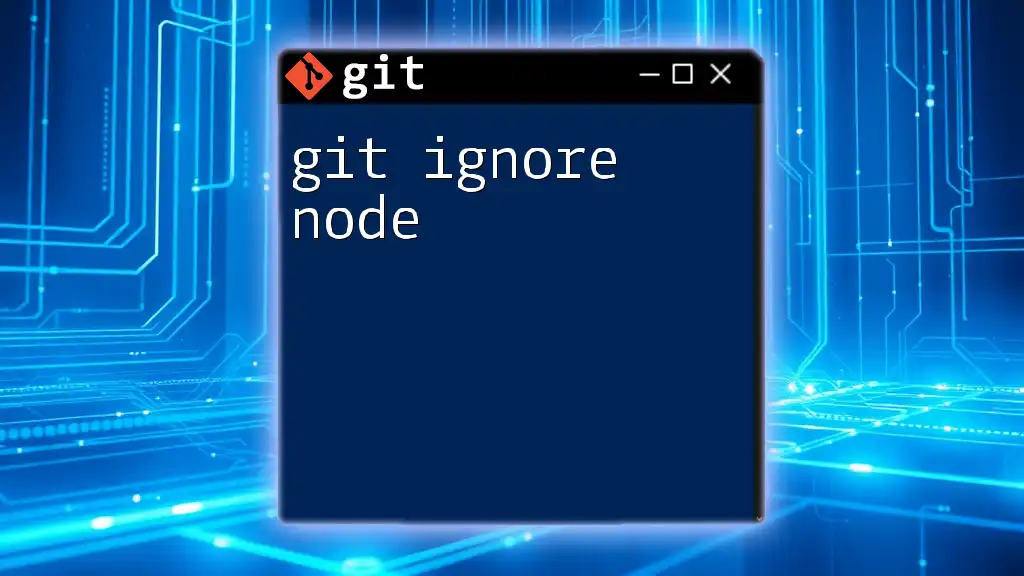
Conclusion
In summary, managing line endings in Git is essential for ensuring the quality and consistency of your codebase. From utilizing `.gitignore` and `.gitattributes` files to setting the correct `core.autocrlf` configurations, taking these steps helps prevent common pitfalls associated with line endings. By embracing these practices, you'll not only streamline collaboration among team members but also maintain the integrity of your version-controlled projects. Embrace the power of Git and safeguard your developers from the woes of line ending issues!