In Git, a `.gitignore` file is used to specify which files or directories should be excluded from version control, such as environment files that contain sensitive information, preventing them from being committed to the repository.
Here's a code snippet to create a `.gitignore` file that ignores environment files:
echo ".env" >> .gitignore
What is Git?
Git is a powerful version control system widely used for tracking changes in source code during software development. It allows multiple developers to collaborate on a project, manage different versions of their code, and ensure that changes are documented and reversible. Understanding Git is essential for modern development workflows, and mastering commands can significantly enhance productivity and teamwork.
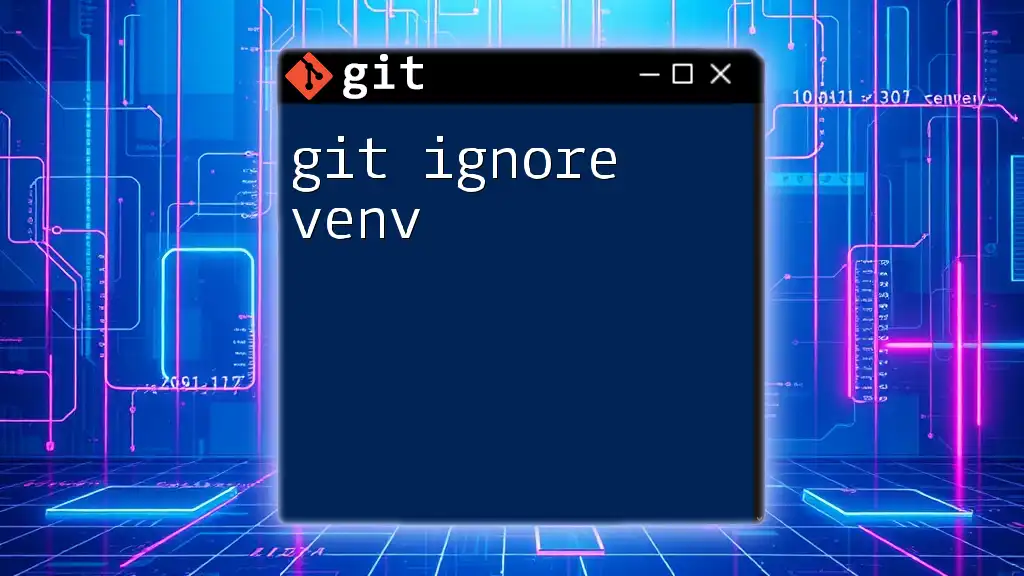
Understanding Environment Files
Environment files, often named `.env`, are crucial for managing application configurations and sensitive information, such as API keys, database credentials, and other secrets needed for a project to run. These files are typically used in local development environments to configure how software behaves without hardcoding sensitive information into the source code.
Importance of Security: Storing sensitive information directly in repositories can lead to security vulnerabilities. If a repository is inadvertently made public or shared without proper precautions, sensitive data could be exposed, leading to potential data breaches, identity theft, and other security issues.
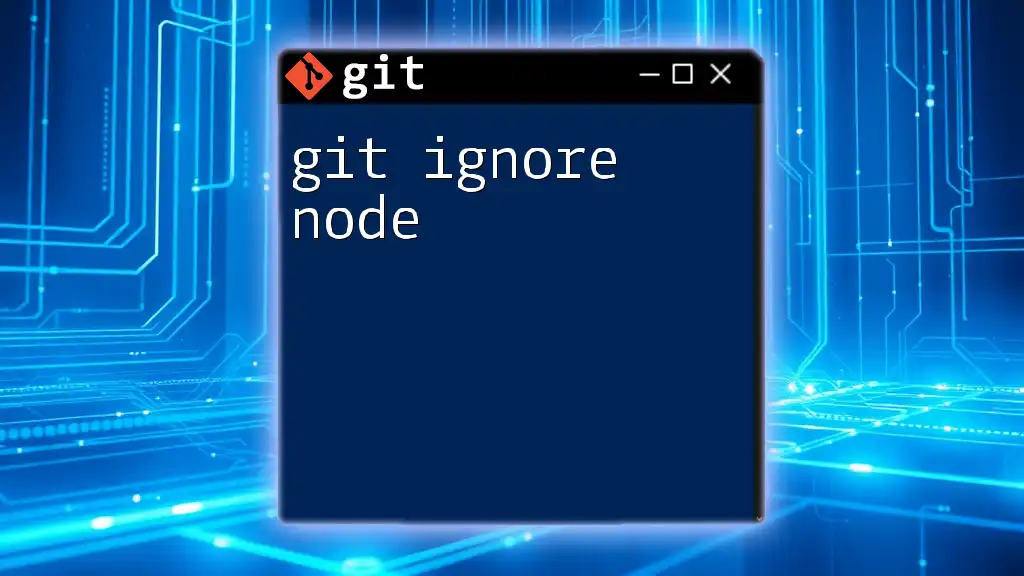
What is `.gitignore`?
Definition of `.gitignore`
The `.gitignore` file tells Git which files or directories to intentionally exclude from version control. This is particularly useful for avoiding unnecessary files—like build artifacts, logs, or personal configurations—from being committed to the repository, keeping the project clean and secure.
General Syntax of `.gitignore`
Entries in a `.gitignore` file follow a specific syntax. Here are a few important points:
- Blank lines or lines starting with `#` are ignored (these can be used for comments).
- `*` matches any string of characters.
- `/**/` matches directories recursively.
This flexibility allows you to specify which files or patterns to ignore effectively.
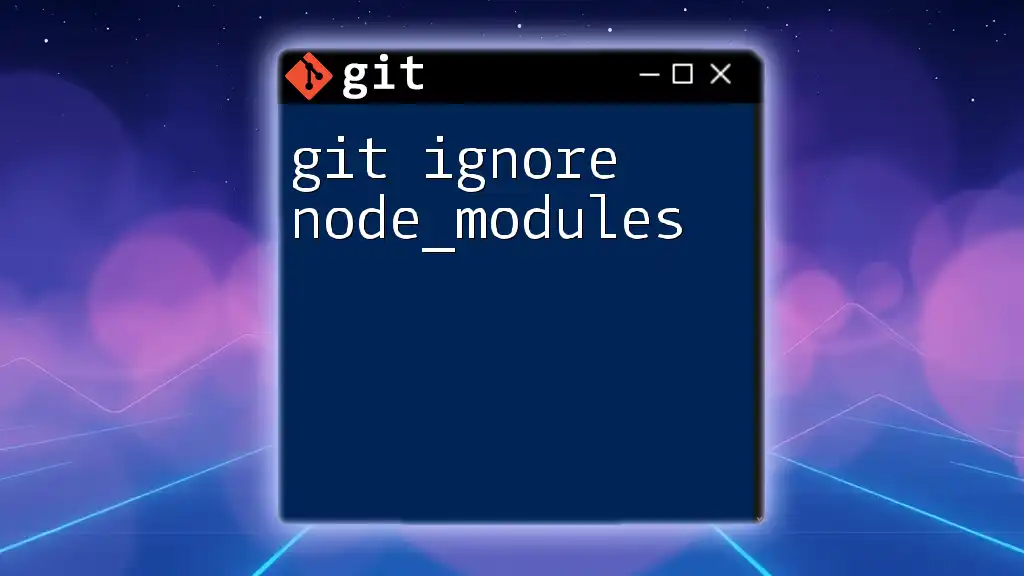
Ignoring `.env` Files
Why Ignore `.env` Files?
Ignoring `.env` files is essential for protecting sensitive information from being included in version control. If you track a `.env` file, you risk exposing secrets whenever you share the repository or collaborate with others.
Benefits of Ignoring `.env` Files:
- Protect sensitive data from unauthorized access.
- Simplify collaboration by ensuring developers use their own local configurations instead of sharing sensitive information.
- Facilitate easier onboarding of new team members without the risk of exposing credentials.
Creating a `.gitignore` File
To create a `.gitignore` file in your project directory, you can either use the command line or create the file manually. The command to create a new `.gitignore` file is:
touch .gitignore
Once the file is created, you need to specify which files to ignore.
Ignoring Specific `.env` Files
To prevent tracking of your `.env` file, add the following line to your `.gitignore`:
# Add this line to your .gitignore file
.env
With this entry, Git will no longer track changes to the `.env` file, ensuring that it remains protected from unwanted exposure.
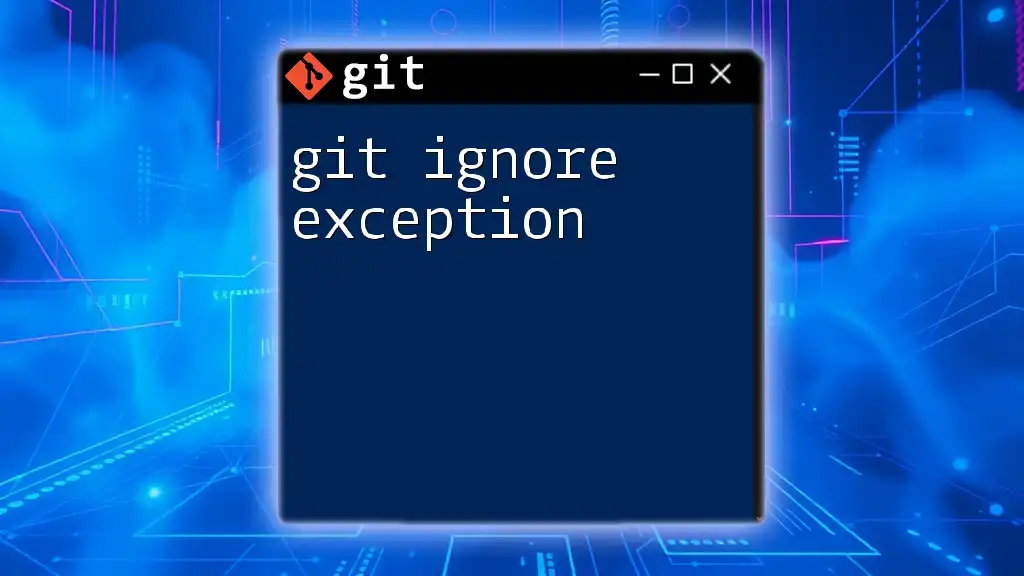
Best Practices for Managing `.env` Files
Use a Sample `.env` File
To assist other developers in setting up their local environment, create a sample file named `.env.example` or `.env.sample`. This file should contain the same keys as your production `.env` but without actual sensitive values.
Example of a `.env.sample` file:
# .env.sample
DB_HOST=localhost
DB_USER=root
DB_PASS=password
This approach allows team members to reference the necessary configuration without exposing actual credentials.
Handling Local and Production Environment Variables
Managing secrets and configurations between development and production environments can be tricky. Use environment variable management tools like dotenv or services like AWS Secrets Manager to keep your keys secure and easily configurable for different environments.
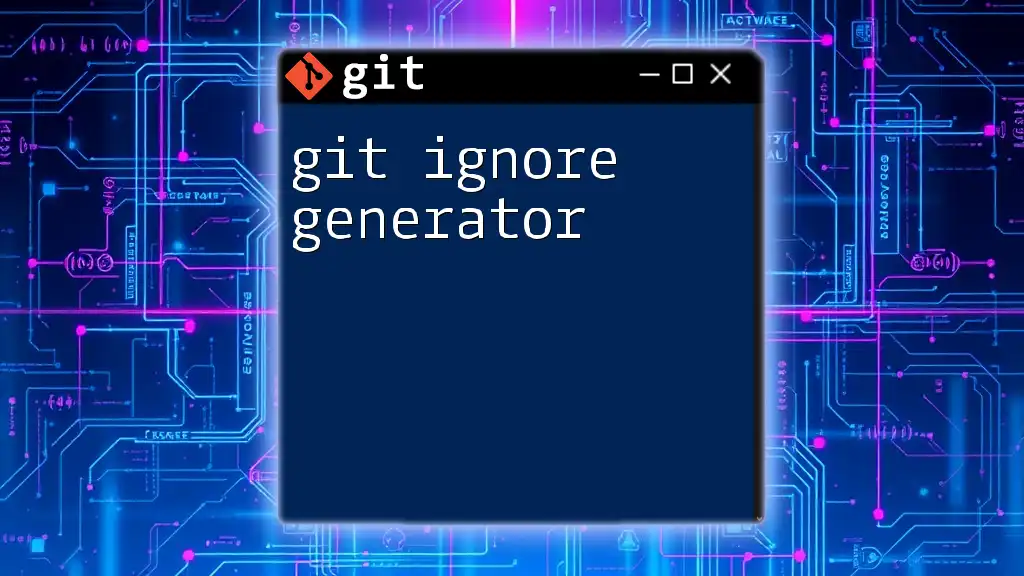
Common Pitfalls and Solutions
Files Already Tracked
If you have already committed your `.env` file to Git, it will continue to be tracked despite your changes in `.gitignore`. To untrack the file without deleting it, use the following command:
git rm --cached .env
After running this command, remember to update your `.gitignore` file to ensure it is no longer tracked in the future.
Ignoring Other Sensitive Files
Besides `.env` files, consider adding other sensitive or unnecessary files to your `.gitignore`. Examples include:
# Ignore Node.js specific files
node_modules/
npm-debug.log
# Ignore configuration files
config/config.yml
This practice helps streamline your repository by controlling what gets committed and shared.
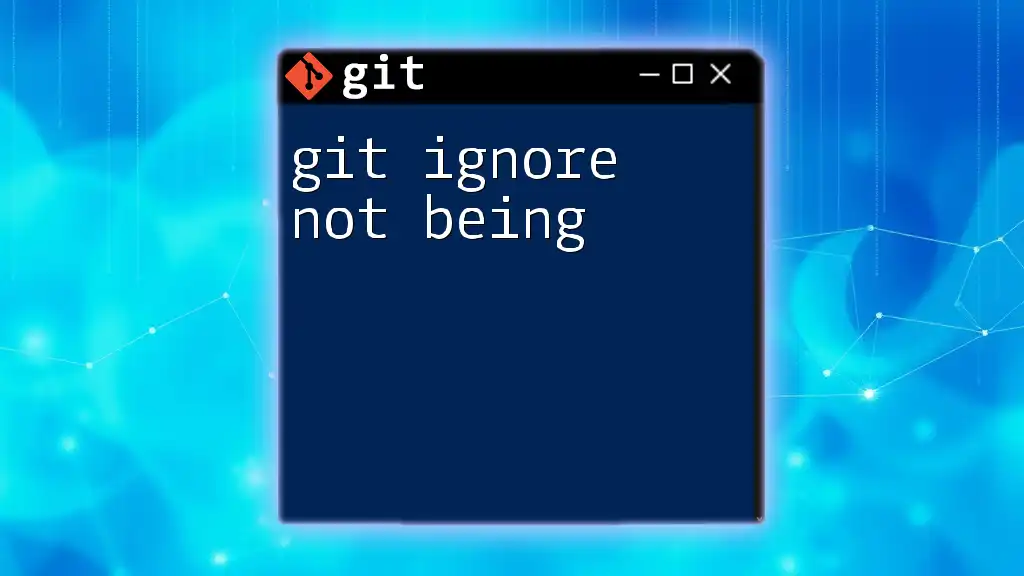
Conclusion
In summary, understanding how to configure a `.gitignore` file for environment files is fundamental for maintaining security and clarity in your Git repositories. Protecting sensitive data like API keys and configurations is crucial for a seamless and secure development process. Regularly reviewing and updating your `.gitignore` will facilitate a cleaner workflow and safeguard your team’s sensitive information.
Take charge of your security practices today—make sure your `.env` files are safely ignored! If you have experiences or questions about managing `.env` files with Git, feel free to share in the comments, and check out additional resources for even deeper insights into mastering Git.