In Git, a `.gitignore` file is used to specify files or directories that should be ignored by version control, while a "git ignore exception" allows you to track a previously ignored file by using an exclamation mark (`!`) before its path in the `.gitignore` file.
Here's an example of how to set an ignore exception:
# .gitignore
*.log # Ignore all .log files
!important.log # Exception: Do not ignore important.log file
What is a Git Ignore Exception?
A Git Ignore Exception refers to the ability to include specific files or directories in tracking even when they would normally be ignored by the rules set in your `.gitignore` file. Understanding exceptions is crucial for managing your Git repositories effectively, especially when you want to maintain selective control over the files being tracked.
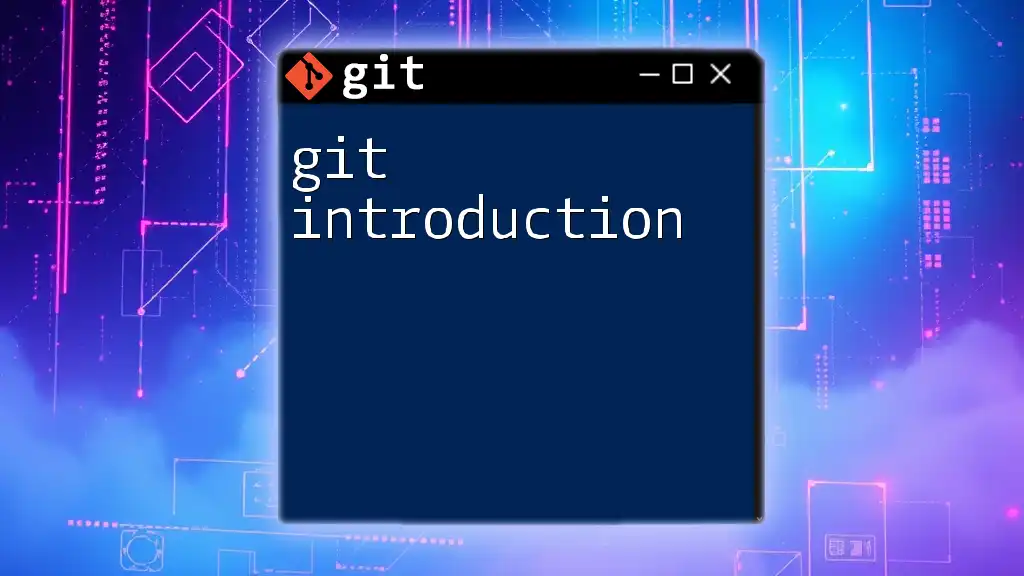
How to Create a .gitignore File
Creating a `.gitignore` file starts typically with a basic understanding of its syntax. The `.gitignore` file is a simple text file that tells Git which files or patterns to ignore in your project directory.
Basic Syntax of .gitignore
The syntax of a `.gitignore` file is straightforward. Each line contains a pattern that Git will use to determine whether to ignore certain files. Here’s an example of a simple `.gitignore` file:
# Ignore all .log files
*.log
In this example, any file ending with the `.log` extension will be ignored by Git.
Common Patterns for Ignoring Files
You can ignore various types of files using specific patterns:
- Ignoring file types: Use an asterisk () to denote file types you want to ignore. For instance, the pattern `.log` will ignore all log files.
- Ignoring directories: Adding a trailing slash (/) denotes that you want to ignore a directory. For example, `node_modules/` will ignore the entire `node_modules` folder.
Here’s a quick reference for a few common patterns:
# Ignore all log files
*.log
# Ignore node modules directory
node_modules/
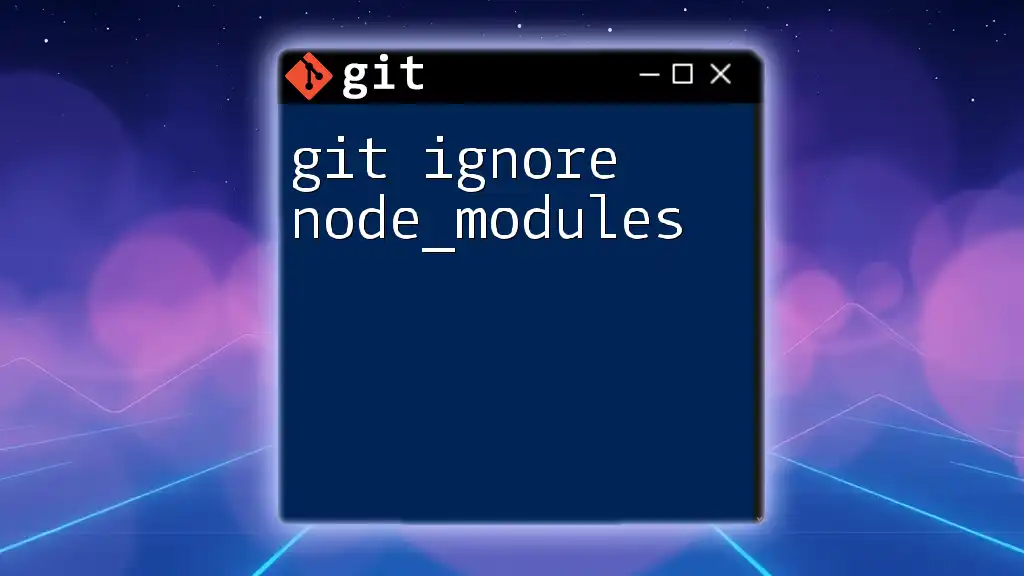
Adding Exceptions to Git Ignore
What is an Exception?
When working with `.gitignore`, you may need to include certain files that would otherwise be ignored. This is where git ignore exceptions come into play. They allow you to specify exceptions to the rules set in your `.gitignore` file.
Syntax for Exceptions
To create an exception, prefix the file or directory you want to include with an exclamation mark (!). Here is an example demonstrating how to include an exception in your `.gitignore`:
# Ignore all .log files except for development.log
*.log
!development.log
In this case, all log files will be ignored, except for `development.log`, which will be tracked by Git.
Understanding the Order of Rules
Understanding the order of your rules in the `.gitignore` file is essential. Git processes the rules in the order they appear in the file, meaning that if you have a general ignore rule followed by an exception, the exception will take precedence.
Consider this example:
# Ignore all files in the temp directory
temp/*
# Don't ignore temp/readme.txt
!temp/readme.txt
Here, `temp/*` will ignore all files in the `temp` directory, but the exception `!temp/readme.txt` ensures that `temp/readme.txt` is still tracked.
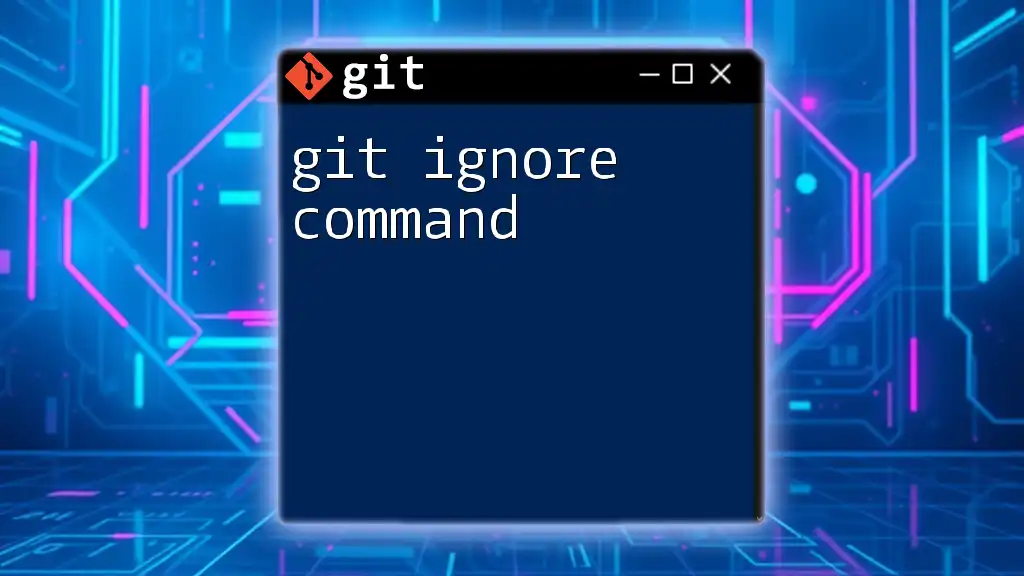
Practical Examples of Git Ignore Exceptions
Scenario: PHP Project
In a PHP project, you might want to ignore the entire `vendor` directory, which is commonly used for dependencies, yet you may want to keep specific files. Here’s how you can set that up:
vendor/
!vendor/composer/
This tells Git to ignore all contents of the `vendor` directory except for the `composer` folder.
Scenario: Python Project
In a Python project, you might have a `pycache` directory that you want to ignore while keeping certain `.py` files. Here’s an example:
__pycache__/
!__pycache__/app.py
With this setup, all cached files will be ignored, but `app.py` will still be tracked.
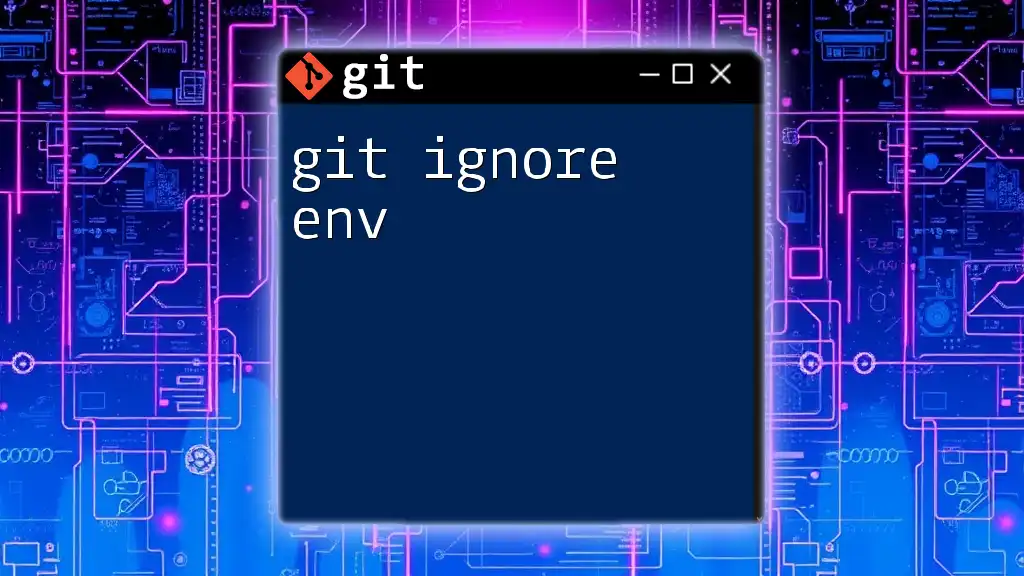
Advanced Usage of .gitignore Exceptions
Combining Patterns
Sometimes, you may need to combine multiple exception patterns to exclude many files while keeping specific ones. For instance, if you wanted to ignore all text files except for two specific documents, you would write:
# Ignore all .txt files except notes.txt and summary.txt
*.txt
!notes.txt
!summary.txt
This will effectively ignore all `.txt` files, while still tracking `notes.txt` and `summary.txt`.
Using Wildcards in Exceptions
You can also utilize wildcards to enhance your exceptions. For example, if you want to ignore all files in an `assets` directory while keeping the `images` subdirectory, you would write:
# Ignore all files in assets/ but not images/
assets/*
!assets/images/
This tells Git to ignore everything in the `assets` folder, but it will still track anything within the `assets/images/` subdirectory.
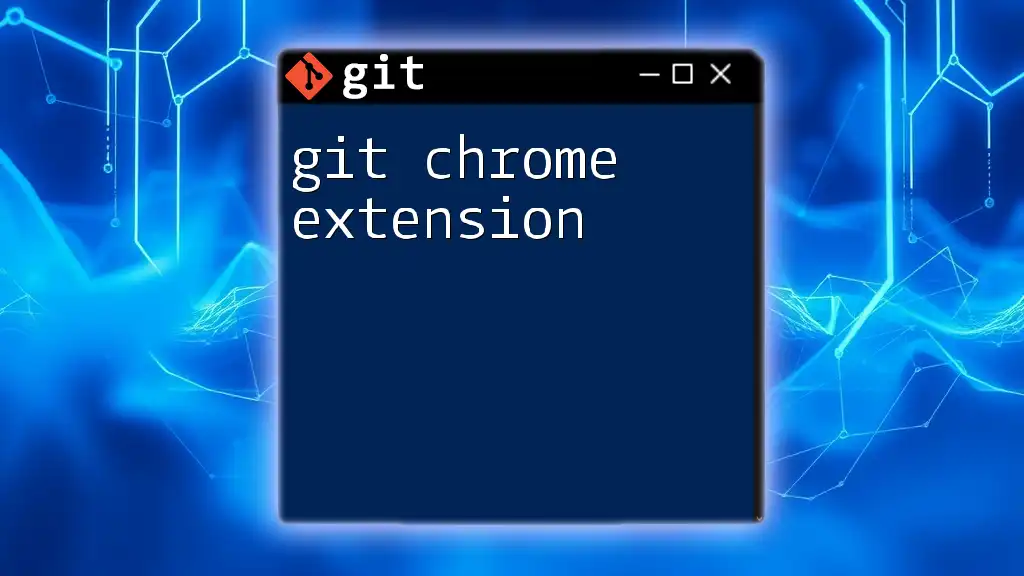
Best Practices for Using Git Ignore Exceptions
Keep it Simple
When defining rules in your `.gitignore`, simplify your file structure as much as possible. Overcomplicating it with numerous exceptions can lead to confusion and potential mistakes. Aim for clarity in your rules to ensure they are easily understandable for you and others who may work on the project.
Commenting Your .gitignore
Adding comments in your `.gitignore` file is a good habit that helps convey what each rule does. This can be particularly helpful as your project grows. For instance:
# Ignore log files, except for development log
*.log
!development.log
Such comments can serve as a helpful reference for you and your teammates later on.
Reviewing .gitignore Regularly
Maintaining your `.gitignore` is just as important as creating it. Regularly review the file to accommodate any new files, directories, or requirements that develop as your project evolves.
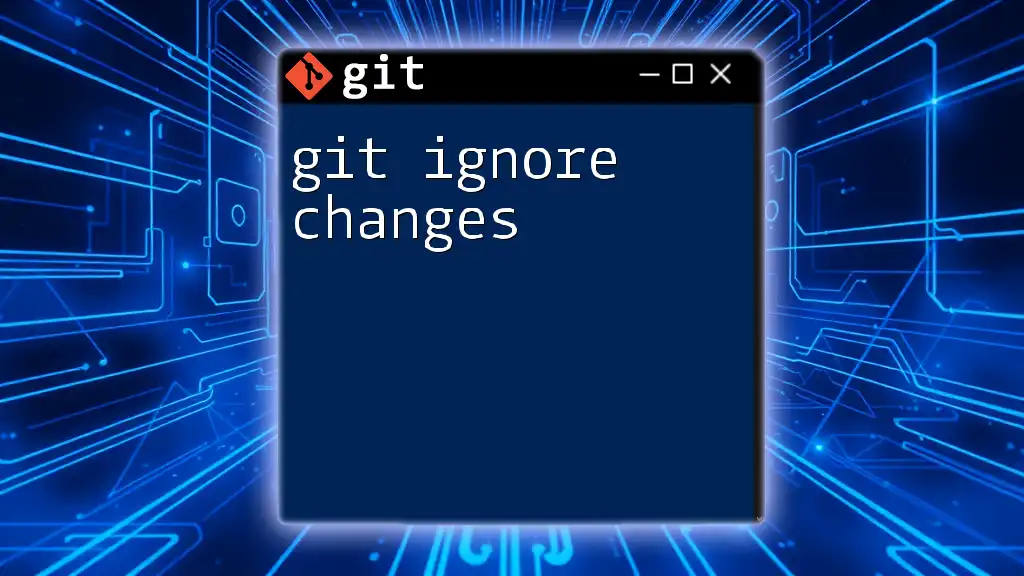
Common Pitfalls and Troubleshooting
Files Still Being Tracked
A common issue users face is when files that should be ignored still show up in the Git status. This often happens because the files were already tracked by Git before being added to `.gitignore`. To untrack files, you can run:
git rm --cached <file>
This command will remove the file from the index without deleting it from the working directory.
Misunderstanding Exceptions
Another common pitfall is misunderstanding how exceptions work. Always remember that exceptions must logically follow the ignored patterns. If the general ignore rule precedes an exception improperly, Git might not process it as intended.
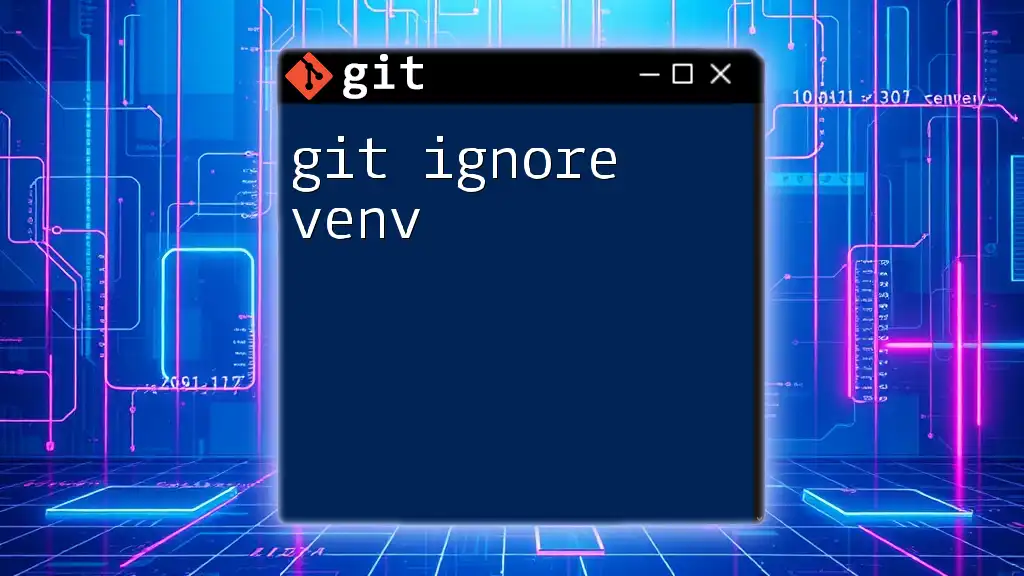
Conclusion
Understanding how to use git ignore exceptions effectively can significantly enhance your workflow within Git. By properly managing your `.gitignore` file and utilizing exceptions, you can maintain control over which files are tracked in your projects. As you continue using Git, actively practice creating `.gitignore` files that cater to your specific needs, and you'll become increasingly proficient in handling version control.
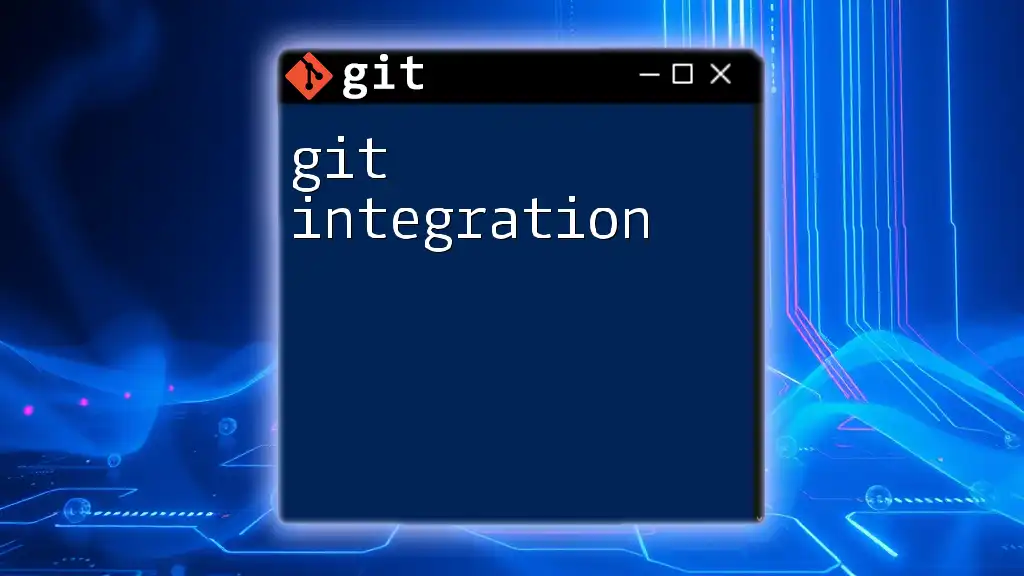
Further Reading
For further exploration of Git, consider checking out the official Git documentation and other resources that delve deeper into version control best practices. This will provide you with additional insights and techniques to streamline your Git experience.