To prevent the `node_modules` directory from being tracked by Git, you can add it to your `.gitignore` file by including the following line:
echo "node_modules/" >> .gitignore
Understanding `node_modules`
What is `node_modules`?
The `node_modules` folder is a crucial component of any Node.js project. When you install packages via npm (Node Package Manager), all the dependencies and their respective modules get stored in this directory. This can include anything from essential libraries to frameworks that your application needs to function.
In a typical Node.js project structure, you might encounter a layout like this:
my-node-project/
├── node_modules/
│ ├── express/
│ ├── mongoose/
│ ├── ...
├── package.json
└── index.js
Each of the folders within `node_modules` represents a package that you’ve installed, along with its sub-dependencies, resulting in a directory that can easily bloat in size.
Why Ignore `node_modules`?
There are compelling reasons to configure your Git repository to ignore the `node_modules` folder:
-
Size: The `node_modules` folder can become extremely large, sometimes exceeding hundreds of megabytes, which makes pushing and pulling from repositories slow and inefficient. Ignoring it keeps your repository lightweight.
-
Reproducibility: All dependencies for a project are properly listed in the `package.json` file. This allows anyone cloning your repository to run a simple command like `npm install` to recreate the exact same environment without actually tracking the `node_modules` folder.
-
Collaboration: When working in teams, ignoring `node_modules` reduces unnecessary clutter in your version history and mitigates potential merge conflicts caused by differing versions of packages that might already be present in someone else's local `node_modules`.
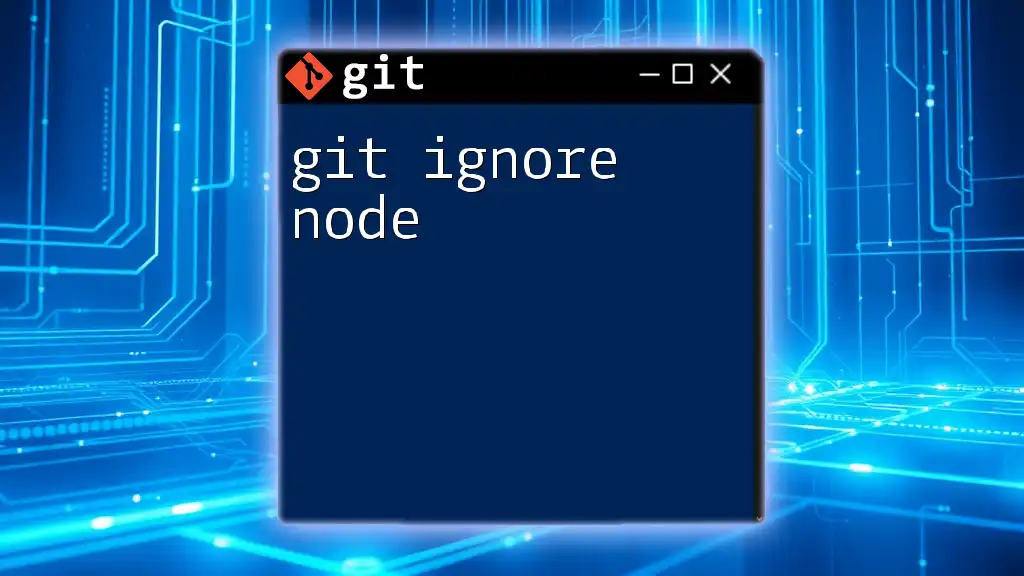
What is `.gitignore`?
Definition and Purpose
The `.gitignore` file is a simple text file that tells Git which files or directories it should disregard. By defining patterns for specific files or folders, you can effectively streamline your version control process, ensuring that no unwanted files are included in your commits.
Syntax of `.gitignore`
The syntax for a `.gitignore` is straightforward but allows for powerful functionality:
- Patterns: You can specify exact filenames or patterns that match multiple files.
- Wildcards: Use `` to match any number of characters (e.g., `.log` ignores all `.log` files).
- Comments: Lines beginning with `#` are treated as comments and are ignored.
Example of a simple `.gitignore` entry for `node_modules`:
# Ignore node_modules
node_modules/
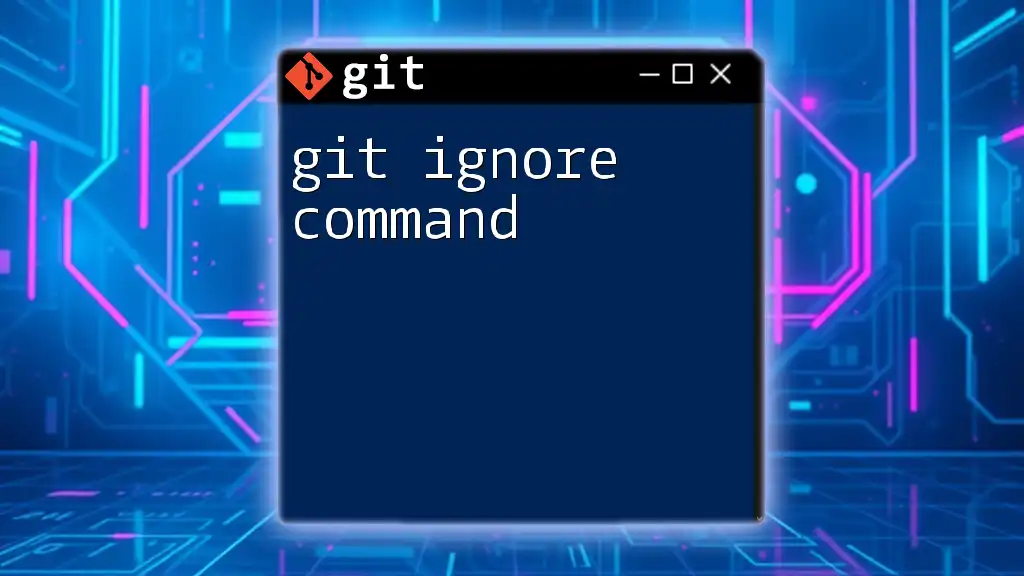
How to Create and Configure a `.gitignore` File
Creating a `.gitignore` file
Creating a `.gitignore` file is a quick and seamless process. You can do this directly from the command line or using your preferred IDE. Here are steps for both methods:
Using the command line:
touch .gitignore
Using an IDE: Simply create a new text file in the root directory of your project and name it `.gitignore`.
Adding `node_modules` to `.gitignore`
Once the file is created, you can easily add the `node_modules` directory to it. Open the `.gitignore` file in your text editor and include the following line:
node_modules/
Additionally, for quick command line entry:
echo "node_modules/" >> .gitignore
Testing `.gitignore`
After setting up your `.gitignore`, validate whether Git is ignoring the `node_modules` folder. You can do this by running:
git status
This command should not list `node_modules` as an untracked file, confirming that Git is operating correctly.
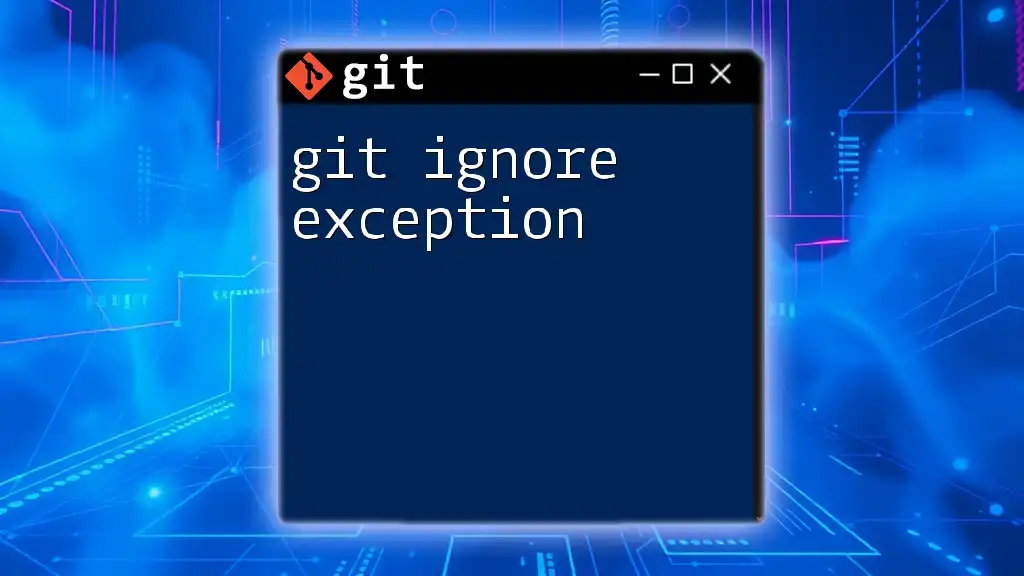
What to Do If You've Already Committed `node_modules`
Removing `node_modules` from Git
If you realize that you've accidentally tracked your `node_modules` directory, don’t worry. You can easily untrack it without deleting the actual files from your filesystem. To do so, execute:
git rm -r --cached node_modules/
After that, make a new commit to update your repository:
git commit -m "Remove node_modules from tracking"
Confirming Removal
To confirm that `node_modules` is no longer part of your repository, again use:
git status
At this point, `node_modules` should not appear in the output of this command.
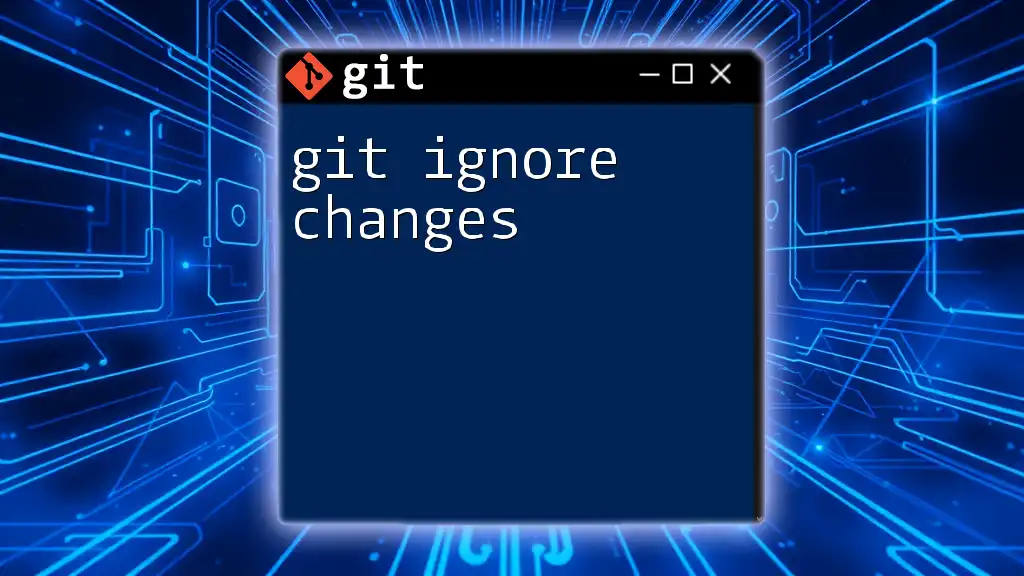
Best Practices for Using `.gitignore`
Standard Patterns for Node.js Projects
When working on Node.js projects, it’s advisable to have a comprehensive `.gitignore` that addresses more than just `node_modules`. Common inclusions are:
# Node.js Best Practices
node_modules/
.env
npm-debug.log
By including patterns for logs and environmental files, you prevent sensitive data from being exposed and keep your repository clean.
Keeping `.gitignore` Updated
A great practice is to periodically review your `.gitignore` file, especially when adding new tools or libraries to your project. This ensures that it remains relevant and effective at ignoring unnecessary files and directories.
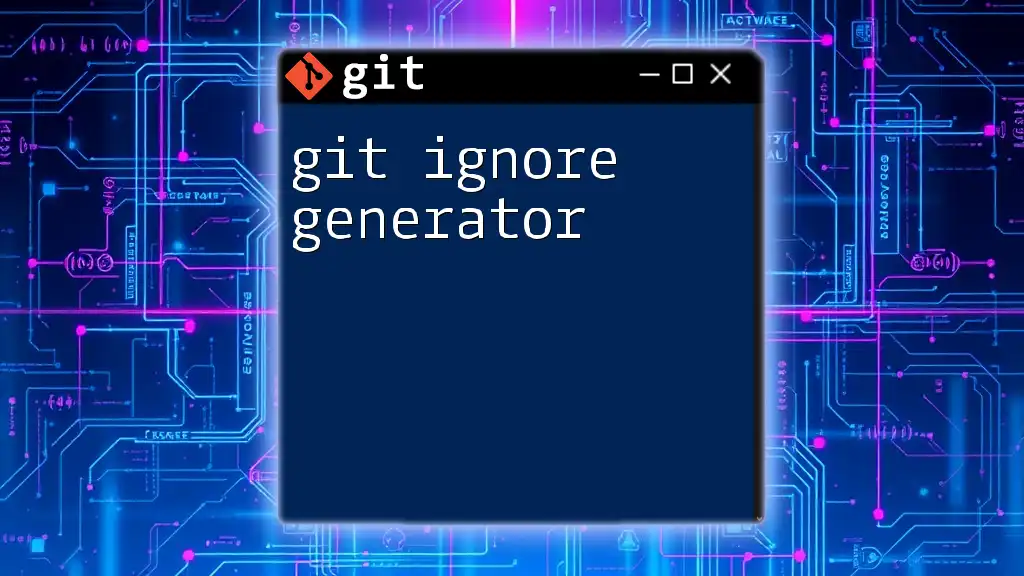
Common Issues and Troubleshooting
Troubleshooting `.gitignore` Problems
If you find that `.gitignore` isn’t functioning as expected, there are a few common issues to check:
- Ensure there are no leading slashes. Using a leading slash makes patterns match only in the root directory.
- Double-check the spelling and syntax of your entries.
To debug specific files, you can use:
git check-ignore -v path/to/file
Additional Resources
For further reading, refer to the official [Git documentation on .gitignore](https://git-scm.com/docs/gitignore) to explore different patterns and options available for ignoring files.
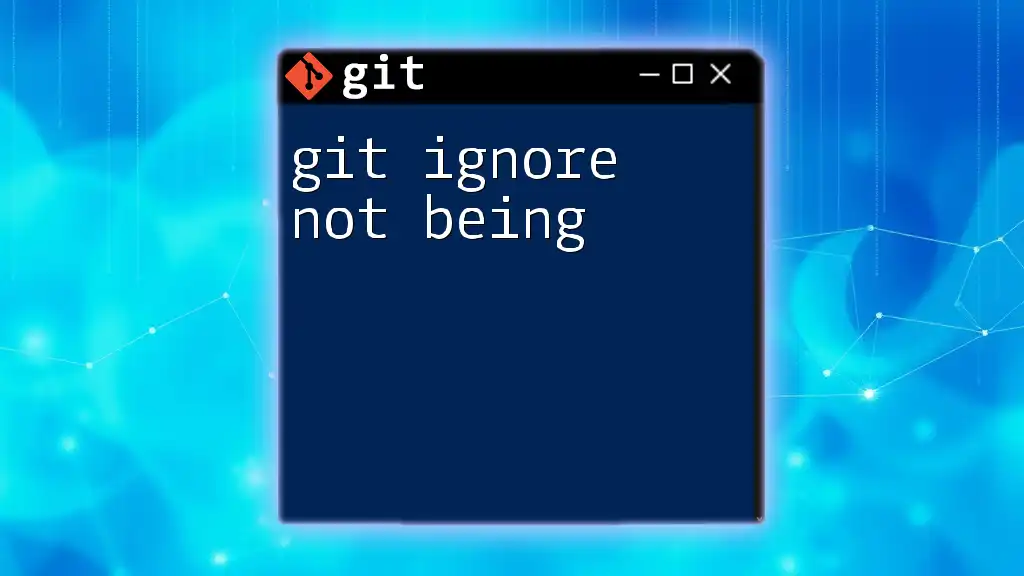
Conclusion
Ignoring the `node_modules` folder in Git is essential for maintaining a clean, efficient, and reproducible codebase in your Node.js projects. By setting up a proper `.gitignore` file, not only do you streamline your Git workflow, but you also foster a collaborative environment that minimizes clutter and potential conflicts.
Make sure to adopt these practices in your future projects, and feel free to share your experiences or questions in the comments below!
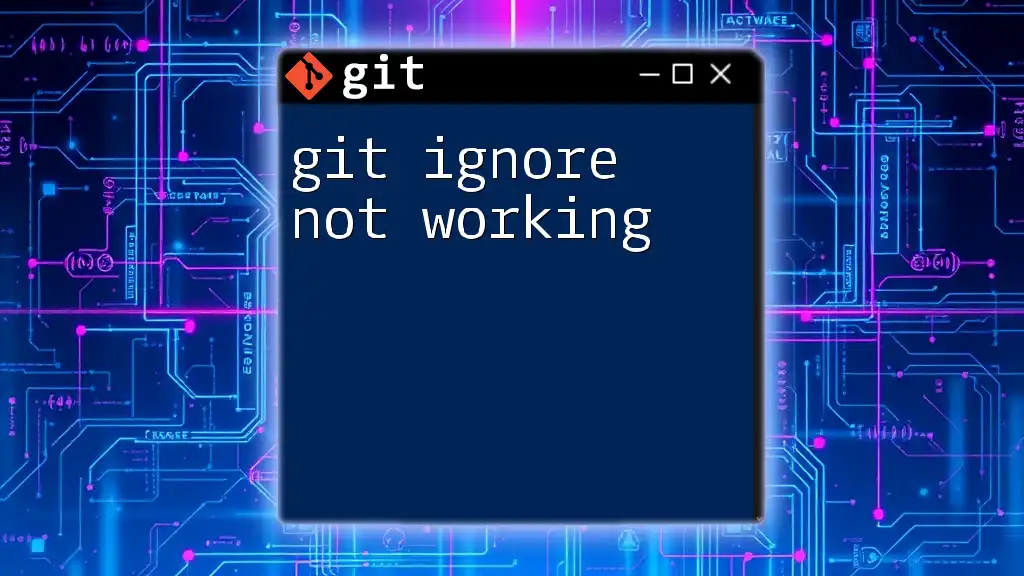
FAQs
What happens if you accidentally push `node_modules`?
If you've pushed `node_modules` by mistake, simply follow the steps above to remove it from tracking, and then the changes will reflect in your repository.
Can I use a global `.gitignore`?
Yes, you can create a global `.gitignore` file for your system, which can be particularly useful for ignoring files that all projects should exclude, like IDE settings.
What other files should I consider ignoring in my project?
Consider ignoring logs, personal configuration files, and any environment files that contain sensitive data, as well as build artifacts and cache directories that aren’t necessary to track.
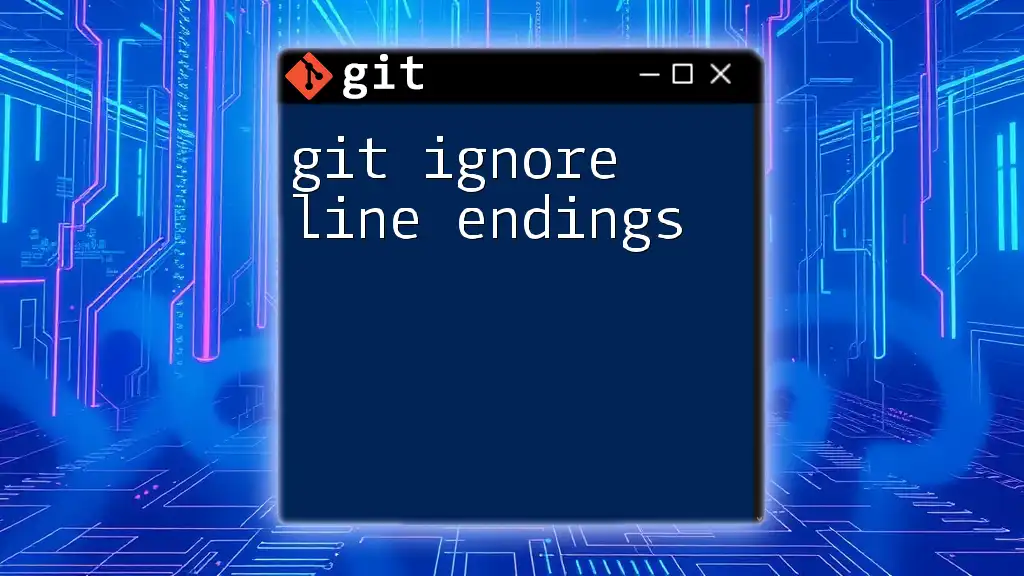
Final Thoughts
By embracing effective Git practices for ignoring unnecessary directories like `node_modules`, you ensure that your version control remains manageable and organized. Explore Git further, and become proficient at incorporating it into your development workflow!