To ignore local changes in a Git repository for a specific file, you can use the `git checkout` command to restore the latest committed version of the file, effectively discarding your local modifications.
git checkout -- <file_name>
What Are Local Changes?
Local changes in Git refer to any modifications made to files in your working directory that have not yet been committed to the repository. These changes can be either staged (ready to be committed) or unstaged (modified but not indexed). Understanding the distinction between staged and unstaged changes is crucial, as it helps you navigate your Git workflow efficiently.
Why You Might Want to Ignore Local Changes
There are several scenarios in which ignoring local changes can be beneficial:
-
Switching branches without committing: Often you want to switch branches to work on a different feature or issue, but you might not be ready to commit your current changes.
-
Experimenting with new features: While testing an idea, local modifications can clutter your view. Ignoring them temporarily allows for a cleaner workflow.
-
Avoiding clutter in your working directory: A clean working environment can boost productivity by minimizing distractions and confusion.
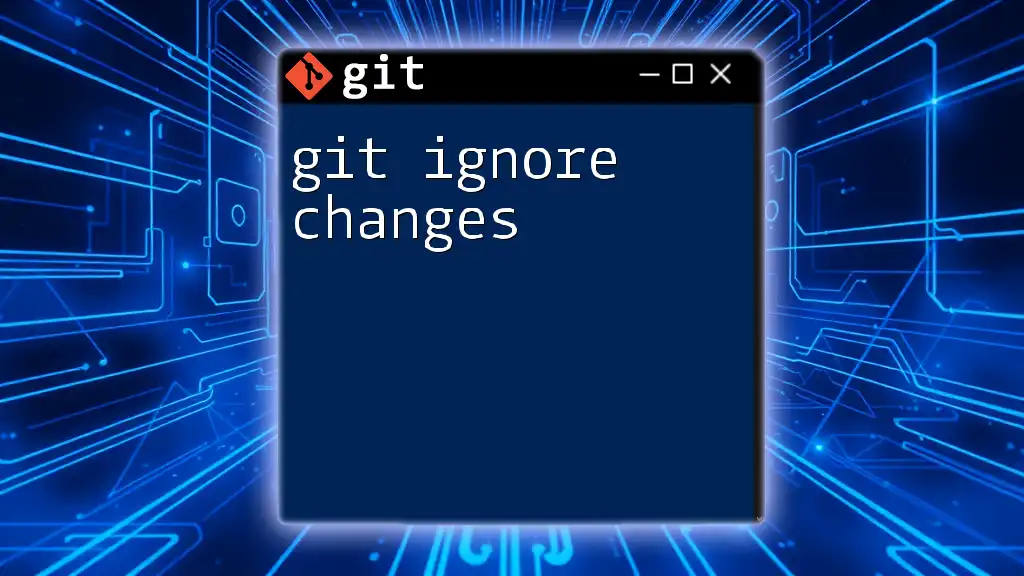
How to Ignore Local Changes in Git
Using `git checkout -- <file>`
If you need to discard local changes in a specific file and revert it to its last committed state, the `git checkout` command is your friend. This command restores the file from the index, effectively undoing any local modifications.
To execute this, simply run the following command:
git checkout -- filename.txt
Pros and Cons: While this method is quick and effective, be aware that it permanently erases your local changes. If you haven’t backed up your modifications, they will be lost forever.
Using `git stash`
`git stash` is a powerful command that temporarily shelves changes you've made so you can work on something else without losing your progress.
How to Stash Changes: Simply run:
git stash
This command will save your local changes (both staged and unstaged) into a stash, giving you a clean working directory to switch branches or work on other tasks.
Retrieving Stashed Changes: When you're ready to retrieve those changes, you can use:
git stash apply
If you want to apply the last stash and keep it in your stash list, the above command suffices. To pop it out of your stash, use:
git stash pop
Example of Running `git stash` in a Project: Imagine you’re working on a feature update but need to switch to a different task. By running `git stash`, you can quickly save your work, switch branches, and come back effortlessly.
Using the `--ignore-unmatch` Option
Another way to ignore local changes is via the `--assume-unchanged` option, which can be quite useful in certain situations. This option tells Git to treat the file as unchanged, effectively ignoring any modifications.
You can achieve this by running:
git update-index --assume-unchanged <file>
This command allows you to modify the file without having to worry about committing those changes inadvertently.
When to Use This Option: This method is particularly helpful when you have configuration files that require local adjustments but should not be propagated across branches or shared with collaborators.
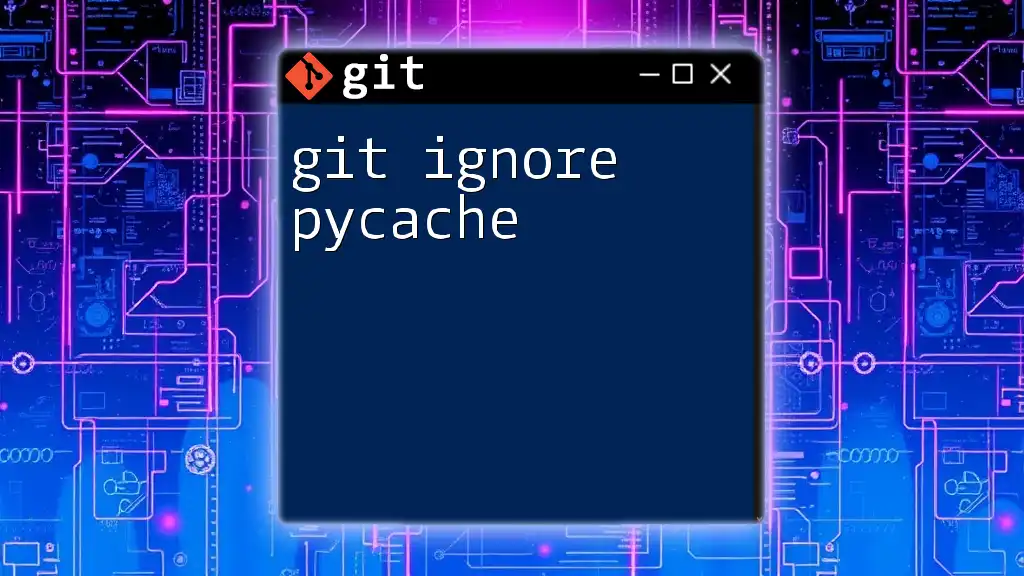
Best Practices for Ignoring Local Changes
Cleaning Up Your Working Directory
Keeping a tidy working environment is essential for maintaining productivity. Regularly review your local changes and utilize `git stash` to clear out temporary edits that you don't want to commit yet. Consider setting a schedule to clean your working directory to avoid confusion.
Documenting Temporary Changes
It’s wise to keep a log of any important changes you temporarily ignore. Use notes or issue trackers to document what modifications you made and why. This practice ensures you can remember what you've ignored and return to it later if necessary.
Using .gitignore and .git/info/exclude
When you're looking to prevent certain files from being tracked in the first place, `.gitignore` and `.git/info/exclude` come into play. The key difference is that `.gitignore` is typically part of your committed repository, while `.git/info/exclude` is only local.
Example of a .gitignore file:
*.log
temp/
Files matching these patterns will not be tracked, ensuring your commits remain clean.
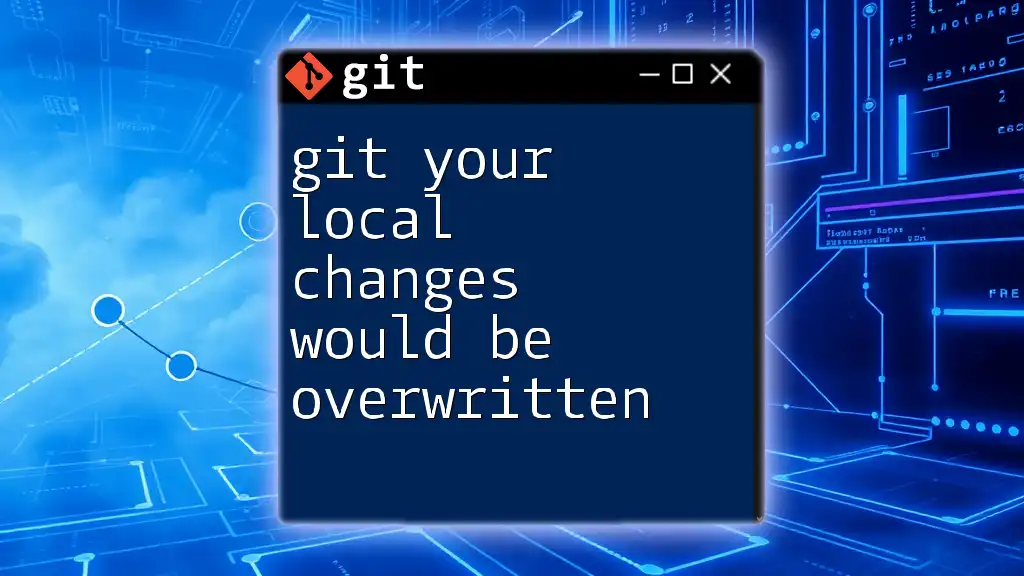
Common Mistakes When Ignoring Local Changes
Forgetting Stashed Changes
An often overlooked aspect of `git stash` is the potential for losing work over time. If you forget about your stashed changes and do not apply them, you risk losing critical updates. To avoid this mistake, you can always review your stash list with:
git stash list
This command will show you all the stashes you've created, helping you keep track of them.
Confusing Stashing with Commit
It’s vital to understand the difference between stashing and committing. Stashing temporarily saves changes, while committing includes them in the project's history. Use commits for changes ready to be shared with others and stashes for immediate but temporary work.
Ignoring Important Changes
The risks of ignoring crucial updates cannot be overstated. If you neglect to track essential modifications, you may end up losing valuable progress. It is best practice to regularly check your working directory and ensure that vital changes are not overlooked.
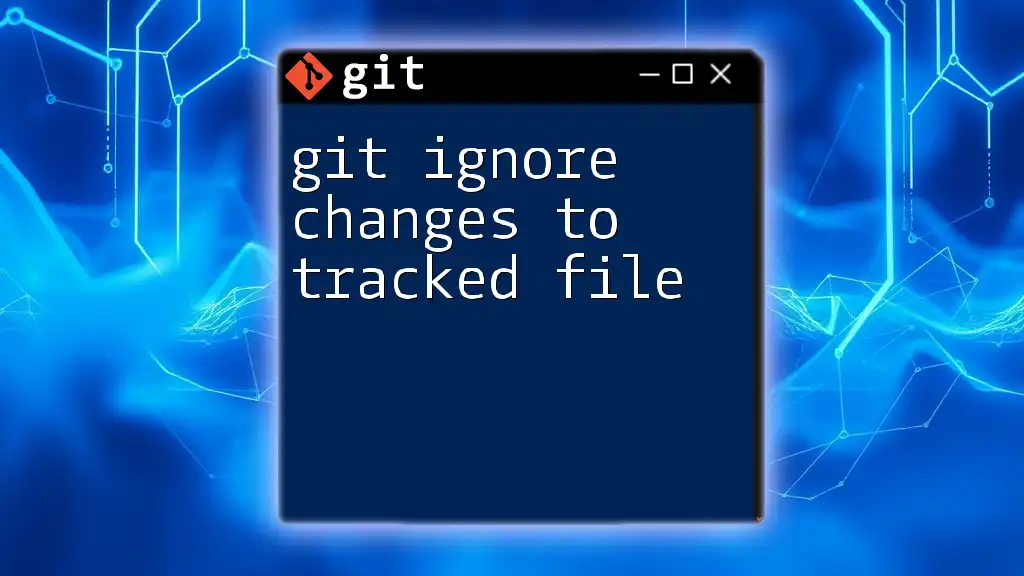
Conclusion
Managing local changes effectively is essential to working with Git. Whether you're using `git checkout`, `git stash`, or the `--assume-unchanged` option, understanding how to ignore these changes can streamline your Git workflow and prevent clutter. Embrace these methods to enhance your productivity and maintain clarity in your projects.
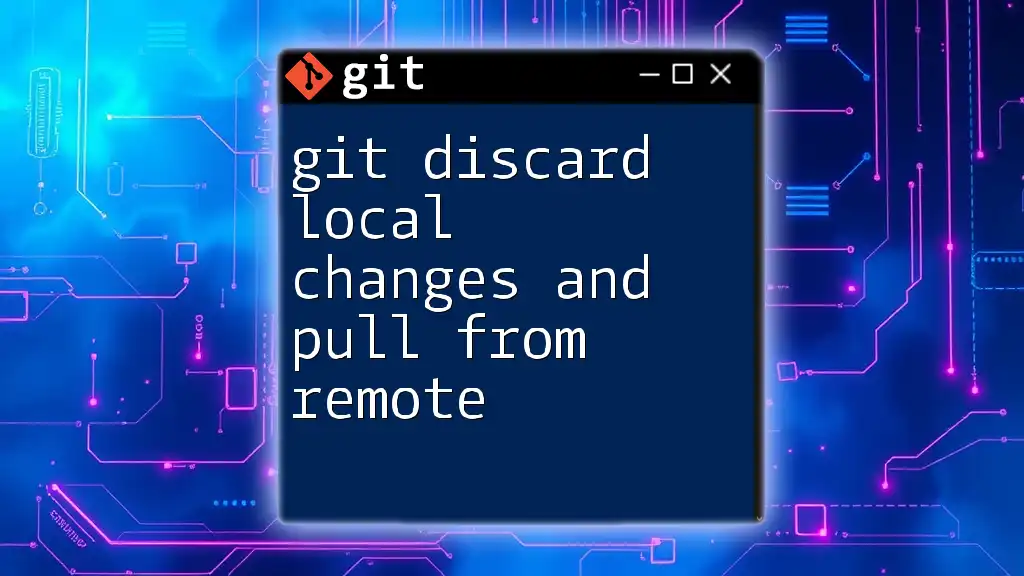
Additional Resources
To further enhance your Git skills, consult the official Git documentation and explore various tools and tutorials available online. These resources will provide you with the knowledge to navigate more advanced features and improve your overall Git experience.