To undo changes in Git, you can use the following command to discard modifications in your working directory and revert to the last committed state:
git checkout -- <file>
Understanding Git's The Working Directory, Staging Area, and Repository
The Working Directory
In Git, the working directory is essentially your current project state. It contains all the files you are currently working on, and any modifications you make in this area are not part of the version history until they are committed. Understanding the working directory is crucial because this is where all your real-time edits occur.
The Staging Area
The staging area (also known as the index) acts as a buffer between the working directory and the repository. When you modify your files and want to include those changes in the next commit, you move them into the staging area. This allows you to collect multiple changes that will be committed together, ensuring that your commit history reflects the logical progression of your work rather than every trivial edit.
The Repository
The repository stores all your version-controlled files and their entire history. Once you commit changes, they move from the staging area to the repository, where they become a permanent part of your project's history. Understanding how these three components work together is key to effectively managing changes in Git and knowing how to undo them.
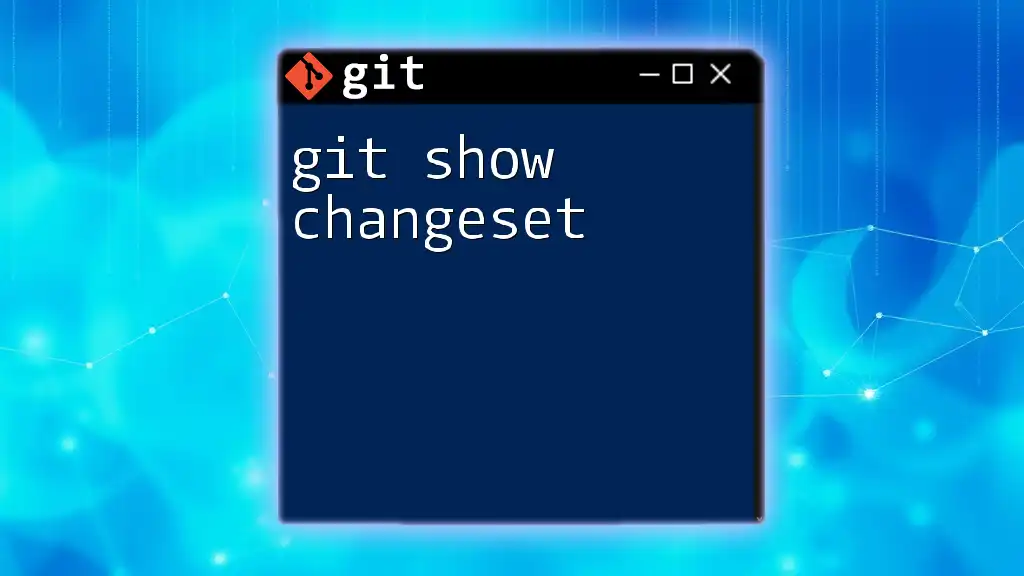
Git Commands for Undoing Changes
Undoing Uncommitted Changes
Reset for the Working Directory
If you find yourself needing to discard uncommitted changes in the working directory for a specific file, you can use git checkout. This command restores the file to the last committed state, effectively undoing any modifications you've made since.
Example:
git checkout -- <filename>
Important: Be careful with this command, as it completely removes any changes made to the specified file. There is no way to recover those lost changes through Git.
Reset for the Staging Area
If you've added files to the staging area but change your mind about them before committing, you can unstage them using git reset. This command removes the specified file from the staging area but leaves the working directory unchanged, allowing you to continue editing.
Example:
git reset <filename>
This command is beneficial when you want to adjust what changes you will commit without losing work.
Undoing Committed Changes
Using git reset
The git reset command is versatile and can be used to undo commits in various ways. There are three primary types of resets: soft, mixed, and hard.
-
Soft Reset: Moves the HEAD to the previous commit but keeps all changes in the staging area. This is useful when you want to adjust your last commit message or tweak changes before committing again.
Example:
git reset --soft HEAD^
-
Mixed Reset: Moves the HEAD to the previous commit and unstages the changes, leaving them in the working directory. This is the default mode of git reset.
Example:
git reset --mixed HEAD^
-
Hard Reset: Moves the HEAD to the previous commit and discards all changes in the working directory. This should be used with caution, as it will permanently delete any uncommitted work.
Example:
git reset --hard HEAD^
Note: Understanding the implications of choosing the appropriate reset type is crucial, especially in collaborative environments where others may rely on your branches.
Using git revert
An alternative to git reset is git revert, which is often safer for undoing changes in public branches. Instead of deleting a commit, it creates a new commit that effectively negates the previous one.
Example:
git revert <commit_hash>
This method ensures that the commit history remains intact while allowing you to eliminate unwanted changes. It’s particularly useful when collaborating, as it conveys intent without rewriting commit history.
Other Useful Git Commands
git stash
Sometimes, you may find yourself in a situation where you want to set aside your changes temporarily without committing them. The git stash command accomplishes this by saving your modifications and reverting your working directory to the HEAD state.
Example:
git stash
git stash apply
You can revisit your stashed changes later once you're ready to resume work. This is particularly helpful when you need to switch contexts but still want to keep your progress.
git reflog
If you accidentally delete a commit or lose track of where your branch was, git reflog becomes your ally. It maintains a log of where your HEAD has been, allowing you to retrace your steps and recover lost commits that aren’t reachable via normal commands.
Example to view reflog:
git reflog
The reflog tracks every move made in your repository, including commits, checkouts, resets, and more, which can be vital in recovering code you thought was lost.
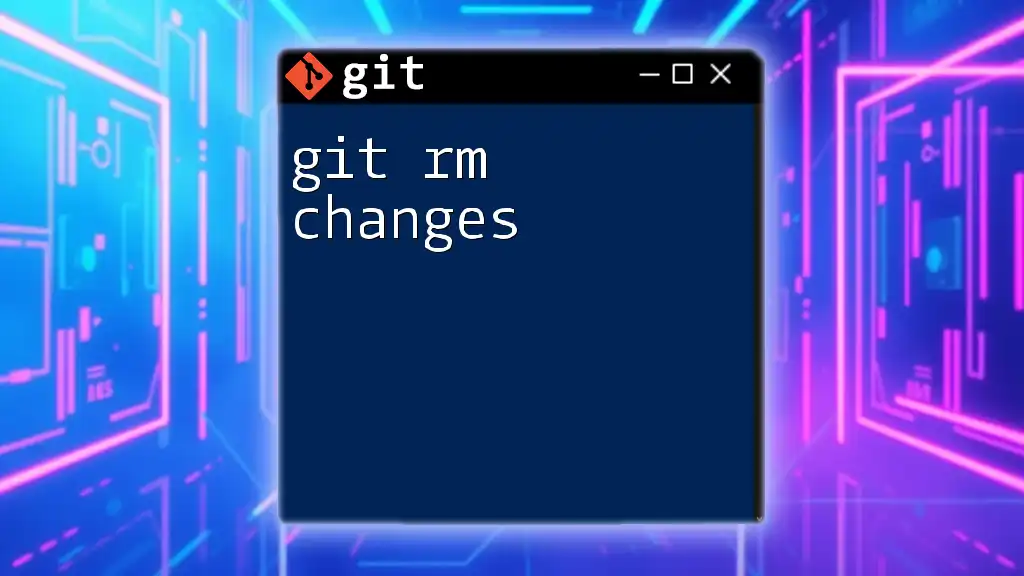
Best Practices for Avoiding Mistakes
Commit Messages Responsibility
One of the most important aspects of using Git effectively is writing clear and descriptive commit messages. A well-structured commit history clarifies the reasoning behind changes, making it easier for you and your collaborators to understand the evolution of the project. Make a habit of committing with meaningful messages that reflect what changes were made and why.
Regular Backups
Implementing a backup strategy for important changes can save you from potential disasters. This could involve regularly pushing your branches to a remote repository or using Git's built-in features to create tags for critical versions of your project.
Creating Branches for Features
Utilizing branches to work on features separately helps to minimize risks of errors on the main branch. Ensure that you create a new branch for each feature or bug fix, allowing you to integrate changes into the main branch only when they are fully tested and ready.
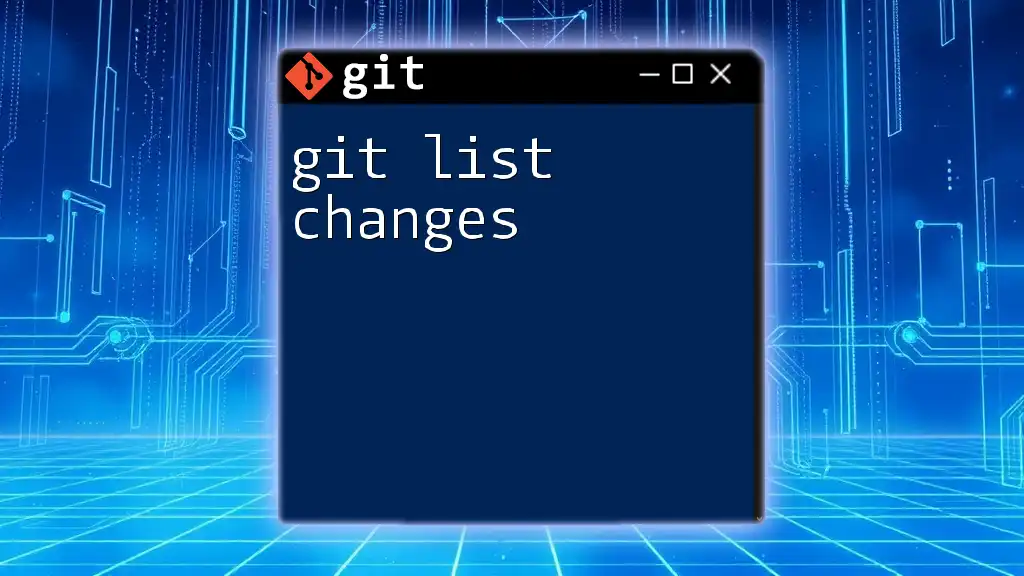
Conclusion
In summary, knowing how to effectively and safely undo changes in Git is a fundamental skill every developer should master. This guide has covered essential commands such as `git checkout`, `git reset`, `git revert`, `git stash`, and `git reflog`, offering you tools for navigating common scenarios in your workflow.
Practice these commands regularly, and don’t hesitate to experiment in a safe environment. With a solid understanding of these concepts, you'll minimize errors and streamline your development process, ultimately leading to greater productivity and success in your projects.
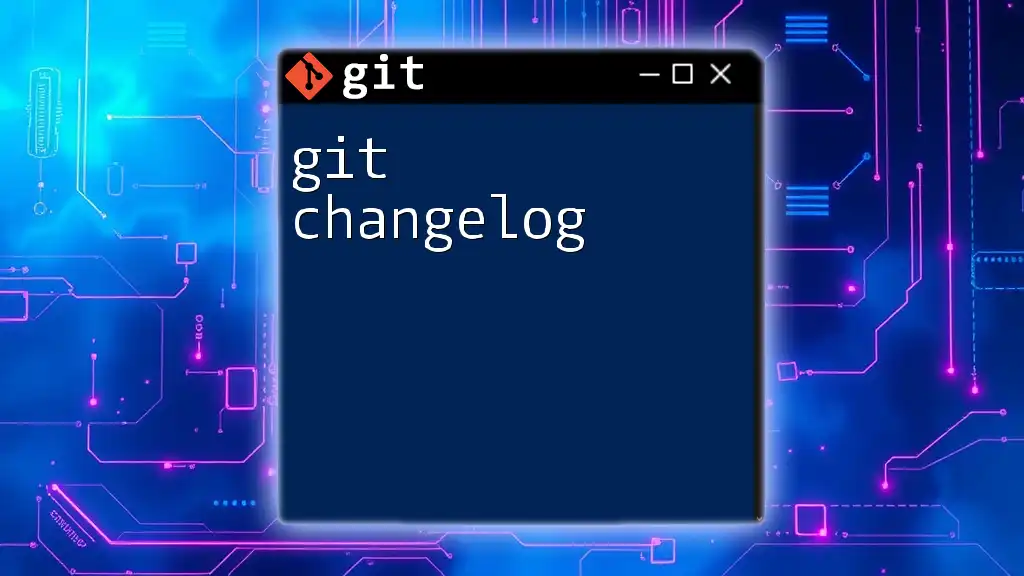
Additional Resources
To further enhance your Git skills, consider exploring these resources:
- Books and Online Courses: Look for material focused on Git fundamentals and best practices.
- Documentation: The official Git documentation provides valuable insights and detailed explanations.
- Git Cheat Sheets: Printable Git cheat sheets can be a handy reference as you continue to develop your Git proficiency.