To undo staged changes in Git and unstage files while keeping your changes in the working directory, use the following command:
git reset <file>
Replace `<file>` with the name of the file you want to unstage.
Understanding Staged Changes
In Git, staged changes refer to files that have been modified and added to the staging area, ready to be included in the next commit. The staging area acts as a middle ground between the working directory (where files reside in their current state) and the repository (where committed files are also tracked).
Understanding the distinction between staged, unstaged, and committed changes is essential for effective version control. Staged changes are essentially snapshots of your modifications that await confirmation via a commit. Unstaged changes are modifications that exist in your working directory but have not yet been added to the staging area. Commits, on the other hand, are saved versions of your project at specific points in time.

Why You Might Want to Undo Staged Changes
There are several scenarios where you might find yourself needing to undo staged changes:
- Mistakenly Staging Files: You might have accidentally added files that you don’t want to include in your commit.
- Reconsidering Changes Before Committing: Perhaps upon reflection, you decide some changes aren’t ready to be saved.
- Comparing Changes Before Finalizing: Reviewing your staged changes before making a commit might reveal needed tweaks.

Git Commands to Undo Staged Changes
Git offers a few commands that can effectively help you undo staged changes. Below, we’ll discuss the most commonly used commands: `git reset`, `git restore`, and `git checkout`.
git reset
The `git reset` command is a fundamental command for managing your Git repository. When used to unstage changes, it moves the specified files out of the staging area but leaves your working directory intact.
Usage of `git reset` for unstaging changes:
To unstage a specific file, you would use:
git reset <file>
For example, if you want to unstage a file named `example.txt`, you can issue:
git reset example.txt
This command will remove `example.txt` from the staging area while keeping your current modifications intact in your working directory.
If you want to unstage all changes, you can run:
git reset
This command clears the entire staging area, allowing you to start over with your modifications.
git restore
Introduced in later versions of Git, `git restore` provides a more intuitive way to manage staged changes. This command is specifically dedicated to restoring files to a previous state.
Usage of `git restore` for unstaging:
To unstage a single file, the command syntax is:
git restore --staged <file>
For example:
git restore --staged example.txt
This command will unstage the file `example.txt` from the staging area, similar in effect to `git reset`. The distinction lies in its explicit functionality as a restoration command, which makes it an excellent tool for clarity in your version control processes.
git checkout (deprecated in newer versions)
Historically, `git checkout` was used to both switch branches and revert files. While it has mostly been replaced by more specific commands, you may still encounter it with users who are accustomed to the older syntax.
If you want to use `git checkout` to unstage a file, the command syntax is:
git checkout -- <file>
For instance, using:
git checkout -- example.txt
This command will revert the file back to the state of the last commit, throwing away any modifications made after staging. However, it is important to note that `git checkout` is now considered deprecated for this purpose, and users are encouraged to embrace `git restore` for clarity.

Understanding What Happens After Undoing Staged Changes
After using the commands above to unstage files, it is critical to understand the state of your repository. The unstaged files will remain in your working directory with their modifications intact, meaning you can re-add them later if desired or continue to work on them.
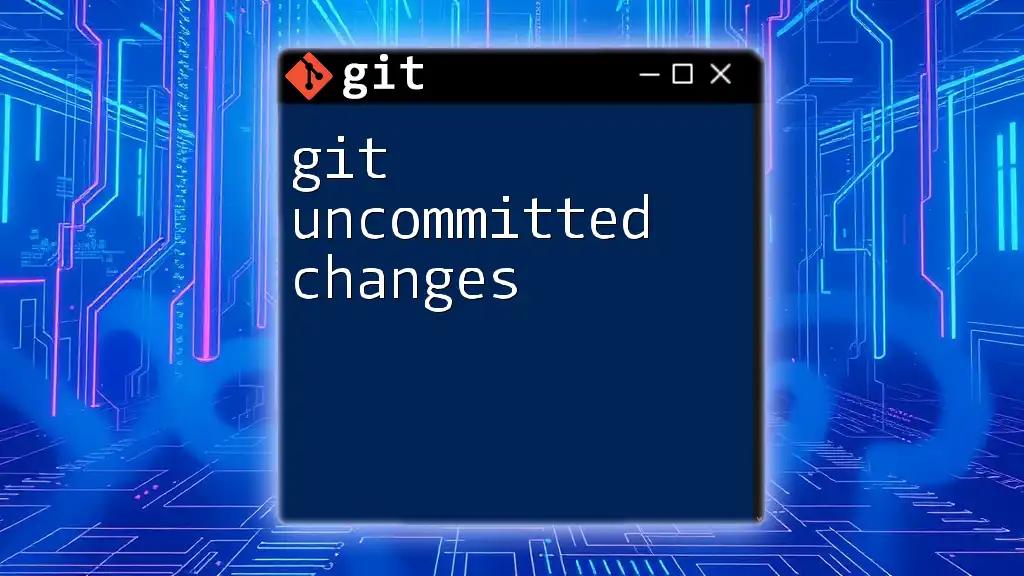
Best Practices for Managing Staged Changes
To avoid common pitfalls when staging files or undoing staged changes, consider these best practices:
-
Review Staged Changes: Always use `git status` to confirm which files are staged or unstaged. This command gives you a clear overview of your repository’s current state.
-
Use `git diff`: Before staging, utilize `git diff` to review changes in your working directory compared to what’s currently staged. This allows for a more informed staging process.
-
Practice Changes: Familiarize yourself with the commands through practice. Experiment in a test repository to build confidence without risking important work.
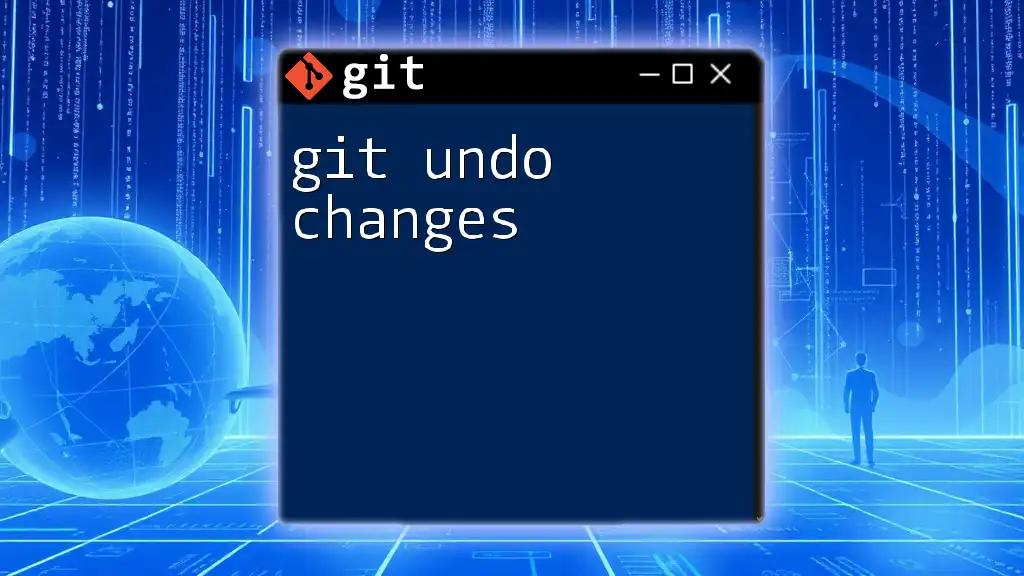
Troubleshooting Common Issues
You may encounter issues while trying to undo staged changes, such as restoring changes that you didn’t intend to lose or misunderstanding how commands function.
If you mistakenly stage changes and then commit them, you can use `git reflog` to view recent commits and understand what happened at each stage. This can help you recover lost changes or clarify any confusion.
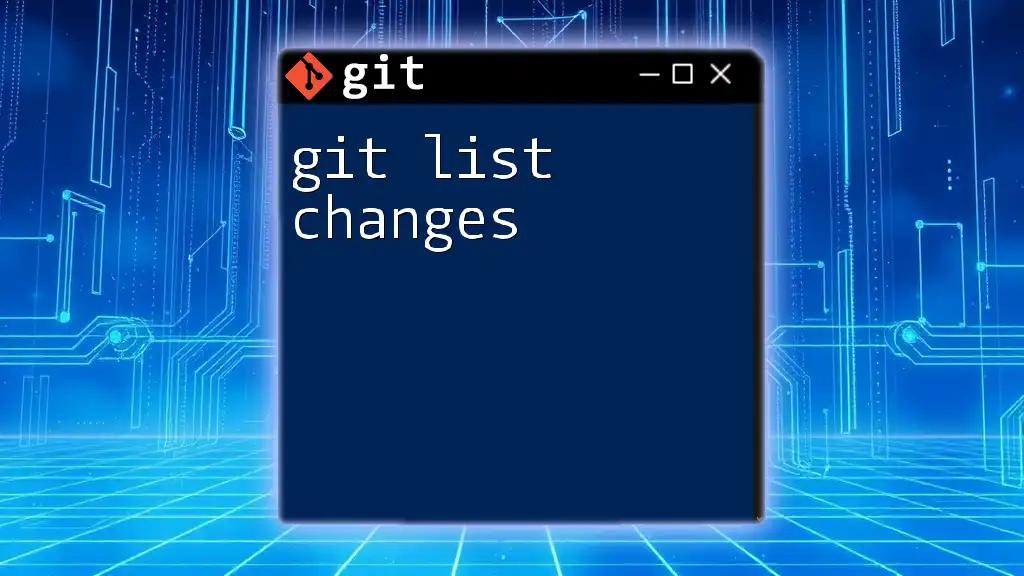
Conclusion
To sum up, understanding how to git undo staged changes is crucial for effective version control. Whether using `git reset`, `git restore`, or the now deprecated `git checkout`, you possess the tools necessary to control your staged changes efficiently. Practicing these commands will not only build your confidence but will also enhance your overall workflow in Git.
By mastering these techniques, you're well on your way to becoming proficient in Git, ultimately improving your productivity and the integrity of your projects.