The `git unstage` command is used to remove files from the staging area before a commit, effectively reverting them back to the working directory without affecting the actual file changes.
git reset HEAD <file>
Understanding Git Staging Area
What is the Staging Area?
The Git staging area, often referred to as the index, acts as a middle ground where changes to files are prepared before finalizing them in a commit. When you modify files in your repository, those changes don’t instantly enter the Git history. Instead, they reside in this staging area until you decide to commit them. This design provides a powerful workflow, allowing you to review and curate your changes selectively before recording them in your repository’s history.
Why You Might Need to Unstage Files
There are several scenarios where you may need to unstage files:
- Accidental Additions: If you mistakenly added files you didn’t intend to commit.
- Last-Minute Changes: When you realize certain changes need to be modified further before committing.
- Selective Commits: If you're refining what you want to include in your commit, you might need to remove some staged files.
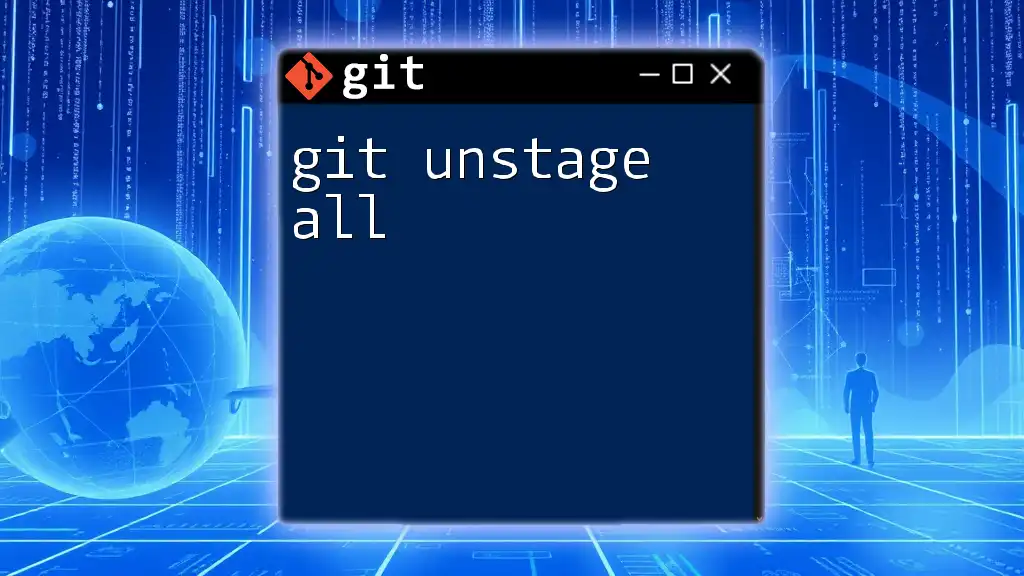
The Basics of Git Unstage
What Does `git unstage` Mean?
The term "unstage" refers to the action of removing changes from the staging area. The primary purpose of the `git unstage` command is to revert files back to the state they were in before they were staged, making them untracked for the upcoming commit.
How to Unstage Files in Git
Using `git reset`
One of the most common ways to unstage files is with the `git reset` command. The syntax is straightforward:
git reset <file>
For example, if you have added a file named `myfile.txt`, you can unstage it with the command:
git reset myfile.txt
Upon executing this command, `myfile.txt` will be removed from the staging area and will not be included in the next commit. It’s important to note that this command does not discard changes in the working directory.
Using `git restore`
With the introduction of Git 2.23, a new command, `git restore`, streamlines the process of unstaging. You can use the following syntax:
git restore --staged <file>
For instance, to unstage the same file, simply run:
git restore --staged myfile.txt
This command also removes `myfile.txt` from the staging area. Using `git restore` can be more intuitive for users transitioning from other version control systems, as it better conveys the action of restoring files to their prior state.
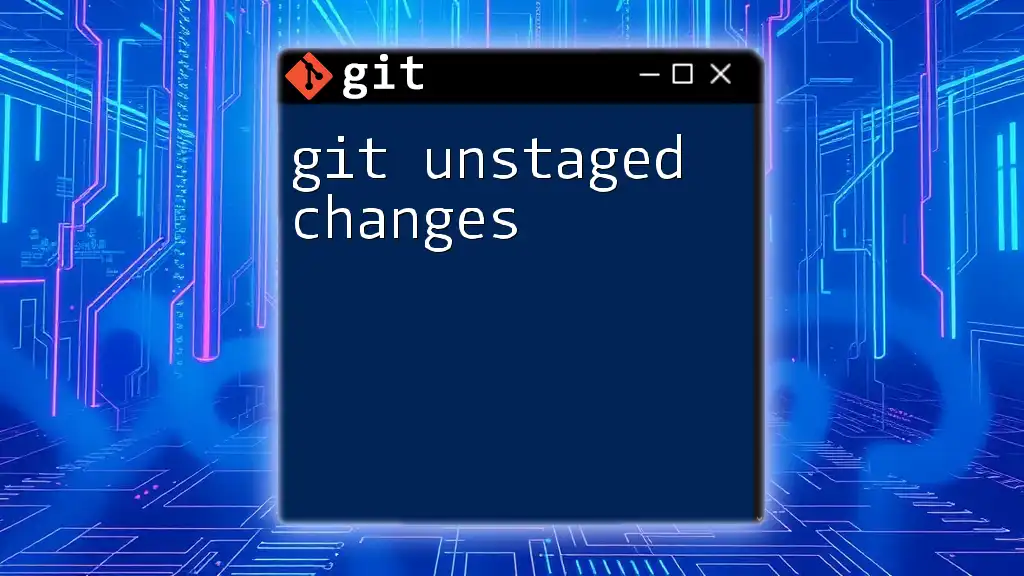
Unstaging Multiple Files
Unstaging All Changes
If you find yourself in a situation where you want to unstage all changes at once, you can easily do so with the command:
git reset
This command resets the entire index, effectively unstaging all files that have been added. However, be cautious, as this will remove all staged changes, not just specific files.
Unstaging Specific File Types
When working on larger projects, you might want to selectively unstage files based on patterns or types. For instance, to unstage all `.txt` files, you can use:
git reset *.txt
This flexibility is particularly useful in managing extensive codebases, allowing you to refine exactly what you want to commit.
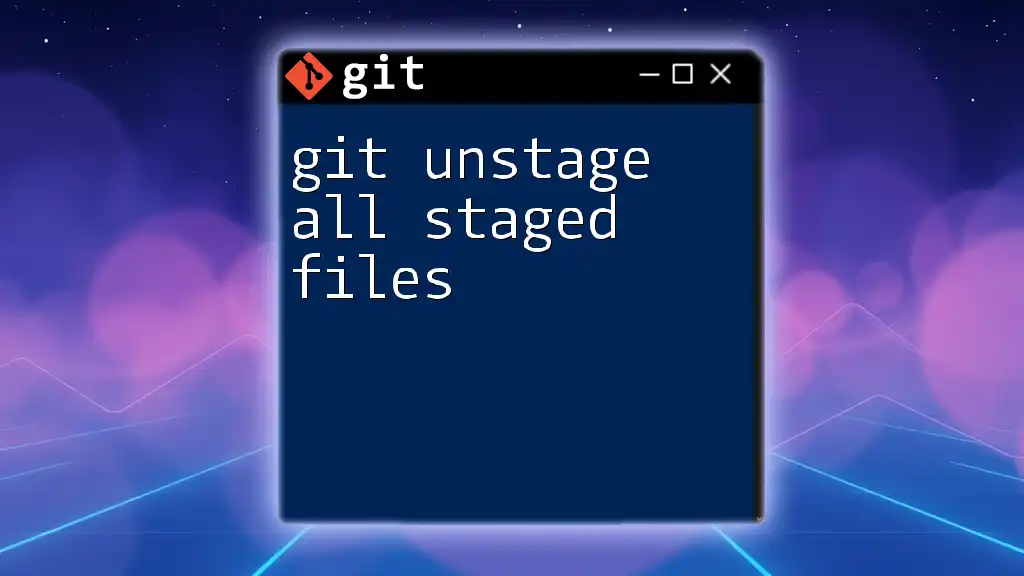
Situations and Best Practices
When to Use `git unstage`
Using `git unstage` is beneficial in several situations:
- Review Process: Before making a commit, take a moment to review what is staged and decide if there’s anything that no longer belongs there.
- Correcting Mistakes: If you’ve added a file accidentally, quickly unstaging it can help maintain a clean commit history.
- Collaboration Consideration: If you're working in a team, ensuring that your commits are precise and contain only relevant changes is essential for collaborative success.
Common Mistakes When Unstaging
While `git unstage` is a straightforward command, beginners can make a few common errors, such as:
- Not Checking the Staging Area First: Always run `git status` before unstaging to confirm what is currently staged.
- Unintentionally Losing Changes: Remember, unstaging does not delete changes in the working directory, but confusion may arise if there are multiple changes across several files.
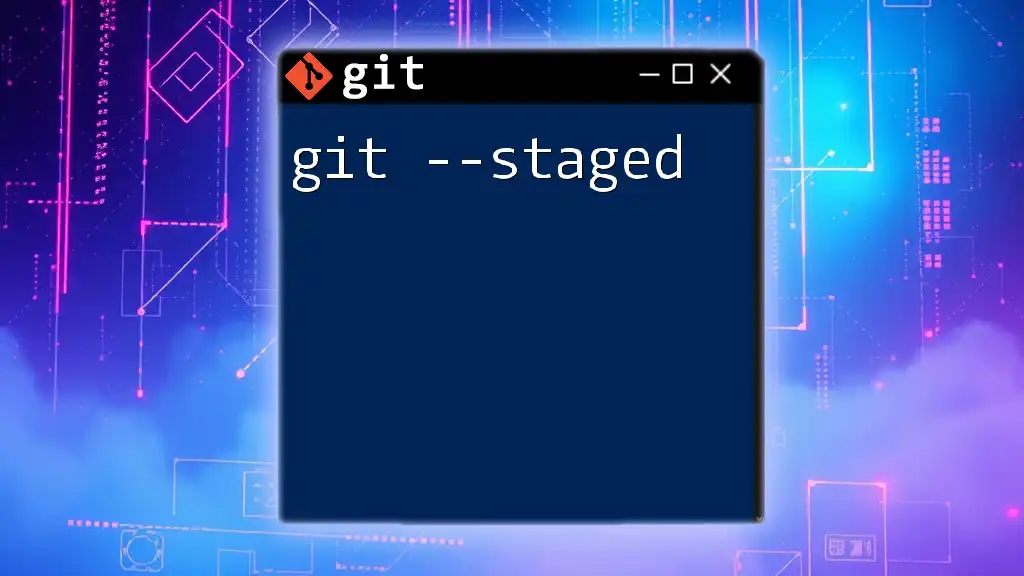
Troubleshooting Unstaging Issues
Unstaging Does Not Work as Expected
If you find that unstaging isn’t working as planned, consider the following:
- Check for Typos: Ensure you are referencing the correct file names in your commands.
- Inspect the Staging Area: Running `git status` can clarify what’s currently staged and what changes remain in your working directory.
Sometimes if unstaging seems ineffective, you may need to reevaluate your command usage or the state of your Git repository.
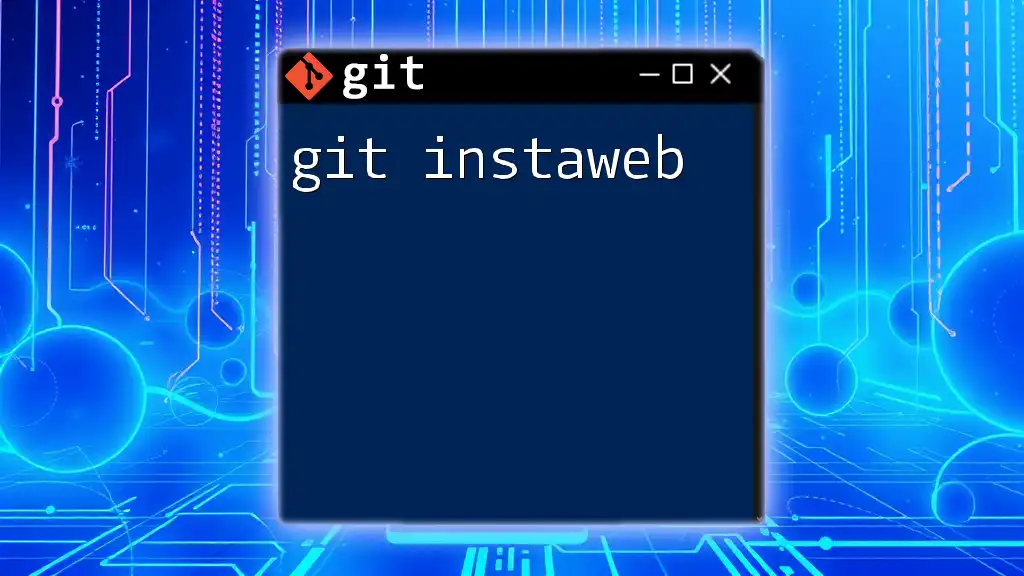
Conclusion
Recap of Key Points
In summary, understanding how to use `git unstage` effectively allows you to maintain a smooth and organized workflow when working with Git. Whether through `git reset` or `git restore`, the ability to refine your staged changes is essential for creating clean and meaningful commits.
Call to Action
Practice using the `git unstage` command in your own projects. Experiment with different files and methods to get comfortable with Git’s functionalities. For further learning, explore additional resources and documentation to deepen your understanding of Git and strengthen your version control skills.
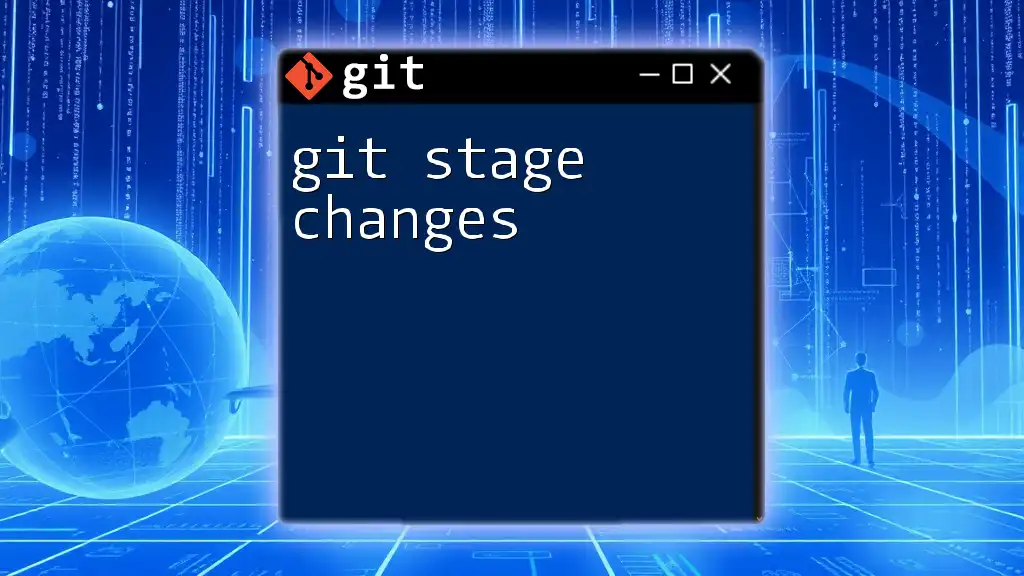
Additional Resources
Recommended Reading and Tools
To further enhance your Git proficiency, consider delving into the following resources:
- Official Git Documentation: Comprehensive and reliable.
- Online courses on Git: Platforms like Udemy or Coursera offer structured learning paths.
- Community forums: Engage with communities such as Stack Overflow for peer support and advanced tips.
With the practical knowledge of the `git unstage` command at your disposal, you are now better equipped to navigate the complexities of version control efficiently.