Git staging is the process of selecting and preparing changes in your working directory for the next commit to the repository.
Here’s a code snippet demonstrating how to stage changes for commit:
git add <file1> <file2> # Stages specific files
git add . # Stages all changes in the current directory
Understanding Git Staging
What is Git Staging?
Git staging refers to the area in Git where changes are prepared before they are committed to the repository. This staging area acts as an intermediary between your working directory, where you make changes, and the Git repository, where those changes are permanently recorded. Understanding git staging is crucial for effective version control, as it allows you to select which changes to include in your next commit.
The Difference Between Staging and Committing
The concepts of staging and committing are often conflated, but they serve distinct purposes within Git.
-
Staging involves selecting specific changes and moving them to the staging area with the `git add` command. This is basically preparing changes that you want to include in your next commit.
-
Committing is the process of recording those staged changes into the repository with the `git commit` command. Once committed, the changes are considered part of the project's history.
Visualizing this flow can help clarify these processes: changes are first made in the working directory, then staged to the staging area, and finally committed to the repository.
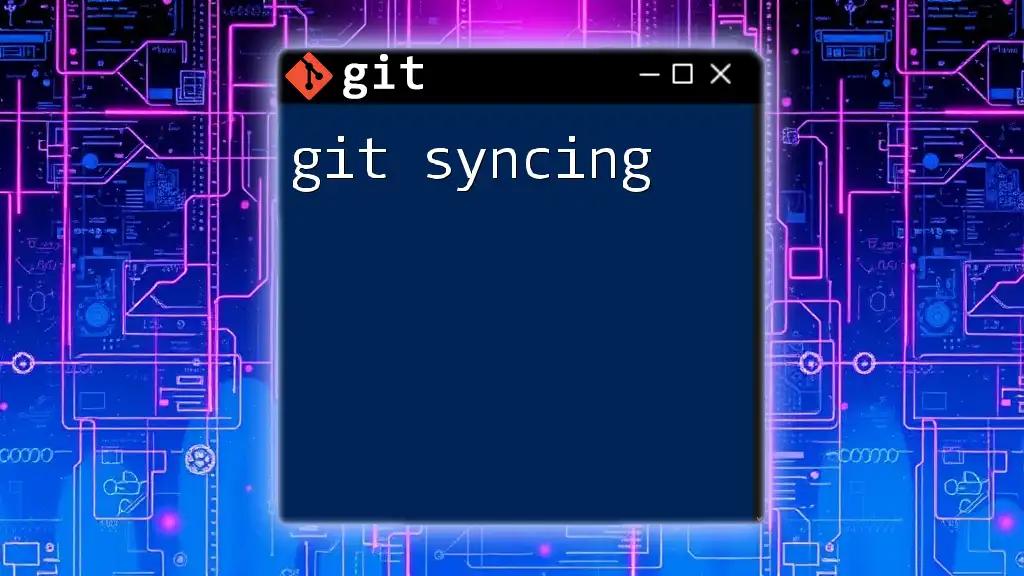
The Git Staging Process
How to Stage Changes
Using `git add`
The fundamental command to stage changes in Git is `git add`. You can stage specific files or all changes.
-
To stage a single file, use the command:
git add myfile.txt
-
If you want to stage all changes within your current directory, you can use:
git add .
Both commands move changes from the working directory to the staging area, where they are prepared for the next commit.
Staging Specific Parts of a File
In some cases, you may only want to stage specific changes within a file. Git provides an excellent feature for this with the `git add --patch` command. This allows you to select hunks of changes interactively.
To stage selected portions of a file, use:
git add -p myfile.txt
You'll be prompted to review each change, and you can decide whether to stage it or not. This granularity can be incredibly helpful for maintaining clean commit histories.
Viewing Staged Changes
Using `git status`
Once you've staged your changes, you might want to review them. The command `git status` provides crucial information about your staging area. It indicates which files are staged and which are modified but unstaged, giving you a comprehensive overview of your current state.
Using `git diff --cached`
To see what you have staged specifically, you can use:
git diff --cached
This command will show the differences between the last commit and what you currently have staged, allowing you to review what you are about to commit in detail.

Common Staging Scenarios
Staging Untracked Files
Untracked files are those that have been created but not yet added to Git. These files won't show up in commits until staged. To stage untracked files, you can use the same `git add` command as before. For example:
git add newfile.txt
If you want to stage all untracked files in your directory, using `git add .` will also capture them.
Modifying Staged Changes
Sometimes, you may find that you've staged changes that you no longer want to include. This can be easily managed with the `git reset <file>` command. For instance, if you’ve staged `myfile.txt` but wish to unstage it, simply run:
git reset myfile.txt
This action will remove `myfile.txt` from the staging area, leaving it in the working directory for further edits or to be excluded from the next commit.
Undoing Changes in Staging
If you need to revert staged changes back to their last committed state, the `git checkout` or `git restore` commands can be useful. Let’s say you’re unsure of what you staged and want to revert changes for a particular file:
git restore --staged myfile.txt
This command will unstage `myfile.txt`, allowing you to make further adjustments without affecting the commit history.

Best Practices for Git Staging
Use Commit Messages Wisely
When committing changes, taking the time to craft thoughtful commit messages is essential. Clear, concise messages give context to changes and are invaluable for future reference. A good guideline is to start with a short summary followed by a more detailed explanation if necessary.
Commit Frequently
Frequent commits of smaller changes enhance productivity and maintain a clear project history. It’s often advisable to stage and commit related changes together rather than batching everything at once. This facilitates easier tracking of progress and enhances collaboration with other team members.
Keep Your Staging Area Clean
Managing which changes are staged can significantly streamline your workflow. Regularly review your staged files and make sure only relevant modifications are included. Avoid staging unnecessary files or changes that are incomplete; this will help keep your commit history clear and understandable.
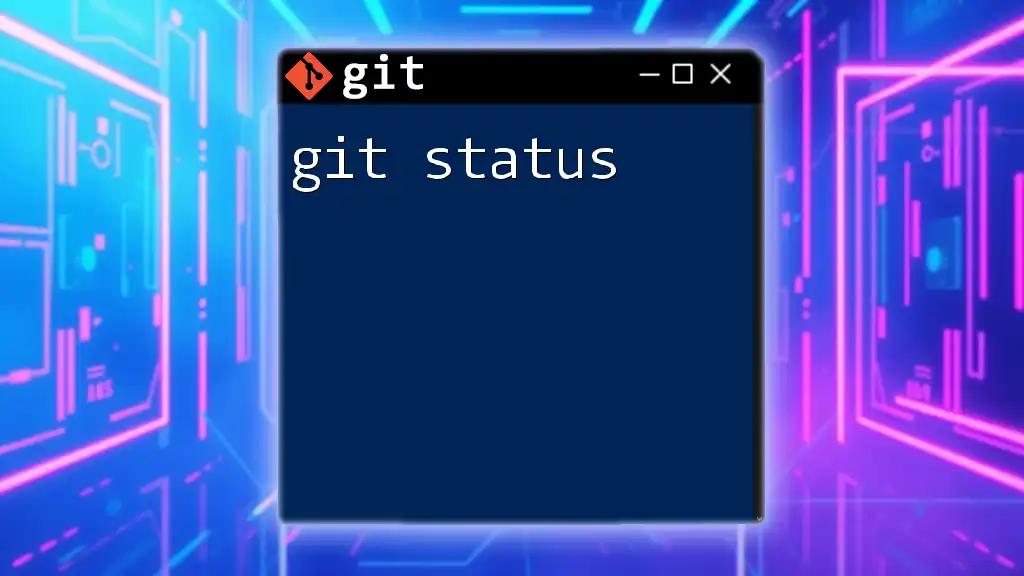
Advanced Staging Techniques
Staging for Multiple Commits
A strategic approach to staging allows you to prepare changes for multiple commits. This can be particularly useful when working on features or bug fixes that consist of several discrete changes. Organizing commits in this manner enhances clarity and makes tracking tasks easier.
Staging with Aliases
Git allows you to create custom aliases for commonly used commands, making your workflow smoother and more efficient. For example, if you often use staging commands, you might create an alias for it:
git config --global alias.s stage
Using this alias, you can streamline your command inputs and boost productivity.
Using Git GUIs for Staging
While the command line offers powerful functionality for staging, many users benefit from graphical interfaces. Popular Git GUI tools, like GitKraken, SourceTree, and GitHub Desktop, provide easy-to-navigate visual staging features. These tools can simplify the staging process, particularly for those who are less comfortable with command line operations.
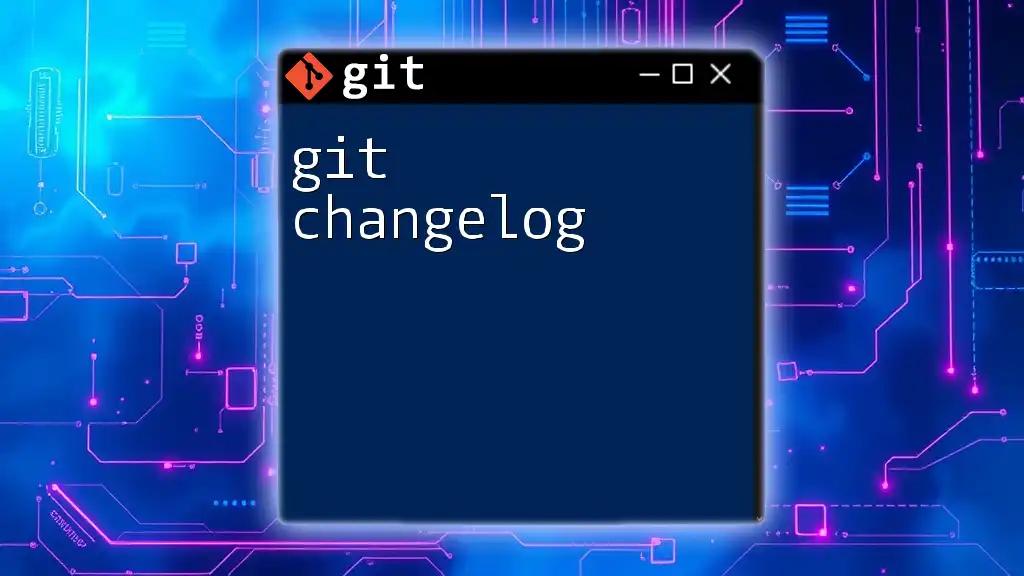
Conclusion
In this comprehensive guide, we’ve explored the essential components of git staging, a fundamental concept in Git version control. By understanding how to effectively use the staging area, you will become proficient at managing changes before committing them to your Git repository.
Practicing staging commands in a safe environment can reinforce these concepts and improve your workflow. Don't hesitate to share your experiences and questions about git staging, as community engagement can lead to deeper learning and skill development.