Git syncing refers to the process of ensuring that your local and remote repositories are in sync by fetching changes from the remote and merging them into your local branch.
Here’s a basic code snippet to demonstrate how to sync your local repository with the remote repository:
git fetch origin
git merge origin/main
Understanding Git Terminology
Basic Concepts
Repository is a crucial term in Git that refers to a storage space for your project. There are two main types of repositories:
- Local Repository: This resides on your own computer and is where you perform your work.
- Remote Repository: Hosted on a server (like GitHub, GitLab, or Bitbucket), it enables collaboration with others.
Branching in Git allows you to diverge from the main line of development, enabling you to work on features or fixes separately without affecting the main codebase. This is essential for maintaining organized and effective syncing among team members.
A commit is a snapshot of your changes. Each commit acts as a checkpoint that you can revert to or reference later, so it’s essential to commit often and with clear commit messages.
Git Remote
Understanding the distinction between local and remote repositories is vital. A remote repository is a version of your project that is hosted on the internet or a network. This allows multiple developers to collaborate on a project seamlessly.
Some commonly used remote platforms include:
- GitHub: Widely used, especially for open-source projects.
- GitLab: Offers additional features like CI/CD.
- Bitbucket: Popular for private repositories and team collaboration.
Push and Pull
Understanding Push Using the command `git push` sends your committed changes from your local repository to the remote repository. It’s crucial for sharing your work with your team and ensuring that collaboration is seamless.
For example, to push your changes to the main branch on your remote repository, you would use:
git push origin main
Understanding Pull The `git pull` command is your go-to for updating your local repository with changes from the remote repository. This command fetches and merges changes so that you're always working with the latest code.
You can execute:
git pull origin main
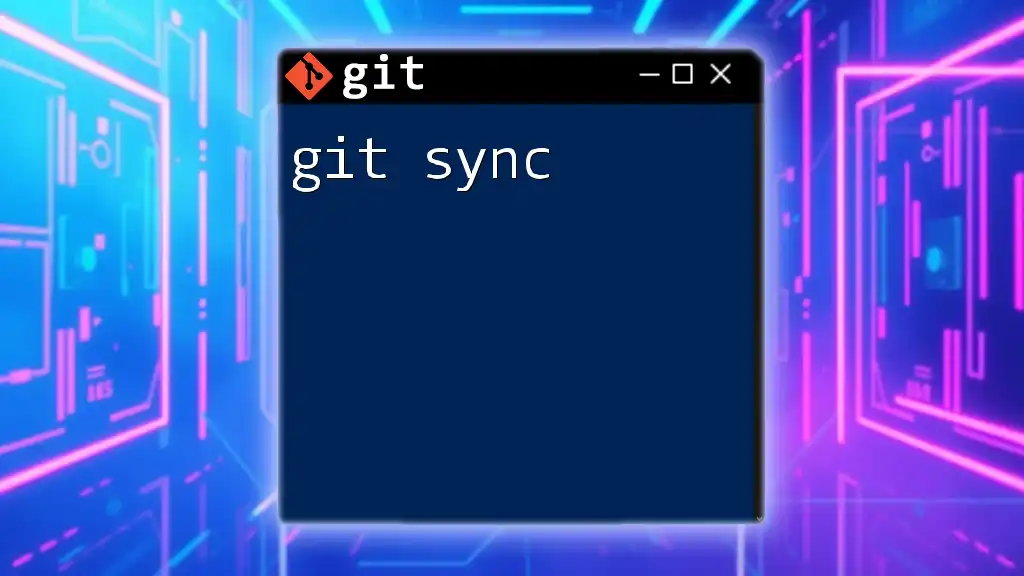
Setting Up Your Remote Repository
Creating Your First Remote Repo
To get started with Git syncing, you first need a remote repository. For instance, creating a new repository on GitHub is straightforward:
- Log in to your GitHub account.
- Click on the "New" button in the repositories section.
- Fill out the repository name and description.
- Choose public or private, then create the repository.
Linking Your Local Repository to Remote
After creating the remote repository, you will need to link it to your local repository. This is done using the command `git remote add`:
git remote add origin https://github.com/username/repo.git
This command establishes a connection between your local and remote repositories, allowing you to push and pull changes seamlessly.
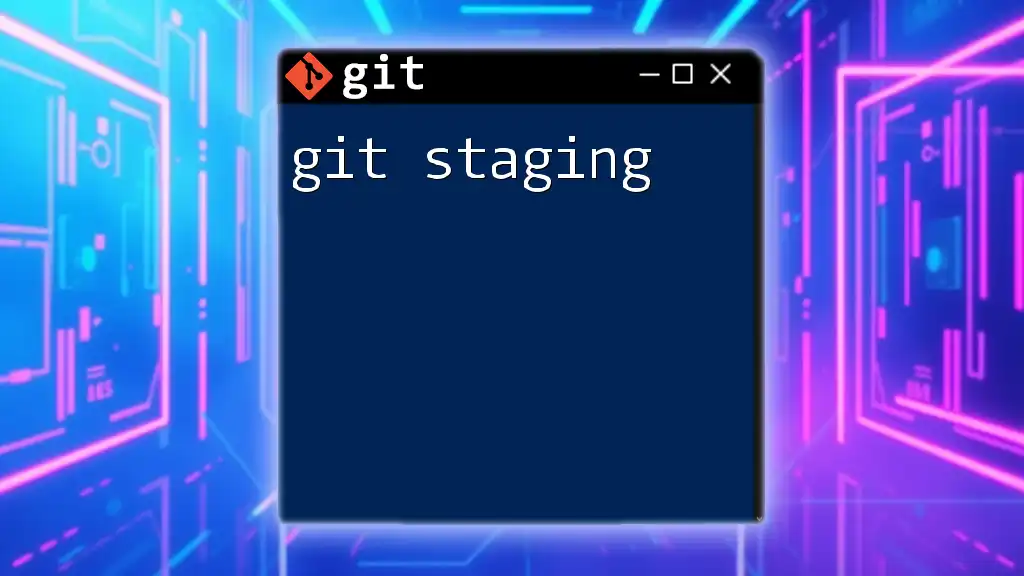
The Syncing Process
Best Practices for Syncing
To maintain an effective workflow, consider these best practices while syncing your changes:
- Keep Your Local Repository Updated: Regularly pull changes from the remote repository to avoid conflicts.
- Commit Changes Frequently: This not only saves your progress but also allows for clearer version tracking.
- Use Clear Commit Messages: Descriptive messages will save you and your team effort when going through project history.
Steps to Sync Your Changes
Syncing Your Local Repository to the Remote
When you’re ready to share your work, follow these steps:
-
Stage your changes:
git add .
-
Commit your changes with a message:
git commit -m "Your commit message"
-
Push your changes to the remote repository:
git push origin main
Fetching Updates from the Remote
To get the latest changes from your team, it's critical to fetch before you proceed with your own work. Use the command:
git fetch origin
This command will retrieve the latest updates without merging them into your current branch automatically.
Handling Merge Conflicts
What is a Merge Conflict?
A merge conflict arises when two developers have made changes to the same line of code or when one person has deleted a file that another has modified. These conflicts must be resolved before the code can be successfully merged.
Resolving Merge Conflicts
Resolving conflicts involves several steps:
- Identify the conflicting files Git will mark them.
- Open the files and look for conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`).
- Edit the file to resolve the conflicts.
- After resolving, stage the changes:
git add <file>
- Commit the resolved changes.
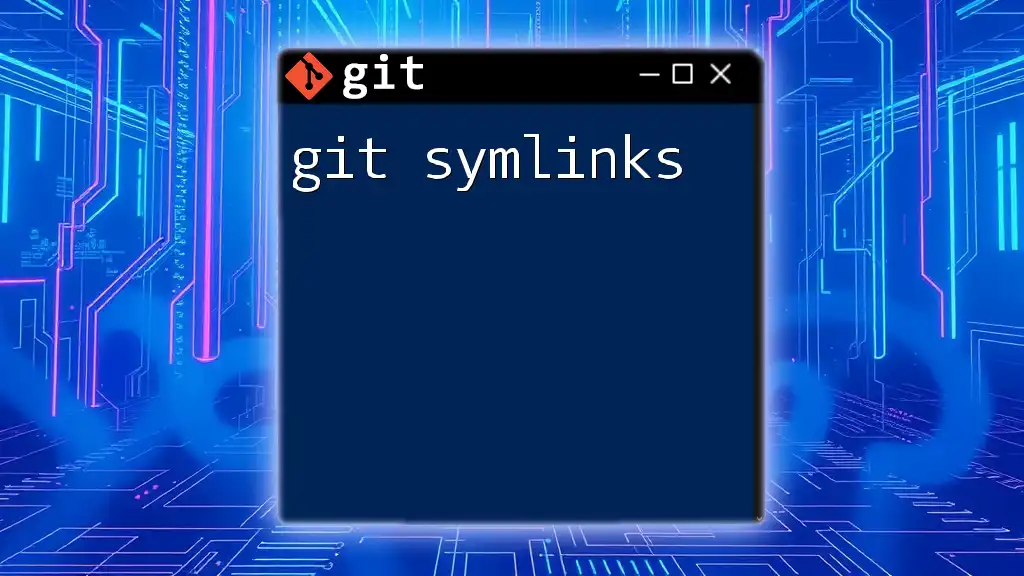
Advanced Syncing Techniques
Using `git rebase` for Cleaner History
A powerful tool in Git syncing is `git rebase`. This command allows you to apply a sequence of commits from one branch onto another, leading to a cleaner project history.
For instance, to rebase the current branch onto the main branch, you would execute:
git rebase origin/main
This replaying of commits results in a linear project history, which can make the log much cleaner and easier to understand.
Checking Sync Status with Git Status and Log
To monitor the health of your repository, you can check the status and log of your commits using:
-
Checking Status:
git status
This command will show you the current state of your working directory and staging area.
-
Checking Log:
git log --oneline --graph --decorate
This command displays a graphical representation of your commit history, making it easier to visualize branches and merges.
Dealing with Large Repositories
For large repositories, syncing can become a challenge. Here are some strategies to maintain efficiency:
- Using .gitignore: Create a `.gitignore` file to specify which files should not be tracked by Git. This can prevent unnecessary files from being pushed, speeding up your sync process.
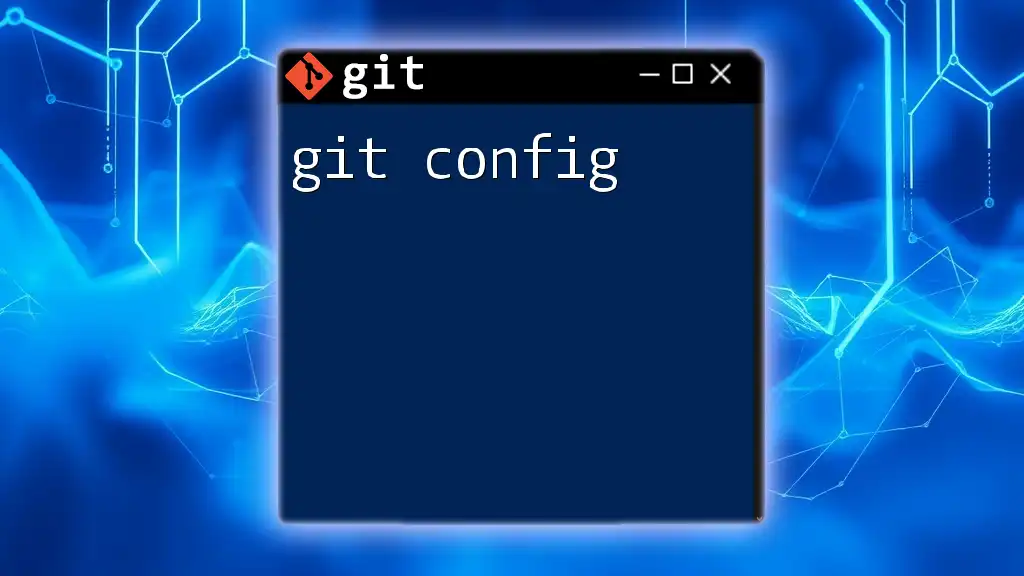
Troubleshooting Common Issues
Sync Errors and Solutions
One common issue is the non-fast-forward error that occurs when your local branch is behind the remote branch. To remedy this, you generally need to pull the latest changes before pushing:
git pull origin main
Keeping Your Team Synchronized
Utilizing Git hooks can help automate processes that keep your team synchronized. For instance, a pre-push hook can run tests before allowing a push to the remote repository, promoting code quality across your team.
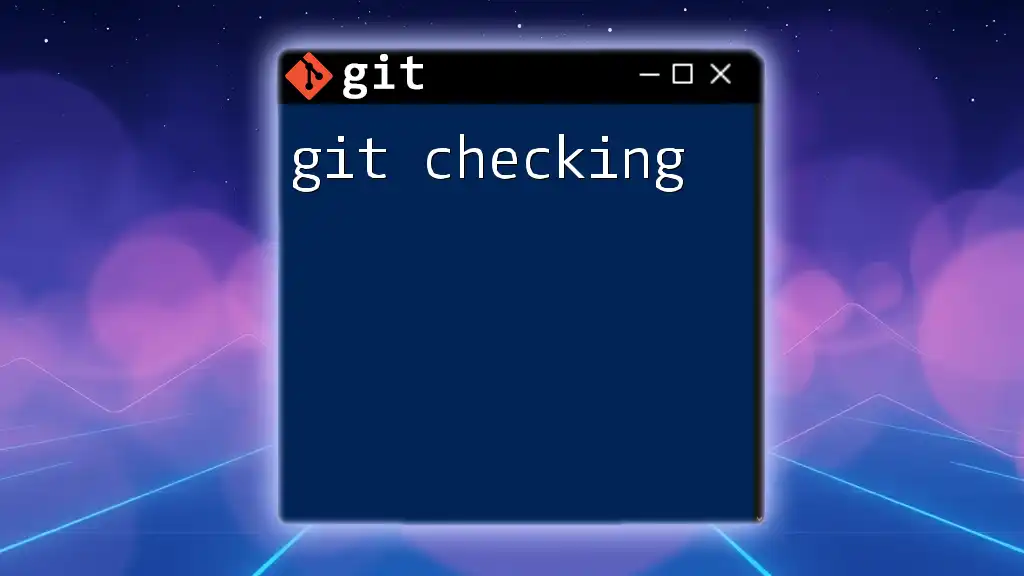
Conclusion
This guide has covered essential concepts and practices surrounding git syncing. With the knowledge of push and pull commands, handling merge conflicts, and employing advanced techniques like rebase, you're now equipped to collaborate effectively on your projects.
Embrace the practice of syncing regularly, use clear commit messages, and remember to stay informed about any changes made by your team. With time and experience, git syncing will become a natural and integral part of your workflow.
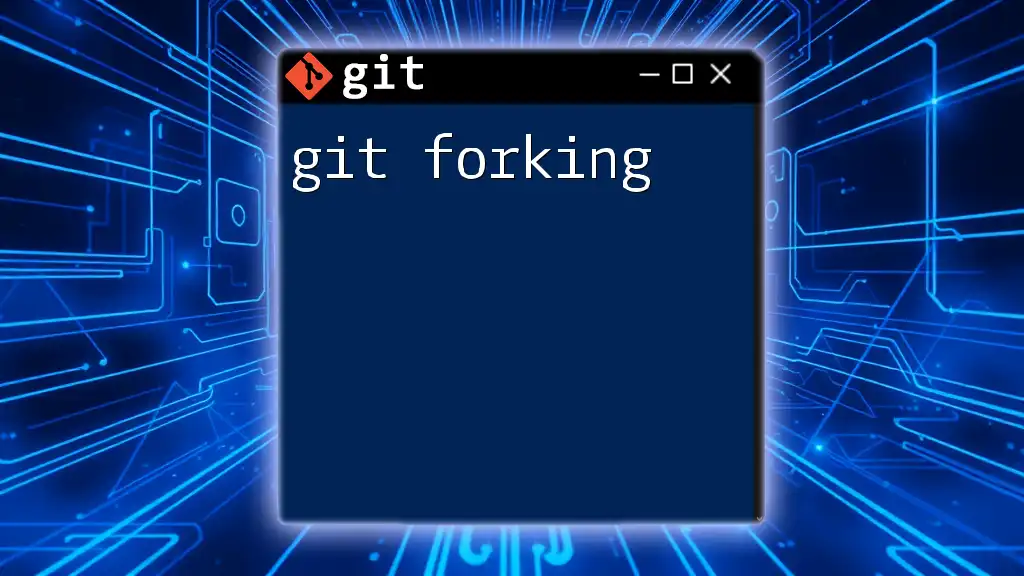
Additional Resources
For further reading and exploration, check out the official Git documentation, recommended books, and online courses. Engaging with community forums can also be an excellent way to enhance your understanding and keep up with best practices in the ever-evolving world of Git.
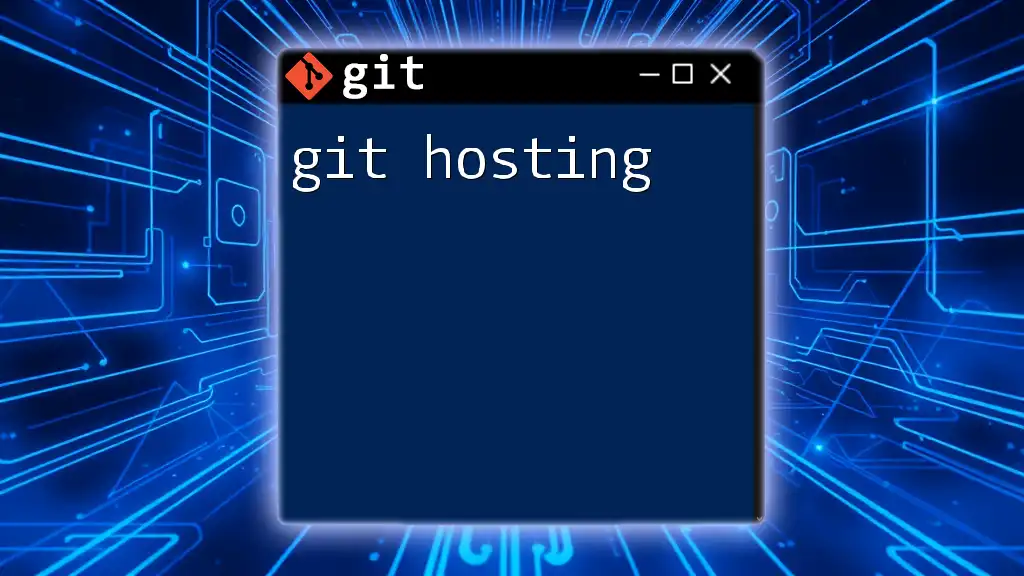
Call to Action
If you are eager to deepen your Git knowledge, consider signing up for our comprehensive Git learning courses. Join our online community for support, tips, and best practices on your journey to becoming a Git expert!