The `git config --global` command is used to set global Git configuration options for all repositories on your system, such as user name and email.
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Understanding Git Configuration
What is Git Configuration?
Git configuration refers to the settings that influence how Git behaves in different contexts. It governs everything from user identity to performance settings and is pivotal for effective version control management.
Overview of Configuration Levels
Git configuration exists at three levels:
-
System Configuration:
- This applies to all users on the system. You can find these configurations in a system-wide Git configuration file, typically located under `/etc/gitconfig`.
-
Global Configuration:
- The global config affects the user's environment. Settings made here apply to all repositories for the specific user. The file is generally located at `~/.gitconfig`.
-
Local Configuration:
- Local configuration is specific to a single Git repository. This means settings made here will override both global and system configurations for that repository. The local config file is found within the `.git` directory of the repository.
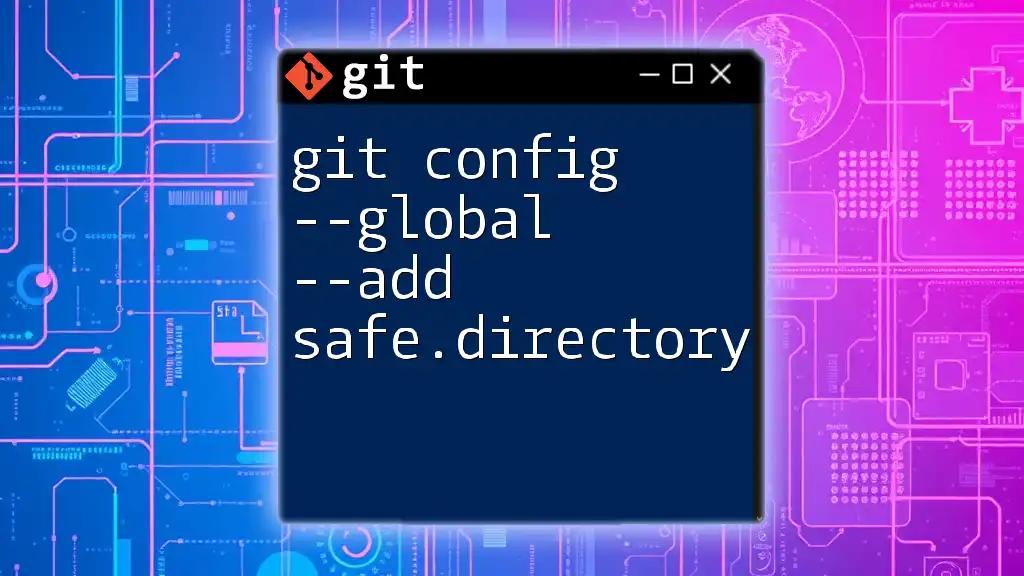
What is `git config --global`?
Purpose of Global Configuration
The `git config --global` command allows you to establish preferences that affect all your projects. For example, when you set your user name and email globally, Git will automatically use this information with every commit you make, ensuring a consistent identity across repositories.
Common Use Cases
Using `git config --global`, you can accomplish several tasks, such as:
- Setting your user information (name and email).
- Configuring your default text editor.
- Adjusting settings for line endings and merging.
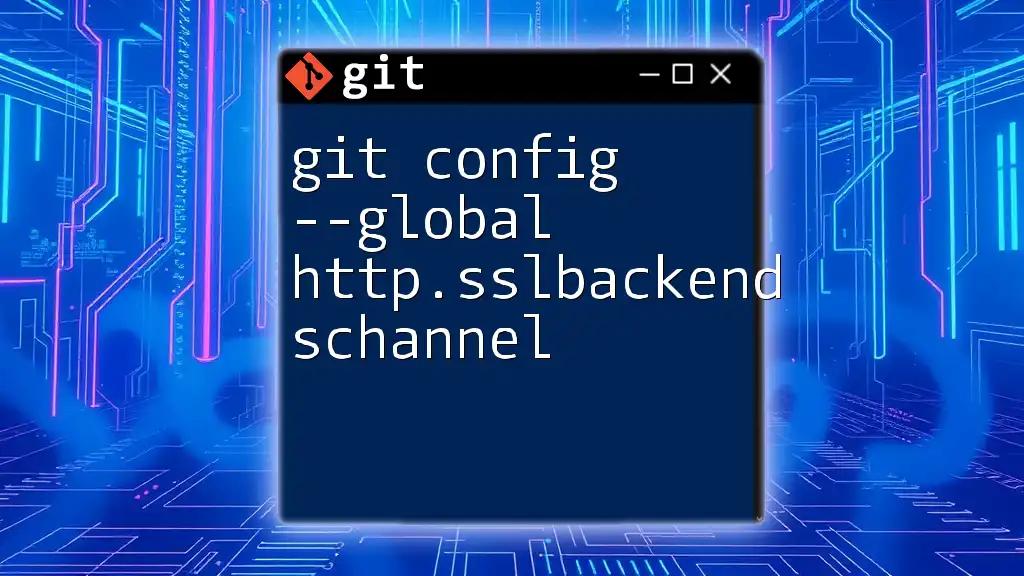
Setting Up Global Configuration
Setting Global User Information
To set your user name, you can use the following command:
git config --global user.name "Your Name"
This configuration ensures that your commits reflect your actual name, which is essential for collaboration and accountability in version control.
Next, to set your email, use:
git config --global user.email "your.email@example.com"
Including your email is equally crucial as it identifies you as the author of commits. This information becomes visible in the commit history and is invaluable, especially in open-source or collaborative projects.
Configuring Default Editor
You can specify a default text editor for Git, which will be used when you need to enter messages (e.g., when committing).
To set this, use:
git config --global core.editor "editor-name"
Replace `"editor-name"` with your preferred text editor, such as `vim`, `nano`, or `code` for Visual Studio Code. This eliminates frustration when Git prompts you to input messages, giving you a comfortable working environment.
Additional Global Options
Configuring Line Endings
To enhance compatibility across different operating systems, you can configure line endings:
git config --global core.autocrlf true
This setting automatically converts line endings to LF (Linux/Unix) upon committing but converts back to CRLF (Windows) when checking out files, protecting your repository from potential issues.
Enabling Color Output
Make your Git commands visually distinct by enabling color output:
git config --global color.ui auto
With this setting, commands provide color-coded output, significantly improving readability and making it easier to spot changes.
Setting Different Configuration Parameters
You can customize various aspects of Git using additional parameters. For example, using aliases can simplify your command input:
git config --global alias.st status
Now, you can simply type `git st` instead of `git status`.
You may also manage pull behaviors with:
git config --global pull.rebase true
This helps streamline merge workflows and can result in a cleaner commit history.
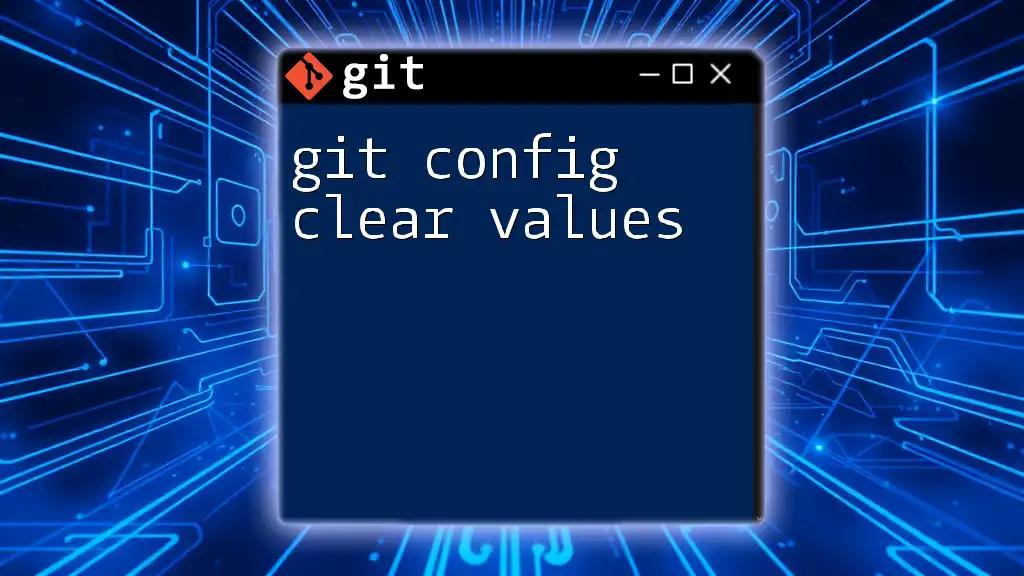
Viewing and Editing Global Configuration
Viewing the Global Configuration
At any time, you can view your global configuration settings by executing:
git config --global --list
This command displays all the settings you’ve applied, along with their respective values. Understanding your current configurations is essential for maintaining an organized and functional workflow.
Modifying Existing Configuration
If you need to update an existing setting, such as your email, you simply run:
git config --global user.email "new.email@example.com"
This command will overwrite the previous email address in your global configuration, keeping your information up to date.
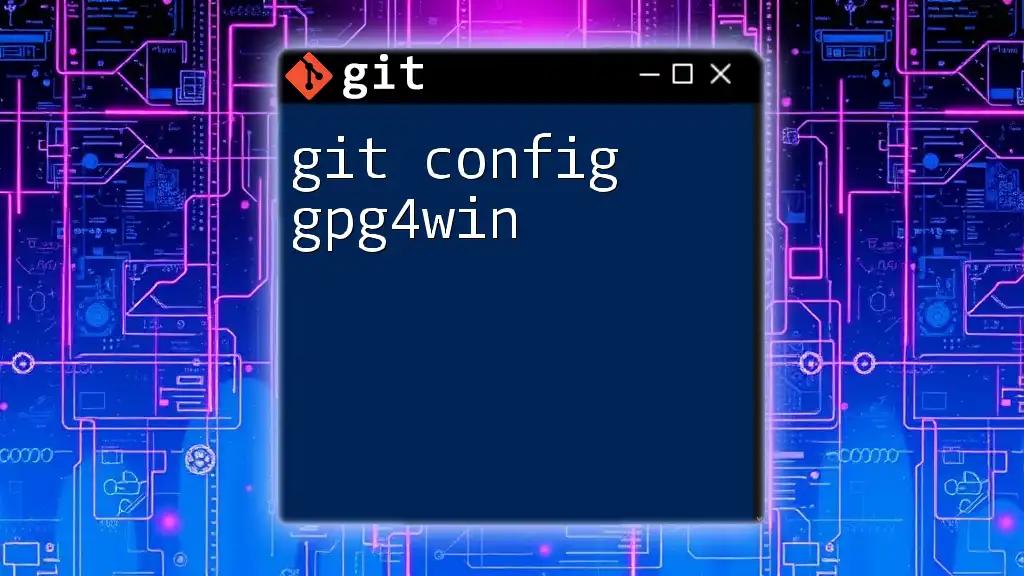
Removing Global Configuration
Deleting a Global Setting
If you need to remove a specific global setting, you can use the `--unset` command:
git config --global --unset user.email
This command deletes the global email configuration, which may be necessary if switching to a different address or when you no longer need a specific setting.
Resetting to Default Configuration
While you can modify individual settings, you might wish to remove all global configurations. This can be achieved by manually editing the `~/.gitconfig` file and deleting unwanted entries.
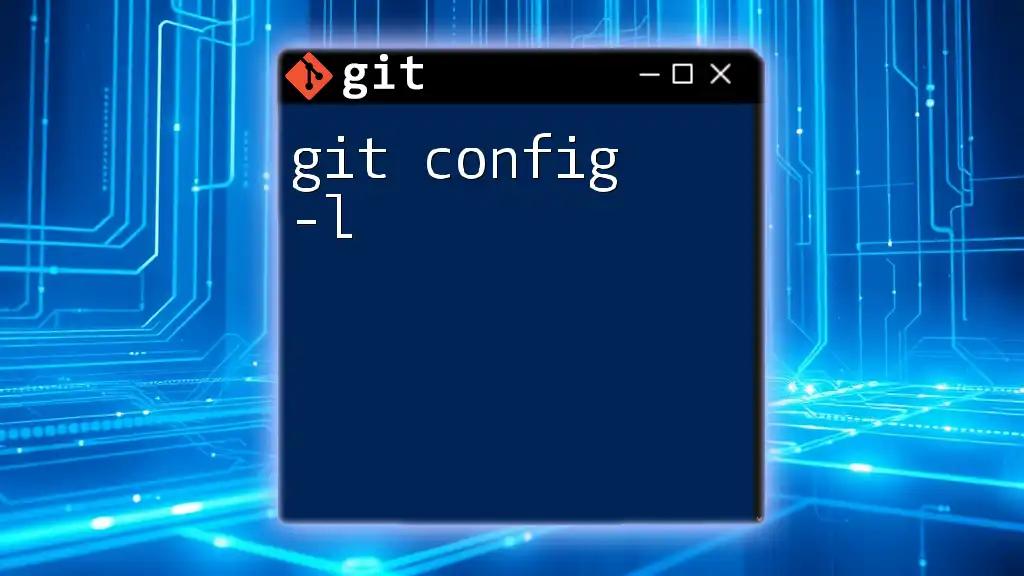
Troubleshooting Common Issues
Common Errors
Issues often arise from misconfigurations in the global settings. For instance, if you forget to set your user email, Git will raise an error when attempting to commit changes. Always check your configuration settings if you encounter such issues.
Checking Configuration Conflicts
Sometimes, conflicts may arise between local and global configurations. Use the commands:
git config --list --show-origin
This command shows where each configuration variable is defined, helping you identify where conflicts occur.
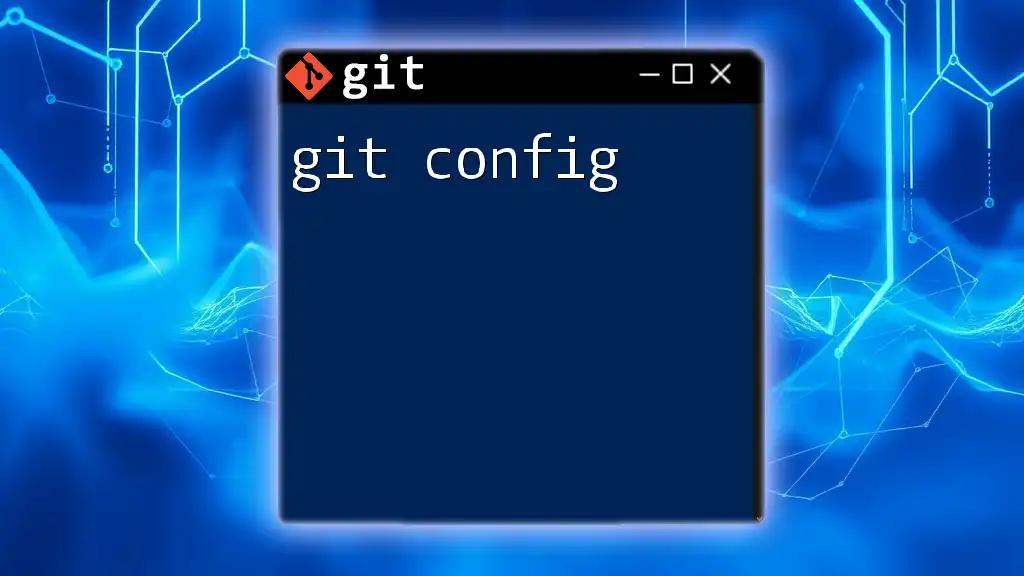
Best Practices for Global Configuration
Use Meaningful User Information
Using your real name and email ensures clarity in contributions, especially when collaborating with teams or contributing to public repositories. It's a best practice that encourages transparency and trust within the Git community.
Regularly Review Your Settings
Periodically revisit and review your global settings to ensure they remain relevant. As your projects evolve or change, so too should your Git configurations. Consistency is key for a streamlined workflow.
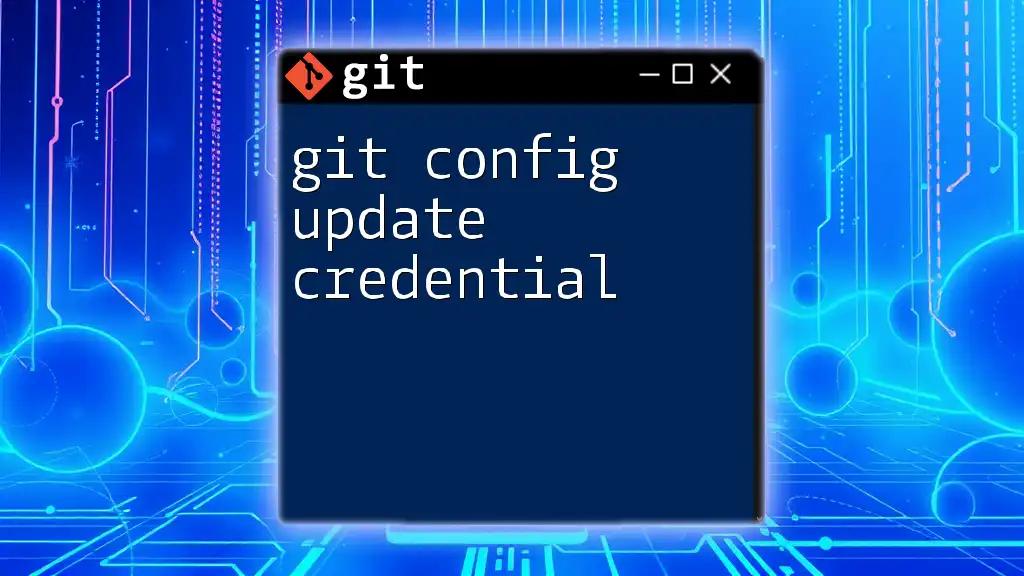
Conclusion
By harnessing the power of `git config global`, you streamline your Git experience and maintain consistent practices across repositories. By setting up user information, default editors, and additional configurations, you enhance both personal and team collaboration in version control. This efficient setup is critical for Git's successful application, allowing you to focus on what truly matters: your code.
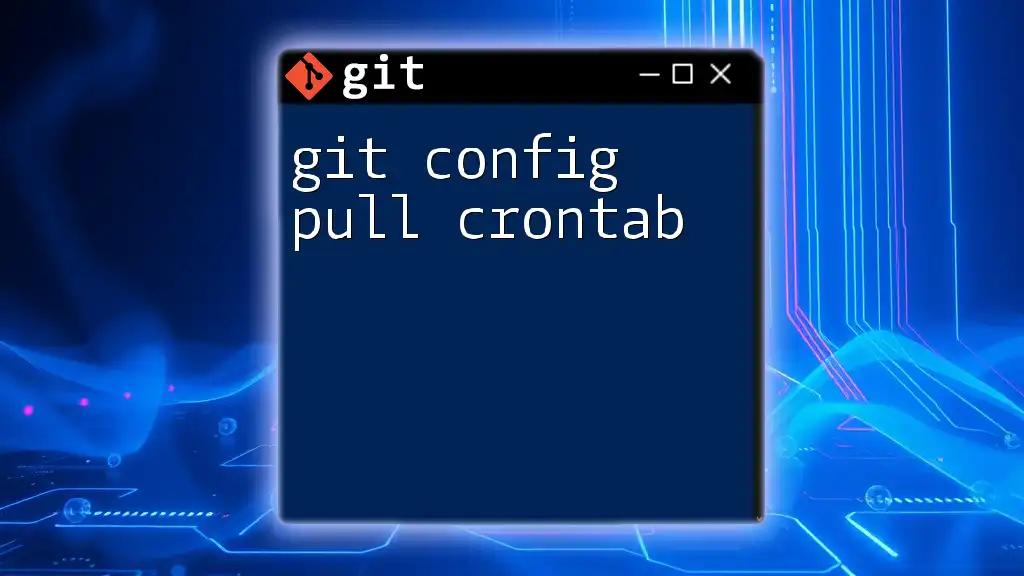
Additional Resources
For further learning, consult the official Git documentation and explore various blogs and tutorials tailored to improving your Git skills. Happy coding!