To configure Git to use a specific pull behavior and set up a crontab job for automation, you can use the following commands:
git config --global pull.rebase true
crontab -e
# Then add a line to schedule the git pull command, for example, to run every hour:
0 * * * * cd /path/to/your/repo && git pull
Understanding Git Config
What is Git Config?
The `git config` command is a vital tool for managing your Git environment. It allows you to set configuration options that control the behavior of Git functions. There are three levels of configuration:
- Global: Affects all repositories for a particular user.
- System: Applies to all users on a system.
- Local: Specific to a single repository.
By modifying these configurations, you can tailor Git to your preferences and needs.
Key Git Config Commands
Some essential commands include:
-
Setting your global username and email: This information is associated with your commits.
git config --global user.name "Your Name" git config --global user.email "youremail@example.com"
-
Choosing a default editor: Customize how you edit commit messages or other Git commands.
git config --global core.editor "code --wait"
Setting Up Your Git Environment
To set up Git effectively on your system, follow these steps:
- Install Git following the official documentation for your operating system.
- Open a terminal or command prompt.
- Execute the `git config` commands mentioned above to customize your setup.
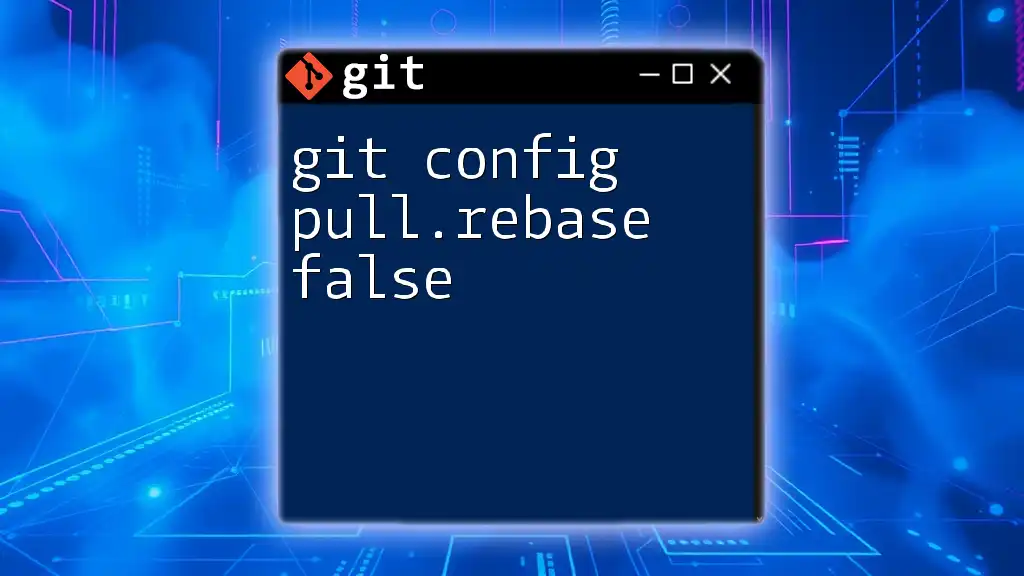
The Git Pull Command
What is Git Pull?
The `git pull` command is fundamental in ensuring that your local repository is up-to-date with its remote counterpart. It fetches data from the specified remote repository and merges changes into your current branch.
How to Use Git Pull
The syntax for `git pull` is straightforward:
git pull [<options>] [<repository> [<refspec>...]]
Common use cases include:
- Collaborating on Projects: Regularly updating your local copy to integrate others' contributions.
- Deployment: Fetching the latest code onto production servers.
Example: To pull the latest changes from the main branch on the origin remote, use:
git pull origin main
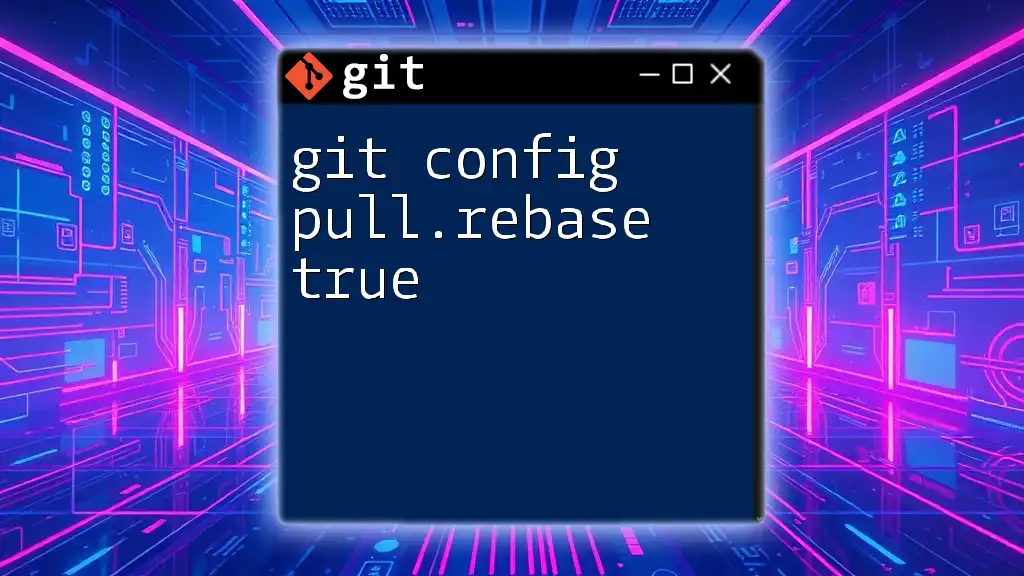
Introduction to Crontab
What is Crontab?
Crontab, short for “cron table,” is a time-based job scheduler in UNIX-like operating systems. It enables you to run scripts or commands at scheduled intervals, such as every minute, hour, or day.
Examining the Crontab Syntax
The syntax of a crontab entry consists of five fields followed by the command to execute. Each field corresponds to a time unit:
- Minute (0-59)
- Hour (0-23)
- Day of the month (1-31)
- Month (1-12)
- Day of the week (0-7, where both 0 and 7 represent Sunday)
Example: A crontab entry to run a command every day at midnight looks like this:
0 0 * * * /path/to/your/script.sh
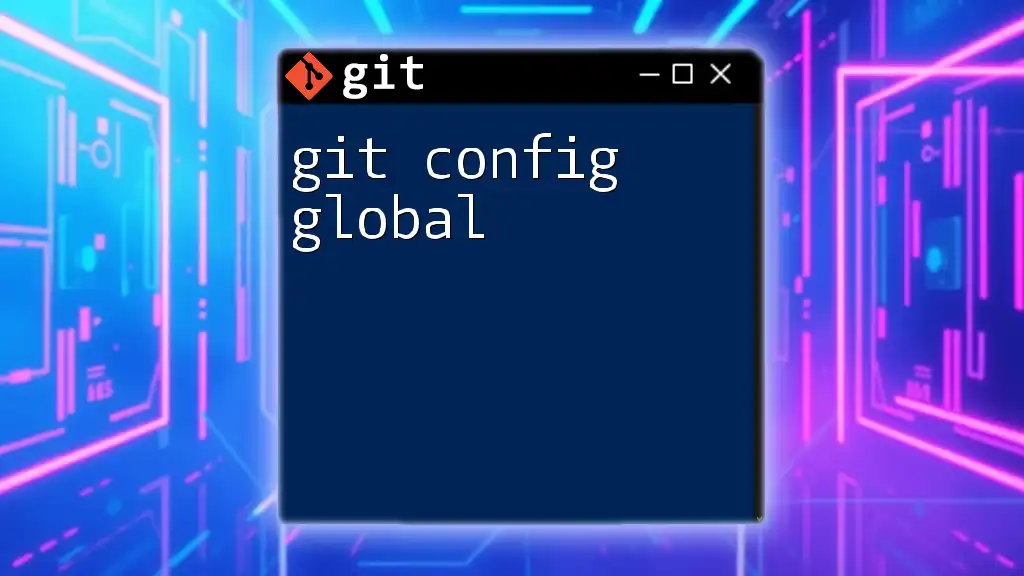
Combining Git Pull with Crontab
Why Use Crontab for Git Pull?
Automating `git pull` via crontab can be a game changer in maintaining a synchronized local repository without manual intervention. This setup is particularly beneficial if your development workflow relies on frequent updates from a shared project.
Setting Up a Crontab Entry for Git Pull
To have your system execute a `git pull` command automatically, you will need to set up a crontab entry. This involves the following steps:
- Open the terminal.
- Type `crontab -e` to edit your crontab file.
- Add an entry specifying when to execute the command.
Example:
* * * * * cd /path/to/your/repo && git pull origin main
In this example, the command changes the directory to your Git repository and executes a `git pull` every minute.
Common Issues and Troubleshooting
When setting up automatic git pulls, various issues may arise:
-
SSH Authentication: If your repository uses SSH, ensure that your SSH keys are correctly set up and that the necessary key is loaded in your SSH agent.
-
Environment Variables: Crontab runs in a limited environment. If you encounter problems, check if the required environment variables are set, especially if your repository requires specific paths.
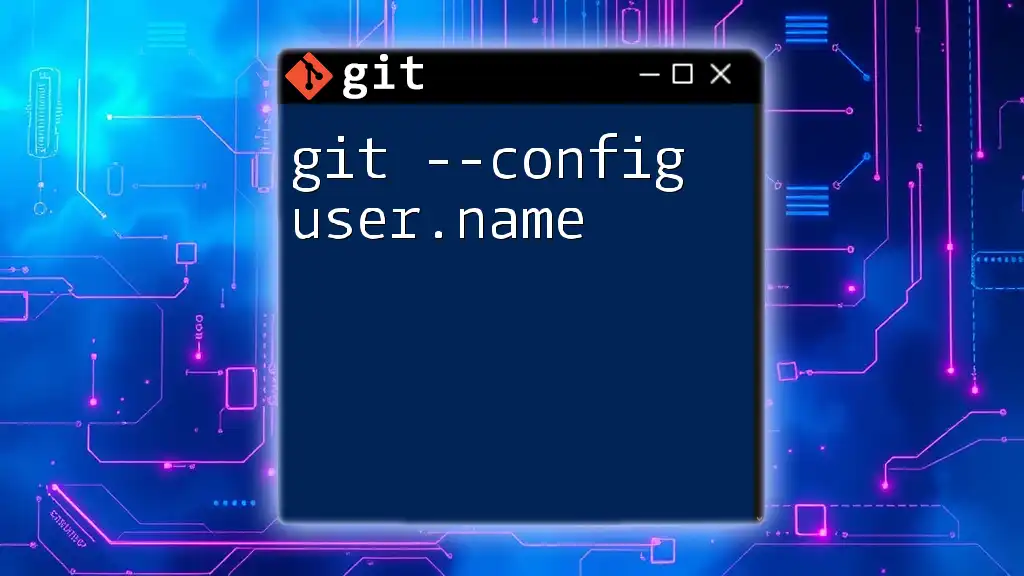
Best Practices for Using Git with Crontab
Minimizing Conflicts
Automating pulls can lead to conflicts if there are uncommitted changes or if pulls happen before local work is completed. To manage this, ensure you commit your changes frequently or set up a strategy to handle merges effectively.
Log Output for Troubleshooting
Logging the output of your crontab jobs is essential for diagnosing issues. To do so, you can redirect output to a log file:
* * * * * cd /path/to/your/repo && git pull origin main >> /var/log/git-pull.log 2>&1
By using `>> /var/log/git-pull.log 2>&1`, any errors or messages from the pull command will be captured in the specified log file, providing insight into what happens during each execution.
Security Considerations
Automating git pulls raises security concerns regarding credentials. To avoid risks, utilize credential managers or SSH keys instead of storing plain-text passwords. This layer of security ensures safer interactions with your repositories.
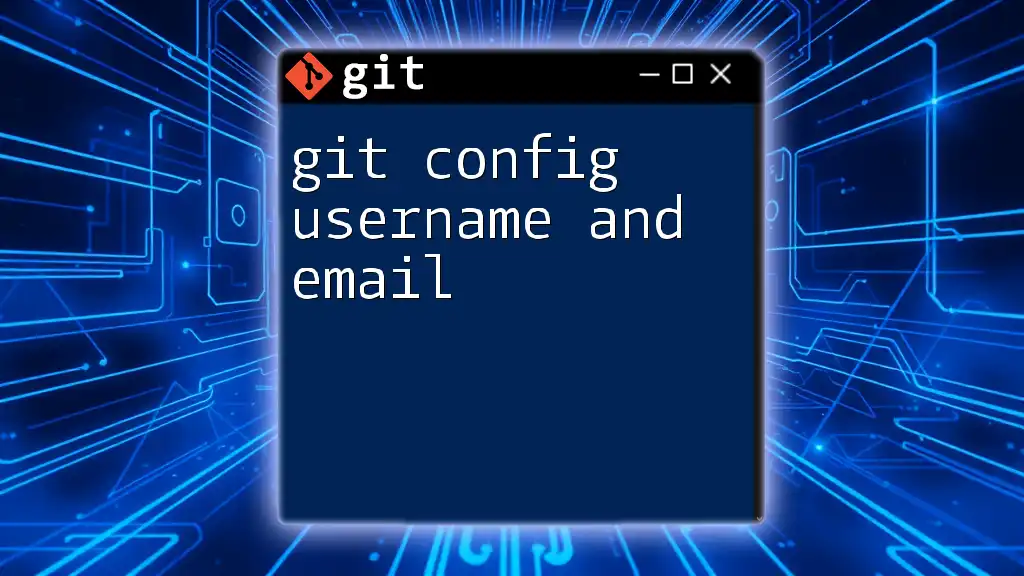
Conclusion
Combining git config, the git pull command, and crontab can dramatically enhance your workflow. By automating the process of pulling updates from remote repositories, you can ensure that your local environment remains current, allowing you to focus on what truly matters: your development work.
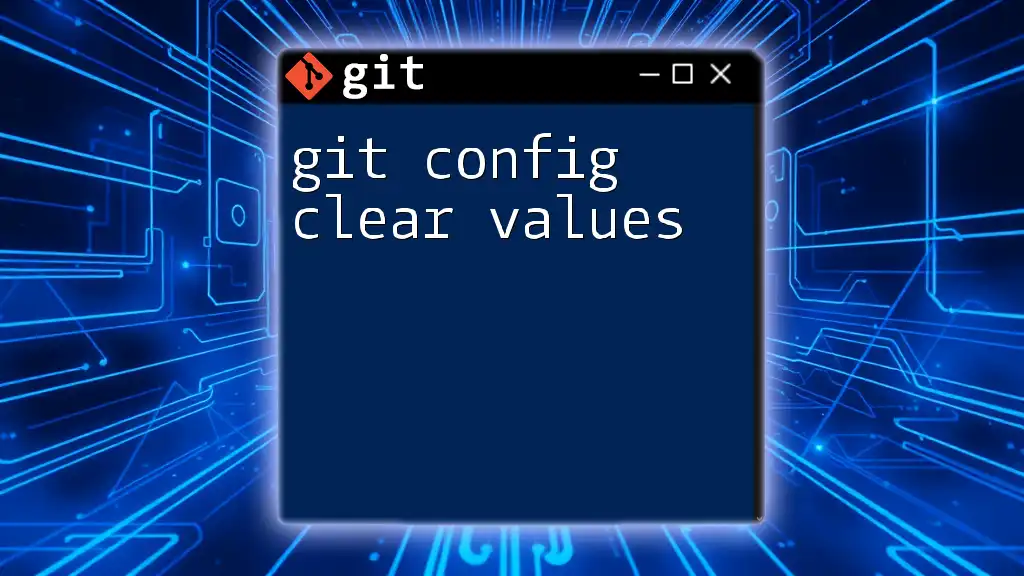
Additional Resources
Visit the official Git documentation for in-depth knowledge on `git config` and `git pull`, and explore resources on crontab management for further learning.
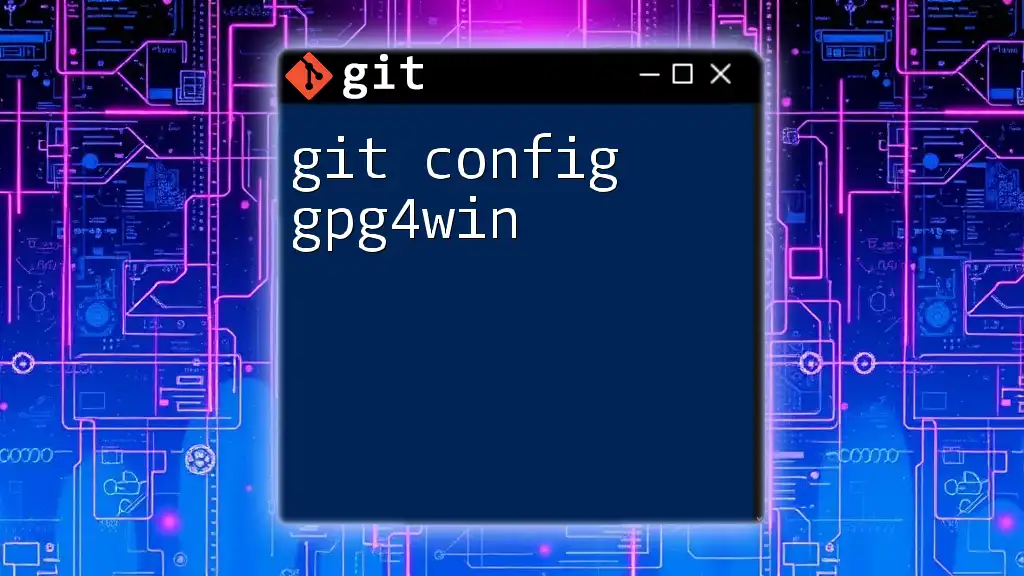
FAQs
-
What if my repository requires authentication? Make sure your SSH keys are set up properly, or consider using a credential caching helper.
-
Can I pull from multiple repositories? Yes, you can create multiple crontab entries for different repositories.
-
How do I stop a crontab job? Edit your crontab using `crontab -e` and remove or comment out the desired entry.