The `git config set token value` command is used to specify a personal access token for Git operations, enhancing security while interacting with remote repositories.
git config --global credential.helper store
git config --global user.token <your_token_value>
What is `git config`?
Understanding Git Configuration
Git is structured with three different configuration levels that dictate how settings are applied. Each of these levels serves a specific purpose:
- System Level: These settings apply to every user on the system and all their repositories. They are stored in the Git installation directory.
- Global Level: These configurations are specific to a user and apply to all repositories owned by that user. They are stored in the user’s home directory in a file named `.gitconfig`.
- Local Level: This setting applies to a specific repository and is stored in the `.git/config` file within that repository.
Each of these levels can hold various settings that dictate how Git behaves in different contexts.
Types of Config Settings
Git configuration settings can be categorized into core, user, remote, and others depending on the needs of the operation. For example, to quickly view your current configuration settings, you would run:
git config --list
This command outputs all settings available at your current context level, giving you a comprehensive overview of how Git is configured on your machine.
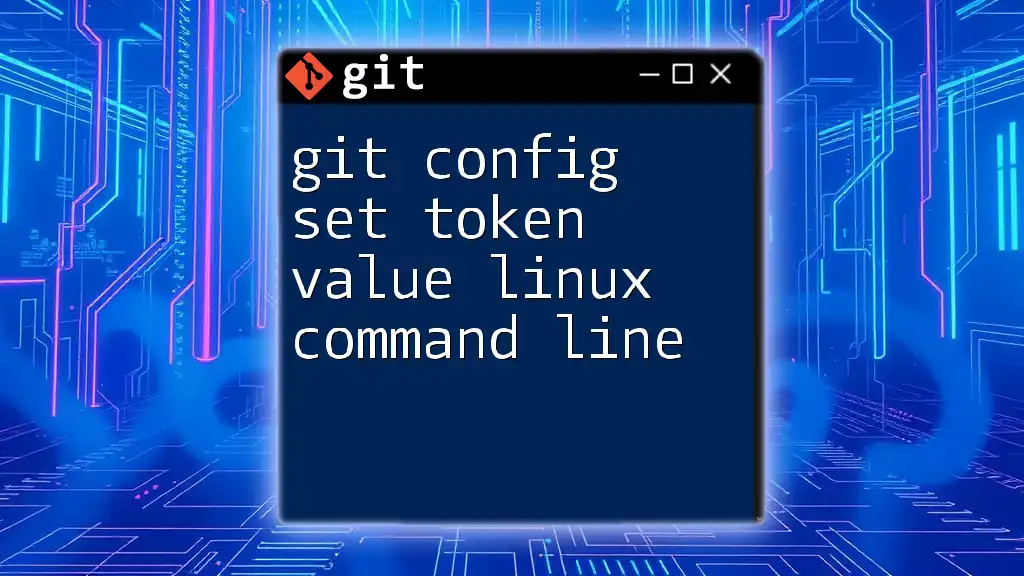
What is a Token Value?
Definition and Importance
In the context of Git, a token value refers to a personal access token (PAT) that acts as a substitute for a password when interacting with repositories, especially in remote settings. This is especially significant for operations such as cloning, pushing, or pulling to a remote repository without requiring your GitHub or GitLab password repeatedly.
Use Cases for Token Authentication
Token authentication simplifies processes in several scenarios, particularly:
- Third-party integrations: When using Continuous Integration/Continuous Deployment (CI/CD) tools that need to access your repositories.
- REST API interactions: When automating tasks that require access to Git operations without exposing your credentials.
By recognizing the importance of a token value, you reinforce secure practices in your Git workflow.
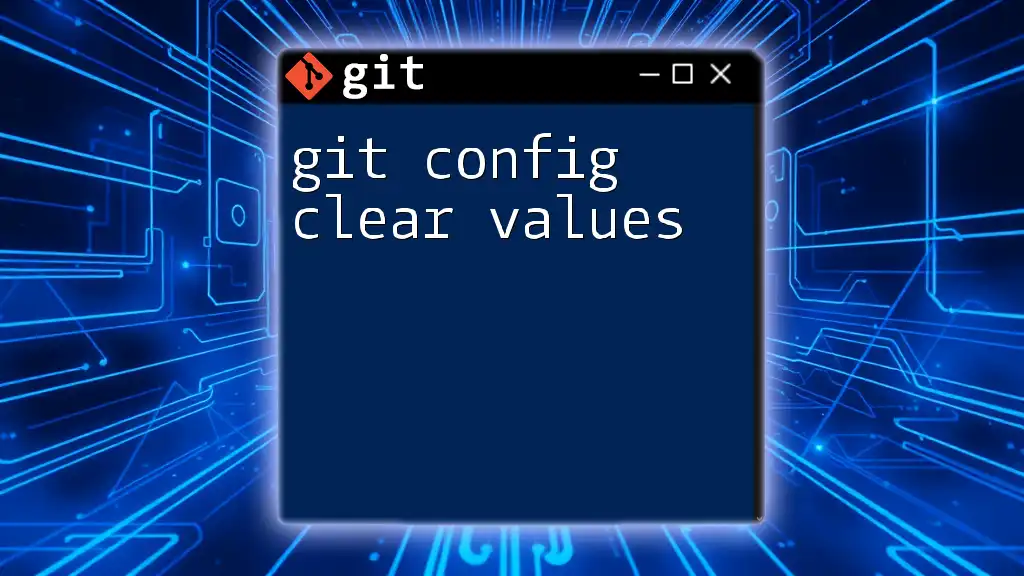
Setting a Token Value with `git config`
Syntax of the Command
To configure Git to use a token value, the following syntax is employed:
git config --global credential.helper <helper>
This command tells Git how to handle credentials, including token values, efficiently.
Steps to Set a Token Value
Step 1: Generate a Personal Access Token (PAT)
The first step in using token values is generating a personal access token. Here's a simplified step-by-step guide for GitHub users:
- Log in to your GitHub account.
- Navigate to Settings > Developer settings > Personal access tokens.
- Click on Generate new token, specify a note, select desired scopes, and click Generate token.
- Copy the generated token immediately—once you leave this page, you won't be able to view it again.
Step 2: Configure Git to Use the Token
Once you have your PAT, you configure Git to leverage this token for authentication:
git config --global credential.helper store
This command allows Git to store the token securely on your machine for future use, making the authentication process seamless.
Persistent Configuration
The `--global` flag in the above command means that this configuration will be applied across all repositories for the user. If you need to set a token for a specific repository, you can run the same command without the `--global` flag while being inside the repository:
git config credential.helper store
This way, you can tailor the configuration according to your project needs without compromising security.
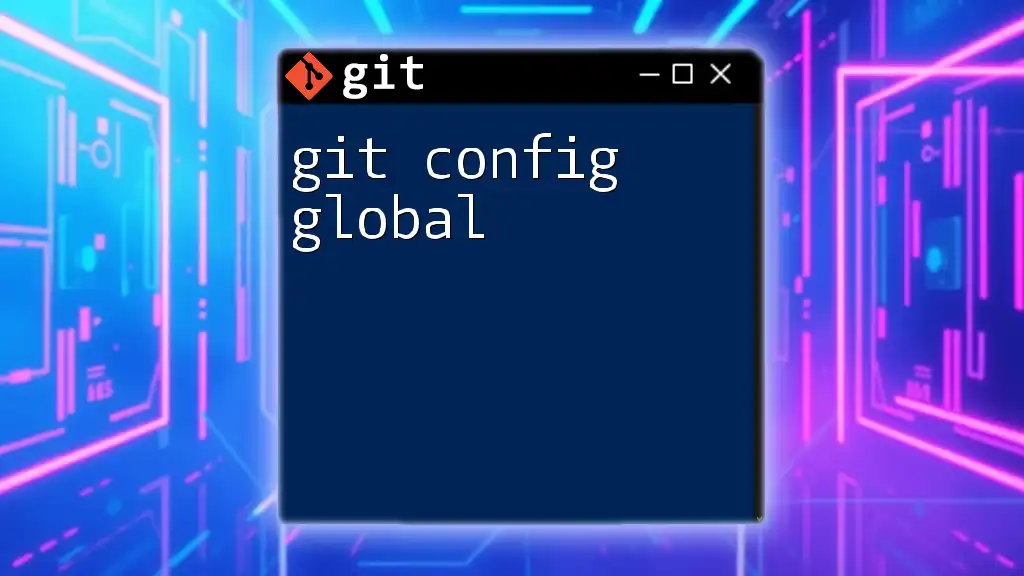
Example: Setting Up Token Authentication in Git
Real-World Example
Imagine you're working on a private GitHub repository and need to push changes frequently. Setting up token authentication can enhance security while easing the process.
- Generate your PAT as mentioned in the previous section.
- Follow the command to store your token:
git config --global credential.helper store
- The next time you push to your repository, Git will prompt for your username and password. Enter your GitHub username and paste your PAT as your password. After this initial prompt, the token will be saved, and you won't need to input it again for future interactions.
Testing Your Configuration
To test if your token setup is correct, make a simple commit and push it to your repository:
git add .
git commit -m "Test commit with token configuration"
git push origin main
If the push goes through without asking for credentials, your token authentication is successfully configured!
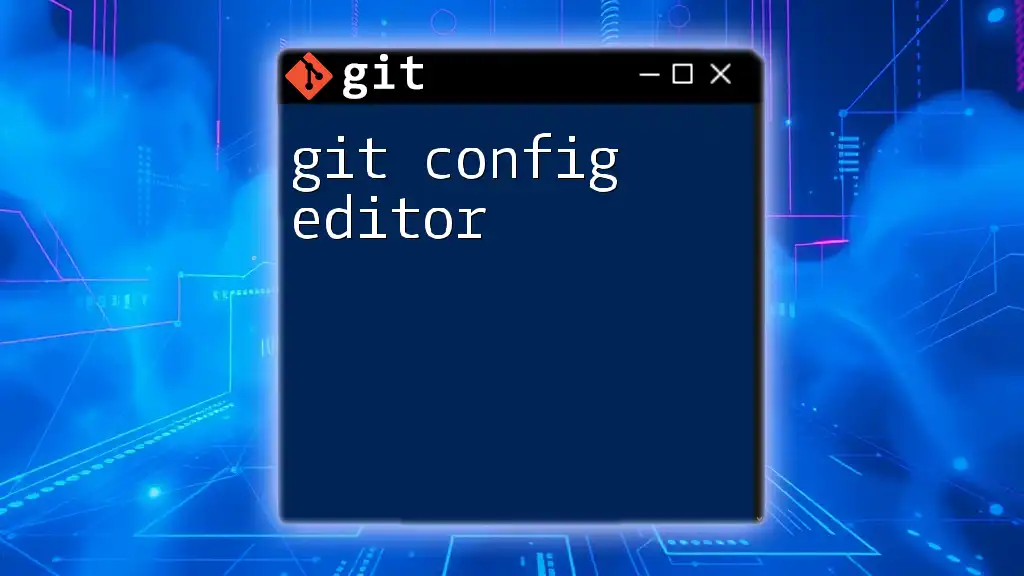
Troubleshooting Common Issues
Token Expiry Problems
One of the common issues with token values is their expiration. Tokens can be set to expire after a certain period as a security measure. If you encounter an authentication error due to an expired token, you will need to generate a new token and configure it again.
Access Denied Errors
If you receive an "access denied" error, it often indicates permission problems associated with your token. Verify that your token has the necessary scopes for the actions you wish to perform. Update the token settings accordingly in the GitHub or GitLab settings.
Checking Your Configuration Again
After making any changes, especially if you suspect an issue, revisit your configuration settings:
git config --list
This knowledge helps you troubleshoot and reaffirm that your token is set up as intended.
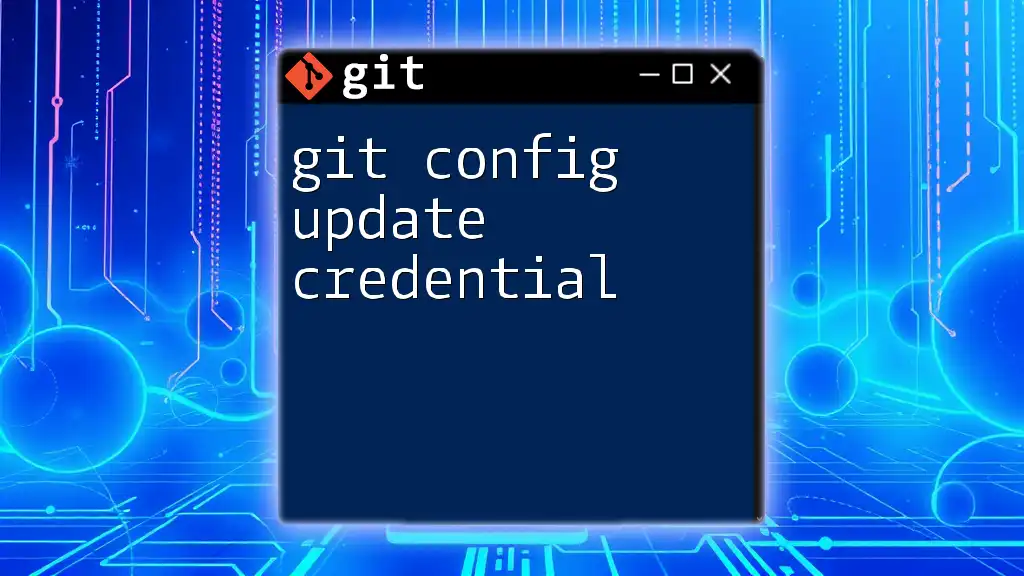
Best Practices for Using Token Authentication
Security Considerations
Properly managing how you store tokens is critical. Avoid storing tokens directly in your source code or using them in scripts that could be exposed. Utilizing environment variables can also add an extra layer of security.
Regularly Updating Tokens
To maintain security, it's recommended to regularly update your tokens and manage access effectively. If you suspect a token has been compromised, revoke it immediately and create a new one.
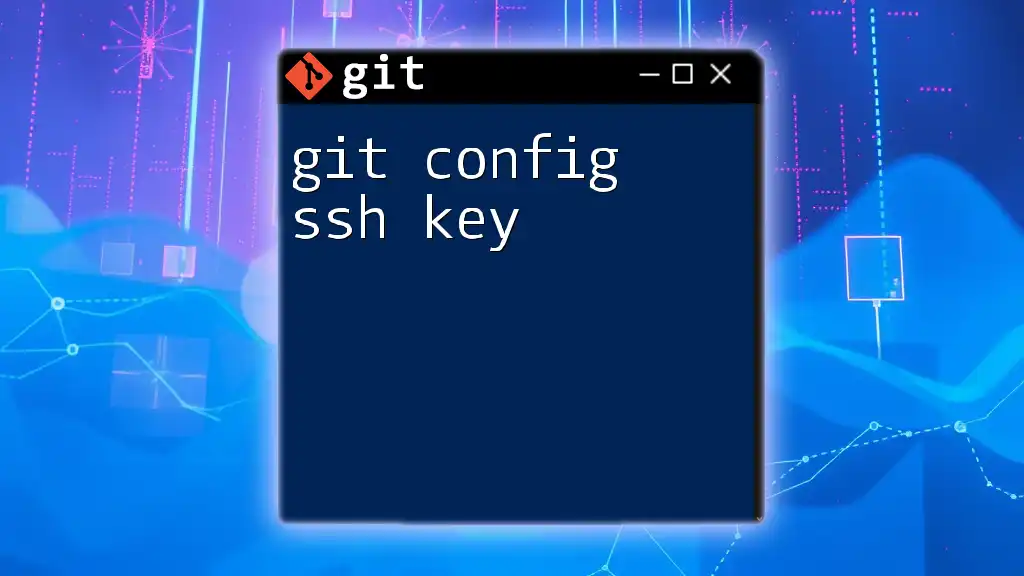
Conclusion
Setting up a token value with `git config` is a crucial step for any developer looking to enhance the security of their Git operations. By embracing this practice, you not only protect your credentials but also streamline and simplify your Git workflow.
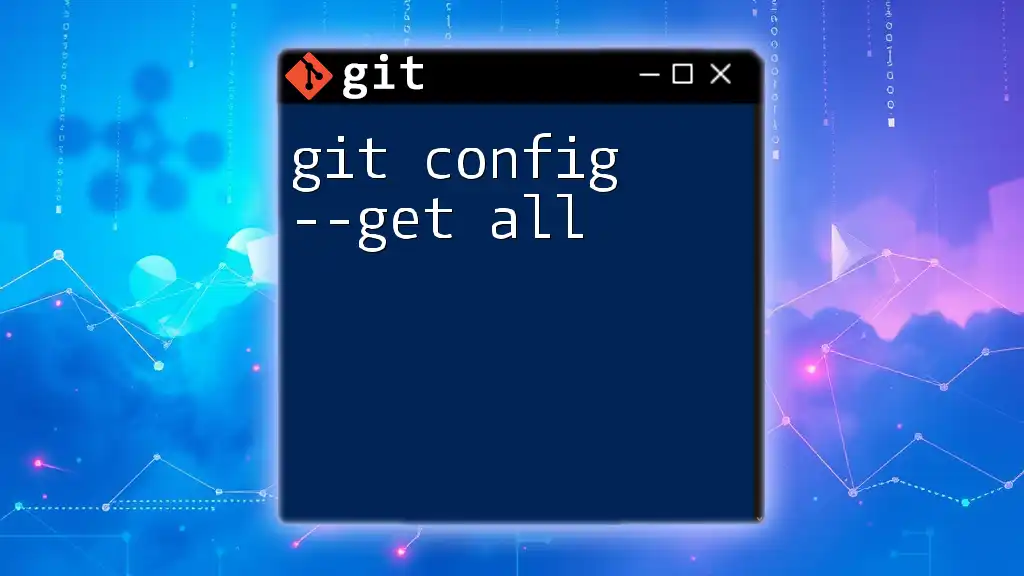
Additional Resources
For further reading and exploration, you can refer to Git's official documentation, which provides in-depth resources on configuration and authentication, as well as extensive tutorials for those looking to deepen their understanding of Git's capabilities.
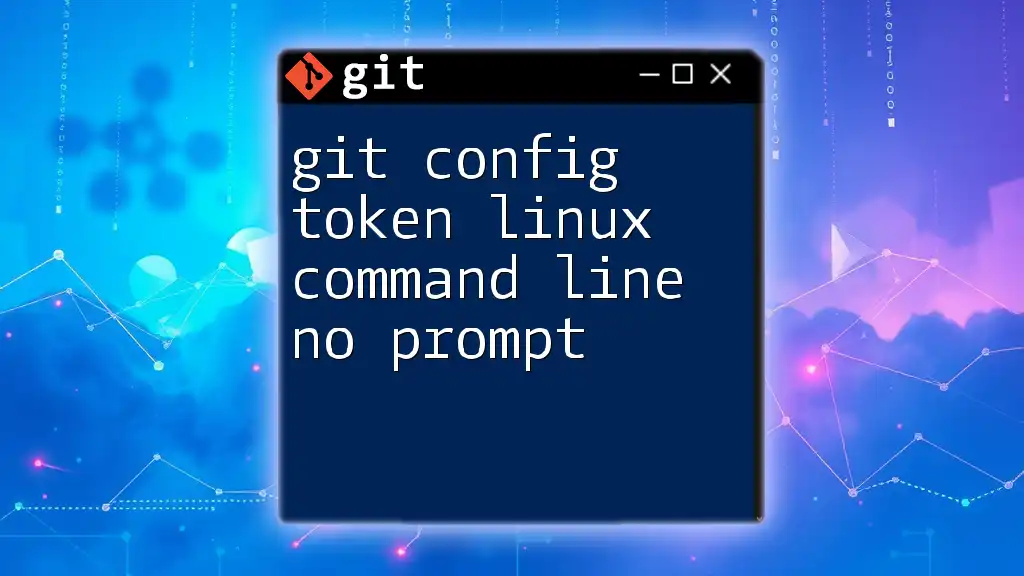
Call to Action
We encourage you to share your experiences and challenges with token authentication in Git. Subscribe for more concise guides and tips on mastering Git commands effectively!