To configure Git to use a personal access token without prompting for input in the Linux command line, you can set the token directly in the remote URL.
git remote set-url origin https://<TOKEN>@github.com/username/repository.git
Understanding Git Tokens
What is a Git Token?
A Git token is a secure, unique string used for authentication instead of a username and password combination. Tokens are particularly important for services like GitHub, GitLab, and Bitbucket, where managing access and permissions is critical.
When to Use Tokens:
- When integrating Git with CI/CD pipelines.
- When accessing Git repositories via APIs.
- When performing operations that involve sensitive data or automation that shouldn't expose your credentials.
Creating Your Git Token
To effectively use the git config token linux command line no prompt strategy, you'll first need to create a Git token. Here's how to do it:
- Visit Your Git Service Provider: Log into your Git account (e.g., GitHub).
- Navigate to Settings: Locate the Developer settings or Access tokens section.
- Generate a New Token: Choose the appropriate scope for your token. For example:
- `repo` gives full control of private repositories.
- `workflow` is for interacting with GitHub Actions.
- Make Sure to Save Your Token: Git tokens are typically shown once. Make sure to keep it in a safe place, as you won't be able to retrieve it again.
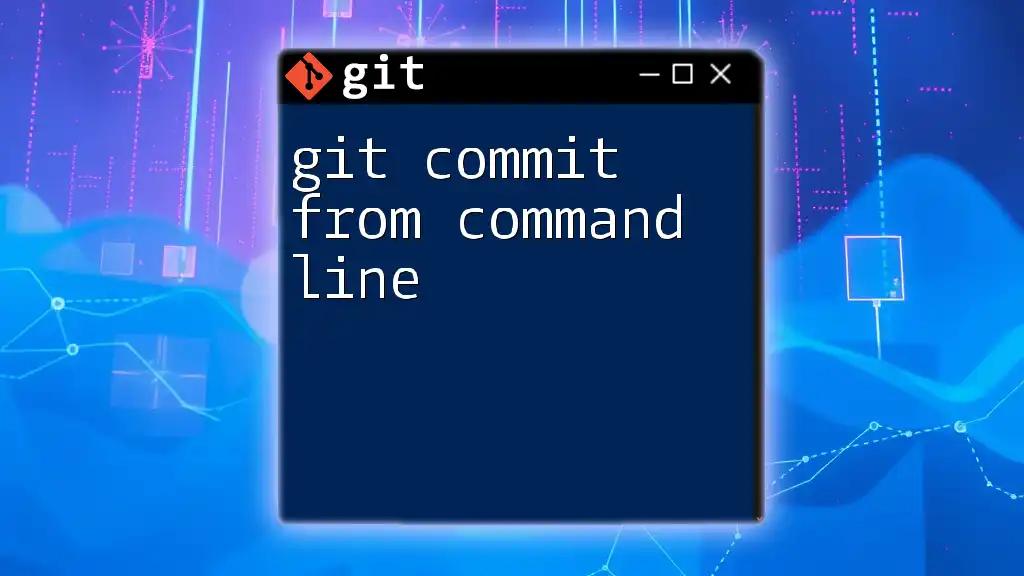
Configuring Git with a Token
The git config Command
The `git config` command is critical in managing your Git configuration settings. Through this command, you can set your username, email, and other important settings.
Common Use Cases include:
- Setting your username and email, which are used for commits.
- Defining how you want Git to handle credentials.
Setting Up Token Configuration Without Prompts
Avoiding prompts during authentication can streamline your workflow, especially for automated systems. This is particularly valuable in scripts and CI pipelines where user input is impractical.
Steps to Configure Git with a Token
Using the Command Line
To configure your Git client to use a token without prompting, you can follow these commands:
-
First, set up credential storage by running:
git config --global credential.helper store
This command tells Git to store your credentials in a plain text file on your system.
-
Next, you need to add your token to the credential storage. Use the following commands to set your username and email, and store the token:
git config --global user.name "Your Username" git config --global user.email "your_email@example.com" git config --global credential.helper store echo "https://<token>:x-oauth-basic@github.com" > ~/.git-credentials
In this command:
- Replace `<token>` with your actual Git token.
- This command sets up your Git client to authenticate using your token directly.
Verifying the Configuration
To ensure that your configuration is set up correctly, run:
git config --list
This command will display a list of the current configuration settings. Look for `user.name`, `user.email`, and any other credential settings you have applied.

Common Issues and Troubleshooting
Permission Denied Errors
When trying to push or pull, you might encounter permission denied errors. These often stem from the token's scope. For example, if your token does not have permission to access a private repository, you will get an error.
Solution Steps:
- Re-examine the scopes assigned to your token.
- Regenerate the token with the correct permissions and update your configuration as necessary.
Git Credentials Not Saved
Sometimes, you may find that Git does not save your credentials as expected. This can happen due to various reasons, such as:
- The credential helper being misconfigured.
- A potential typo in your `.git-credentials` file.
Fixing the Issue:
- Make sure that the credential helper is set correctly. Revisit the setup steps and ensure that the path to `.git-credentials` is accurate and accessible.
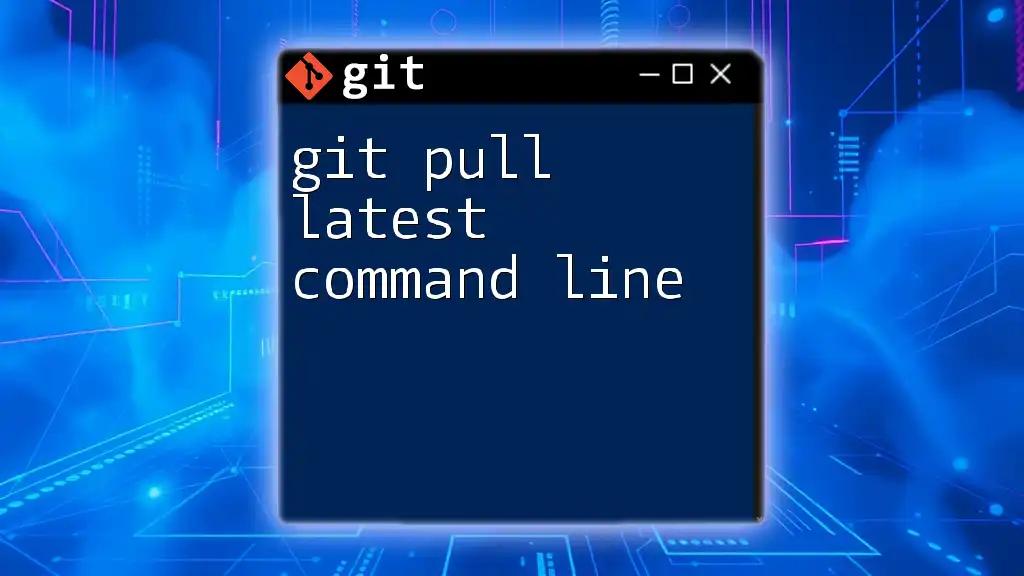
Additional Configuration Tips
Enhancing Security
When working with tokens, it is essential to maintain high-security standards. Always avoid hardcoding tokens directly into your scripts. Instead, consider using environment variables or secure secret management solutions.
Best Practices for Handling Tokens:
- Rotate your tokens regularly to minimize risks associated with token leakage.
- Store your tokens securely using password managers or encrypted files.
Automating with Scripts
Using shell scripts can significantly reduce the chances of errors in your configuration process. Below is an example of a simple script that automates token configuration:
#!/bin/bash
git config --global user.name "Your Username"
git config --global user.email "your_email@example.com"
git config --global credential.helper store
echo "https://<token>:x-oauth-basic@github.com" > ~/.git-credentials
This script can be easily run on a new workstation or during a setup process, ensuring that Git is configured consistently.

Conclusion
By configuring Git with a token through the command line without prompts, you streamline your development workflow, especially in automated environments. This guide has covered the essential aspects of generating and storing tokens, troubleshooting common issues, and following best practices for secure token management.
Remember that effective Git configuration not only improves your productivity but also enhances the security of your applications. As you explore more advanced Git functionalities, apply these principles to ensure a smooth and efficient coding experience.
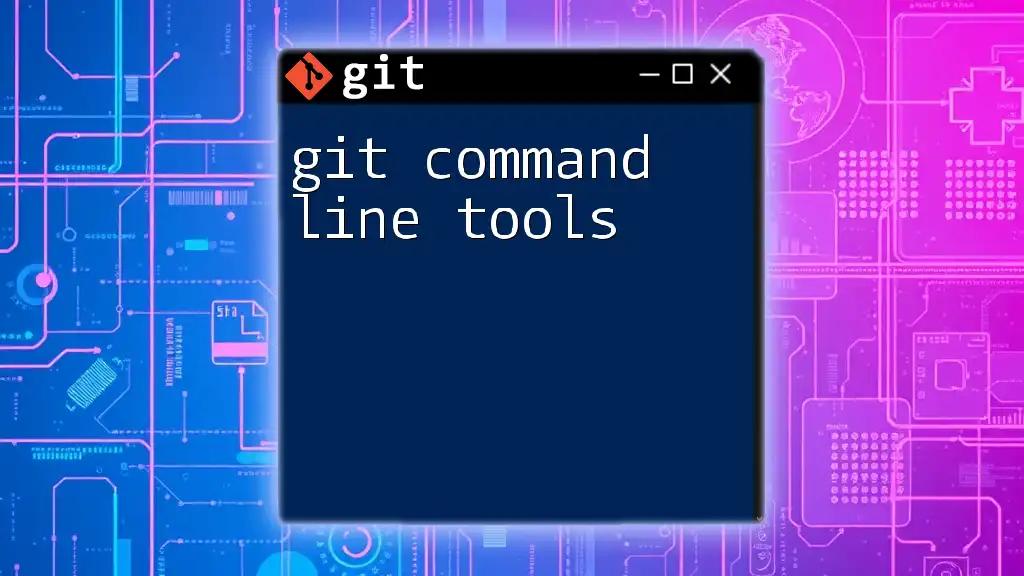
Additional Resources
For further information, consider checking out the official Git documentation, or explore tutorials from reputable sources to deepen your understanding of Git commands and best practices.