The `git pull` command fetches the latest changes from the remote repository and merges them into your current branch, ensuring your local code is up to date.
git pull origin main
Understanding `git pull`
What is `git pull`?
The `git pull` command is a crucial tool within Git, designed to update your local repository with changes from a remote repository. When executed, this command performs two main actions: it first fetches the latest changes (commits) from the specified remote repository and then merges them into your current branch. Understanding the distinction between these actions is key to effectively utilizing `git pull`.
Why Use `git pull`?
Using `git pull` ensures that your local copy reflects the most recent changes made by other collaborators in a project. This is particularly important in team environments, where multiple developers may be working on different features simultaneously. By regularly executing this command, you help prevent merge conflicts down the line and maintain a smooth workflow.
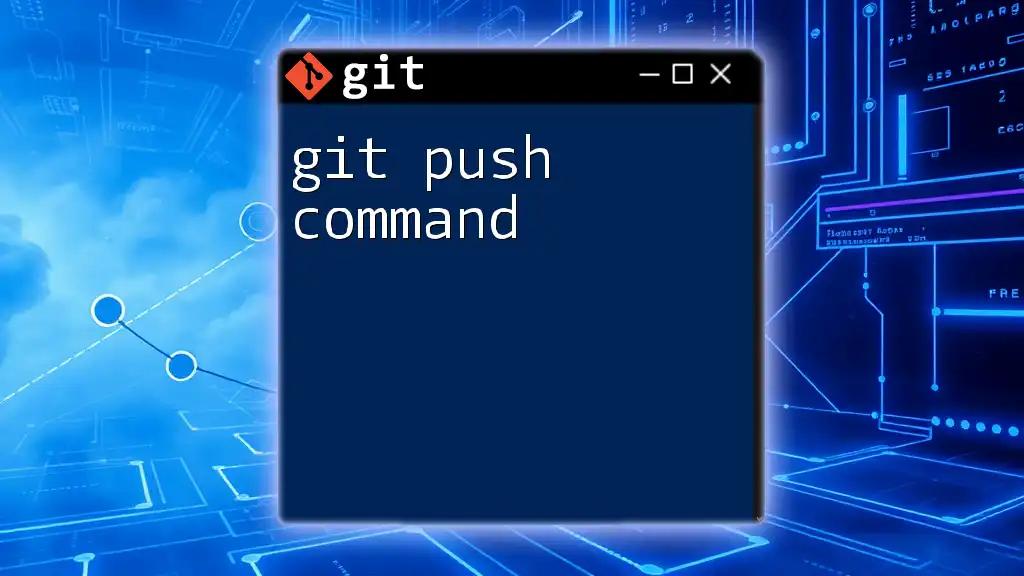
Basic Syntax and Structure
Command Structure
The general syntax for the command is as follows:
git pull [options] [<repository> [<refspec>...]]
Here, options allow for customization of the command's behavior, while `<repository>` and `<refspec>` specify the source from which to pull.
Key Components
- `<repository>`: This indicates the remote repository you want to pull from. If not specified, Git uses the default remote—typically named `origin`.
- `<refspec>`: This option allows you to specify a particular branch or tag to pull, rather than pulling all changes.
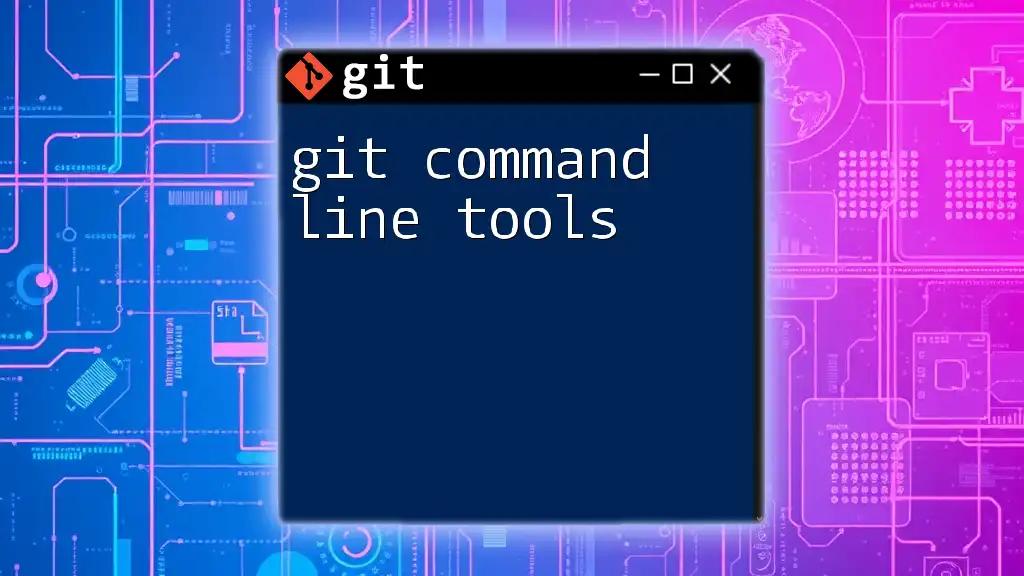
How to Use `git pull` Command
Pulling from the Default Remote
Basic Usage
To perform a standard update of your local repository, you can simply enter:
git pull
This command will fetch and merge changes from the default remote (usually `origin`) into the current branch you are working on. If your local branch is already tracking a remote branch, you will get the most recent commits automatically.
Pulling from a Specific Remote
Using Remotes
If you need to pull from a remote that is not the default, specify the remote name. For example:
git pull origin main
In this command, `origin` refers to the remote repository, and `main` indicates the branch from which you want to pull. Specifying a remote can be particularly useful when working with multiple shared repositories.
Pulling a Specific Branch
Branch Considerations
If you have to pull updates from a particular branch, you can do so with:
git pull origin feature-branch
Make sure you are on the branch that you want to merge changes into. This command pulls the latest changes from the `feature-branch` in the `origin` remote, merging them into your local branch.
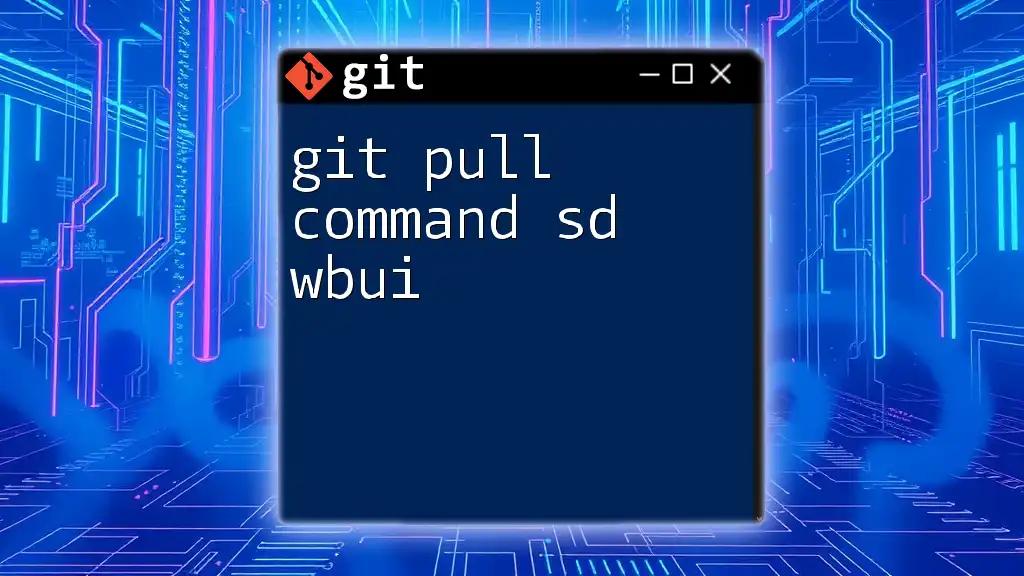
Handling Merge Conflicts
What are Merge Conflicts?
Merge conflicts occur when changes from the remote branch and your local branch alter the same line of code, or when one branch deletes a file that the other modifies. This situation requires manual intervention to resolve.
Resolving Merge Conflicts
To resolve conflicts, Git will halt the merging process and mark the affected files. You will see something like this in your code:
<<<<<<< HEAD
Current changes
=======
Incoming changes
>>>>>>> feature-branch
To resolve the conflict, you'll need to edit the affected files, deciding which changes you'd like to keep. After resolving conflicts, run the following commands to mark the conflicts as resolved and finalize the merge:
git add <file-with-conflict>
git commit
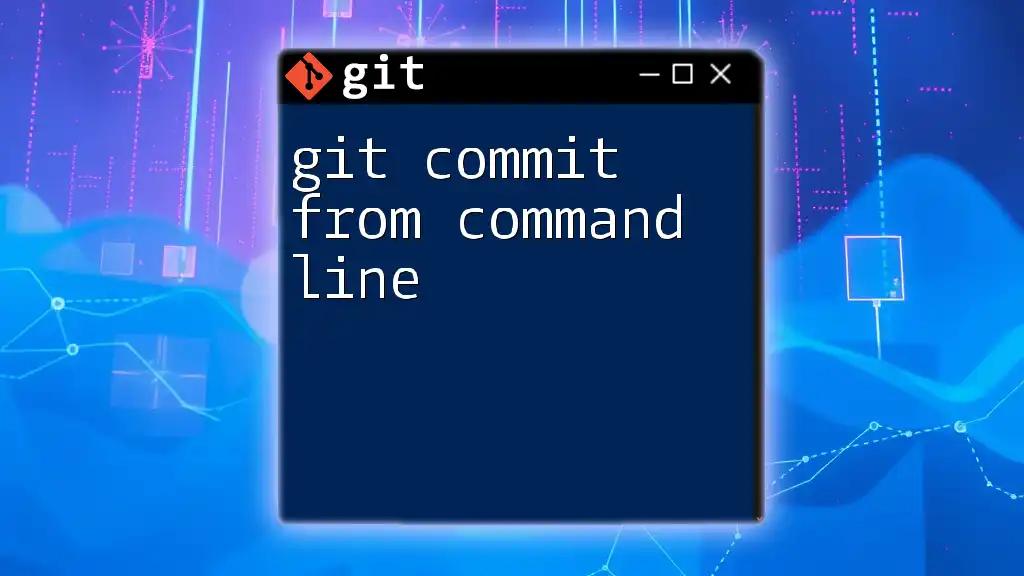
Advanced `git pull` Options
Commonly Used Options
--rebase
Using the `--rebase` option modifies how changes are applied. Instead of merging, it re-applies your local changes on top of the fetched changes:
git pull --rebase
This makes for a cleaner project history and can help avoid unnecessary merge commits. It is particularly beneficial when working on feature branches.
--ff-only
The `--ff-only` option enforces a fast-forward merge if possible. If there are conflicts that would prevent this, the command will fail rather than creating a merge commit:
git pull --ff-only
This option is excellent for keeping a linear history, but use it cautiously—only when you are sure that your local branch has no diverged commits.
Customizing Pull Behavior
You can customize the behavior of `git pull` via your Git configuration settings in `.gitconfig`. For instance, you might want to always use rebase when pulling changes. This can be configured with:
git config --global pull.rebase true
Such customizations can significantly streamline your workflow.
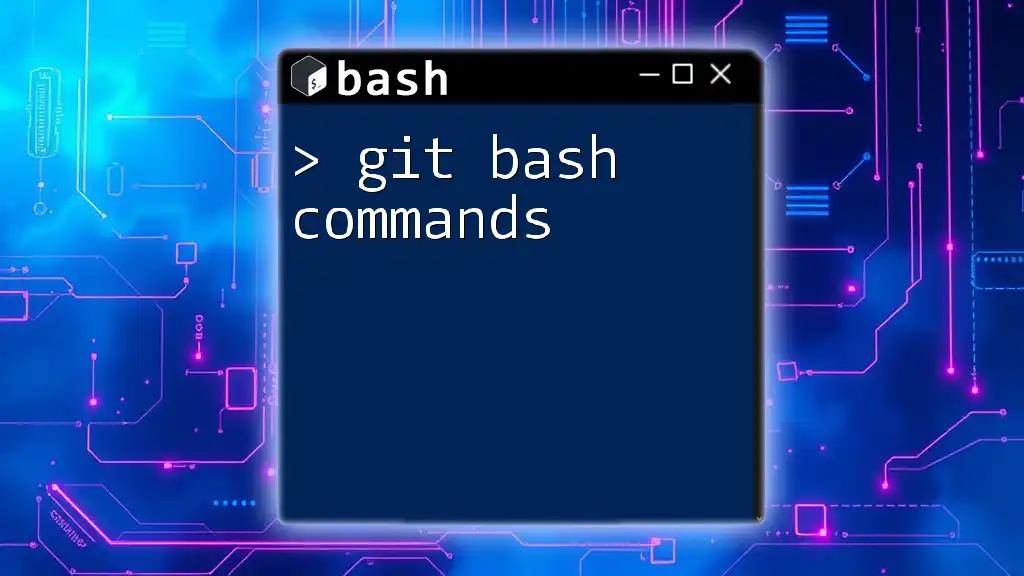
Practical Examples of `git pull`
Real-world Scenarios
Let's say you are collaborating on a project with multiple developers. Your team has been actively working on various features. Regularly running `git pull` will help you integrate their changes into your local work and ensure you’re building on top of the most current base.
Example Workflows
Consider this step-by-step example of a typical `git pull` workflow:
- Ensure you are on the correct branch using:
git checkout main
- Execute the pull command:
git pull
- Verify that you have the latest changes using:
git log
- If any merge conflicts occur, resolve them as discussed in the previous section and commit your changes.
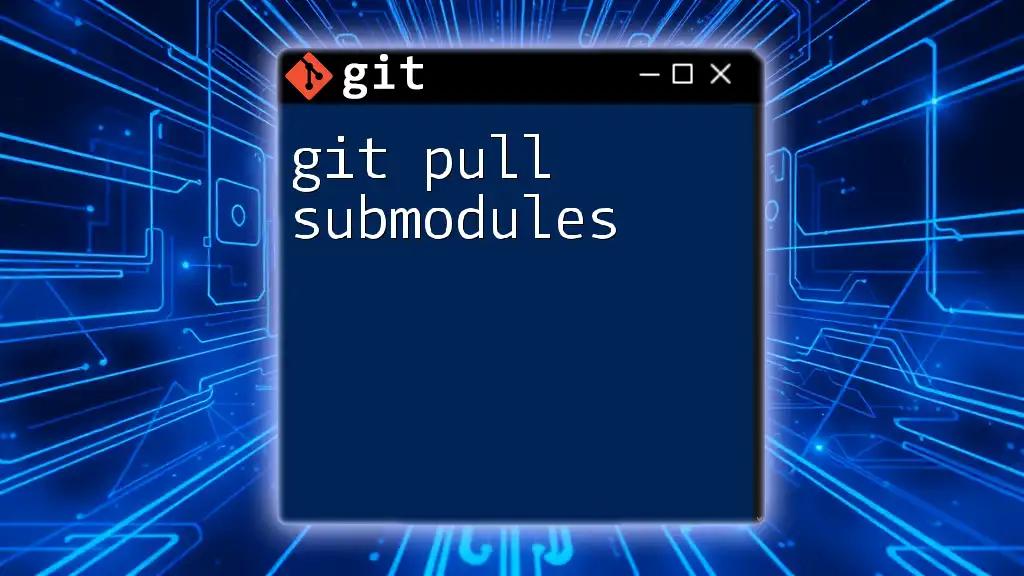
Troubleshooting `git pull`
Common Issues
While using `git pull`, you may encounter several issues:
- Network Issues: Sometimes network connectivity may fail, preventing Git from reaching the remote repository.
- Remote Repository Not Found: If Git fails to find the specified remote, verify the repository name and ensure it exists.
- Local Changes Preventing Pull: If you have uncommitted changes, Git may prevent a pull. Use `git stash` to temporarily set aside your work.
Solutions and Tips
To check your network connectivity, you might use:
ping github.com
For resolving local changes, consider stashing your changes:
git stash
After pulling the latest changes, you can reapply your stashed work with:
git stash pop
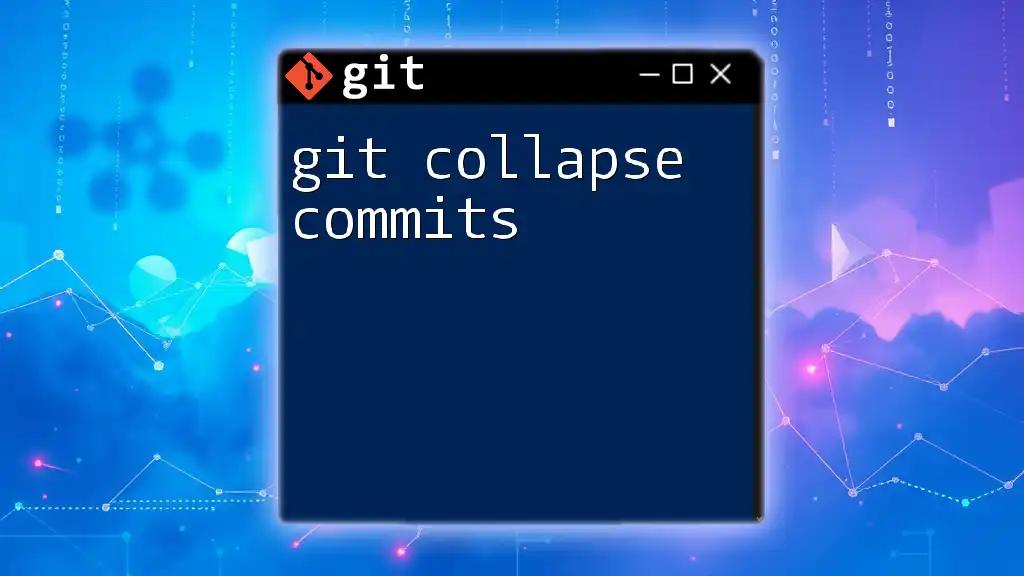
Conclusion
Using the `git pull` command is vital for maintaining an up-to-date local repository in collaborative environments. By incorporating this command into your routine, you can mitigate merge conflicts and keep your development workflow efficient. Practice regularly to master the nuances of the `git pull latest command line`, and you'll find it indispensable in your Git toolbox.
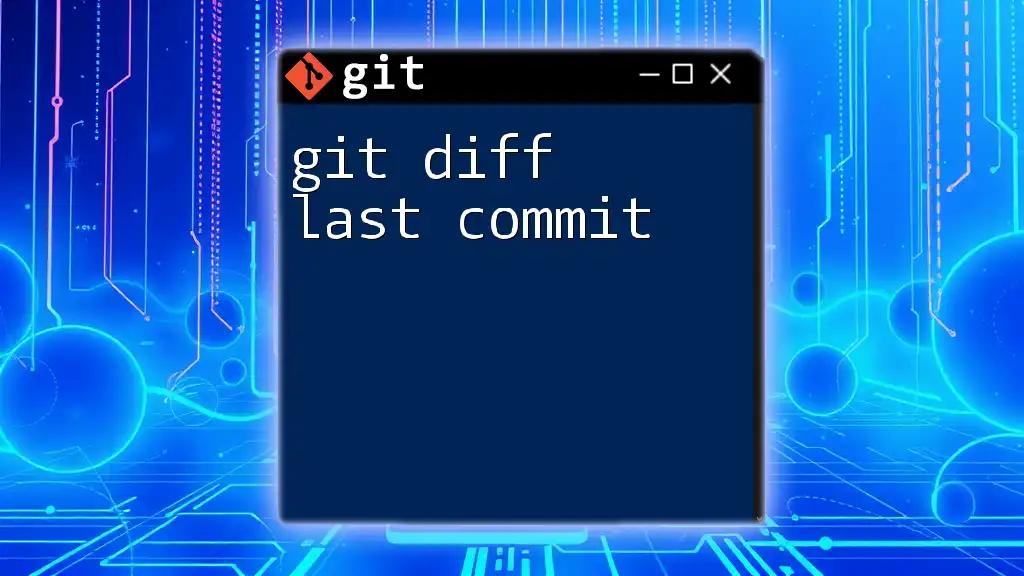
Additional Resources
For further reading and a deeper dive into Git operations, be sure to check out the official Git documentation and consider enrolling in comprehensive Git tutorials that suit your learning style. Feel free to reach out for assistance and join communities dedicated to mastering Git for effective version control.