The command `git diff HEAD~1` shows the changes made in the last commit compared to the current working directory.
git diff HEAD~1
What is `git diff`?
The `git diff` command is essential for comparing changes in your project. This command lets you see what has changed in the files of your repository since the last commit, as well as view differences between various commits. Understanding what git diff does enables developers and teams to review their work efficiently.
The Purpose of Comparing Changes
Using `git diff` helps you visualize modifications made to your code, facilitating better decision-making about what to commit or discard. With clear, structured output, it’s easier to pull out the impact of your changes and understand the evolution of your codebase.
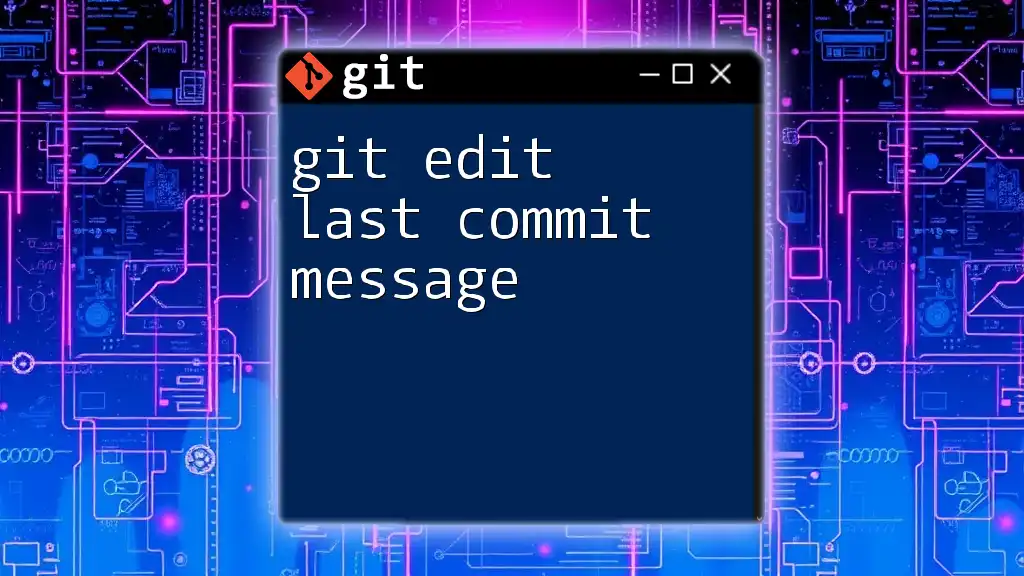
Understanding Commits in Git
What is a Commit?
A commit in Git represents a snapshot of your project at a certain point in time. Each commit contains a unique identifier (hash), information about the author, a commit message describing changes made, and a timestamp.
How to View Your Commit History
To grasp the evolution of your project and see your commits, use the `git log` command. This command displays your commit history, helping you understand the context behind changes.
git log
This simple command provides a chronological list of all commits, detailing the author, date, and commit message.
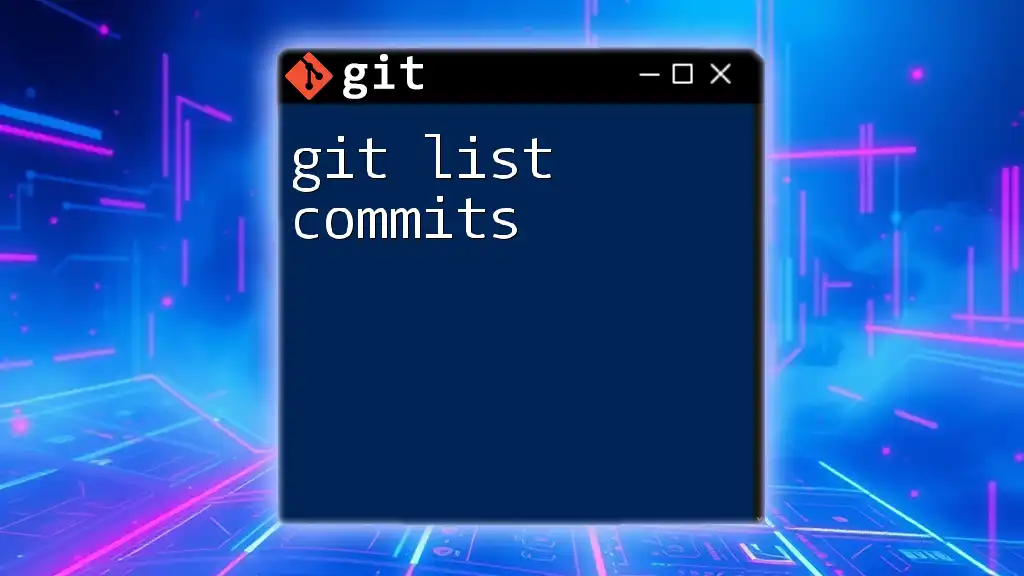
The Last Commit in Git
Identifying the Last Commit
In Git, HEAD is a reference to the last commit in the current branch. This makes it easy to access the most recent snapshot of your project.
Basic Command for Viewing the Last Commit
To view all the details related to the last commit, you can use the following command:
git show HEAD
This will display the changes made in the last commit, including the commit message, author, date, and the associated file changes.
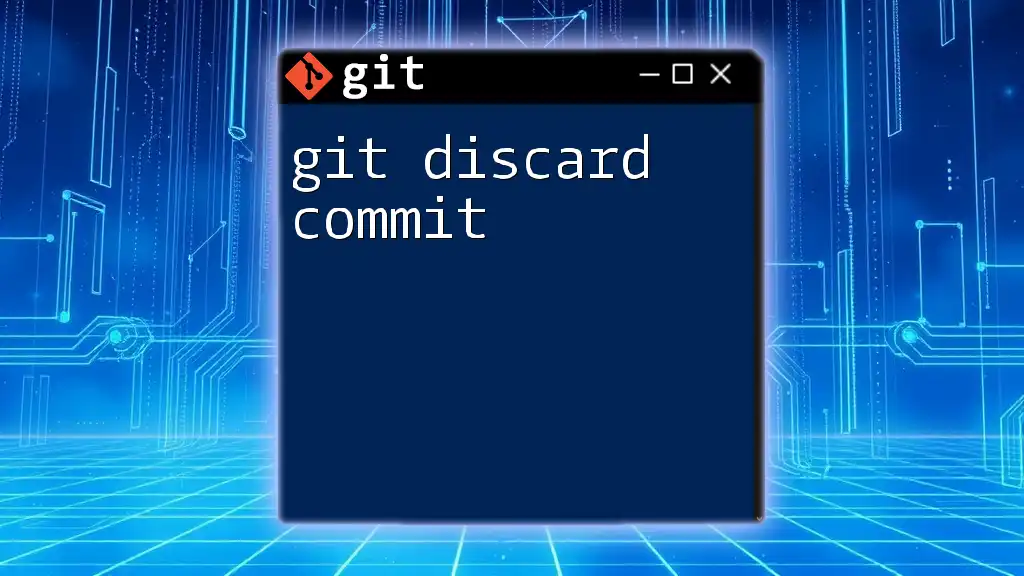
Using `git diff` to Compare Changes
Basic Syntax for Diffing with Last Commit
To compare changes made since the last commit, you can use the following command:
git diff HEAD^ HEAD
In this command, HEAD^ refers to the previous commit, while HEAD points to the most recent one. This allows you to visualize the changes between those two points.
How to Diff Against the Last Commit
If you only wish to see the changes that are in your working directory but not yet staged for commit (i.e., the changes since the last commit), you can simply run:
git diff HEAD
This command provides an overview of the edits made after the last commit, helping you verify your adjustments before committing them.
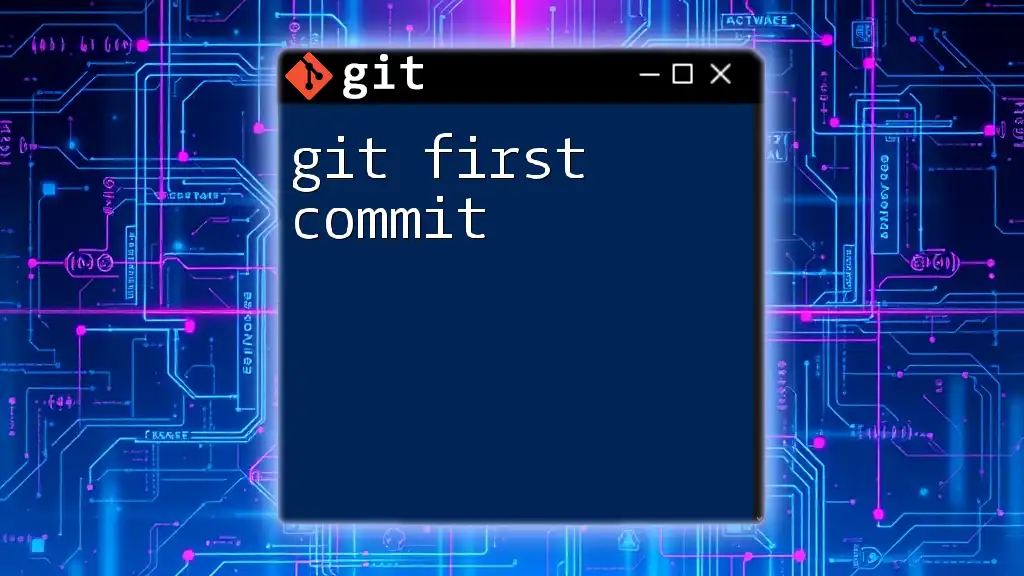
Viewing Specific Files with `git diff`
Diffing Specific Files
Sometimes you might want to check changes made specifically to a particular file. Using `git diff` for a specific file can be done with:
git diff HEAD -- <filename>
Replace `<filename>` with the name of the file you are interested in. This command is particularly useful when you want to focus on changes in a specific part of your code.
Use Cases
- Checking modifications on a critical file before merging.
- Preventing errors by ensuring only the intended changes are staged or committed.
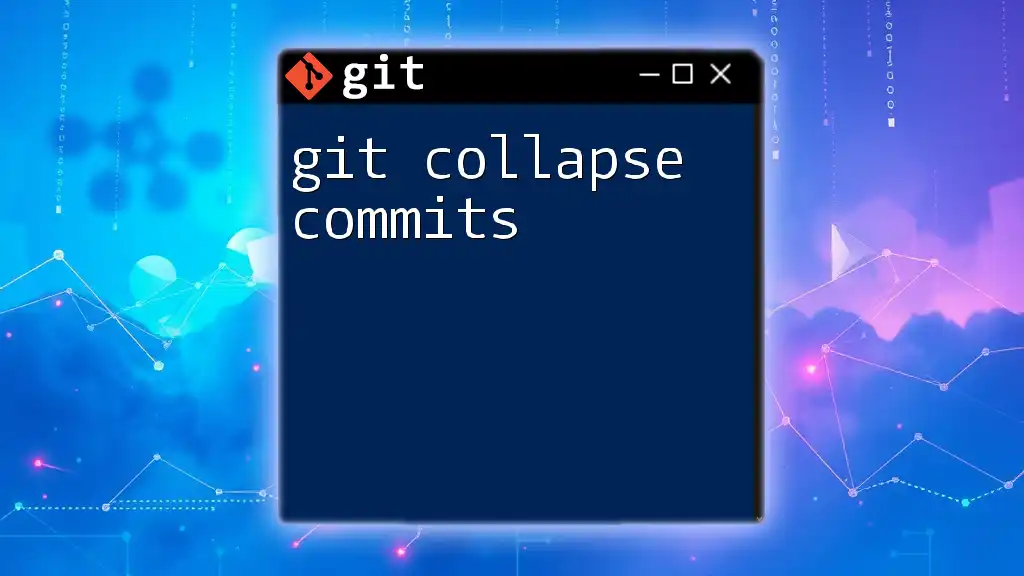
Advanced Options
Using Diff Flags for More Information
To enhance your `git diff` output, there are several useful flags you can include:
- --color: Helps you visualize changes with colors. This is often enabled by default but can be enforced.
- --stat: Provides a summary of changes, showing how many lines were added or deleted.
- --cached: Shows changes staged for the next commit.
An example command with the --stat flag could look like this:
git diff --stat HEAD
This displays the statistics of what has changed since the last commit, giving you a quick overview without diving into line-by-line comparisons.
Comparing Changes Between Two Specific Commits
You can also compare changes between any two specific commits. This can be done by using their commit hashes:
git diff <commit-hash1> <commit-hash2>
This command provides a detailed comparison, enabling you to understand the evolution of features or bug fixes between distinct points in your project’s history.
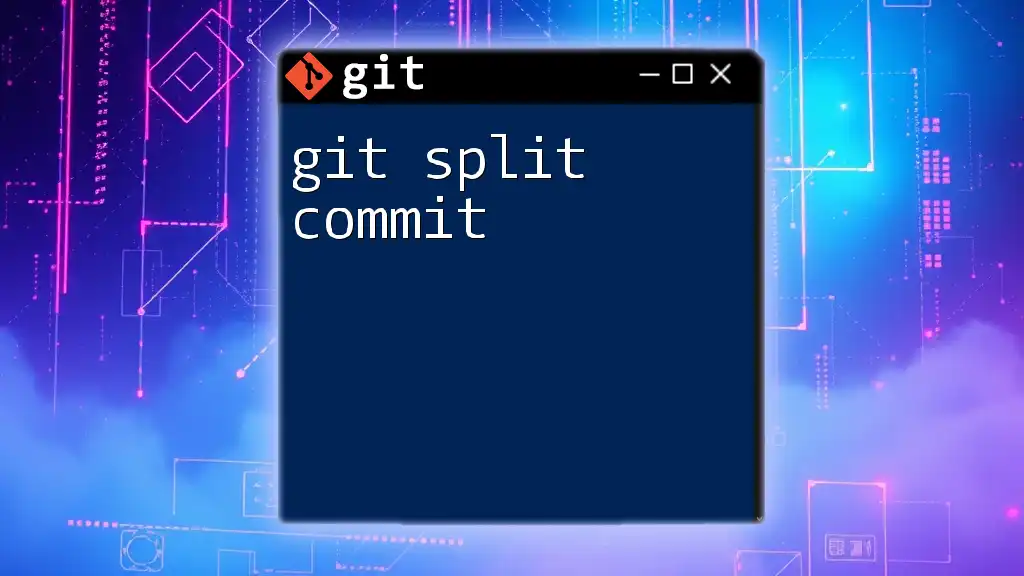
Common Issues and Troubleshooting
Resolving Confusion with Diff Outputs
When reviewing the output from `git diff`, it's important to understand how modifications are presented:
- Lines added will often be marked with a + prefix.
- Lines deleted will usually have a - prefix.
Understanding these indicators is vital for comprehending what changes are being introduced.
What to do if Nothing Shows Up
If you run `git diff` and it returns no output, here are some potential reasons and solutions:
- No changes since the last commit: Ensure there are actual changes made.
- Using the wrong HEAD reference: Double-check you are referring to the correct commits or HEAD.
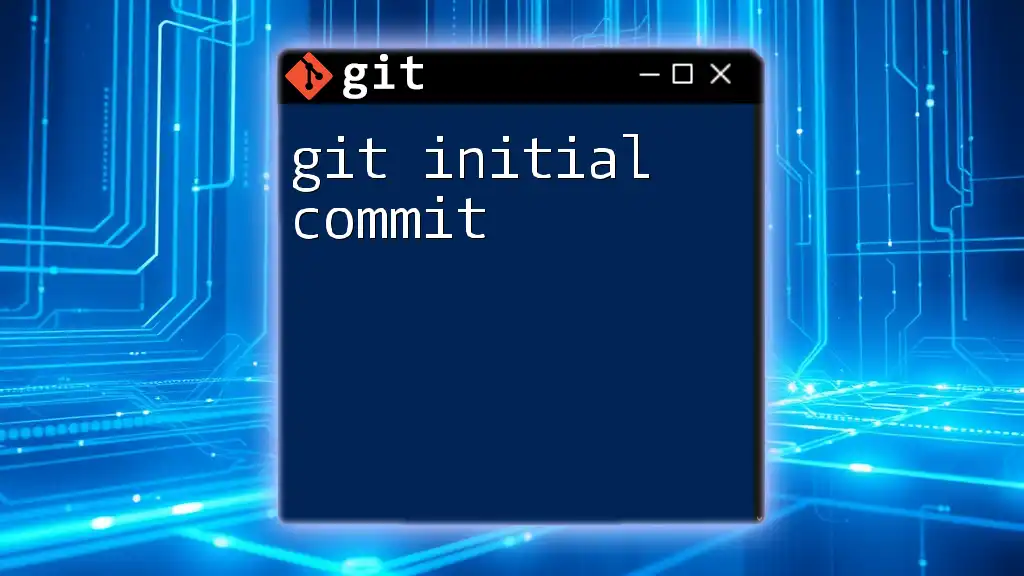
Conclusion
To summarize, mastering the `git diff last commit` command is incredibly beneficial for any developer looking to maintain control over their code changes. Understanding how to compare your current modifications to the last commit allows you to make informed decisions regarding what should be included in your next commit. Practice these commands to make them second nature, and take the next step in optimizing your version control workflows.
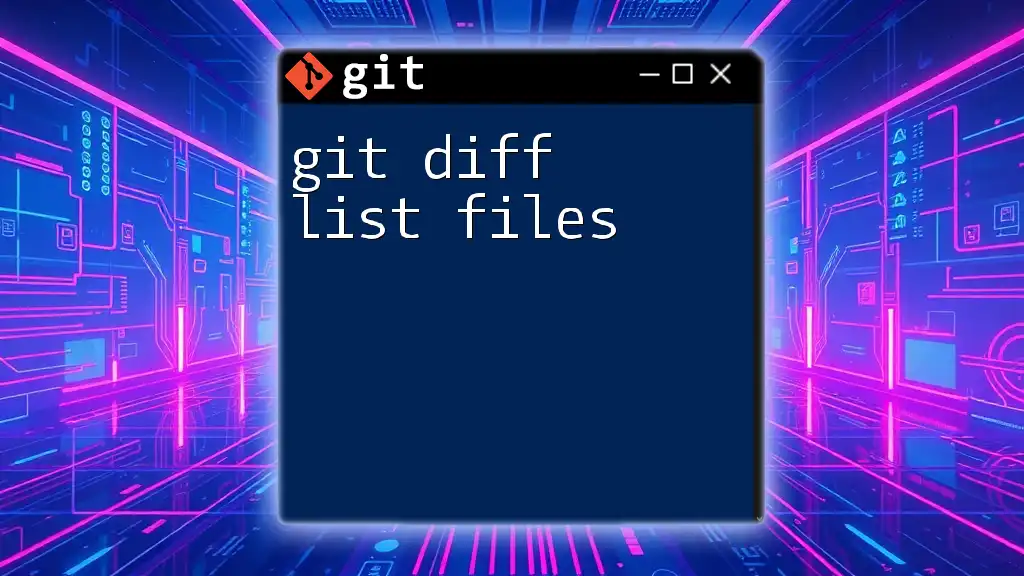
References
For further reading and deeper insights, consider checking out the official Git documentation and additional tutorials focused on Git commands and features.
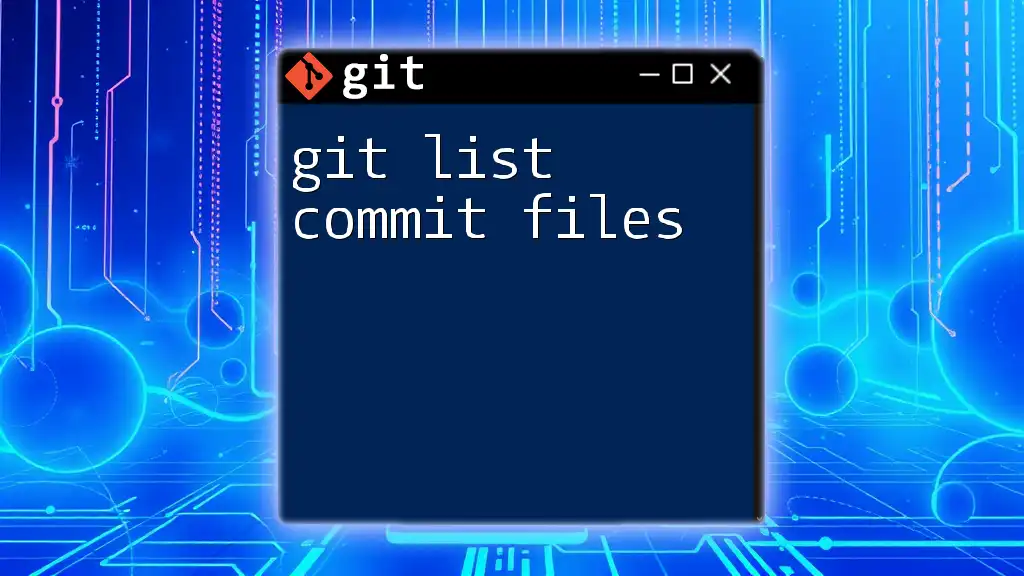
Additional Resources
Explore video tutorials or participate in interactive Git playgrounds to gain hands-on experience and enhance your learning process.