The `git diff` command allows you to compare changes in files between commits, working directories, and branches, and to list the specific files that have been modified.
git diff --name-only
What is `git diff`?
`git diff` is a powerful command utilized in Git that allows users to visualize changes between various file versions in their repositories. Its primary purpose is to facilitate a comparison of code, making it easier for developers to track modifications and identify discrepancies. This command proves invaluable when debugging, collaborating with team members, and reviewing code before commits or merges.
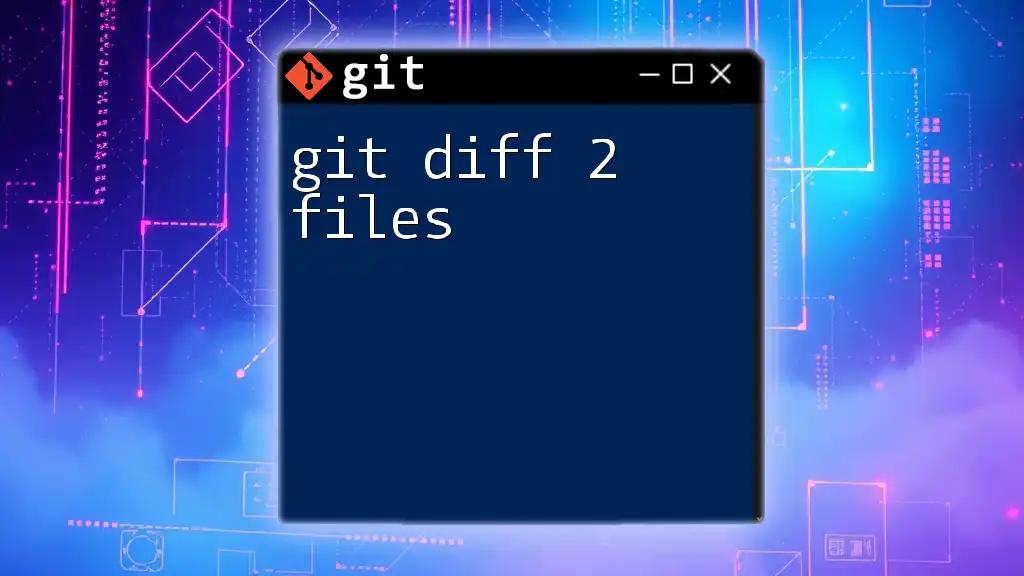
Basic Syntax of `git diff`
Understanding the syntax of `git diff` is fundamental for utilizing it effectively. The command follows this basic structure:
git diff [options] [<commit>] [--] [<path>...]
- options: Flags that modify the command's behavior.
- <commit>: Specifies commmits to compare one with another.
- <path>: Indicates the file or directory to limit the comparison scope.
This foundational knowledge enables developers to tailor their commands to meet specific needs.
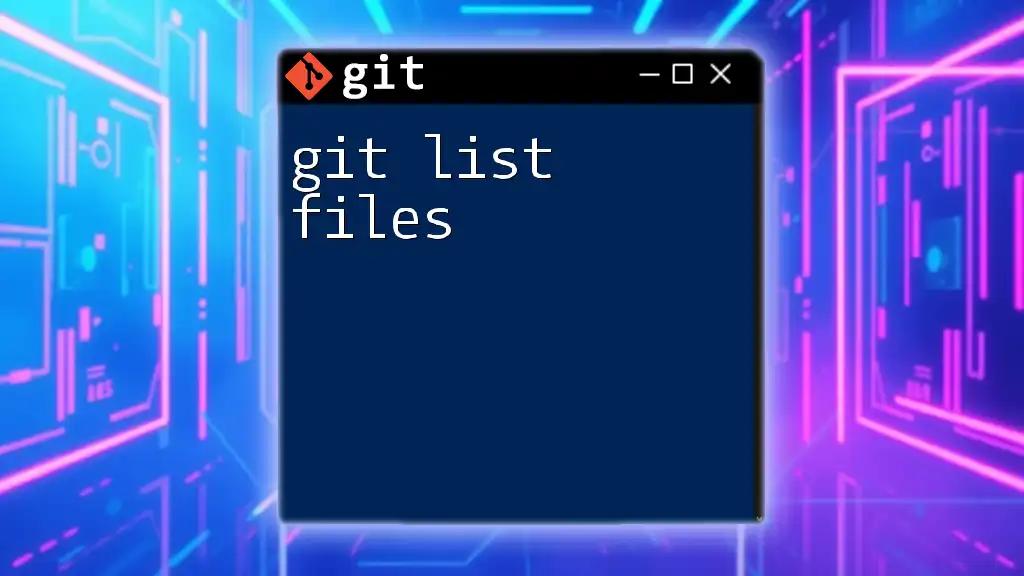
Understanding File Differences
Git tracks file modifications by maintaining a snapshot of each file version in its history. When you run `git diff`, it evaluates the current state of files against their last committed version, highlighting changes with precision.
Types of differences:
- Added changes: Lines of code that have been added to the file.
- Removed changes: Lines that have been deleted from the file.
- Modified lines: Existing lines that have been altered in any way.
This detailed comparison aids developers in identifying exactly what has changed between different versions of a file.
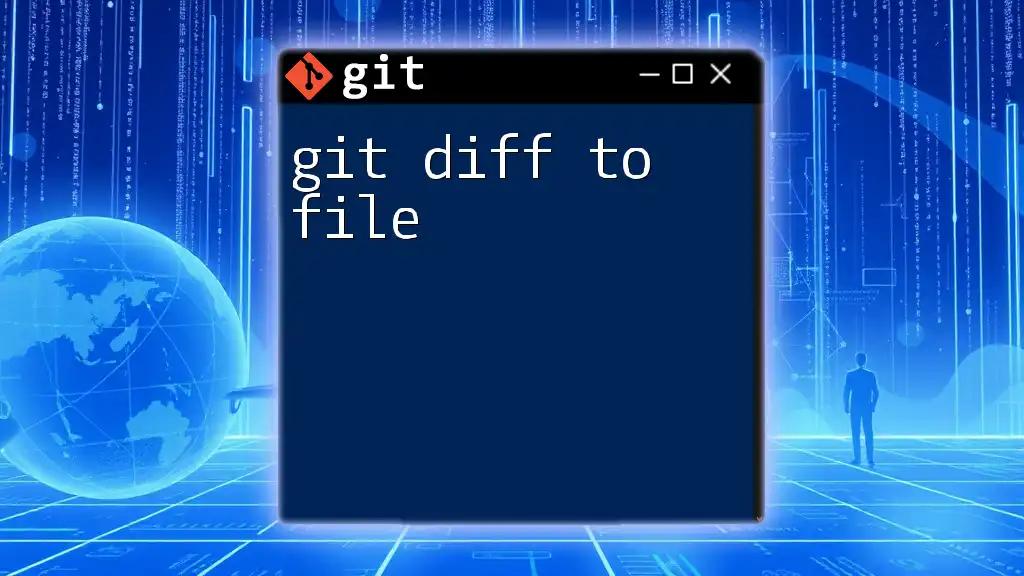
Listing Files Changed with `git diff`
To list files that have changed between commits, you can use the command:
git diff --name-only [commit1] [commit2]
Example Usage:
git diff --name-only HEAD~1 HEAD
In this example, the command compares the most recent commit to its previous version, listing only the names of the files that have changed. The use of the `--name-only` option allows you to view changed files without the clutter of actual code differences, streamlining your review process.
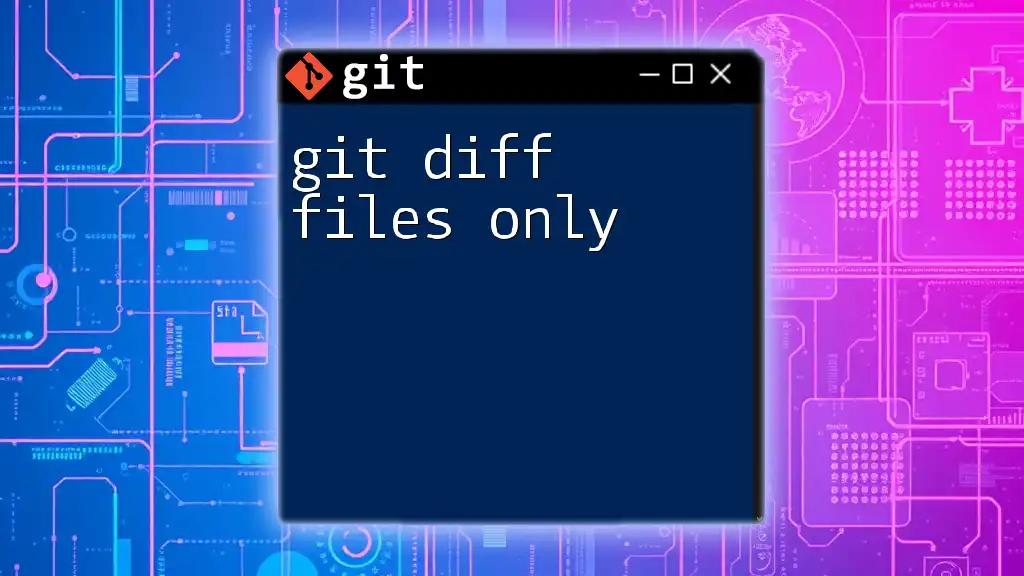
Using `git diff` for Unstaged Changes
When you want to examine changes that have not been staged for commit, you can run:
git diff --name-only
This command efficiently shows you all the files that have been modified but not yet added to the staging area. Understanding your unstaged changes is crucial, as it enables you to decide what modifications to include in your next commit, promoting better version control practices.
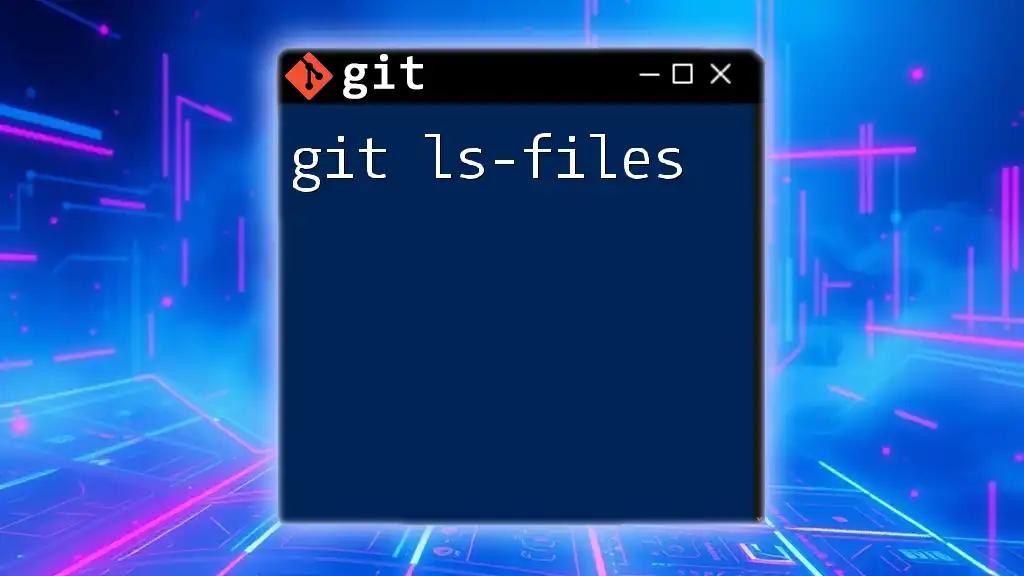
Comparing Specific Commits
You can also compare files between two specific commits using:
git diff [commit1] [commit2] -- [file]
Example:
git diff a1b2c3d e4f5g6h -- myfile.txt
This command allows you to isolate changes within `myfile.txt` between the two specified commits, making it easier to review specific file alterations in the context of the overall project history. This precise comparison is particularly useful for debugging or understanding the evolution of a file over time.
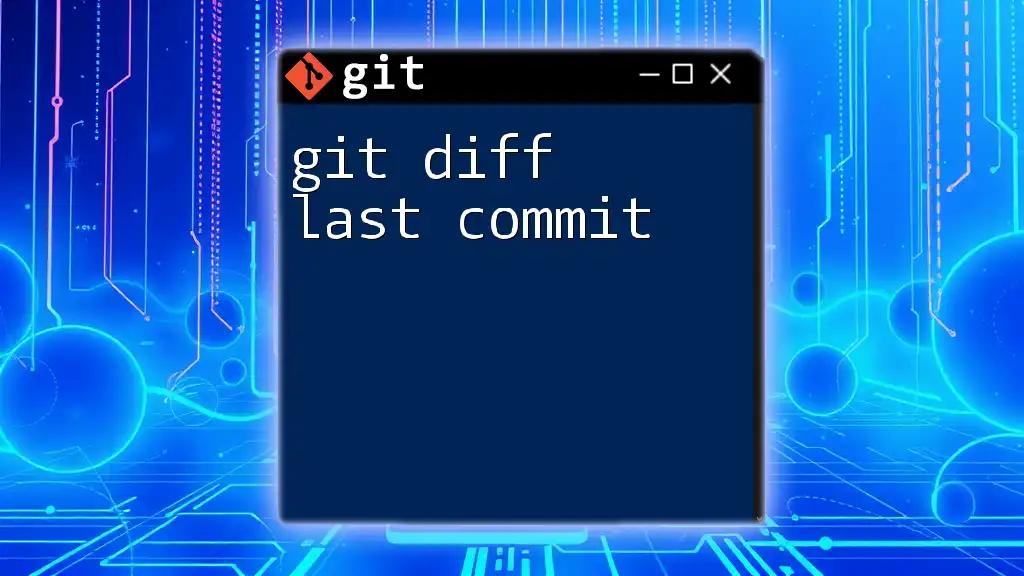
Using Diff with Other Options
Git provides various flags to enhance the capabilities of `git diff`. Some notable options include:
- `--cached`: This option displays differences between the staging area and the last commit, focusing on files that have been staged for the next commit.
- `--stat`: Use this flag to generate a summary of the changes, including how many lines were added or removed.
Example Command:
git diff --cached --name-only
This command will give you a list of files that you have staged for committing, empowering you to confirm the changes you've ready before finalizing your commit.
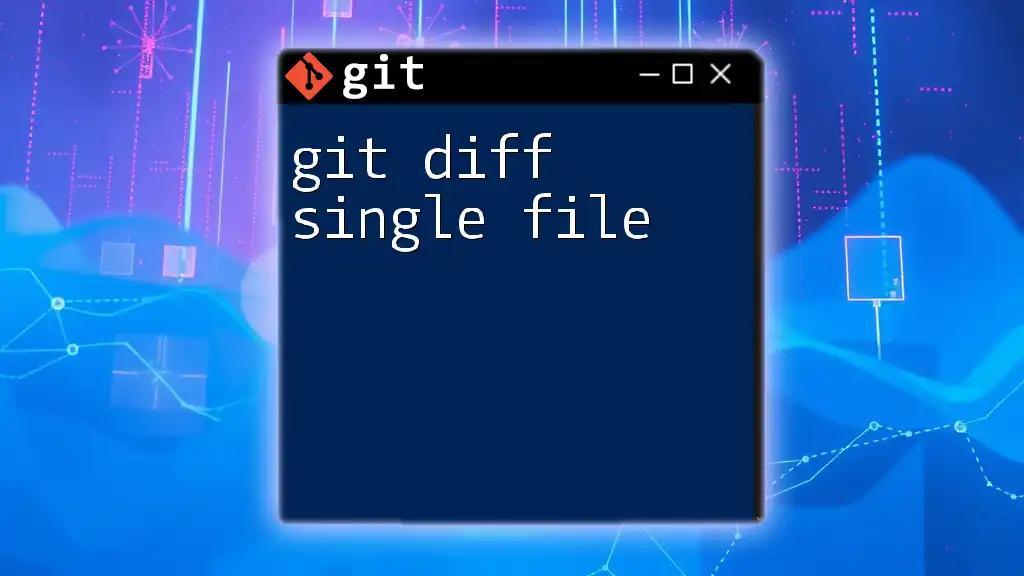
Common Use Cases for `git diff`
Understanding how and when to use `git diff` will significantly enhance your version control workflow. Here are a few real-world scenarios:
- Code Reviews: Before asking teammates to review your code, use `git diff` to identify changes quickly.
- Debugging: Use `git diff` to pinpoint where a bug might have been introduced by inspecting modified files.
- Collaboration: When merging branches, inspecting differences helps prevent conflicts and ensures necessary changes are integrated smoothly.

Troubleshooting `git diff`
While `git diff` is a powerful tool, users may occasionally encounter issues. Common challenges include:
-
No output: This occurs when there are no differences between specified commits or the working directory is clean. Ensure that you are in the correct branch and that changes exist to compare.
-
Confusing output: Sometimes, the output may seem overwhelming. Using options like `--stat` or focusing on `--name-only` can help simplify the information for better clarity.
When addressing any challenge, understanding the context will guide you toward effective solutions.
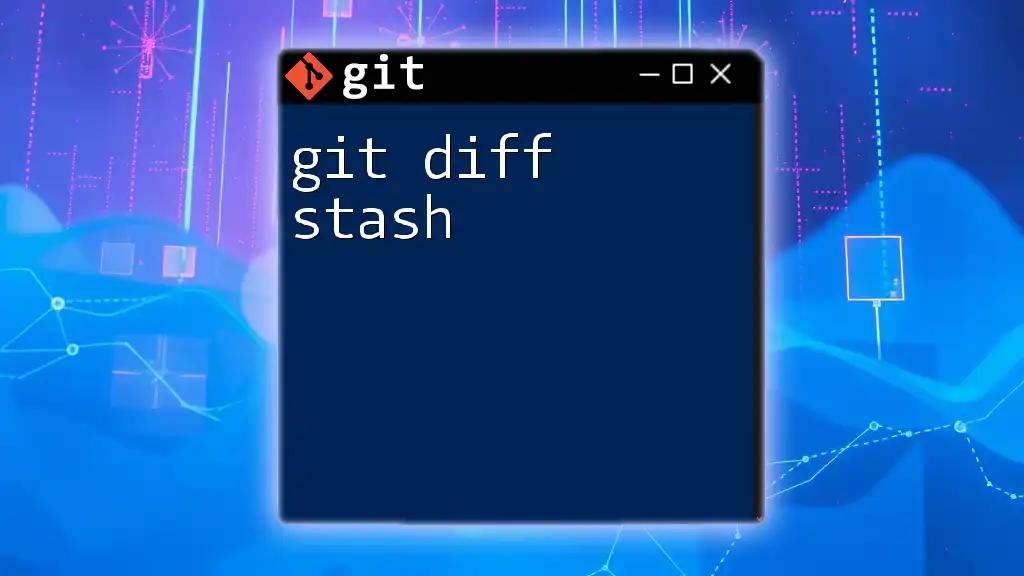
Conclusion
Utilizing `git diff` to manage file changes is essential for maintaining clean, effective code in any project. Mastering this command opens up new opportunities for collaboration, efficient debugging, and overall improved version control practices.
By regularly practicing these commands, you will enhance your Git skills and gain confidence in navigating your repository.
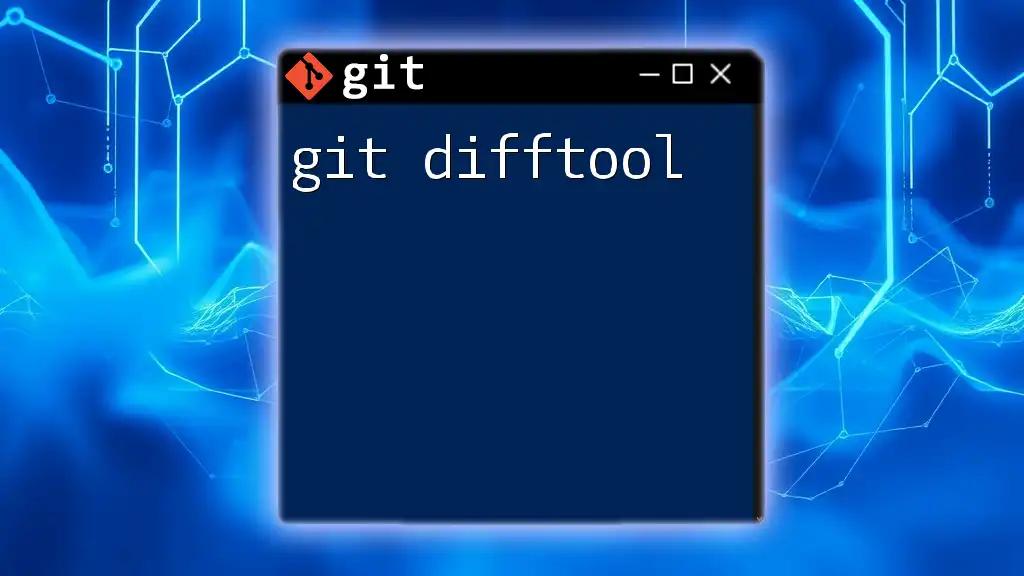
Call to Action
Explore more about Git and elevate your skills! Consider signing up for our classes and tutorials to gain deeper insights into powerful Git commands and best practices tailored to enhance your coding experience.