The `git diff` command allows you to compare the changes between two files in a Git repository, highlighting the differences in their content.
Here's how to use it:
git diff <file1> <file2>
What is `git diff`?
`git diff` is a fundamental command in Git that allows developers to compare changes between different versions of files, helping to visualize what has changed. This command is essential in collaborative environments where multiple contributors might be working on the same codebase, as it provides a clear view of modifications that can inform code reviews and merges.
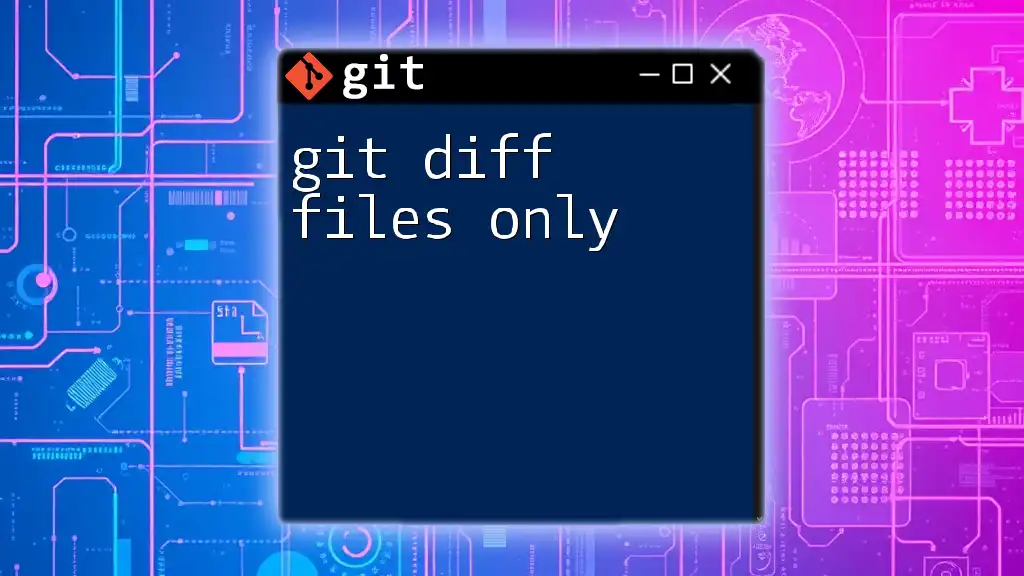
Understanding the Syntax of `git diff`
To effectively use `git diff`, it's crucial to grasp its syntax. The basic structure looks like this:
git diff <options> <commit1> <commit2> -- <file>
Here’s a breakdown of the components:
- options: Various flags that modify the behavior of the diff.
- commit1 and commit2: These represent the two points of reference you want to compare. They could be branches, tags, or specific commits.
- <file>: The specific file you want to examine.
This flexibility allows you to tailor your commands to your needs.
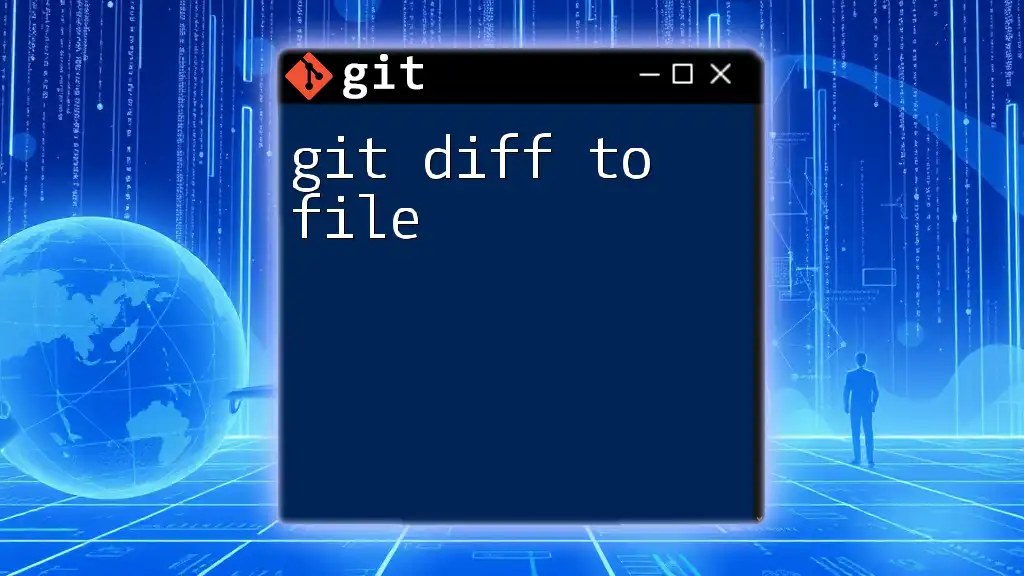
Comparing Two Files with `git diff`
To compare two specific files within the repository, you can use the command directly referencing both files. The syntax is straightforward:
git diff file1.txt file2.txt
In this command, `git diff` will show you the differences between `file1.txt` and `file2.txt`, highlighting what has been added or removed. This is particularly useful when you want to review changes before making a commit or to assess how two versions of a file differ from each other.
Using `git diff` with Version History
In addition to comparing current files, `git diff` can also examine differences across various commits. For example:
git diff HEAD~1 HEAD -- file.txt
In this case:
- HEAD refers to the latest commit on your current branch.
- HEAD~1 designates the commit just before the latest one.
The command compares `file.txt` across these two commits, which is helpful for reviewing changes made recently and understanding the evolution of a file's content.
Common Use Cases for Comparing Files
Understanding the practical applications of `git diff` can enhance a developer's workflow significantly:
- Review changes before committing: By running `git diff`, you can ensure that you only include intended changes in your commit.
- Understanding updates between branches: Use `git diff` to see the differences between files in different branches, which is vital for conflict resolution.
- Identifying conflicts during merges: When merging branches, `git diff` can pinpoint the changes that need careful review to avoid issues.
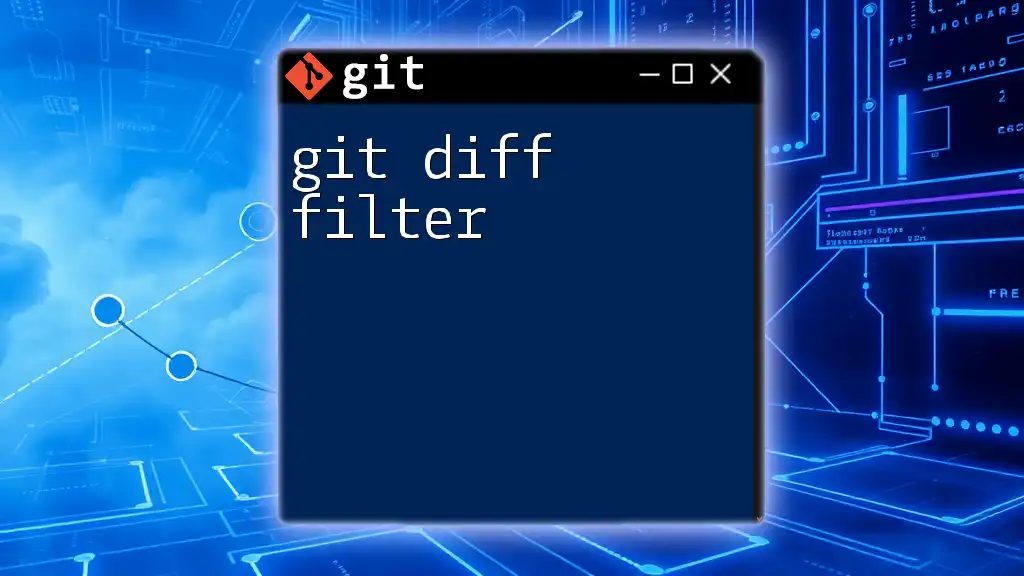
Output of `git diff`
The output of `git diff` is presented in a format that indicates the changes made between the two files or commits being compared.
- Added lines are typically shown in green or preceded by a `+`.
- Removed lines might appear in red or be marked with a `-`.
This visual representation helps developers quickly grasp what has changed at a glance.
Reading the Diff Output
Understanding the output is vital to using `git diff` effectively. The output will typically show the file name, followed by line-by-line differences. Here’s how to interpret the elements:
- Each change is prefixed by a `+` for additions and a `-` for deletions.
- Context lines, which are unaffected parts of the file, are generally shown without symbols.
Reading through the output gives you a comprehensive view of what modifications were made.
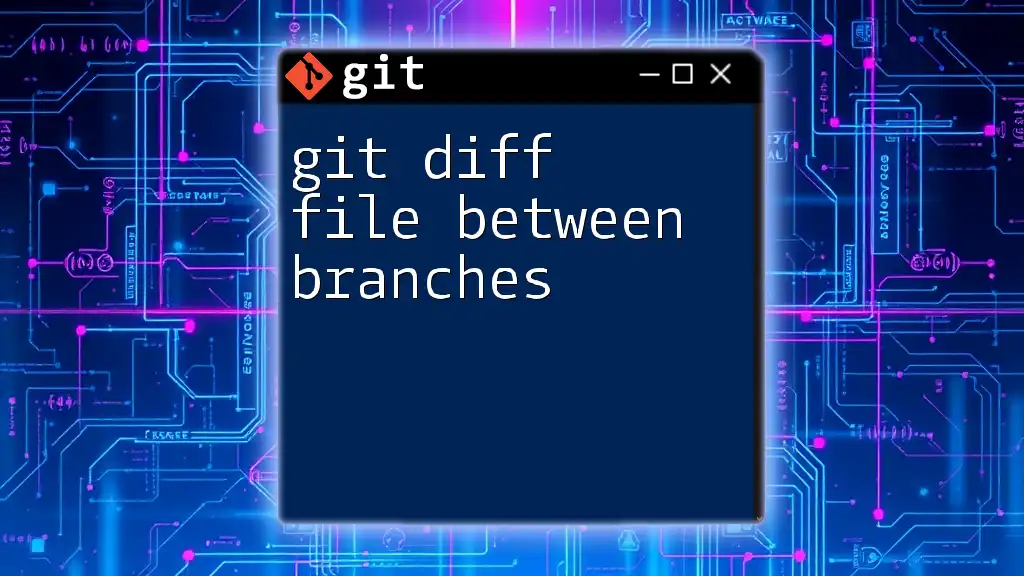
Additional Options with `git diff`
Comparing Ignoring Whitespace
In some cases, whitespace discrepancies may clutter the output. You can choose to ignore these by using the `-w` option:
git diff -w file1.txt file2.txt
This command will compare the two files while disregarding all whitespace changes, making it easier to focus on substantive edits.
Checking Differences in Staged Files
To review changes that have been staged for a commit, you can use:
git diff --cached file.txt
This command compares the staged version of `file.txt` with the latest commit, ensuring you are aware of what changes are ready to be committed.
Customizing Output
Customization is critical for enhancing readability. Various flags can alter how the output is displayed:
- `--color`: Forces colored output, which can be easier on the eyes.
- `--word-diff`: Shows word-level differences instead of line-level, making it easier to spot small edits.
For example:
git diff --word-diff file1.txt file2.txt
This allows for a more granular view of the changes, highlighting each word alteration.
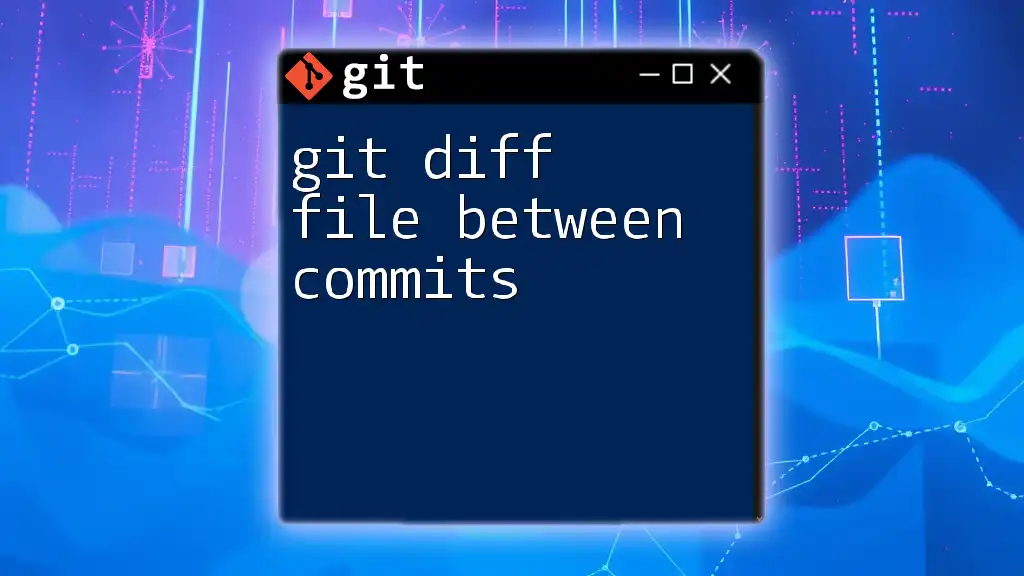
Practical Examples of `git diff`
Consider a typical development process. A developer is working on a new feature and needs to review changes made by a teammate. By executing `git diff`, they can immediately see how their code differs from their colleague's work.
Example Scenario
Let’s say you are collaborating on a project. Your colleague just submitted a branch with several updates. Before merging it into your branch, you might run:
git diff your_branch..colleagues_branch
By performing this comparison, you ensure that you're fully aware of the changes introduced and can make informed decisions about the merge process.
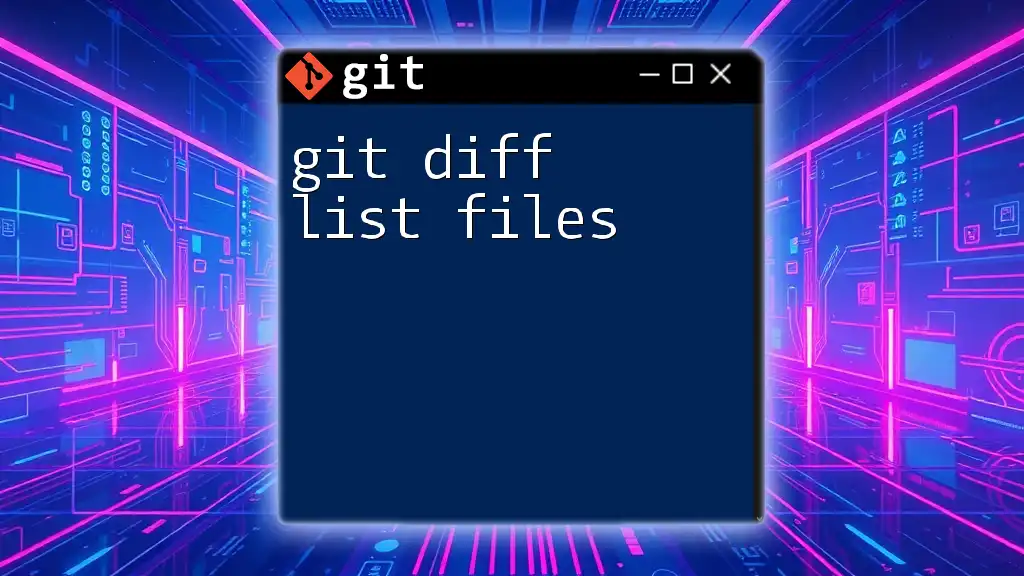
Common Pitfalls and Troubleshooting
While `git diff` is a powerful tool, it can present challenges:
- Confusion about commit references: Ensure that you are clear on which commits or branches you are referencing; using precise terms helps avoid mistakes.
- Overlooking staged changes: Remember that unless you specify `--cached`, `git diff` will only show unstaged changes.
Tip: Regularly practice running `git diff` to become comfortable interpreting its output and understanding its syntax.
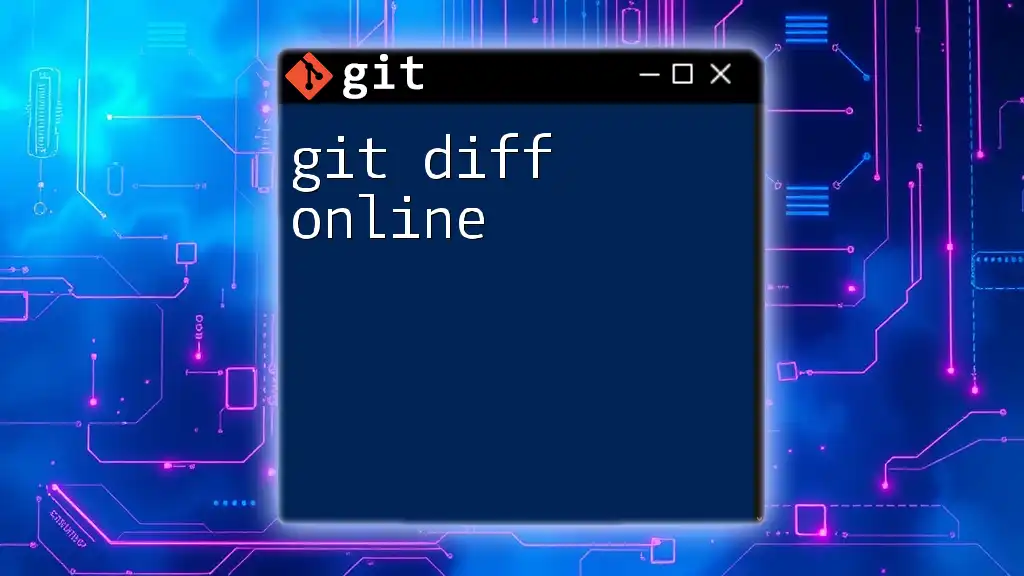
Conclusion
The `git diff` command is an indispensable part of using Git effectively, providing clarity on file changes and contributing to more efficient code collaboration. By regularly utilizing this command, you can sharpen your version control skills and maintain clean, manageable code. Embrace the power of `git diff 2 files` to stay organized and informed as you work within your projects.
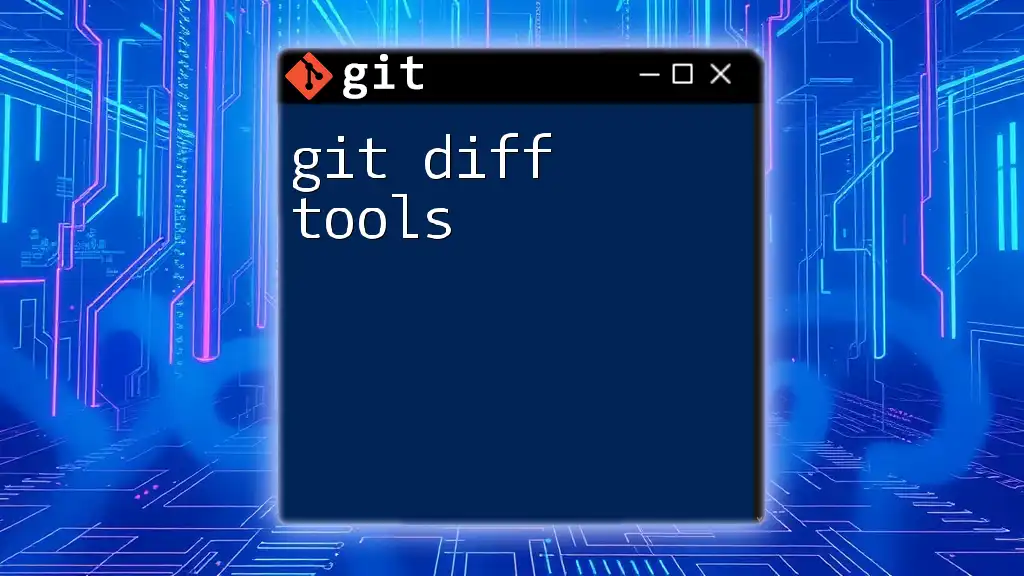
Additional Resources
To further enhance your understanding of Git, consider exploring books, online tutorials, and resources from communities specializing in version control. Engaging with fellow developers can provide additional insights and techniques, fostering your growth as a proficient Git user.