The `git diff` command is used to compare changes in your working directory against the index or a previous commit, and you can save the output to a file for further review using the following command:
git diff > changes.diff
Understanding `git diff`
What is `git diff`?
`git diff` is a powerful command that allows you to see the differences between various states of your files and repositories. It provides insights into what changes are staged, what has been modified, and what remains unchanged. Understanding how to effectively use this command is crucial for anyone working with version control, as it helps in tracking changes, reviewing code, and collaborating with others.
How `git diff` Works
Comparative Analysis: The command primarily acts as a comparator. By default, it compares the working directory against the staging area. However, you can also compare different commits, branches, or tags by specifying those in your command.
Output: The output of the `git diff` command is typically a summary of changes that includes the lines added and removed, marked with `+` and `-` respectively. The default output is in a human-readable format, making it easy to identify changes at a glance.
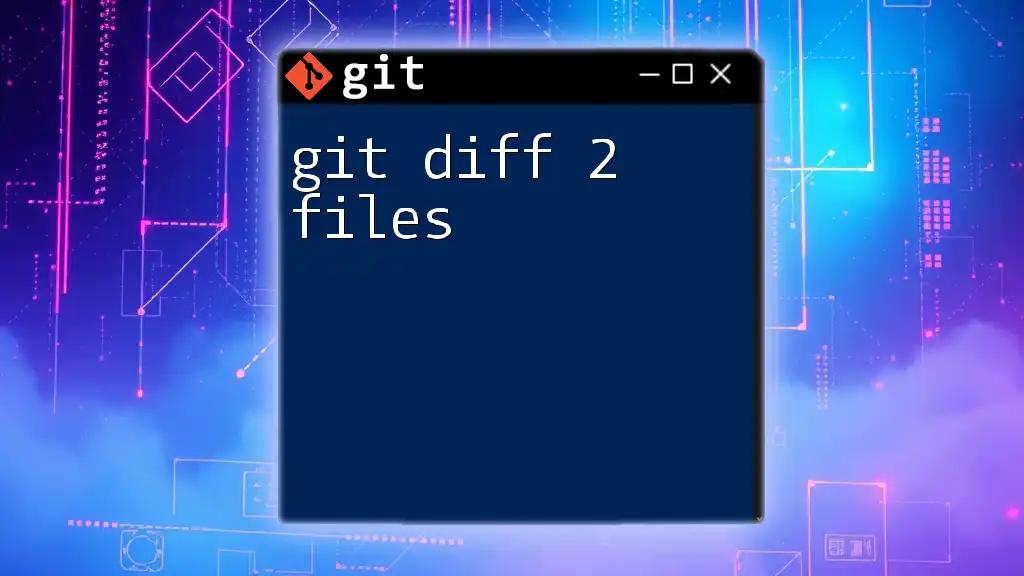
Using `git diff` with File Outputs
Basic Syntax
To utilize the `git diff` command, the basic syntax is as follows:
git diff <options> [<commit> <commit>] [--] [<path>...]
- `<options>` can include various flags to adjust the output format or the scope of the diff.
- `<commit>` specifies the commits you wish to compare; if left empty, it compares the working directory to the staging area.
- `<path>` can be used to limit the scope to specific files or directories.
Redirecting Output to a File
Using Output Redirection
You can easily save the output of `git diff` directly to a file by using the greater-than sign (`>`). This method is straightforward and effective for reference.
For example:
git diff > output.diff
This command captures all the differences in the current working directory and saves them to a file named `output.diff`. You can then open this file in any text editor to review the changes at your convenience.
Using the `--output` Option
Another way to save the output of `git diff` is by using the `--output` option, which allows for clarity in the command.
For example:
git diff --output=my_diff_output.txt
This command performs the same action as the previous one, but explicitly defines the output file in the command itself, which can be easier to read and understand.
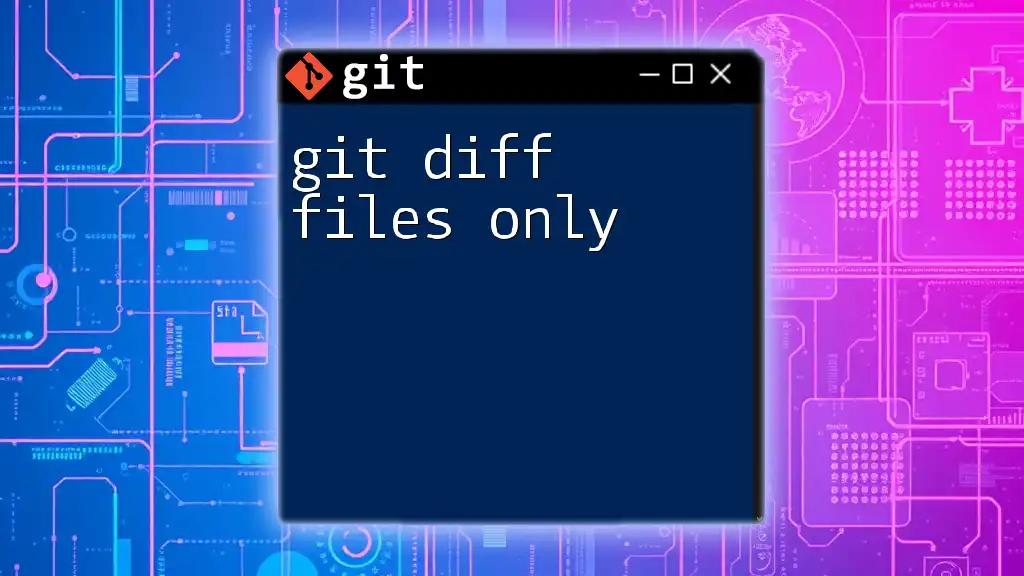
Practical Use Cases for `git diff` to File
Reviewing Changes
Capturing the output of `git diff` in a file can significantly enhance your code review process. By saving the diff to a file, you can take the time to reflect on changes without needing to flip through command line outputs. This makes it easier to comment on or discuss modifications in a collaborative environment.
Debugging Code
When bugs or discrepancies arise, leveraging the diff output file can unveil differences between expected and actual code paths. For instance, after making a series of changes to troubleshoot a bug, running:
git diff > debug_changes.diff
allows you to analyze what was altered and identify potential problems in your logic or implementation.
Collaboration and Sharing
Sharing the diff output file with your team can streamline discussions around code changes. Instead of running `git diff` in your terminal and sharing the output text directly, you can reference specific files that list the changes, making it easier for others to review and provide feedback.
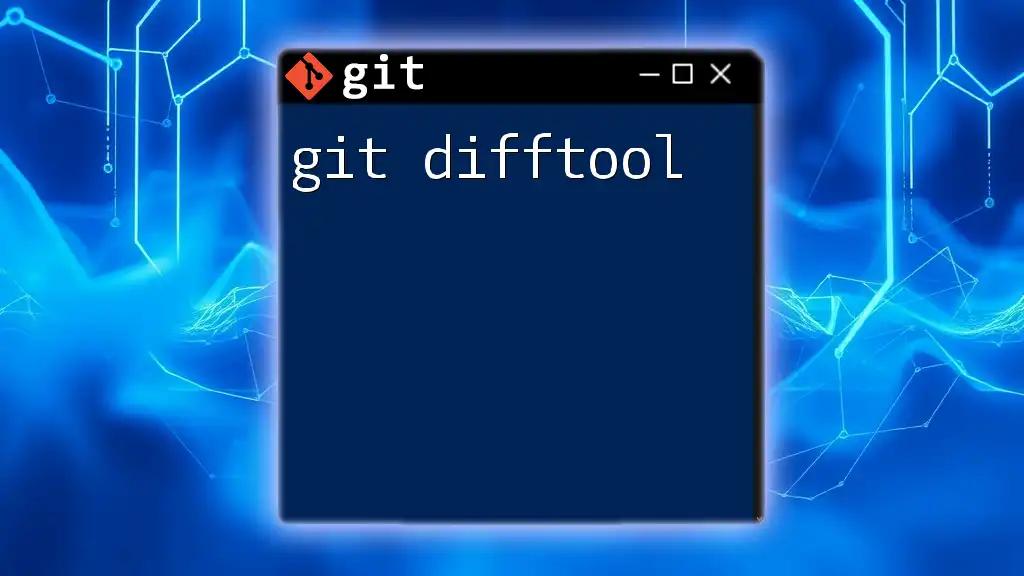
Enhancing Workflow with Aliases
Creating Useful Aliases
Creating a Git alias can dramatically improve your efficiency when using the `git diff` command. By abbreviating complex commands into single-word references, developers can save time and reduce the cognitive load of frequent command usage.
To create an alias for dumping the diff to a file, use the following command:
git config --global alias.difftofile '!git diff > output.diff && echo "Diff saved to output.diff"'
This command sets up a new alias (`difftofile`) that, when executed, runs `git diff`, saves it to `output.diff`, and outputs a message indicating success.
Using Aliases in Command Line
Once you’ve established an alias, using it becomes as simple as typing:
git difftofile
This streamlined approach elevates your workflow efficiency, allowing you to focus more on the coding aspects rather than the mechanics of command entry.
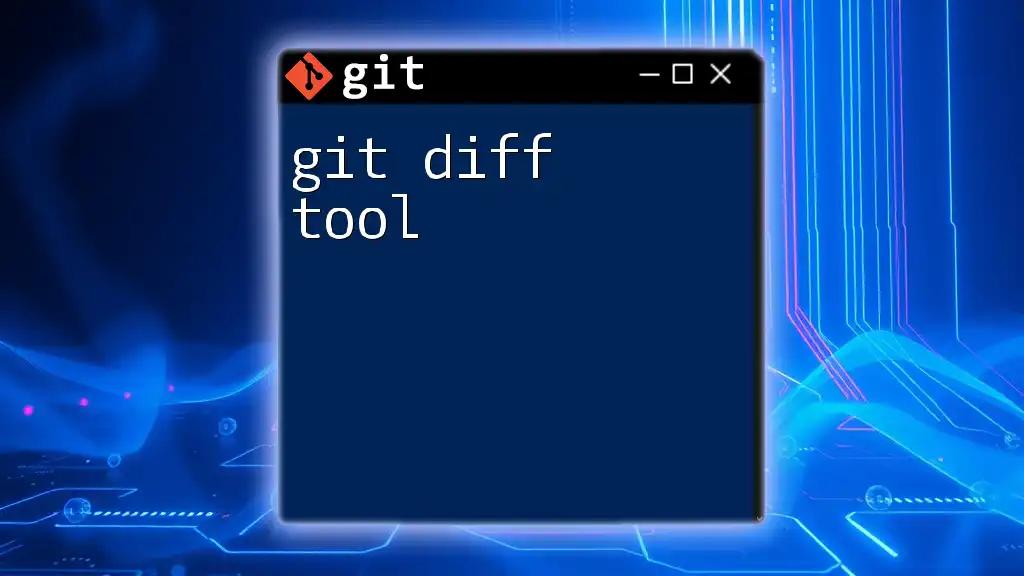
Common Issues and Troubleshooting
Error Messages
While using `git diff` can be straightforward, you may encounter errors, especially related to untracked files or files in the staging area. Common issues include:
- No changes added to commit: This occurs when you attempt to check differences without any modifications to your tracked files.
- Pathspec '...' did not match any files: This error suggests that you've indicated files that Git cannot find within the specified scope.
To troubleshoot, ensure you are in the correct directory and that changes have indeed been made to the target files.
Filtering Output
In certain scenarios, you may only want to see differences relevant to specific file types or changes. For example, if you only want to review changes made in JavaScript files, you can use:
git diff -- '*.js' > js_changes.diff
This command filters the output to only include JavaScript files, saving those differences to `js_changes.diff` for easier review.
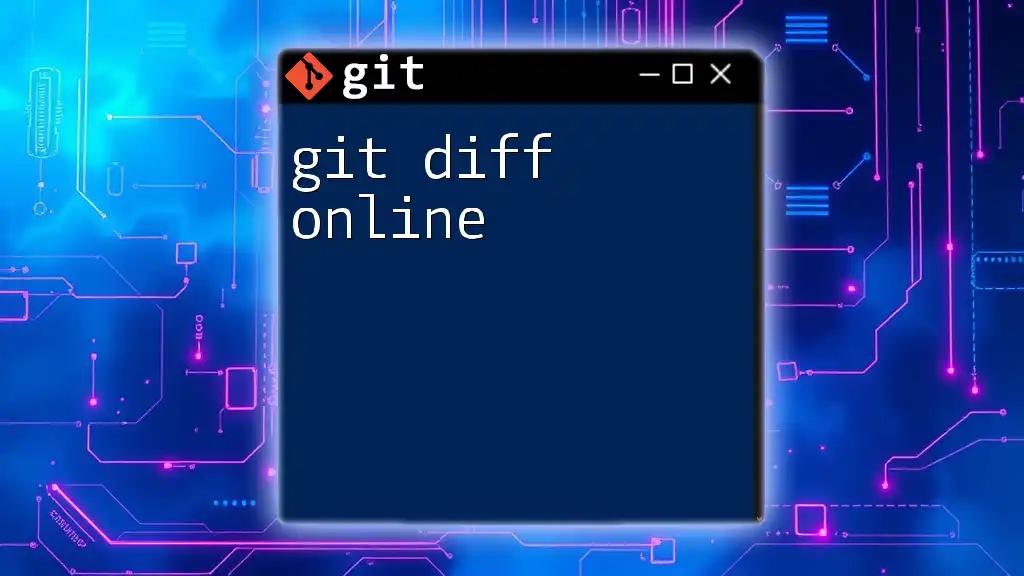
Conclusion
Utilizing `git diff` effectively, especially in creating file outputs, is a vital skill for any developer using Git. It empowers users to review changes comprehensively, debug efficiently, and collaborate seamlessly. By practicing and implementing the strategies discussed in this article, you'll enhance your Git command proficiency and streamline your development process. Explore the powerful capabilities of Git, and don’t hesitate to experiment with `git diff to file` in your own projects for best results.
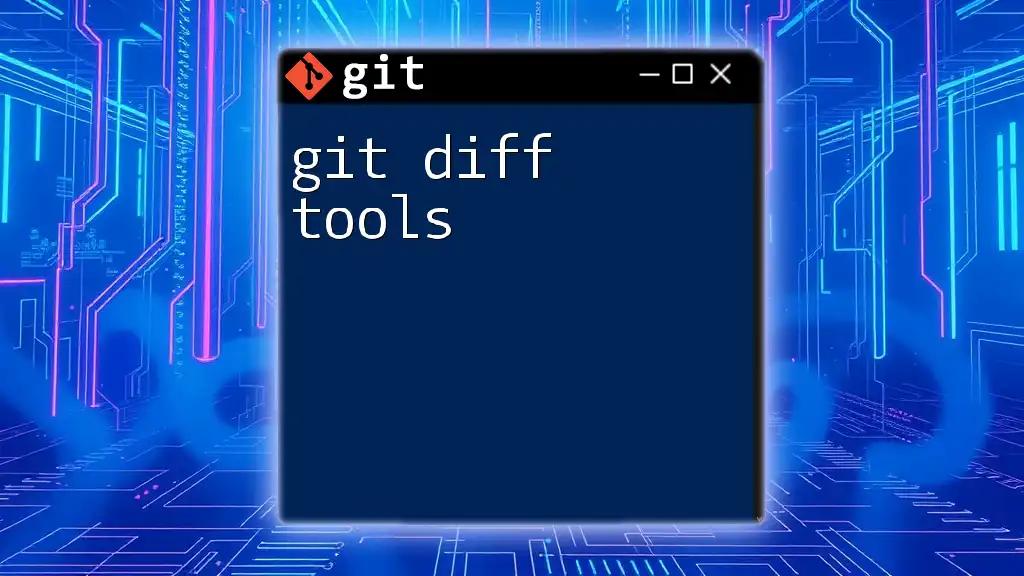
Call to Action
To further enhance your Git skills, consider subscribing for more tutorials and guides that delve into command usage and version control strategies. Share your experiences or questions in the comments section, and engage with the community to build a robust understanding of Git together!