The `git diff` command is used to compare changes between your forked repository and the original repository, allowing you to see what has been modified since you forked it.
git diff upstream/main..HEAD
What is `git diff`?
The `git diff` command is a fundamental tool in Git that allows you to compare changes between various states of your repository. It provides insights into what has been modified, added, or deleted, making it an invaluable asset for any collaborative project, particularly when working with forked repositories.
Basic Syntax of `git diff`
The basic syntax to utilize `git diff` is as follows:
git diff [options] [<commit>] [<commit>] [--] [<path>...]
Here’s a breakdown of the syntax:
- `options`: Any command line options that can modify the output, such as color formatting.
- `<commit>`: You can specify one or two commits to compare. If you provide one commit, it shows the differences from your working directory.
- `<path>`: Optional specification of the path to limit the diff to specific files or directories.
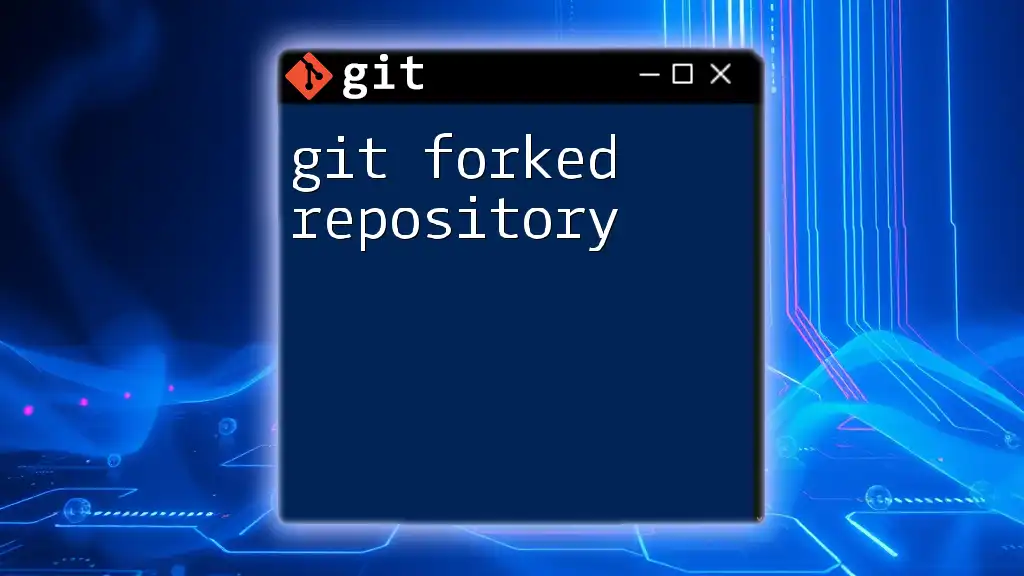
Understanding Forked Repositories
What is a Fork?
A fork is essentially a personal copy of someone else's repository, allowing you to freely experiment and make changes without affecting the original source. This is particularly crucial when collaborating on open-source projects where multiple developers contribute to the same codebase.
Why Fork a Repository?
Forking a repository serves a myriad of purposes:
- Isolated Development: You can make changes without affecting the original project, thus providing a safe environment for experimentation.
- Contribution: Forked repositories allow you to contribute to the original repository through pull requests after making changes in your fork.
- Collaboration: It promotes collaboration by enabling developers to work independently while keeping track of changes.
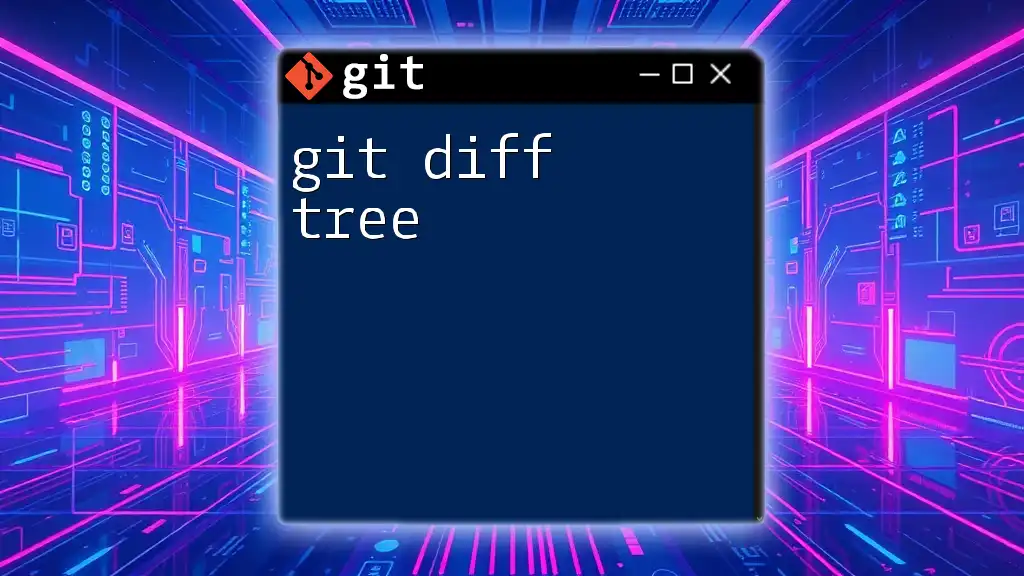
The Importance of Using `git diff` in Forked Repositories
Comparing Changes
The `git diff` command plays a pivotal role in helping you identify the differences between what exists in your fork and the original repository. This is especially important when you need to keep your fork updated or assess what you've changed before submitting a pull request.
Tracking Collaborator Changes
When working in a team, using `git diff` allows you to track changes made by collaborators. This command can be instrumental in ensuring that any modifications align with the overall goals of the project, which fosters better communication and coordination among team members.
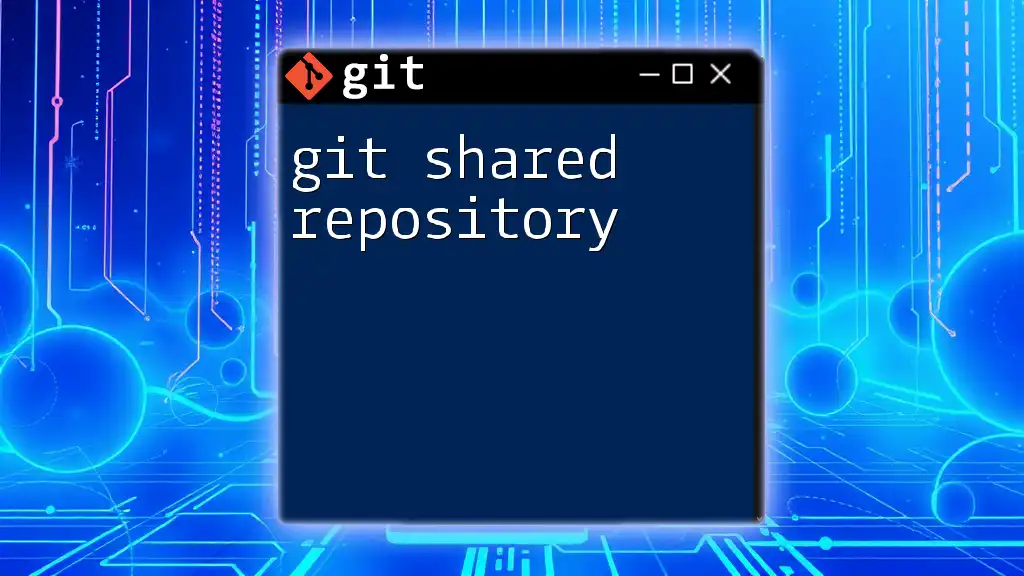
Using `git diff` in Your Forked Repository
Fetching Changes from the Original Repository
First, you will want to synchronize your fork with the original repository to keep it updated. You will need to add the original repository as an upstream remote:
git remote add upstream <original-repo-url>
git fetch upstream
This process enables you to retrieve changes from the original repository without altering your fork. The term “upstream” refers to the original repository from which your fork originated, ensuring you have a reference point for comparison.
Comparing Your Branch with the Original Repository
Once you have set up the upstream remote, you can utilize `git diff` to see how your current branch stands against the original repository. For instance, if you wish to compare your current work against the main branch of the upstream repository, you would run:
git diff upstream/main
This command compares the latest main branch from the upstream with your current branch, displaying any modifications you have made.
Comparing Specific Commits
In some cases, you may want to view the differences between specific commits within your fork. To achieve this, you can use:
git diff <commit-hash-1> <commit-hash-2>
By specifying the commit hashes, you can clearly see the changes between two particular points in your project’s history. Commit hashes enable you to access historical changes and can be found using the log command:
git log --oneline
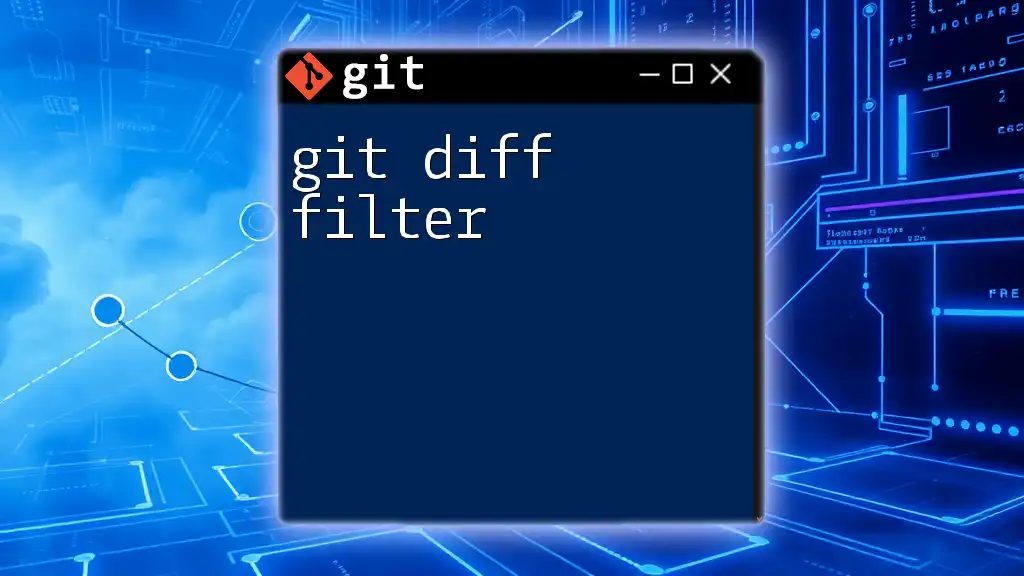
Practical Examples of `git diff`
Example Scenario 1: Finding Unmerged Changes
Imagine you’ve made multiple changes in your forked repository but haven’t committed these changes yet. By running `git diff`, you can see what alterations have been made since the last commit. This helps to maintain a clear view of what modifications are pending, allowing for better organization before you decide to commit.
Example Scenario 2: Code Review Before a Pull Request
Before submitting a pull request, you should ensure that your changes align with your project’s standards. Using the `git diff` command allows you to review your modifications thoroughly. For instance, running:
git diff upstream/main
Here, you check how your branch differs from the original main branch, providing an excellent opportunity to assess whether your changes are ready for inclusion.
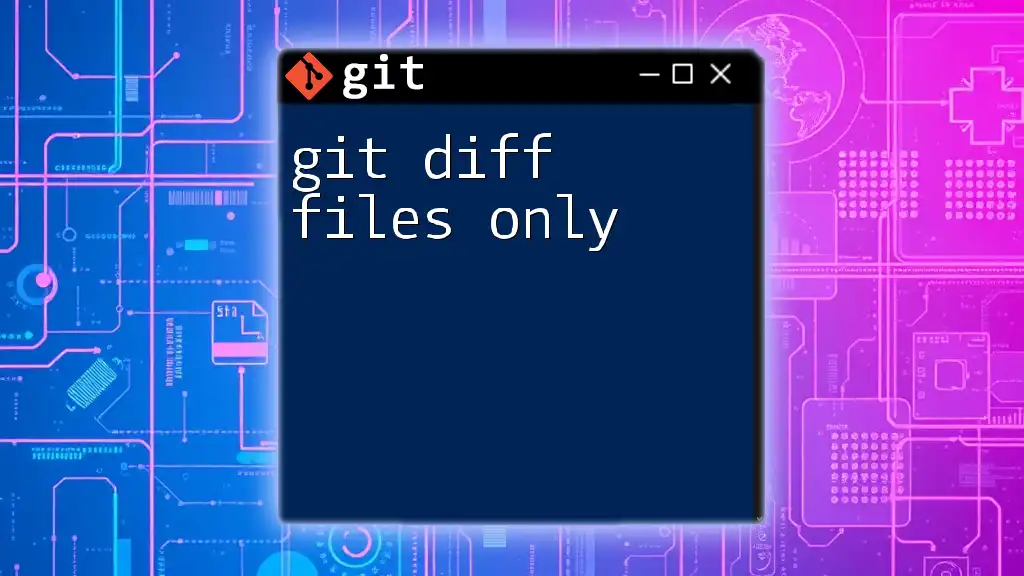
Tips for Using `git diff` Effectively
Use Diff Options
Make the most out of the `git diff` command by utilizing various options. For instance, the following commands can enhance your diff experience:
git diff --color
This command outputs changes with color, making it easier to distinguish between added and removed lines.
git diff --word-diff
This option details word-level changes instead of line-level, which can be particularly useful for tracking smaller modifications in text files.
Learn to Interpret Output
Understanding the output from `git diff` is crucial. When you run the command, you'll see a summary that indicates:
- Added lines — usually marked with a `+`.
- Removed lines — typically marked with a `-`.
- Changed lines — often displayed alongside changed contexts.
By grasping this structure, you can quickly identify the most critical changes needed for your project.
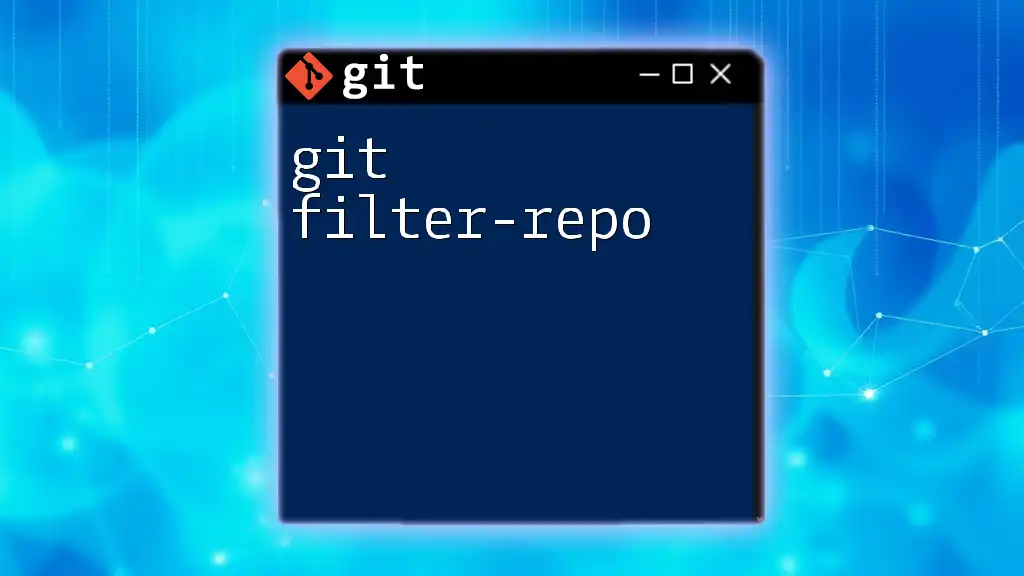
Common Issues and Troubleshooting
Common Mistakes with `git diff`
Users often make mistakes when interpreting changes from `git diff`. It's essential to ensure that you are comparing the right branches or commits. If you are unsure, run `git branch` to verify your current branch.
How to Handle Conflicts
Conflicts arise when two branches have incompatible changes. Although `git diff` isn't directly solvable for this issue, it helps identify the areas causing conflicts. By running `git diff`, you can see precisely where changes diverge, making it easier to reconcile differences before merging or rebasing.
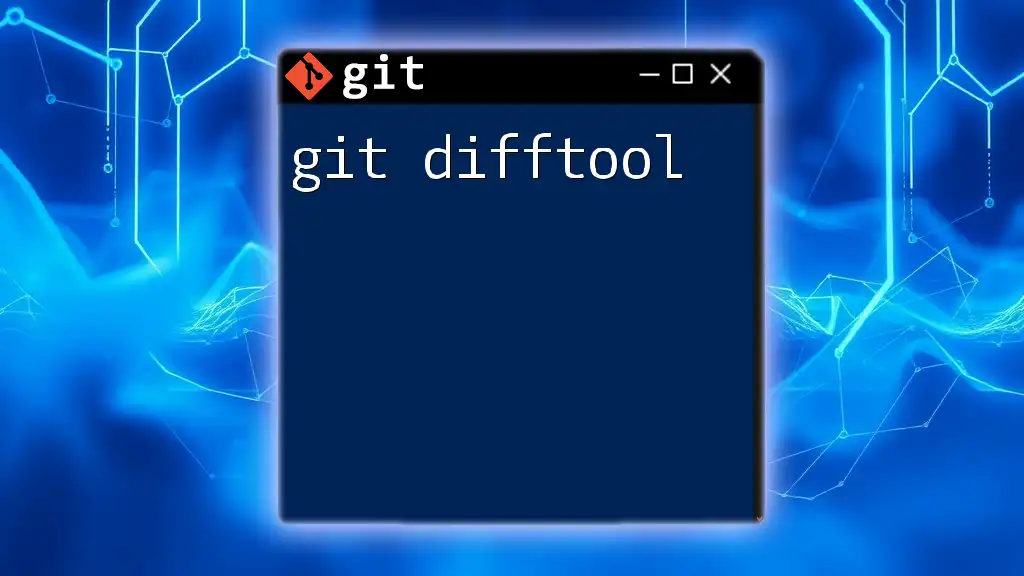
Conclusion
In conclusion, mastering the `git diff` command is vital for those working in forked repositories. It aids in comparing changes, tracking collaborators, and ensuring your work aligns with project standards. We encourage you to practice using `git diff` and become comfortable with its powerful features. By harnessing this command, you'll improve collaboration within your development team, leading to more efficient and organized workflows.
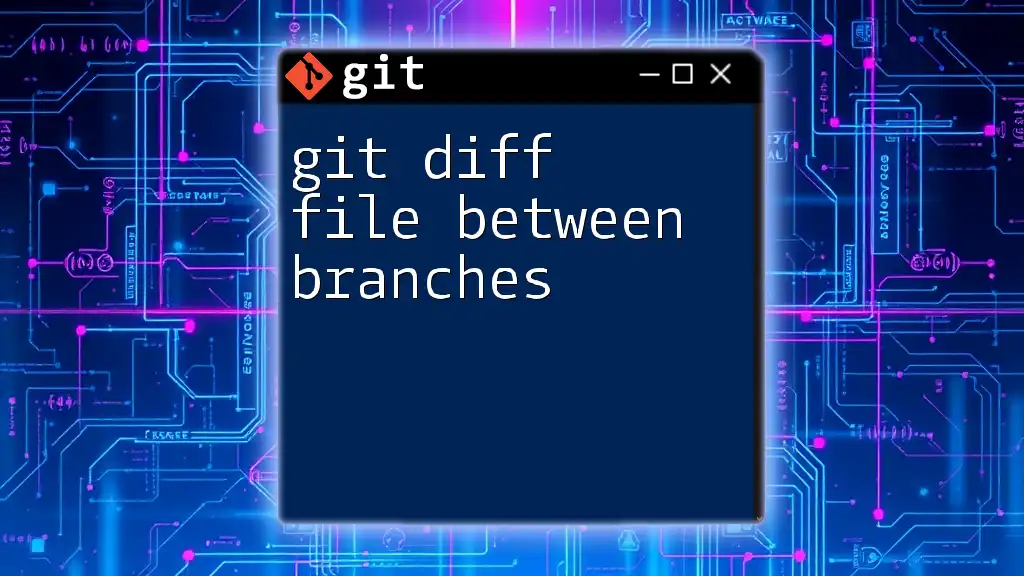
Additional Resources
For further reading, you can explore the official [Git documentation](https://git-scm.com/doc) to deepen your understanding of Git commands and practices. Additionally, consider leveraging tools like Git GUIs for visual representation of changes to reinforce your learning.
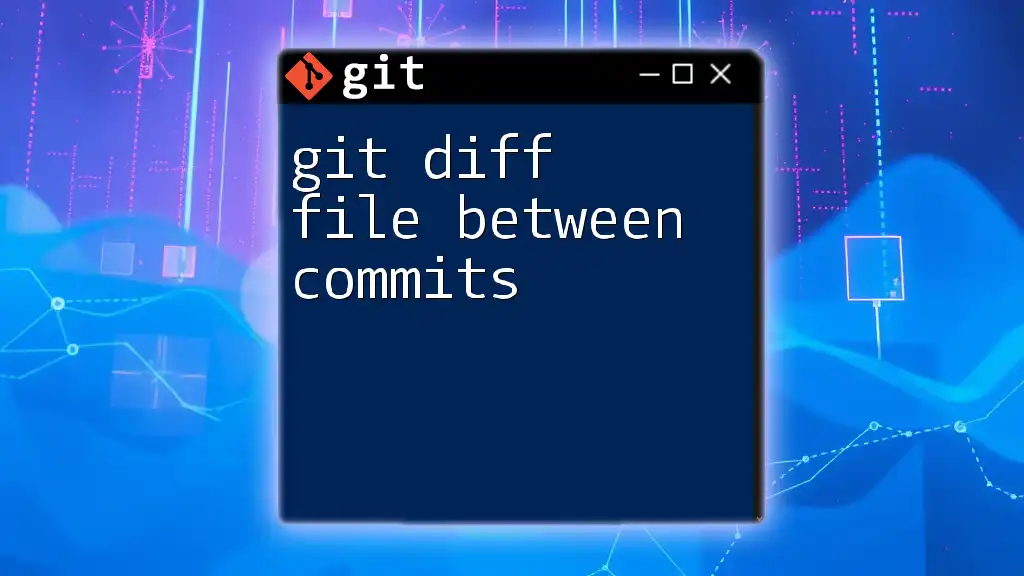
Call to Action
If you’re interested in enhancing your Git skills, be sure to download our cheat sheets or guides on commonly used Git commands. Don't forget to subscribe to our newsletter for ongoing tips and tutorials that will make your development experience more productive!