The `git diff` tool allows you to view changes between various states in your Git repository, such as unstaged changes, staged changes, or differences between commits.
git diff
Understanding `git diff`
The `git diff` tool is a fundamental component of Git, allowing users to visualize changes made in their files, whether those changes are in the working directory, staged for a commit, or already committed to the repository. Its basic syntax is as follows:
git diff [options] [<commit>] [<commit>]
What Does `git diff` Compare?
The `git diff` tool compares changes in various scopes:
-
Working Directory vs. Staging Area: When you modify files in your working directory, these changes are not yet staged for commit. The git diff tool lets you see what you have changed since the last commit.
-
Staging Area vs. Last Commit: Once changes are staged with `git add`, `git diff` can show you what changes are ready to be committed.
-
Between Commits: The tool can also compare two specific commits, which is invaluable for understanding the history of changes within your project.
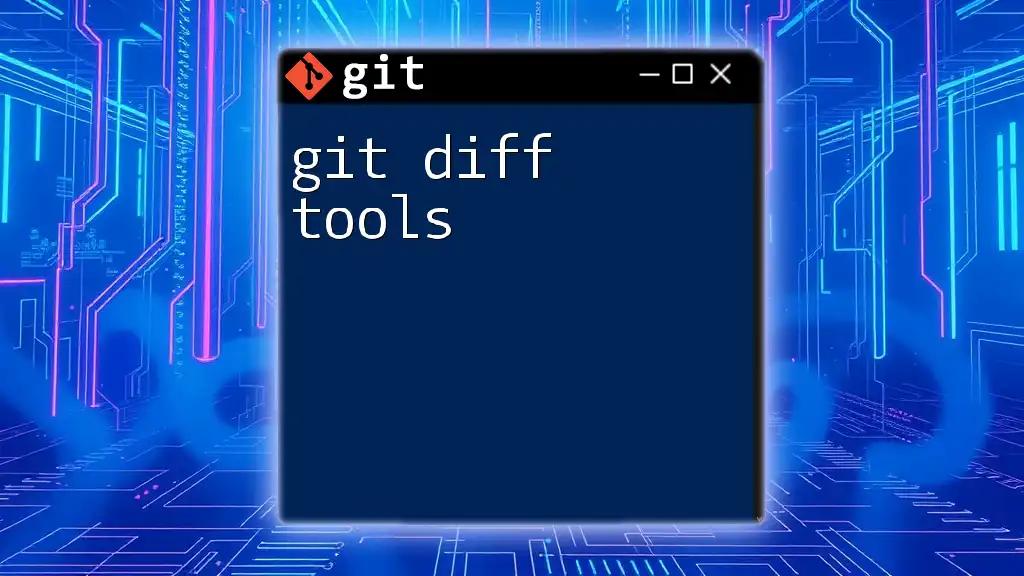
Basic Usage of `git diff`
Comparing Working Directory Changes
To see what you have modified but not staged, simply run:
git diff
This command provides a straightforward output showing lines added and removed, helping you understand your current changes. Lines starting with a plus sign (+) indicate additions, while lines with a minus sign (-) show deletions.
Comparing Staged Changes
To review changes that have been staged but not yet committed, the following command is used:
git diff --cached
or
git diff --staged
This command allows you to see what you are about to commit, ensuring that you only include the changes you intend to. The output is similar to that of the uncommitted changes, highlighting what has been staged.
Comparing Two Commits
To compare specific commits, you can leverage the commit SHA-1 hashes:
git diff <commit1> <commit2>
This comparison shows the differences between two points in your project's history. It can illustrate what has changed from one version to the next, making it easier to track progress over time.
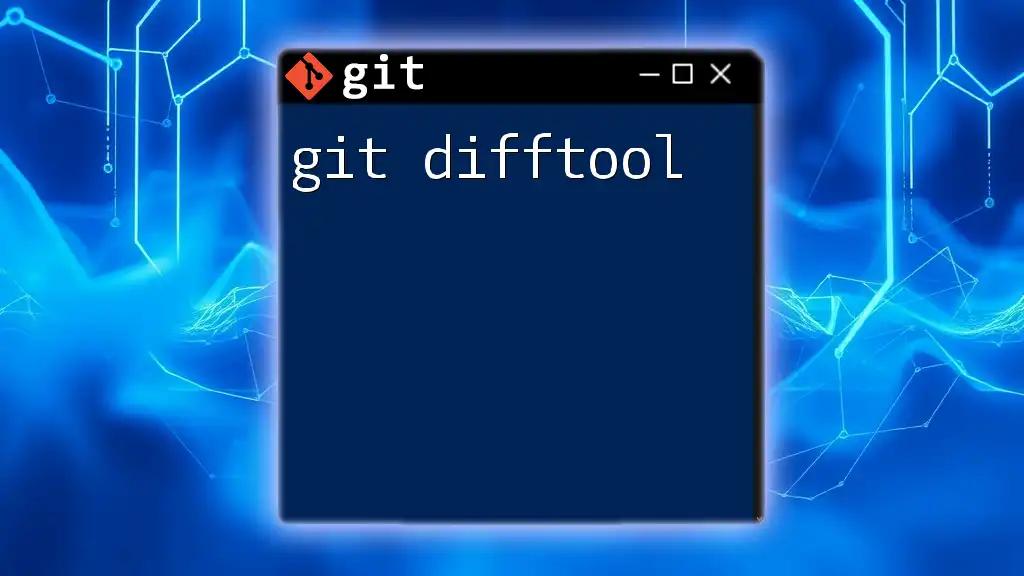
Advanced Usage of `git diff`
Diffing Specific Files
If you want to see changes made to a particular file, simply specify its name:
git diff <file>
This command is particularly useful when you're working in a larger codebase and need to focus on relevant changes for a specific file. The output will show the differences relative to the last committed version of that file.
Diffing Across Branches
To compare the differences between two branches, you can use:
git diff branch1..branch2
This command helps you understand how one branch deviates from another, which is especially useful during collaborative development or when merging features.
Utilizing `git diff` Options
The `git diff tool` comes equipped with several options that enhance its functionality:
- `--word-diff`: This option shows changes at the word level rather than line by line:
git diff --word-diff
It provides a more granular view of modifications, particularly beneficial for text-heavy content such as documentation.
- `--color`: Color-coded output improves readability, making it easier to distinguish between additions and removals. You can use it as follows:
git diff --color
- `--name-only`: If you only want to see the list of files that have changed, this option is apt:
git diff --name-only
The output will display the filenames without any details about the actual changes made, which can be a useful overview.
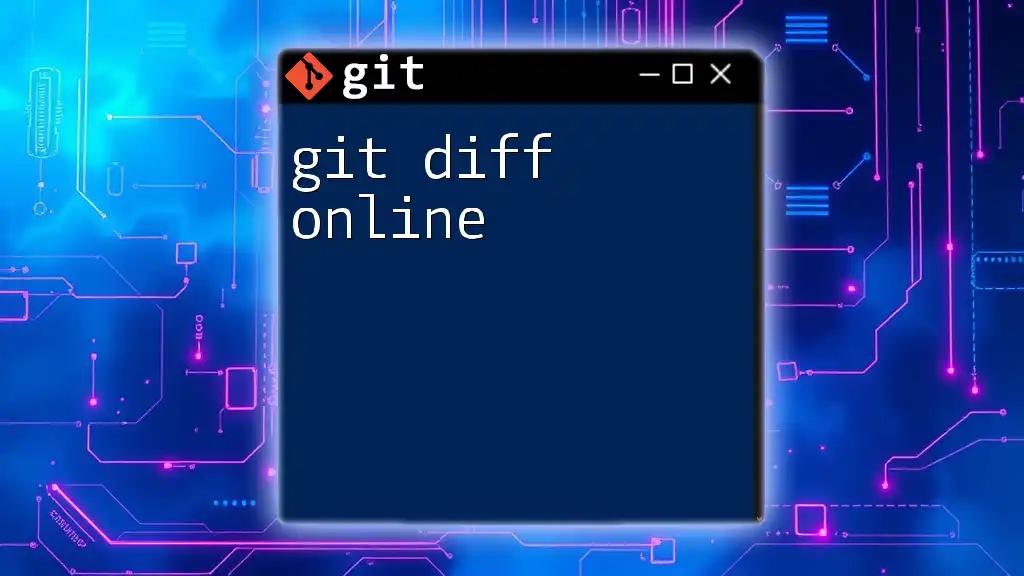
Utilizing External Diff Tools
Configuring External Diff Tools
Using external tools for diff operations can enhance your experience. These tools generally offer better visualization and are visually appealing.
Popular Diff Tools
Some popular diff tools include Meld, Beyond Compare, and WinMerge. Each comes with its own unique features and advantages.
Configuration Commands
Setting up an external diff tool, such as Meld, is simple. You can configure it using the following command:
git config --global diff.tool meld
This setup allows you to leverage Meld's user-friendly interface whenever you run a diff command.
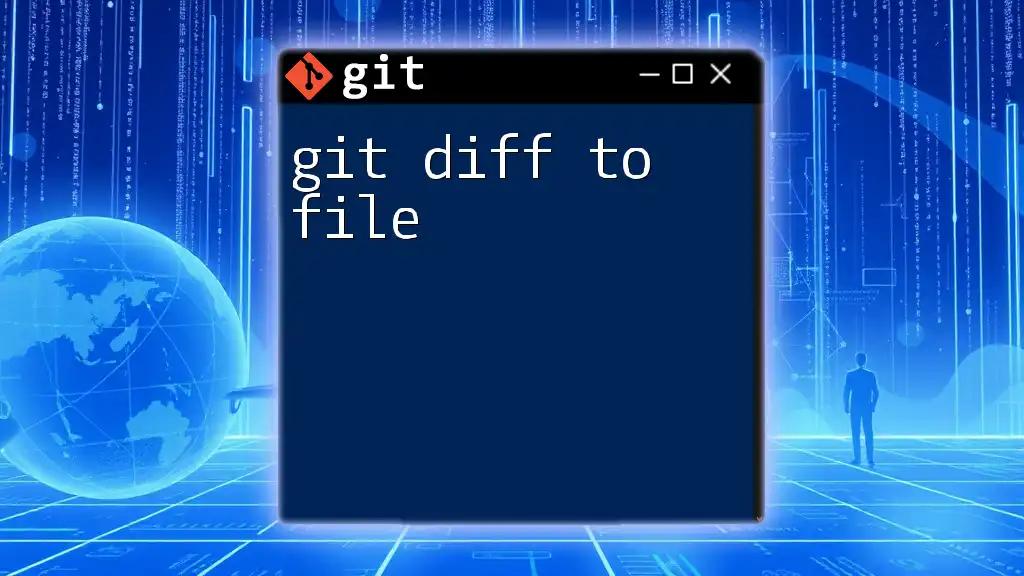
Interpreting `git diff` Output
Highlighting Differences
Understanding the output of the `git diff tool` is crucial for effective version control. The output typically displays:
- Added lines: Indicated by a plus sign (+).
- Removed lines: Marked with a minus sign (-).
For example, you might see:
+ New line of text
- Line of text that has been removed
This output provides a clear visual representation of changes, helping you grasp your modifications quickly.
Context Lines
Each diff output also includes context lines to give you some surrounding content, helping you better understand where changes fit in the overall code. You can adjust the number of context lines using the `-U` option:
git diff -U2
This command changes the context shown to two lines above and below the changes.
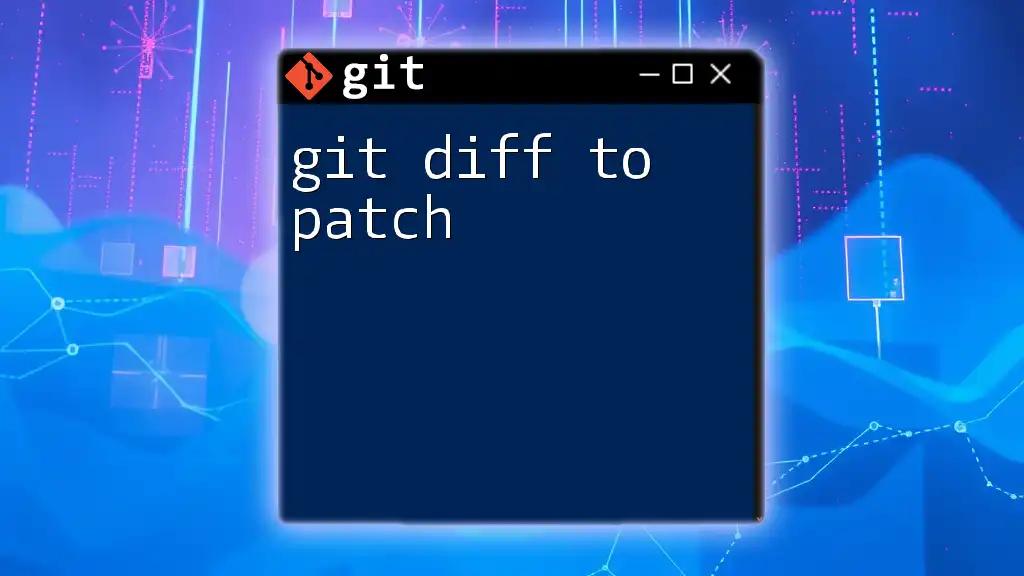
Practical Examples and Use Cases
Real-Life Scenarios
The `git diff tool` is incredibly useful in real-life scenarios such as code reviews, where it helps team members understand what changes have been proposed. A clear view of modifications is essential for providing constructive feedback.
Debugging Changes
When dealing with bugs, the git diff tool allows you to find the point where issues may have started. By comparing last known good commits with the most recent changes, you can narrow down when a bug was introduced.
Combining `git diff` with Other Commands
The `git diff tool` is not limited to standalone usage. It can be effectively combined with other Git commands:
- `git log`: To view diffs for specific revisions, you may use:
git log -p
This command shows the commit history along with diffs for each commit in the log, helping you see how changes developed over time.
- `git stash`: If you are switching branches often, `git diff` can be utilized to check differences with stashed changes:
git stash show -p
This will display the differences of the stashed changes in detail.
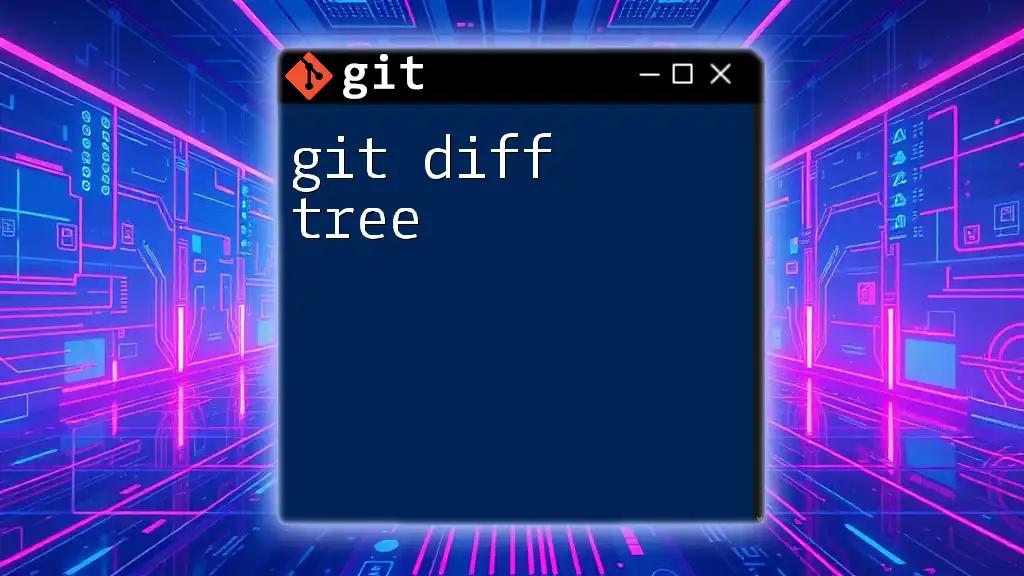
Common Issues and Troubleshooting
Misinterpretation of Diffs
One common issue users encounter is misunderstanding the output of `git diff`. It's vital to pay attention to the symbols used to differentiate between edits clearly. If something seems off, revisiting the diff with focused understanding can clarify confusion.
Handling Merge Conflicts
Using the `git diff tool` can assist when you face merge conflicts. Running `git diff` after a merge conflict allows you to see changes coming from different branches and helps you decide how best to resolve them.
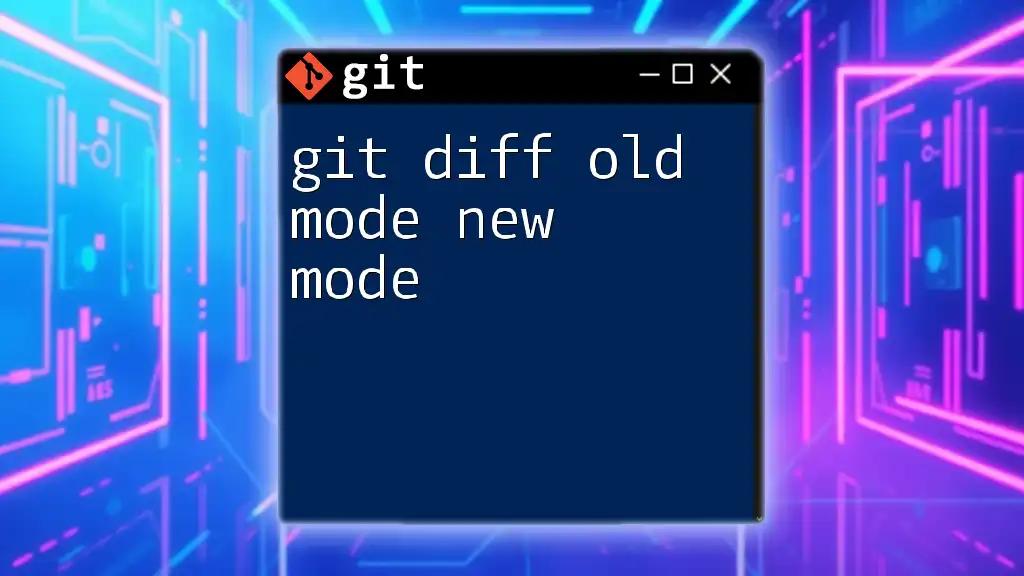
Conclusion
The `git diff tool` is an essential part of version control workflow, offering a straightforward way to visualize differences between file states. Whether you are reviewing changes, debugging issues, or preparing for a commit, mastering this tool will greatly enhance your Git proficiency. Practicing its usage in various scenarios will further solidify your skills. For those looking to dive deeper, the official Git documentation and additional tutorials are excellent resources for further learning.
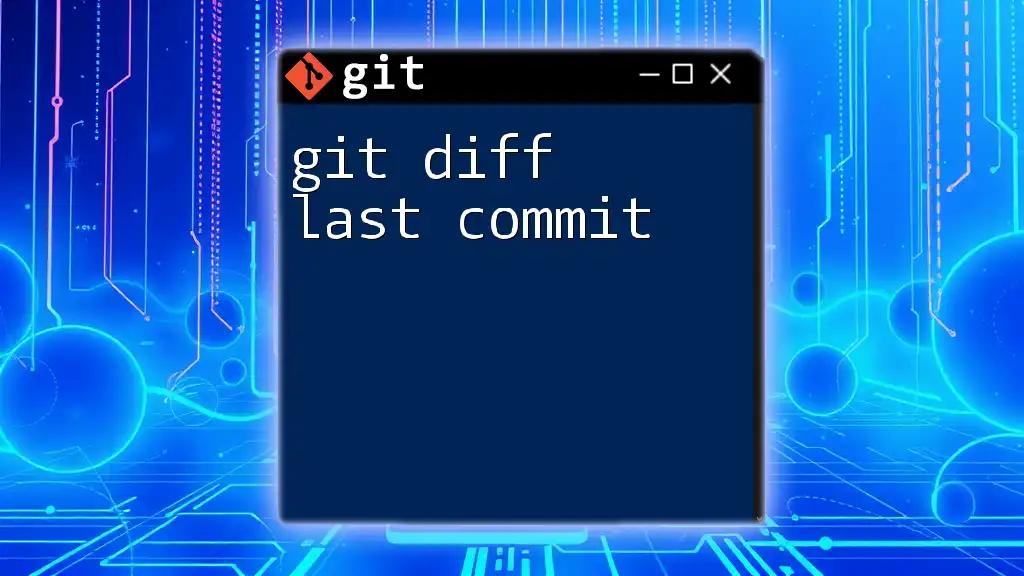
FAQs
What is the difference between `git diff` and `git status`?
While both commands are integral to Git, `git diff` specifically shows differences between file states, whereas `git status` provides an overall view of the repository's status, including which files are staged for commit, modified, or untracked.
Can `git diff` show changes for multiple files?
Yes, the `git diff tool` can display changes for multiple files at once, depending on how you specify the command. Using `git diff` without parameters shows all unstaged changes across all files.
How can I undo the changes I see with `git diff`?
If you find changes you want to undo as indicated by `git diff`, you can revert those changes using `git checkout -- <file>` to reset a file back to the last committed version or using `git reset` for changes that have been staged. However, be cautious not to lose important modifications unintentionally.