The `git diff -u` command displays the differences between the working directory and the index (staged changes) in a unified diff format, making it easier to review changes before committing.
git diff -u
What is Git Diff?
`git diff` is a powerful command that helps developers understand the differences between various states of files within a Git repository. It serves as a crucial tool for tracking changes made to the source code, allowing for better collaboration and more effective version control.

Purpose of `git diff -u`
The `-u` flag stands for unified diff format. This format presents changes in a clear way, showing both old and new lines side by side with context lines. The unified diff format is widely used in patch files, making it essential for code review, collaboration, and project management.
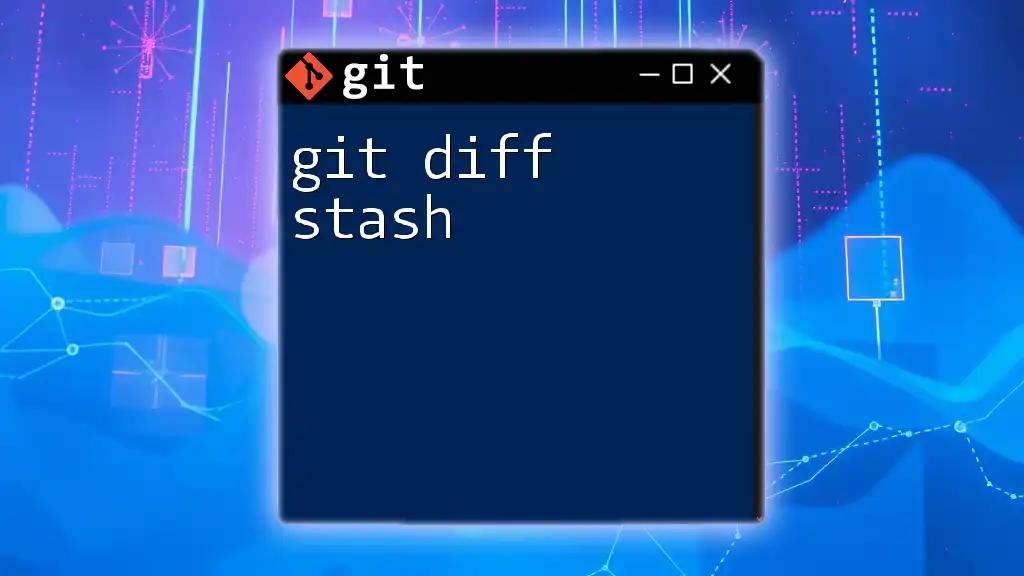
Understanding Diff Formats
Overview of Diff Formats
When using Git, you can produce different output formats for diffs, including unified diff, context diff, and normal diff. Each format serves a specific purpose. The unified diff format, however, is preferred for its clarity and conciseness, making it easier for developers to see what changed, how it changed, and the surrounding context of those changes.
Unified Diff Format Explained
The unified diff format consists of the following key elements:
- Line Numbers: Indicate where changes occur in the files.
- Context Lines: Surround the modified lines, providing a before-and-after view.
- Changes: Lines added or removed are prefixed with `+` for additions and `-` for deletions.
This format allows developers to read diffs without needing to see the entire file, making it ideal for code reviews.
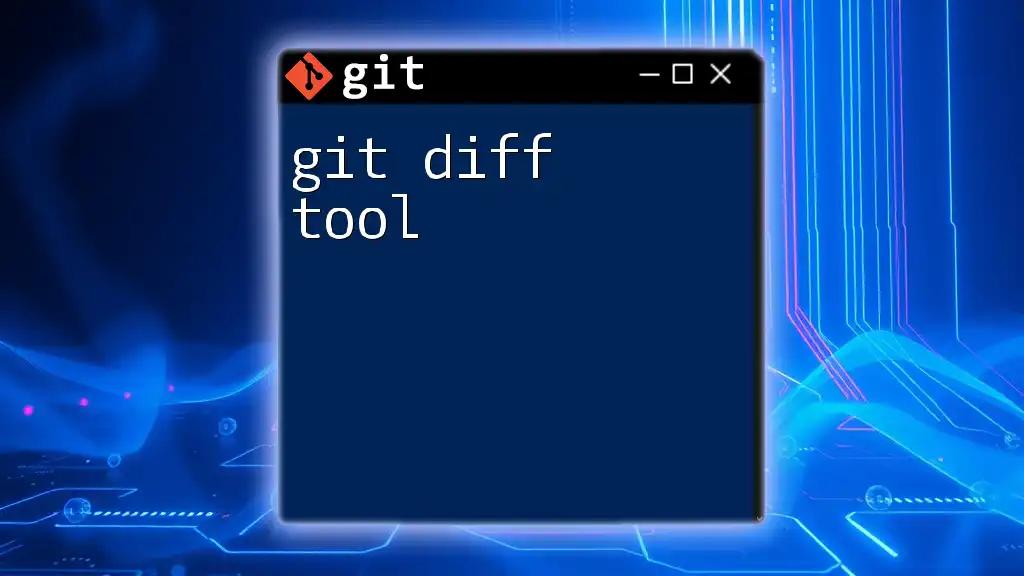
Using `git diff -u`
Basic Command Syntax
The basic command for displaying differences in unified format is:
git diff -u
This command shows changes in your working directory compared to the staging area.
Comparing Changes
Comparing Working Directory with Staging Area
To see the changes you've made in your working directory that have not yet been staged, use:
git diff -u --cached
This command reveals the differences between your staged files and the latest commit. The output shows context lines for better understanding, helping you visualize what will be committed if you run `git commit`.
Example output:
diff --git a/example.txt b/example.txt
index e69de29..d95f3ad 100644
--- a/example.txt
+++ b/example.txt
@@ -1 +1 @@
-Hello World
+Hello Git
In this example, we see that "Hello World" has been changed to "Hello Git," illustrating the change clearly.
Comparing Two Branches
To compare two branches, you can use the following command:
git diff -u branch1..branch2
This command compares the differences between `branch1` and `branch2`. The output will show not just the differences in code, but also any changes in file structure where relevant.
Comparing Specific Files
You can also compare specific files between the working directory and the latest commit with:
git diff -u file1.txt file2.txt
This will output the differences specific to those files. It is a handy way to check changes for particular pieces of code.
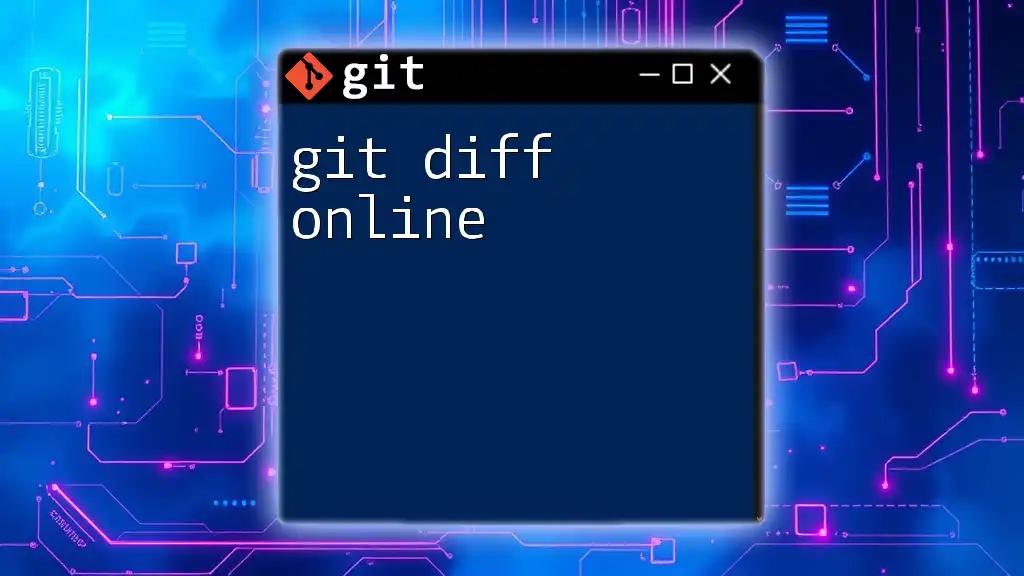
Advanced Usage of `git diff -u`
Adding Context Lines
You have the option to customize the number of context lines you want to display with the `-U` option:
git diff -u -U5
By using `-U5`, you increase the context lines displayed to five above and below the changes. This can be beneficial when you want more surrounding context to understand the modification's impact.
Customizing Diff Output
Formatting Options
`git diff` supports various flags that allow you to customize the output. For example:
- `--color`: Displays diff output in color, making it easy to distinguish between additions and deletions.
- `--word-diff`: Shows changes at a word level rather than a line level, providing even more granularity.
Example command:
git diff -u --color
This command will produce a color-coded output, making it easier to spot significant changes quickly.
Output Redirection
To save the output of `git diff -u` to a file for later analysis or sharing, you can use:
git diff -u > changes.diff
This saves your unified diff output into a file named `changes.diff`, which can be useful for code reviews or patch submissions.
Using `git diff -u` in Scripts
The `git diff -u` command can be effectively integrated into scripts, especially in Continuous Integration/Continuous Deployment (CI/CD) pipelines. Automated tests or checks can utilize this command to ensure that only approved changes make it into production, flagging discrepancies as necessary.
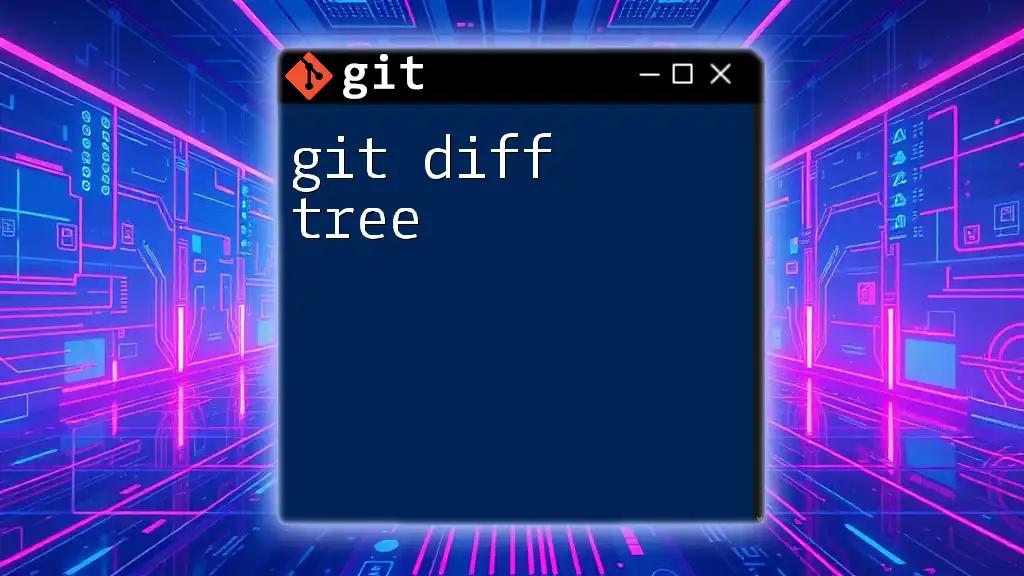
Practical Examples
Example Scenario: Code Review
In a code review, `git diff -u` becomes invaluable. Developers can quickly identify what changes have been made, why they matter, and how they impact existing code. For example, running:
git diff -u feature-branch
will display all changes made in the feature branch compared to the main branch, allowing for streamlined peer reviews.
Example Scenario: Collaborative Development
In collaborative development, knowledge of changes made by team members is crucial. Using `git diff -u`, developers can track progress and communicate effectively about modifications. If a teammate alters existing functionalities, they can run:
git diff -u origin/main
This command helps them understand how their changes diverge from the main project, facilitating better integration.
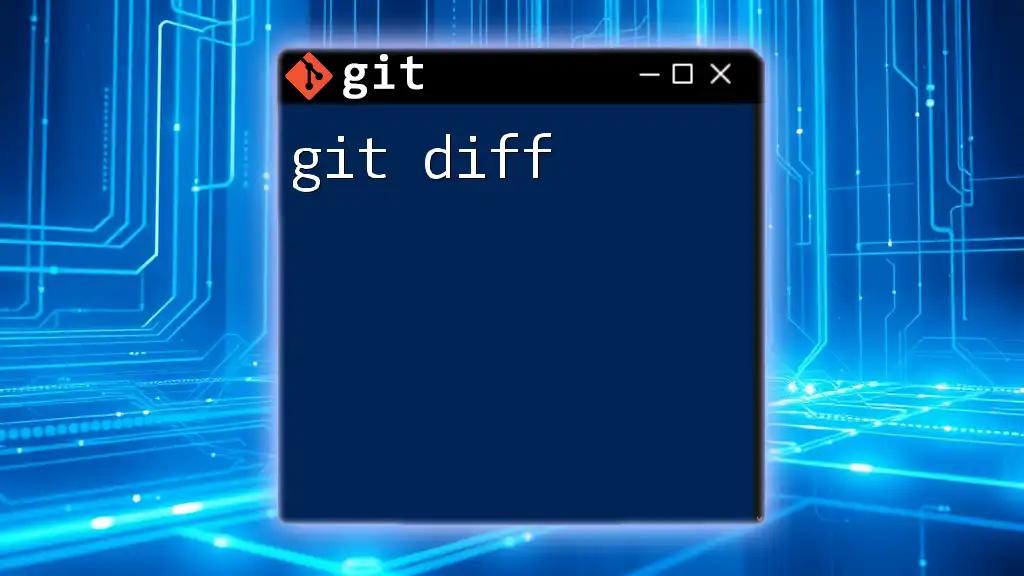
Best Practices with `git diff -u`
Regularly Check for Changes
Integrating regular checks for changes using `git diff -u` into your workflow ensures that you stay on top of modifications in your codebase. This practice aids in maintaining quality by catching errors early in the development process.
Clear and Concise Outputs
Using `git diff -u` to generate clear and concise outputs ensures that your team can easily interpret differences without confusion. This clarity is vital during code reviews or when communicating changes to non-technical stakeholders.
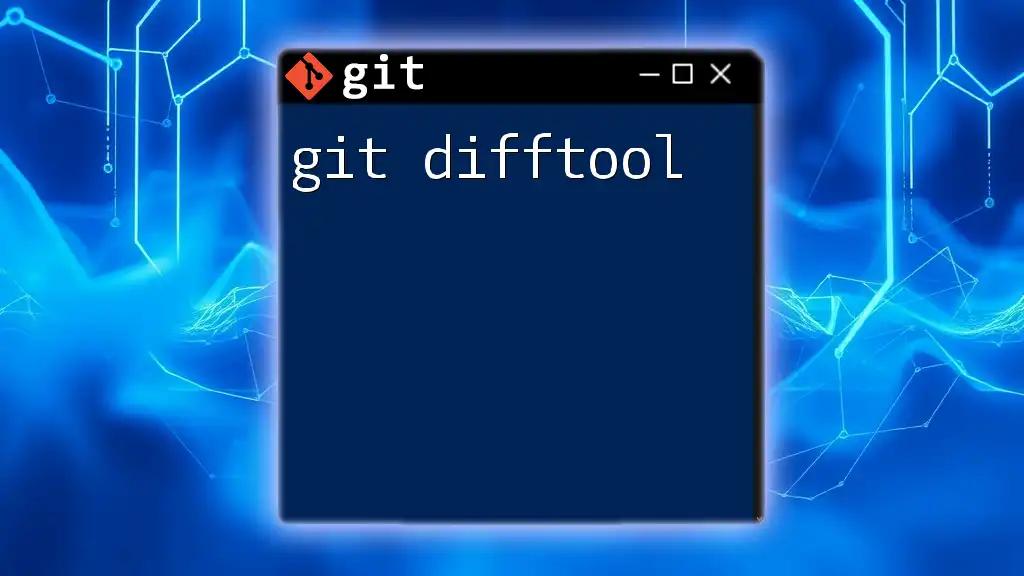
Conclusion
In summary, `git diff -u` serves as a foundational command in Git that allows developers to visualize differences between file versions effectively. By understanding and utilizing this command, those working in collaborative coding environments can improve clarity, enhance communication, and streamline their development processes.
Encouragement to Practice
The best way to become comfortable with `git diff -u` is to practice it regularly. Explore different use cases and scenarios to get accustomed to interpreting diff outputs effectively.
Resources for Further Learning
To deepen your knowledge of Git and `git diff -u`, refer to the [official Git documentation](https://git-scm.com/doc) and check out additional tutorials available online.
Engage with the Community
We encourage you to share your experiences with `git diff -u`, ask questions, and participate in discussions in the comments section. Your engagement helps foster a learning community that benefits everyone involved in version control!