The command `git diff HEAD` shows the changes in the working directory compared to the last committed state in the repository.
Here's the code snippet in markdown format:
git diff HEAD
What is `git diff HEAD`?
Definition of `git diff`
The command `git diff` serves as a powerful tool in the Git version control system, enabling users to compare changes between different states of the repository. This comparison is vital for tracking modifications made to files, whether they are uncommitted changes or changes between various commits. By using `git diff`, developers can identify what lines of code were added, modified, or deleted, facilitating a clearer understanding of their project's evolution.
Definition of `HEAD`
In Git, `HEAD` is a symbolic reference that points to the latest commit in the current branch you are working on. Essentially, it acts as a pointer, allowing you to know your current position in the repository's history. When you refer to `HEAD`, you are usually referring to the snapshot of the project at the most recent commit. Understanding how `HEAD` functions is crucial for using the `git diff` command effectively.
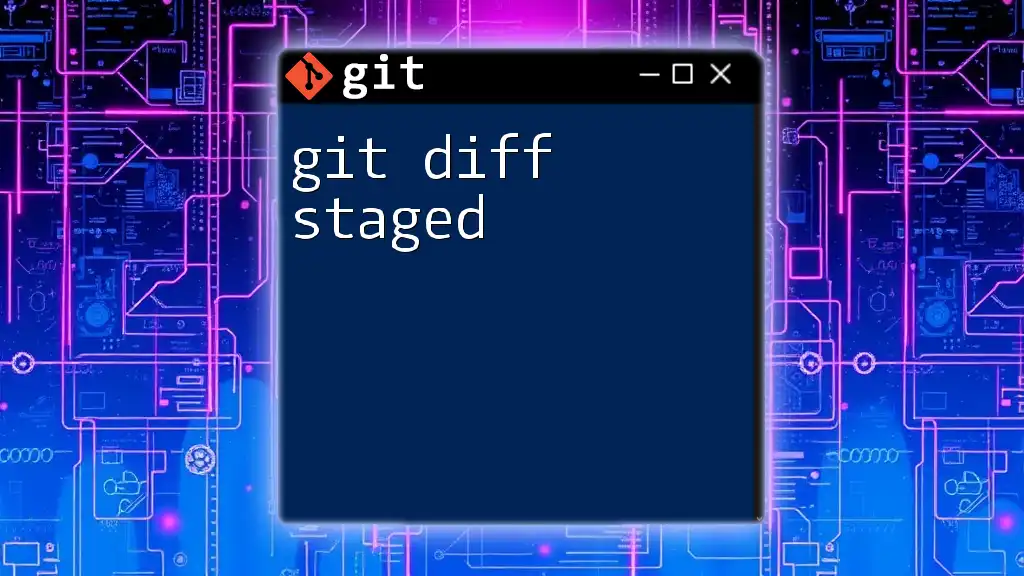
Understanding the Output of `git diff HEAD`
The Format of the Output
When you execute the command `git diff HEAD`, the output reveals the differences between the current working directory and the last commit referenced by `HEAD`. The output is structured in a way that displays:
- Lines that were added are prefixed with a `+` sign.
- Lines that were removed are prefixed with a `-` sign.
This format allows you to quickly assess what changes have taken place since your last commit.
Example Output
Here’s an example output you might see when running `git diff HEAD`:
diff --git a/example.txt b/example.txt
index e69de29..d95f3ad 100644
--- a/example.txt
+++ b/example.txt
@@ -1 +1,2 @@
-Hello World
+Hello Beautiful World
+Welcome to Git
In this example:
- The line `-Hello World` indicates that "Hello World" has been removed.
- The lines `+Hello Beautiful World` and `+Welcome to Git` show that these new lines have been added to the file.
Understanding the specifics of this output can help you make informed decisions about subsequent commits.
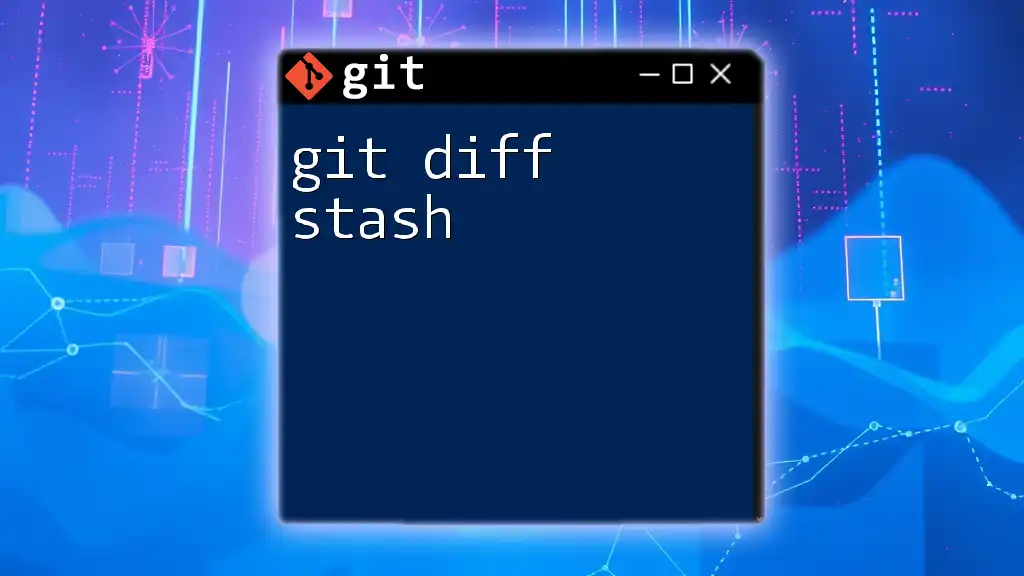
When to Use `git diff HEAD`
Use Cases
The command `git diff HEAD` is invaluable in various scenarios, such as:
- Checking changes before commit: You might want to ensure your modifications are correct and appropriate before finalizing your commit.
- Reviewing modifications made since the last commit: This command helps you recall what you’ve changed after your last save point.
- Understanding team contributions: If multiple developers are collaborating on the same repository, this command aids in recognizing individual contributions.
Scenarios of Use
- Collaborating with others on a project often requires ensuring that you are aware of any changes you’ve made since the last checkpoint in the codebase.
- Debugging issues by tracking changes can help you pinpoint when and why a bug was introduced.
- Creating a clean commit history is essential, and reviewing your changes before making a commit helps fulfill that goal.
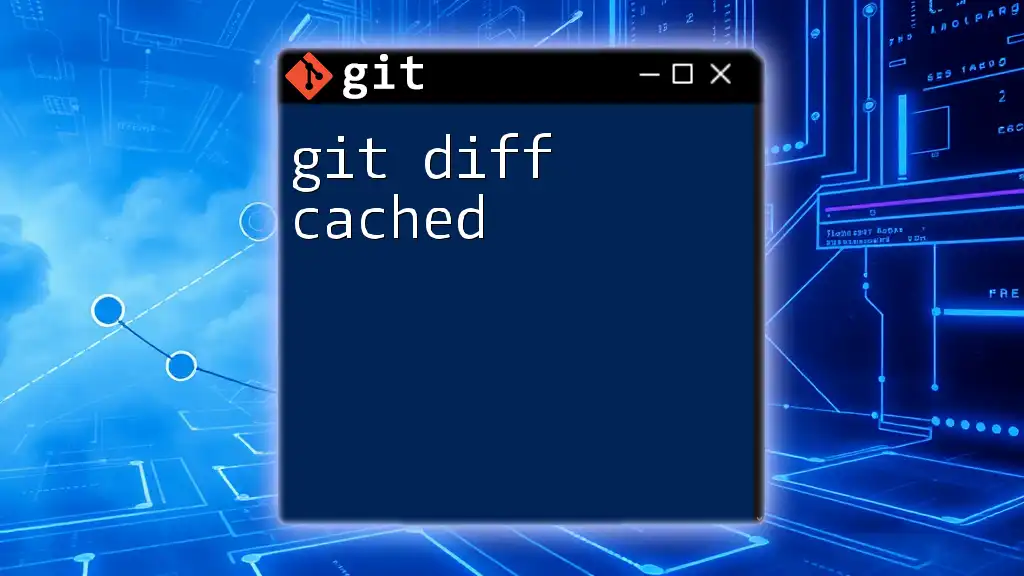
Practical Examples of `git diff HEAD`
Example 1: Basic Usage
To check the differences between your current working directory and the most recent commit, simply run:
git diff HEAD
This command will display all the changes that have not yet been staged or committed. By utilizing this command regularly, you can maintain awareness of your modifications.
Example 2: Comparing Specific Files
If you would like to inspect changes made to a specific file, you can target that file explicitly:
git diff HEAD -- path/to/file.txt
This command narrows down the output, allowing greater focus on particular files, making it easier to review significant changes without being overwhelmed by all modifications in the working directory.
Example 3: Combined with Other Flags
You can also enhance the display and readability of the output by adding additional options. For instance, using `--color` will highlight the changes:
git diff --color HEAD
This makes the output much more visually accessible, especially when working with extensive changes, as it allows for quick recognition of added and deleted lines.
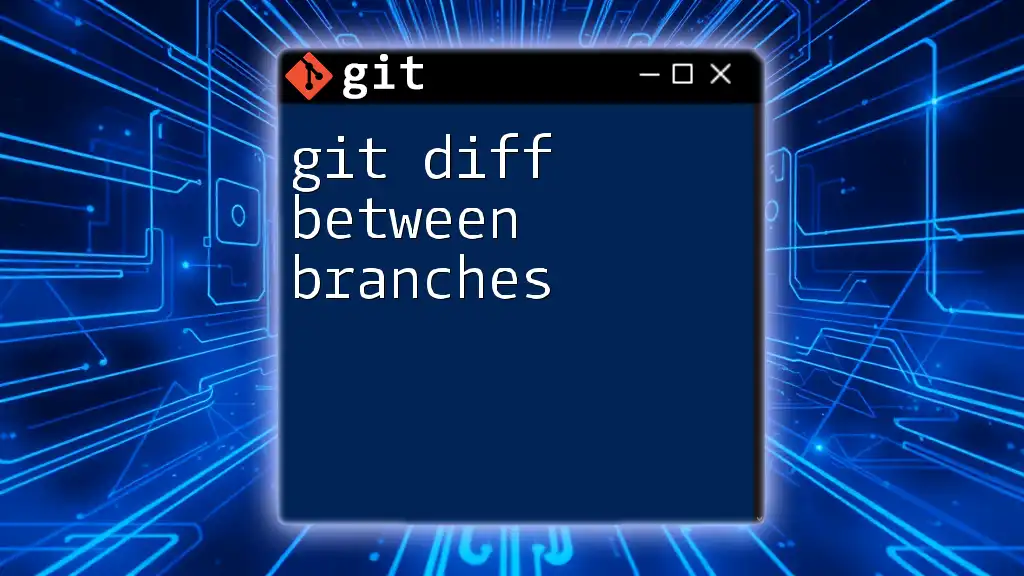
Limitations of `git diff HEAD`
Scenarios Not Accounted For
It’s essential to note that `git diff HEAD` will not show changes that have been staged for commit, as it only considers uncommitted modifications in the working directory.
Alternative Commands
To review changes that are staged in preparation for a commit, you can use the following command:
git diff --cached
This provides a view of what is set to be committed, ensuring you have a complete understanding of what will be included in your next commit.
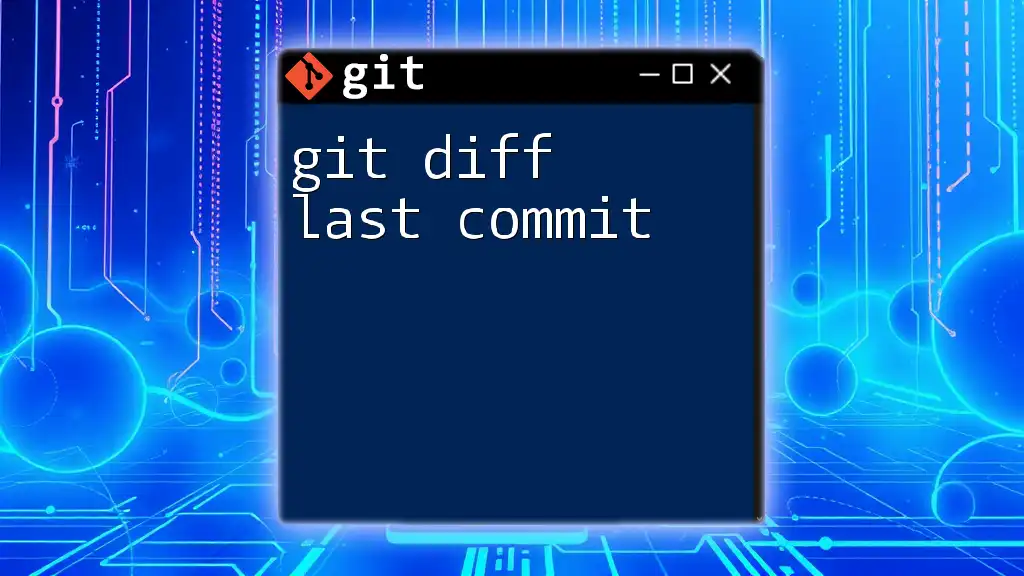
Best Practices When Using `git diff HEAD`
Organizing Your Workflow
Incorporating `git diff HEAD` into your workflow can substantially improve your efficiency. Make it a habit to check differences before committing changes. This practice can save time and avoid errors in your codebase.
Regular Review of Changes
Regularly reviewing your changes helps you maintain a grasp on project progress. Understanding what's been modified before merging or pushing to a remote repository ensures that everyone is on the same page.
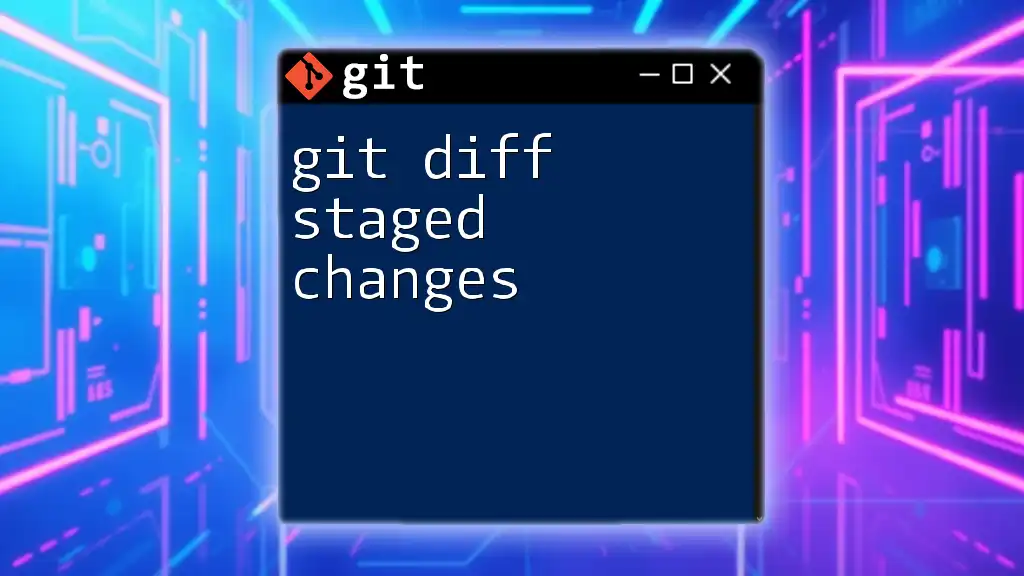
Conclusion
Mastering the command `git diff HEAD` significantly enhances a developer's ability to manage code efficiently, providing insights into modifications made at any point in time. By familiarizing yourself with this command, you can better understand your project’s history and collaborate with others more effectively. Don’t hesitate to experiment with `git diff HEAD` in your upcoming projects—it’s a small but critical step towards a more organized development process.
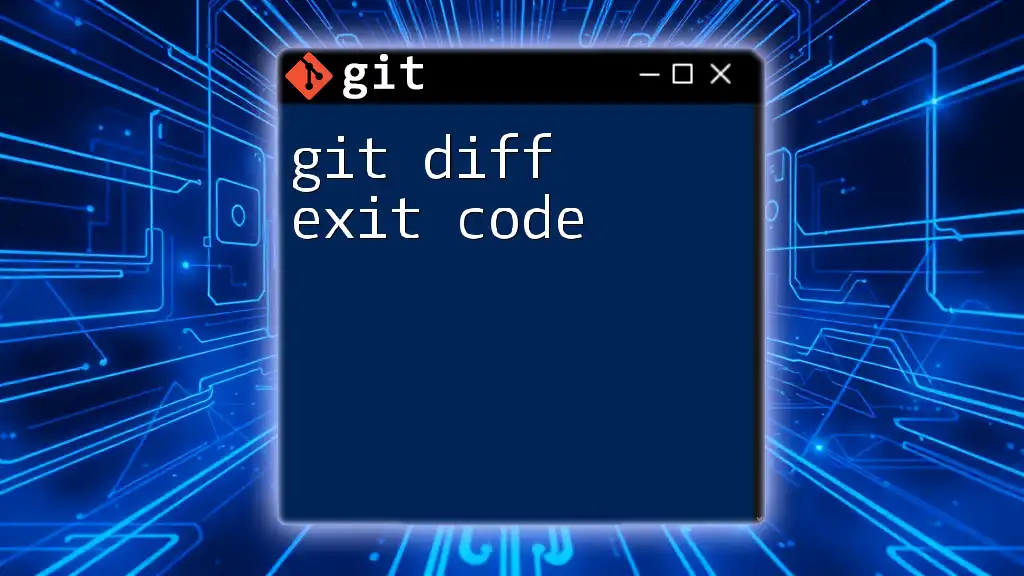
Additional Resources
For further reading and more in-depth understanding, consider exploring the official Git documentation on `git diff`. Additionally, seek out tutorials or videos that delve deeper into the intricacies of Git commands. Keep an eye on our upcoming workshops, designed to boost your Git skills and help you use these commands with confidence.
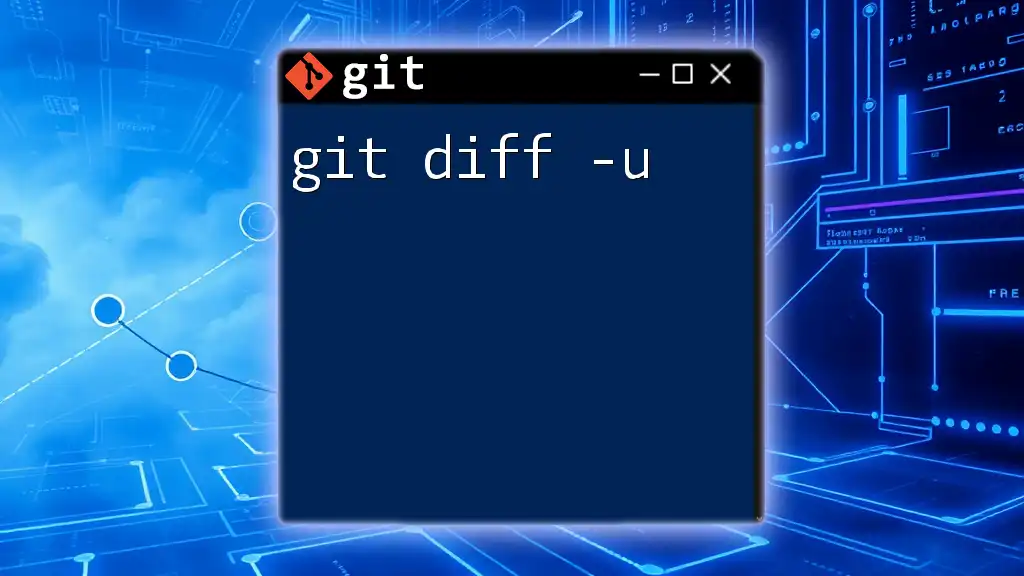
FAQ Section
Common Questions About `git diff HEAD`
What happens if there are no changes to show? If there are no changes since the last commit, running `git diff HEAD` will not display any output, indicating that your working directory is clean.
Can `git diff HEAD` help me with merge conflicts? While it can show changes made, it is not specifically designed for resolving merge conflicts. However, it can help you identify differences before committing after a merge.
Can I use `git diff HEAD` in all branches? Yes, you can use `git diff HEAD` in any branch. The output will simply reflect the latest commit of the branch you’re currently on.