The `git diff stash` command allows you to view the changes between your working directory and the last stashed state, helping you understand what modifications have been temporarily saved.
git stash show -p
What is Stashing in Git?
Definition of Stashing
Stashing in Git is a powerful feature that allows you to temporarily save your changes without committing them. This is particularly useful when you’re in the middle of coding a feature or a bug fix and need to switch to another branch without losing your current work. Stashing lets you “stash” your changes away and come back to them later, keeping your workspace clean and organized.
How to Stash Changes
To stash changes, you use the command:
git stash
When executed, this command stores your modified tracked files and staged changes in a stash, reverting your working directory to the last commit. It’s important to note that untracked files are not stashed by default unless specified.
Viewing Stashed Changes
If you want to see what you have stashed, you can run:
git stash list
This command will display a list of stashed changes, showing each stash along with an index and a short description. The output typically looks like:
stash@{0}: WIP on branch-name: commit-message
stash@{1}: WIP on branch-name: commit-message
Each entry can be referred to by its index to access specific stashes later.
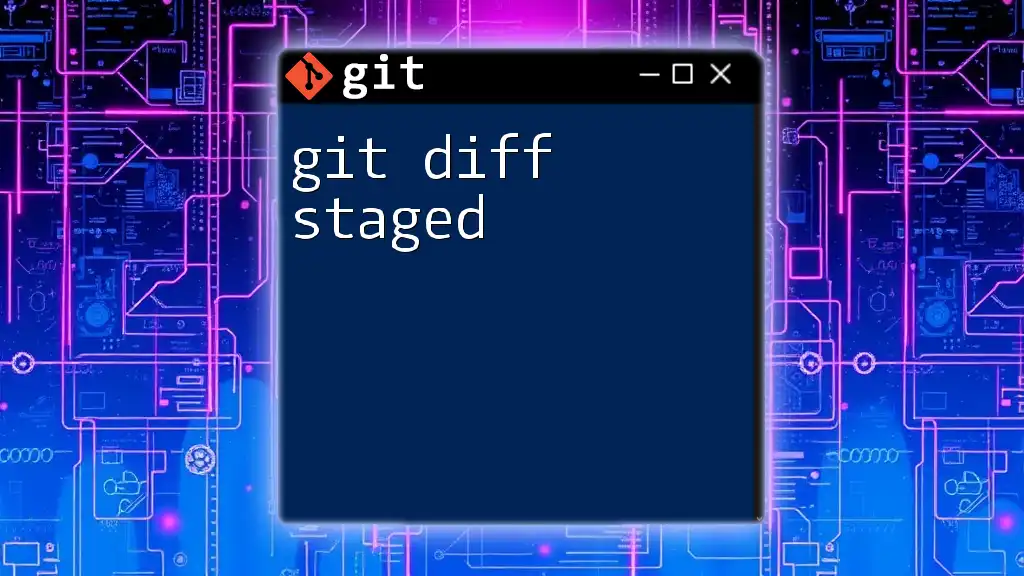
Introduction to `git diff`
What is `git diff`?
The `git diff` command is used to display the differences between various states in your repository. Whether comparing your current work against the last commit or examining differences between two commits, `git diff` serves as a valuable tool for identifying changes.
Common `git diff` Use Cases
One common use case for `git diff` is comparing the working directory changes to the last commit. You can do this simply by running:
git diff
This command shows the precise changes you’ve made since the last commit, helping you review your work before choosing to stage or commit.
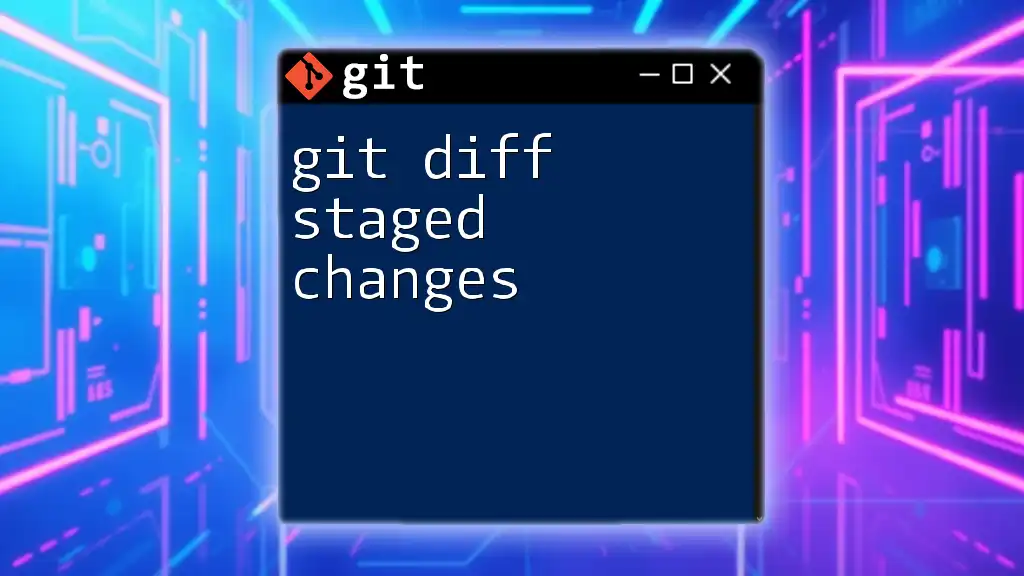
Understanding `git diff stash`
What Does `git diff stash` Do?
The `git diff stash` command allows you to view changes stored in a stash. This is crucial as it enables you to review the modifications you’ve set aside before deciding to apply or drop them. Understanding what’s included in the stash ensures you don’t reintroduce unwanted changes into your working directory.
How to Use `git diff stash`
To view the changes in a specific stash, you can use the following command:
git diff stash@{index}
Here, the `index` specifies which stash entry you want to examine. For example, running:
git diff stash@{0}
will show you the changes from the most recent stash. Remember, you can replace `0` with other index numbers to view previous stashes.
Comparing the Working Directory to Stashed Changes
In some scenarios, you may want to see how your current work compares to a stashed state. You can use:
git diff stash
This command compares the working directory with the latest stash, showcasing the differences effectively and allowing you to analyze what has changed.
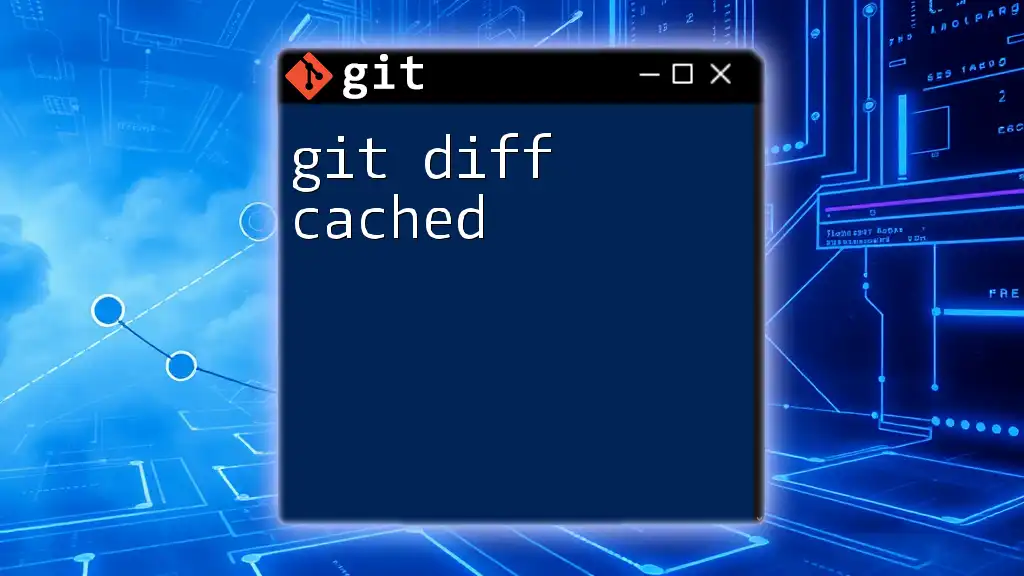
Real-Life Scenarios for Using `git diff stash`
Scenario 1: Reviewing Changes Before Applying Stash
Imagine you’re in the midst of a feature development and need to switch branches. Before applying your stash, you want to ensure it contains only relevant changes. Here’s a step-by-step guide:
-
Stash Your Changes: First, stash your work:
git stash
-
Review Stashed Changes: Check what was stashed:
git stash list
-
Compare Stash to Current Work: View differences between your stash and the current working directory:
git diff stash@{0}
-
Decide to Apply or Drop: After reviewing, if you wish to apply the stash, run:
git stash apply stash@{0}
Scenario 2: Multiple Stashes
Managing multiple stashes is crucial for an organized workflow. Suppose you've created several stashes while working on different tasks. You can use `git diff stash` to conveniently compare specific stashes:
-
List Your Stashes:
git stash list
-
Compare Specific Stashes:
git diff stash@{1} stash@{2}
This command provides insights into the differences between those two stashes, helping you decide which changes to reintroduce into your branch.
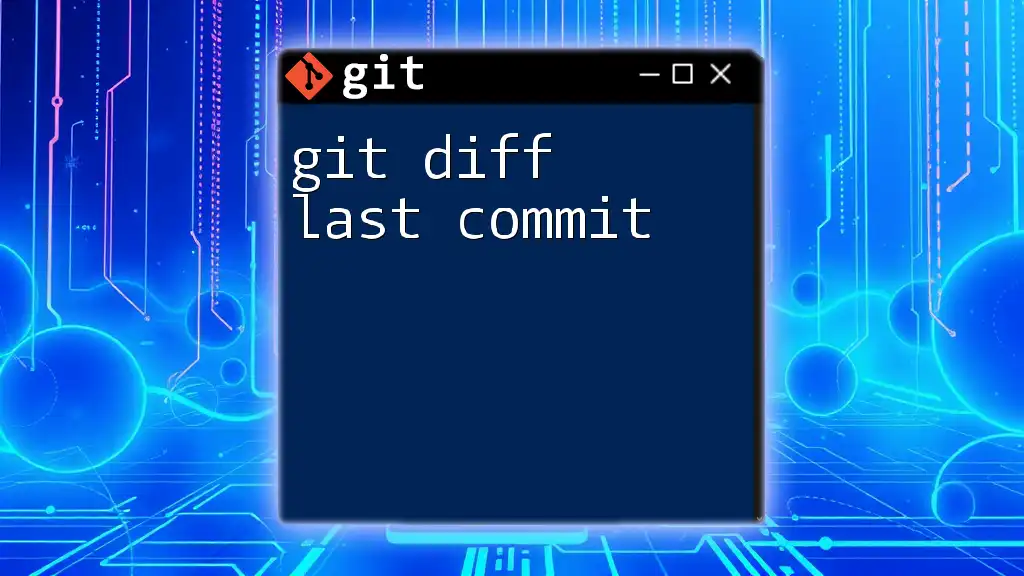
Best Practices for Using `git diff stash`
When to Use Stashing vs. Committing
While both stashing and committing serve to save changes, committing should be reserved for changes you’re ready to share with others or reflect a logical point in your project’s history. Stashing, on the other hand, is meant for temporary storage of ongoing work that isn’t ready for a commit. Understanding this difference is essential for maintaining a clean version control history.
Keeping Your Stashes Organized
To maintain an organized stash list, consider naming your stashes at the time you create them. Using:
git stash save "WIP: refactoring some functions"
allows you to track the purpose of each stash easily. Regularly check your stash list and leverage the `git stash drop stash@{index}` command to clean up unneeded stashes.
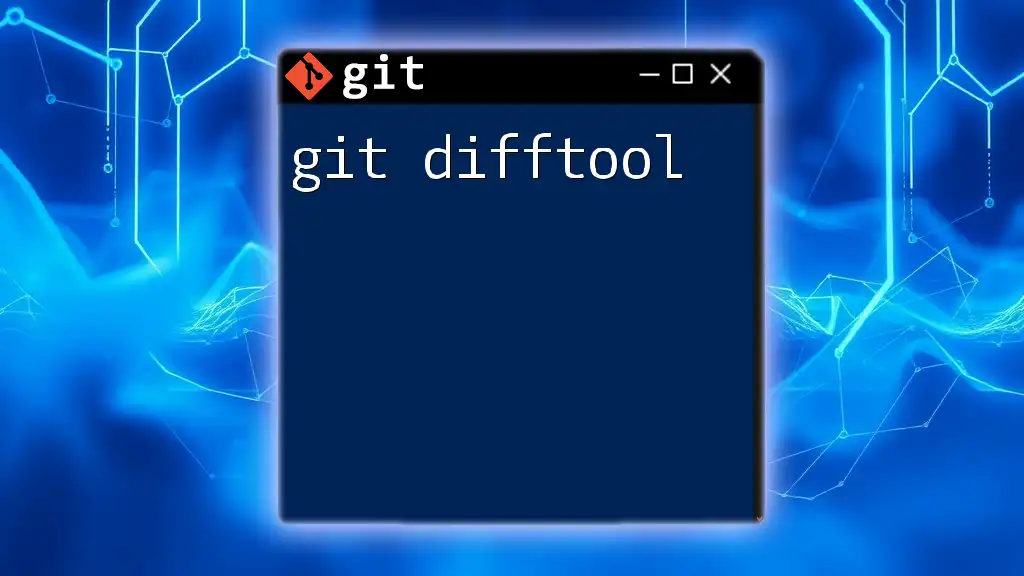
Conclusion
Mastering `git diff stash` is a beneficial skill that enhances your Git workflow. By incorporating this command into your development practices, you’re better equipped to manage and review your code changes efficiently. With practice, stashing and using `git diff` will become seamless aspects of your version control routine, allowing for improved productivity and organization in your projects.