The `git diff --cached` command allows you to view the differences between your staged changes and the last commit, providing a clear comparison of the modifications you've made before committing them.
git diff --cached
Understanding Git Staging Area
What is the Staging Area?
The staging area, or index, is a critical part of the Git workflow. It acts as a bridge between your working directory and the final commit history. When you modify files in a Git repository, those changes typically remain in your working directory until you explicitly add them to the staging area. At this point, you can choose which changes to include in your next commit, giving you a significant amount of control over your version history.
Why Do We Use the Staging Area?
Using the staging area allows you to curate your commits. You might make numerous changes while working on a feature, but you shouldn't necessarily commit every change. Staging lets you select exactly what you want to commit, ensuring that your commit history remains organized and meaningful. For example, if you are working on two related features simultaneously, you can stage and commit changes for one feature without including unfinished work from the other.

An Introduction to `git diff`
What is `git diff`?
The command `git diff` is essential for reviewing changes in your Git repository. This command shows the differences between various states, such as untracked files, staged changes, and those committed. It helps users visualize what has changed in their code, making it easier to review modifications before they become part of the project history.
Why Use `git diff` for Staged Changes?
Reviewing staged changes is vital for ensuring quality commits. When you run `git diff` on staged changes, you can spot any errors or unnecessary modifications before finalizing the commit. This practice can save time and effort in the future by helping you avoid issues that could arise from incorrectly committed changes.

Getting Started with `git diff` for Staged Changes
Basic Syntax of `git diff`
The general syntax of `git diff` is:
git diff [options] [<commit>]
Understanding this syntax is paramount, as it allows for flexibility in comparing various states within your repository.
Viewing Staged Changes
Running the Command
To view staged changes, you can use the command:
git diff --cached
Alternatively, you might encounter `git diff --staged`, as both commands serve the same purpose. After you've staged your changes using `git add <file>`, this command will display the differences for the files that are staged for the next commit.
Example Scenario
Suppose you're working on a project with a file named `feature.js`. After editing `feature.js`, you run:
git add feature.js
git diff --cached
The output will show the differences between the staged version of `feature.js` and its last committed state, allowing you to see exactly what will be committed.

Understanding Output of `git diff --cached`
Reading the Diff Output
When you execute `git diff --cached`, Git outputs the changes in a clear format. The lines prefixed with + represent new additions in your staged file, while - signifies deletions. Understanding this output format is essential for effective code review and error checking.
Analyzing Changes
The output can serve as a powerful review tool. Use it to verify that only the desired changes are being committed. By analyzing the additions and deletions line by line, you can catch mistakes or unnecessary edits before they go on record.

Combining `git diff` with Other Commands
Using `git status` with `git diff`
Before reviewing staged changes, you might want to know which files are staged and unstaged. The command:
git status
provides this overview, showing you the files that have been modified and those that are ready to be committed.
Using `git commit -v` to View Staged Changes
You can also incorporate knowledge of staged changes into your commit process. Running:
git commit -v
includes a diff of the changes that are being committed alongside your commit message editor. This feature provides immediate context, helping reinforce your understanding of what you are about to commit.
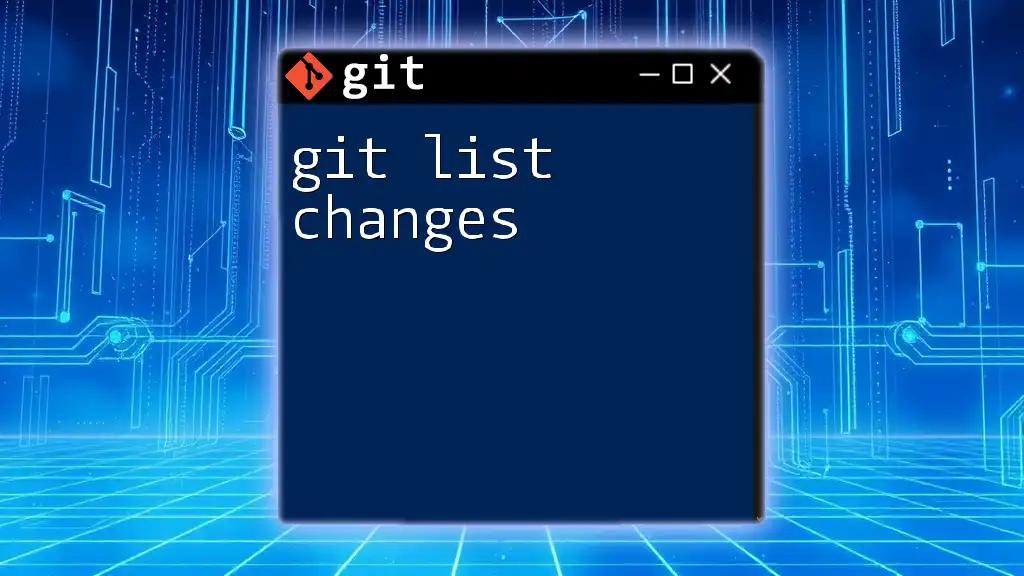
Best Practices for Using `git diff --cached`
Review Changes Before Committing
Make it a habit to run `git diff --cached` each time you prepare a commit. This practice ensures that your commit only includes the changes you intend to make. It avoids the hassle of fixing mistakes later on.
Keeping Your Commits Clean and Concise
Aim for small, focused commits that address particular features or fixes. Each commit message should be descriptive yet concise. Following this guideline helps maintain an organized project history.
Using GUIs for Visual Diff
Although command-line tools are powerful, many developers find GUI applications more intuitive for viewing diffs. Tools like GitKraken, Sourcetree, or GitHub Desktop offer visual representations of changes, assisting in easier code reviews.

Troubleshooting Common Issues
Why `git diff --cached` Might Not Show Expected Results
If you run `git diff --cached` and find unexpected results, consider the following common mistakes:
- You may not have staged any changes. Always confirm with `git status`.
- Ensure you're checking the right branch or commit.
Solutions to Fixing Errors in Your Staged Changes
If you realize you've staged the wrong changes, you can use:
git reset <file>
This command unstages the specified file, moving it back to the working directory. You can then modify or re-stage the file as necessary.

Conclusion
Reviewing your staged changes with `git diff staged changes` is a crucial part of a robust Git workflow. By understanding how to leverage this command effectively, you can improve the quality of your commits and keep your project history clean and organized. Embrace `git diff` as a part of your daily Git practice for a more efficient coding experience.
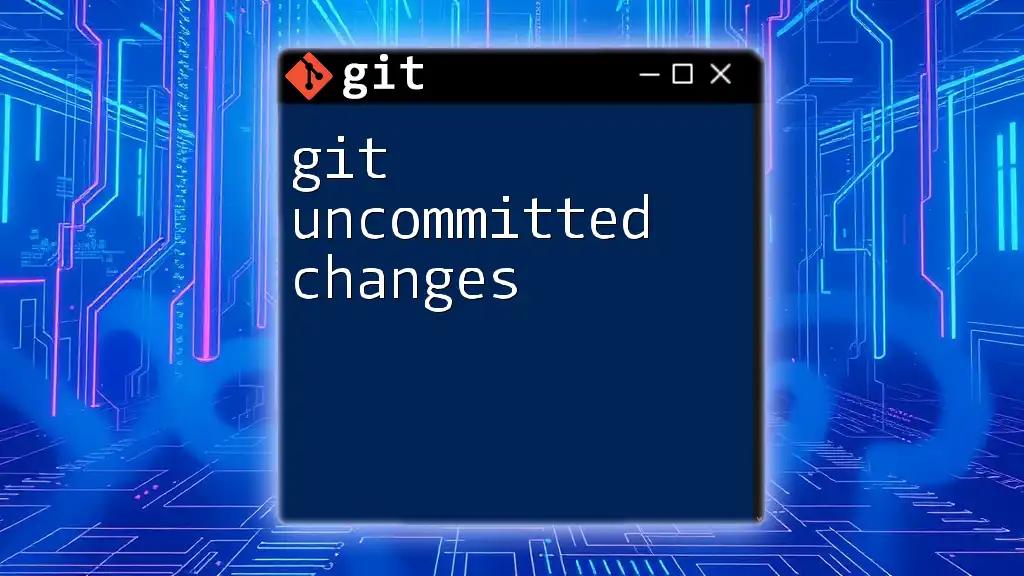
Additional Resources
As you continue to learn, consider exploring further reading on `git diff` and other advanced Git commands. Utilizing multiple resources can deepen your understanding and enhance your skills in version control.