To ignore changes in a tracked file using Git, you can use the `git checkout` command along with `--` followed by the file name to revert the file to its last committed state.
git checkout -- <file_name>
Understanding .gitignore
What is .gitignore?
The `.gitignore` file is a crucial component of Git's version control system, serving as a list of file patterns that Git should ignore when tracking changes or considering file additions. This effectively prevents unnecessary files—such as temporary, log, or backup files—from cluttering your repository and affecting collaboration.
Creating a .gitignore file
Creating a `.gitignore` file is a straightforward process. Simply create a text file named `.gitignore` in the root of your repository. Here’s an example of what a basic `.gitignore` file might look like:
# Ignore all logs
*.log
# Ignore temporary files
*.tmp
These entries tell Git to ignore all files with a `.log` extension and all `.tmp` files. This simplicity leads to a cleaner project, especially in collaborative environments.
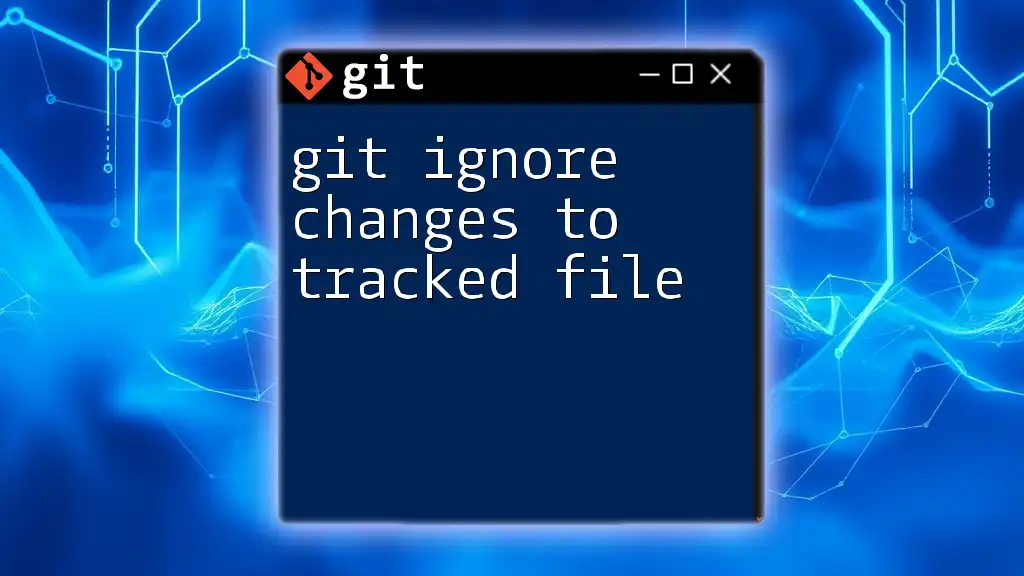
Ignoring Changes in Tracked Files
Using git update-index
Sometimes you may find it necessary to ignore changes to files that are already being tracked by Git. This is where the command `git update-index --assume-unchanged` comes into play. With this command, you can tell Git to temporarily ignore modifications to a specified tracked file.
Imagine you're working on a configuration file that you don't want to commit changes to, for example:
git update-index --assume-unchanged path/to/file.txt
Once you run this command, Git will treat the file as if it hasn't changed, allowing you to leave your modifications locally without affecting the main repository. This can be particularly useful in environments where your local configuration differs from the shared setup.
Reversing Ignored Changes
If you decide later that you want Git to start tracking changes to the file again, you can simply reverse the previous command using `--no-assume-unchanged`:
git update-index --no-assume-unchanged path/to/file.txt
This flexibility offers you the ability to manage local changes effectively, without compromising the integrity of the shared project files.
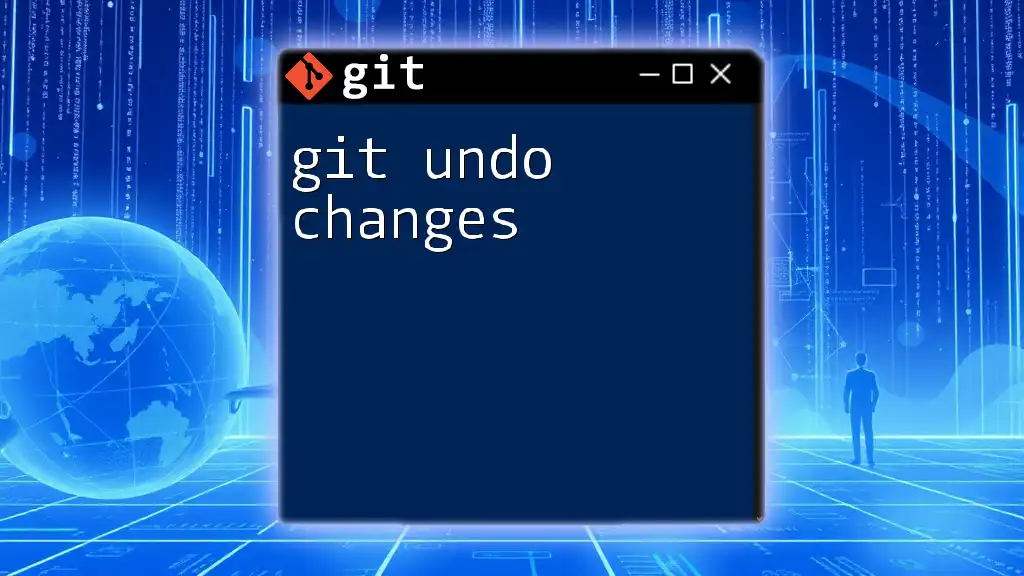
Ignoring Changes in a Temporary Branch
Creating a Temporary Branch
Another approach to handle local changes that you want to ignore is to create a temporary branch. This method is particularly beneficial if you need to keep your changes separate without cluttering the main branch. To do this, simply create and switch to a new branch using the following command:
git checkout -b temp-branch
By working in this temporary branch, you can commit changes without worrying about impacting the main branch. This makes it easy to experiment or modify code while keeping your main development line clean.
Committing Ignored Changes
While in the temporary branch, you can commit your changes as needed. This strategy allows you to test different ideas or configurations while keeping the primary workflow intact. If you decide any of the changes are beneficial, you can later merge them back into the main branch or simply discard them when you’re finished.
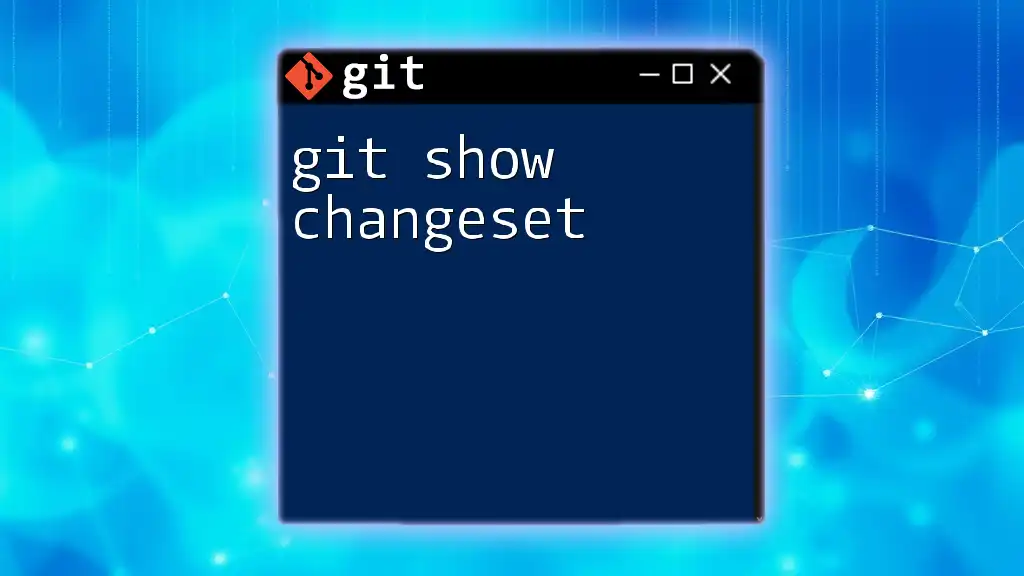
Ignoring Specific Changes
Using .git/info/exclude
If you don't want to create a `.gitignore` file, you can utilize the `.git/info/exclude` file. This alternative works similarly but applies only to your local repository (it's not shared with others).
To exclude files locally, you can add them to the `.git/info/exclude` file. For example:
# Exclude all files with a specific extension
*.bak
This is particularly useful for files that are specific to your local development environment and that you do not wish to share with your team.
Working with Local Changes
Setting up local ignored changes also helps manage custom configuration files. For example, if you're using a local configuration that differs significantly from the shared configuration, you can choose to ignore those local changes. This allows everyone in your team to maintain their unique settings while still collaborating effectively on the codebase.
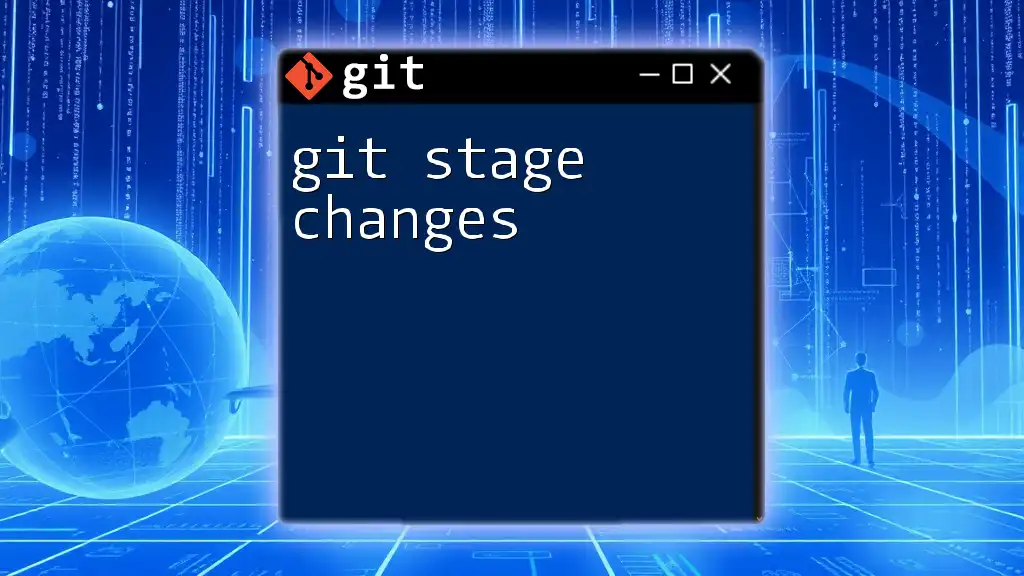
Best Practices for Ignoring Changes
Common Patterns in .gitignore
When creating a `.gitignore` file, it's helpful to adopt common patterns that many developers agree on. Some widely recognized entries include:
- Build artifacts (e.g., `dist/`, `build/`)
- System files or IDE configurations (e.g., `.idea/`, `.vscode/`)
- Dependency files (e.g., `node_modules/`)
By keeping your `.gitignore` organized, you facilitate easier collaboration and minimize the chances of committing unwanted files to the repository.
Establishing Team Guidelines
Creating a team-wide `.gitignore` can prevent confusion. Establish guidelines collaboratively, ensuring that everyone is on the same page regarding what files should be ignored, and why. This communication fosters a more transparent workflow and can significantly enhance teamwork.
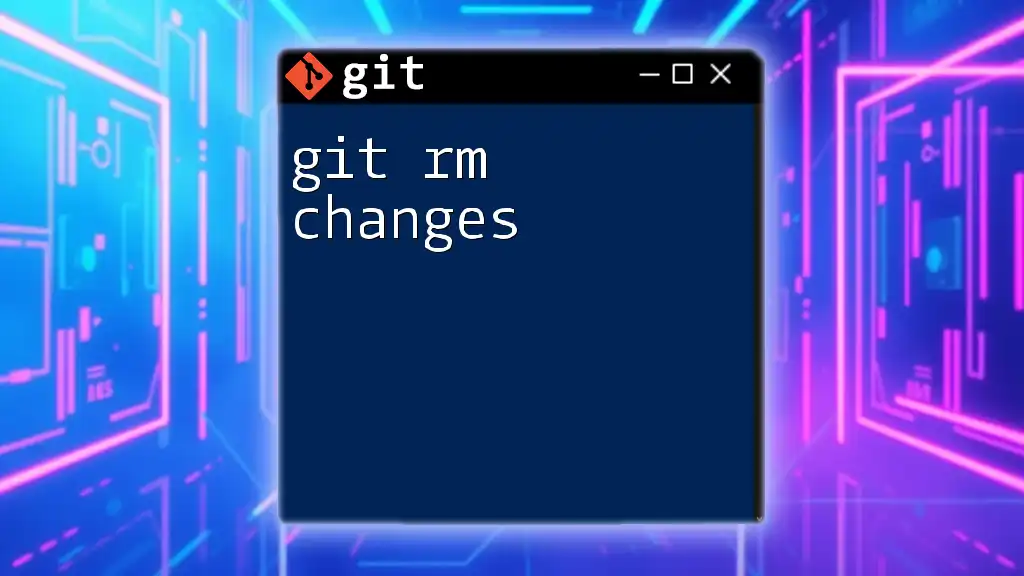
Conclusion
Effectively utilizing git ignore changes is essential in managing your development environment. By understanding how to implement `.gitignore`, use the `git update-index` command, create temporary branches, and adopt best practices, you can maintain a clean and efficient codebase. These practices not only streamline your own workflow but also contribute to a more organized collaboration within your team.
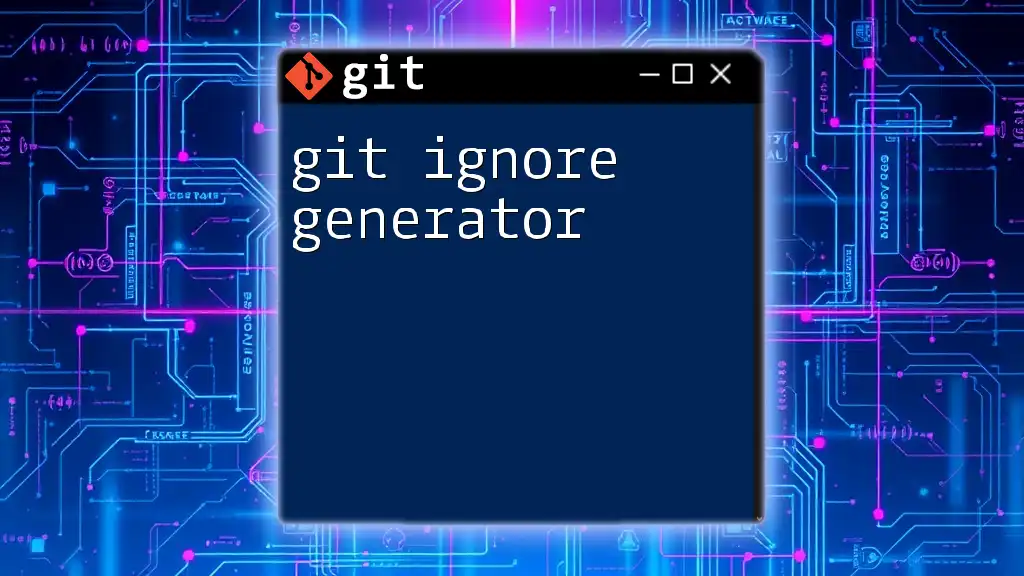
Additional Resources
- Official Git documentation for deeper reading.
- Recommended tutorials and tools for mastering Git.
- Community forums for Git users looking to enhance their skills.
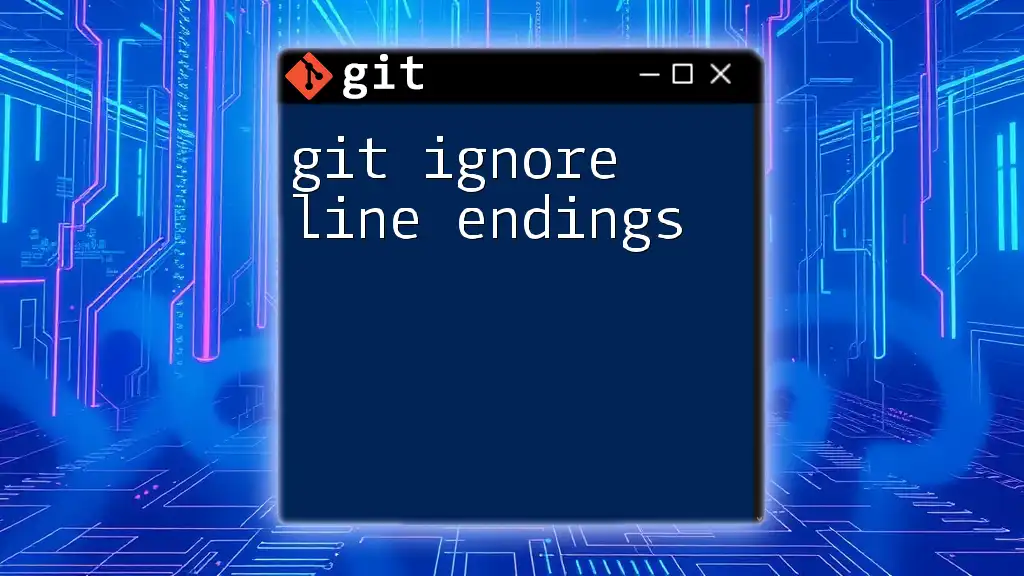
FAQ
What happens if I push a change to a file I marked as ignored?
If you push a change to a file you marked as ignored with `--assume-unchanged`, the change will not be affected. However, the local file will still be tracked, which may cause discrepancies if you switch branches.
Can I ignore changes to a file after it has been committed?
Yes, you can ignore changes to a file after it has been committed by using the `--assume-unchanged` command.
What are some practical scenarios for using `--assume-unchanged`?
This command is particularly useful for local development settings, configuration files for development environments, or any scenarios where you need to maintain flexibility on local changes without pushing them to the remote repository.