The `.gitignore` file tells Git which files or directories to ignore in a project, preventing them from being tracked in version control.
Here's a code snippet to create a `.gitignore` file:
echo "node_modules/" >> .gitignore
What is Git Ignore?
The `.gitignore` file is an essential part of any Git repository. It is a text file that tells Git which files or directories to ignore in a project, meaning they won't be tracked or committed. This is particularly useful for keeping your repository clean and ensuring that only the necessary files are included in version control.
Importance of Excluding Files and Directories from Git Tracking
By employing a `.gitignore`, you can prevent unneeded files from cluttering your Git history. This is crucial for maintaining clarity and organization as your project evolves. Files that you might not want to include often include temporary files, log files, or configuration files that may contain sensitive information.
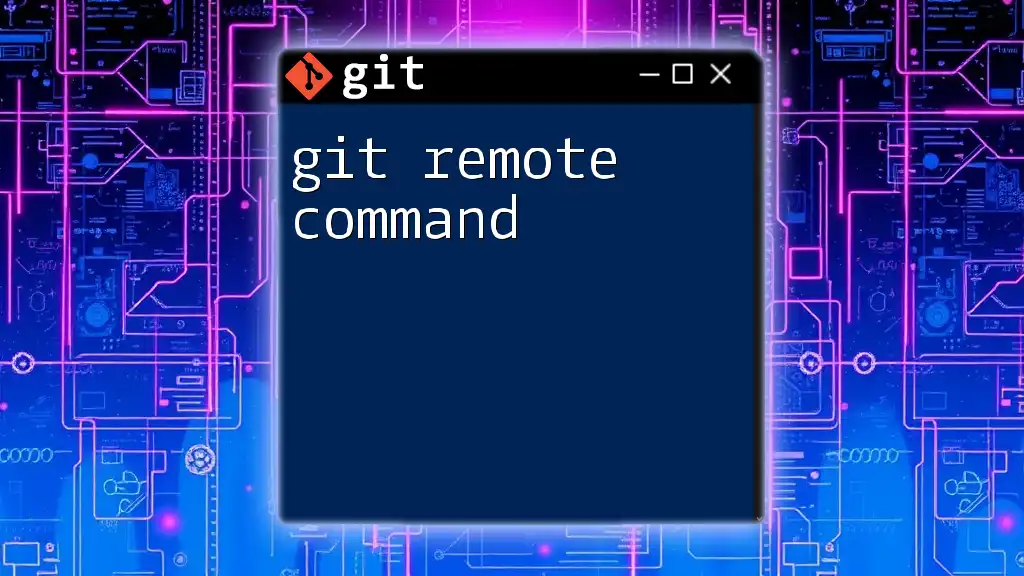
Benefits of Using Git Ignore
The benefits of using a `.gitignore` file are multi-faceted.
-
Improves repository cleanliness: A well-structured `.gitignore` contributes to a tidy repository, enabling easier navigation and understanding of the project.
-
Prevents sensitive data from being uploaded: Keeping sensitive files out of your repository mitigates the risk of exposing API keys, credentials, or personal information.
-
Reduces clutter in version control history: By filtering out irrelevant files, your commit history becomes more concise and focused on the essential changes.
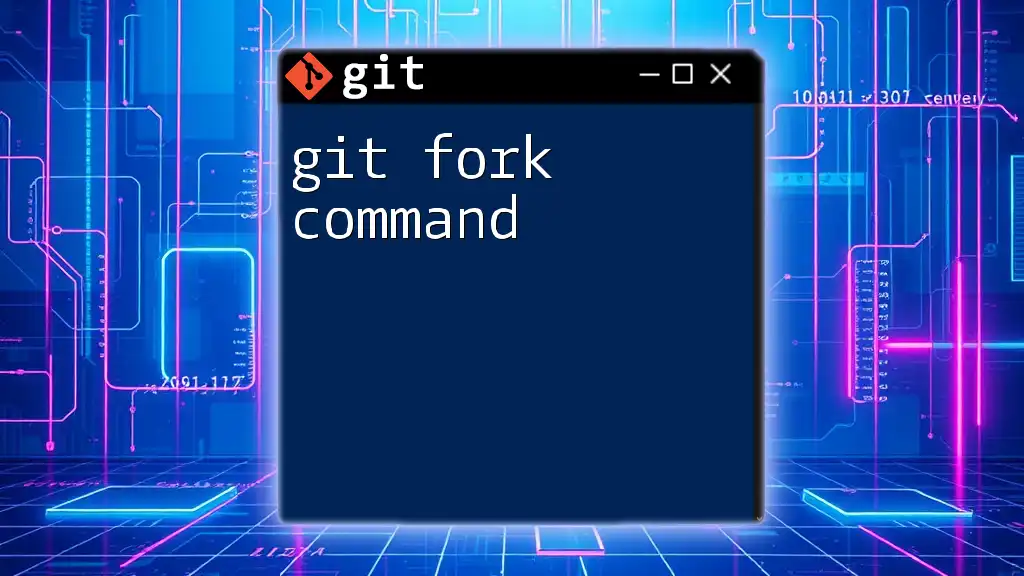
Understanding the Structure of the .gitignore File
Basic Syntax and Format
Creating a `.gitignore` file is straightforward. You just need to create a text file named `.gitignore` in your repository root. The syntax is simple: list the files and directories you wish to ignore, one entry per line.
For example:
# Ignore system files
.DS_Store
Thumbs.db
# Ignore log files
*.log
# Ignore build directories
/build/
In this snippet, `.DS_Store` and `Thumbs.db` are ignored system files generated by macOS and Windows, while all files with the `.log` extension and the entire `build` directory are excluded from tracking.
Wildcards and Patterns
Using Wildcards
Wildcards are powerful tools within the `.gitignore` file that enhance your ability to specify ignored elements. Various symbols are used, such as `*` (matches any number of characters) and `?` (matches a single character).
For example:
# Ignore all .log files in any directory
*.log
This pattern tells Git to ignore all files with the `.log` extension, regardless of their location in the project.
Specific vs. General Patterns
You can differentiate between specific file types and general patterns based on your project's needs. For instance, to ignore any file with a `.tmp` extension anywhere in the repository, you would write:
# Ignore any .tmp file anywhere in the repository
*.tmp
Conversely, if you wanted to target a specific file, you might include its path in the `.gitignore`, like this:
# Ignore specific configuration file
config/settings.ini
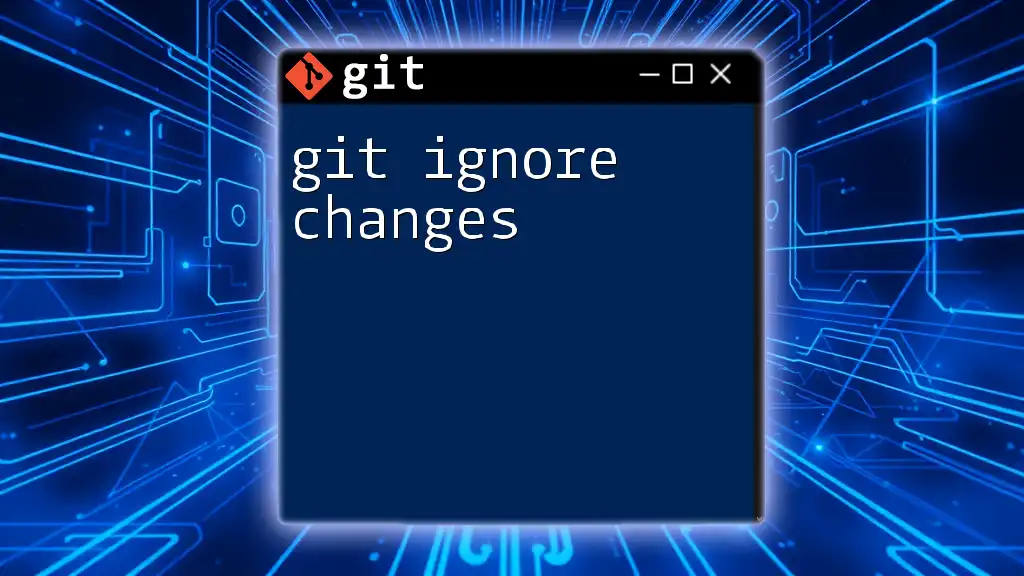
Common Use Cases for .gitignore
Ignoring Specific File Types
There are various scenarios where ignoring specific file types is beneficial. Common examples include ignoring compiled files that are generated during the build process. For instance, if you're working with Python, you may want to ignore the bytecode files:
# Ignore bytecode files
*.pyc
Similarly, for Java, you would ignore `.class` files generated during compilation:
# Ignore compiled Java files
*.class
Ignoring System Files
System-generated files can clutter your repository and provide no value to your project. Examples like `.DS_Store` for macOS and `Thumbs.db` for Windows should be easily ignored to prevent unintended commits:
# Ignore macOS and Windows system files
.DS_Store
Thumbs.db
Project-Specific Files
Dependencies often create their own directories that should also be ignored. For instance, in Node.js projects, the `node_modules` directory can be substantial, and tracking it is unnecessary:
# Ignore npm packages
node_modules/
If your project uses environment variables stored in configuration files, these should also be excluded from version control:
# Ignore environment configuration files
.env
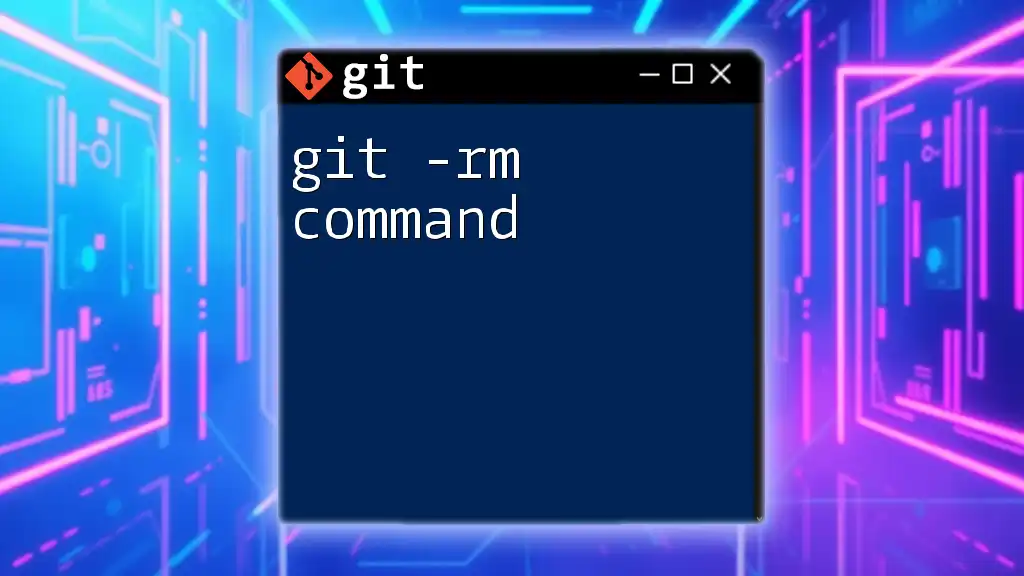
Best Practices for Using .gitignore
Keeping Your .gitignore File Organized
An organized `.gitignore` file is easier to manage and maintain. Group similar rules together and use comments to explain the reasons for ignoring certain files. This practice enhances clarity both for you and for any collaborators involved in the project.
# Ignore log files
*.log
# Ignore compiled binaries
*.exe
*.out
When to Add Files to .gitignore
Deciding when to add files to your `.gitignore` can make a significant difference in your workflow. Preemptively ignoring files or directories before they are committed is a good habit, but it’s also perfectly acceptable to modify your `.gitignore` after a file has been tracked—just remember it will only take effect on future commits.
Versioning the .gitignore File
Tracking your `.gitignore` file is as important as tracking your source code. Ensure that any updates or modifications to this file are committed so that your team can benefit from a consistent ignoring strategy.
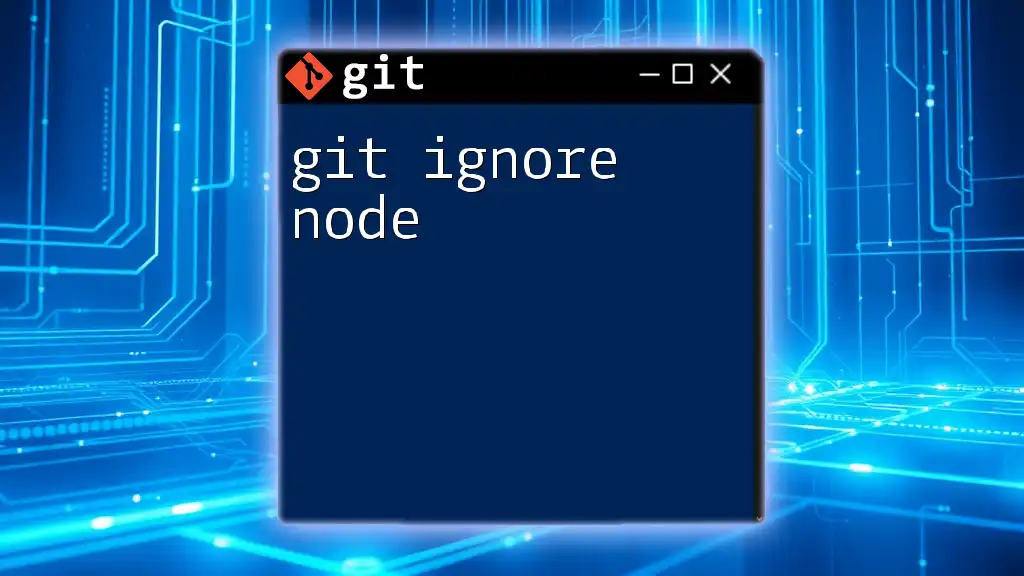
Modifying and Updating .gitignore
Adding New Entries
As your project evolves, you may need to add new entries to your `.gitignore`. For example, if you discover additional temporary files or directories that should not be tracked, simply add them in, following the standard syntax.
Removing Previously Tracked Files
In cases where files have already been committed but you wish to exclude them moving forward, you will need to untrack them. This can be accomplished using the following command:
git rm --cached <file>
Replace `<file>` with the name of the file you want to stop tracking. This command removes the file from the staging area while preserving it on your disk.
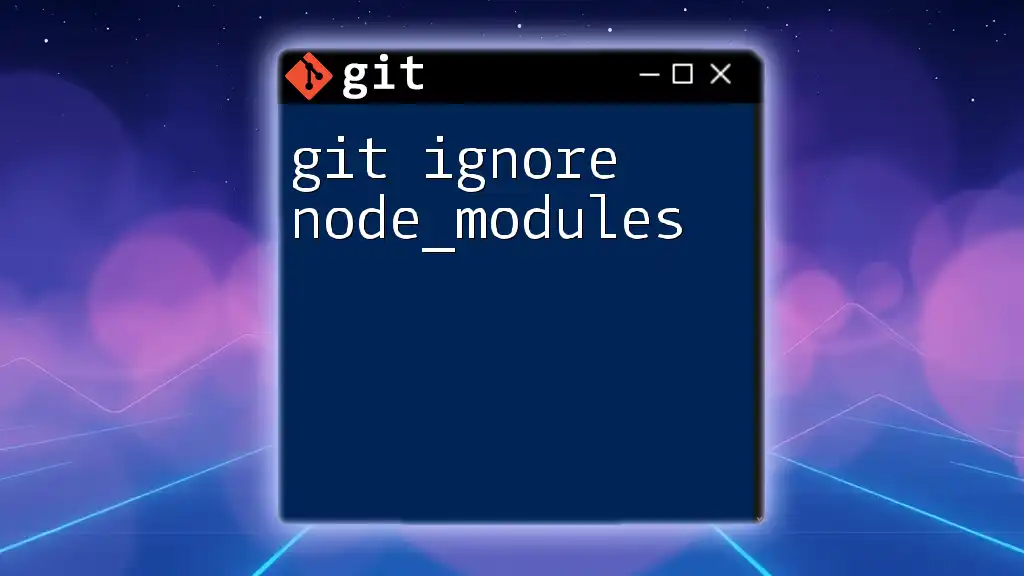
Conclusion
In summary, the git ignore command through the use of a `.gitignore` file is invaluable in keeping your project clean, organized, and secure. By adhering to best practices and employing effective patterns, you can ensure that your Git repository remains focused on what truly matters. It's always worth taking a moment to implement a `.gitignore` file that suits your project needs, safeguarding both your workflow and sensitive information.
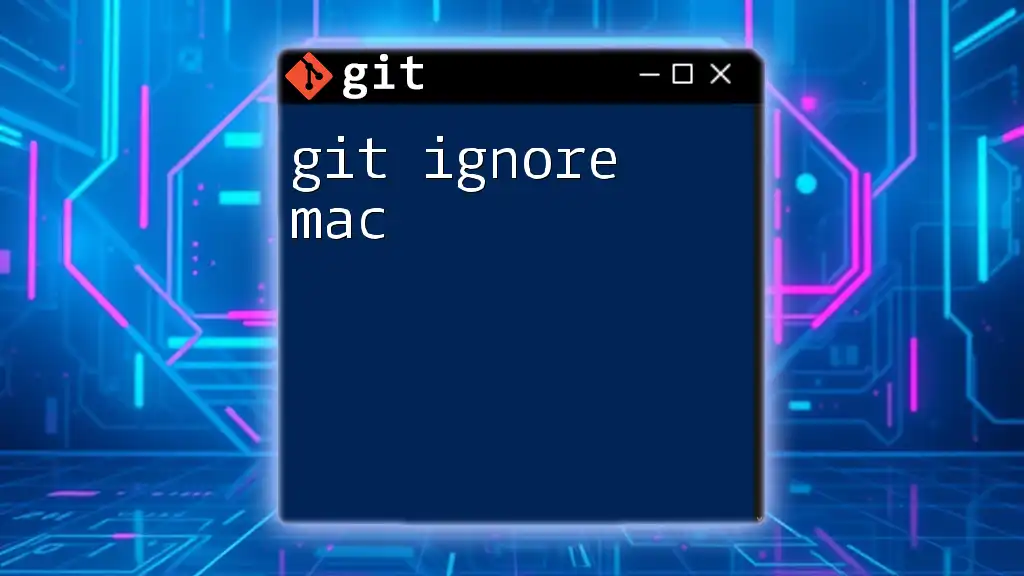
Further Resources
For more detailed insights on the `git ignore command`, consider checking official documentation or engaging with community forums to share experiences and best practices. Learning from others can provide unique perspectives and further enhance your understanding of Git.