The `git delete` command is not a direct command in Git; instead, you use `git rm` to remove files from the staging area and working directory, followed by `git commit` to finalize the changes.
Here’s how you can do it:
git rm <file_name>
git commit -m "Remove <file_name>"
Understanding the Git Delete Command
What is the Delete Command?
The git delete command refers to the various ways in which you can remove files, branches, and commits within a Git repository. Understanding these deletion commands is critical for maintaining a clean and efficient version control history.
Different contexts for deletion in Git
Deletion in Git can occur in multiple contexts: files in the working directory, branches, and commits. Each context has its own specific commands and best practices.
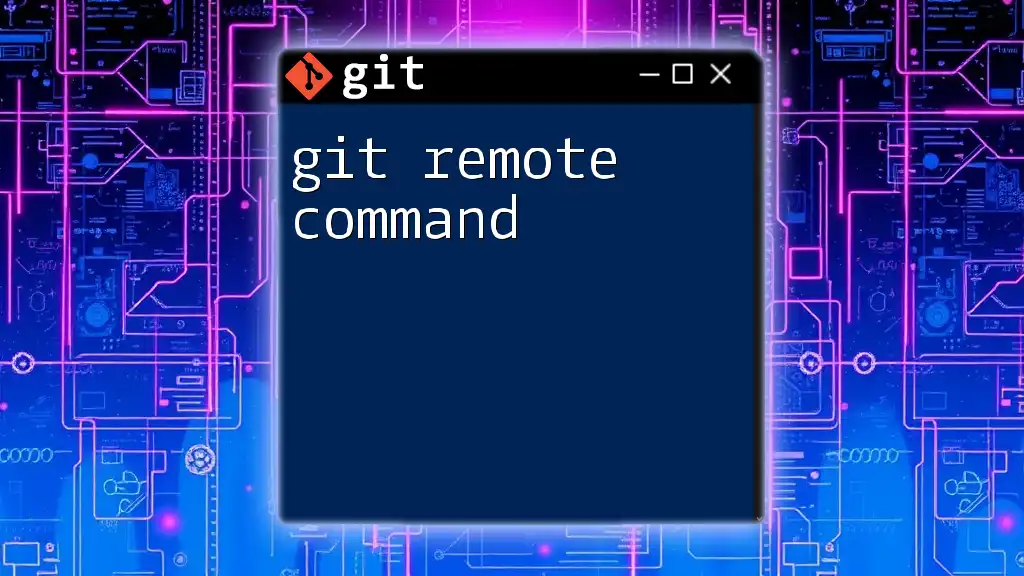
Deleting Files from the Working Directory
Using the `git rm` Command
The `git rm` command is specifically designed to remove tracked files from your Git repository. This command not only deletes the file from your working directory but also stages the removal for the next commit.
Basic syntax
git rm <file>
Examples
Example 1: Deleting a single file
git rm myfile.txt
In this command, `myfile.txt` is removed from both the working directory and the staging area, preparing it for the next commit. This is useful when you no longer need a particular file in your project.
Example 2: Deleting multiple files
git rm file1.txt file2.txt
You can also specify multiple files in a single command to efficiently delete them all at once. This can save time and reduce the number of commands you need to execute.
Safeguarding your changes
If you want to keep a file in your working directory but remove it from staging, you can use the `--cached` option:
git rm --cached myfile.txt
This approach is particularly useful if you accidentally added a file to staging that you wish to remain untouched in your local environment.
Force Deleting Files
Occasionally, you may need to override Git's protection when deleting files that are not tracked. The `-f` option forces the deletion:
git rm -f untracked-file.txt
Use this command with caution, as it can lead to loss of important data. This option is typically required when you are sure that the file should not be kept.
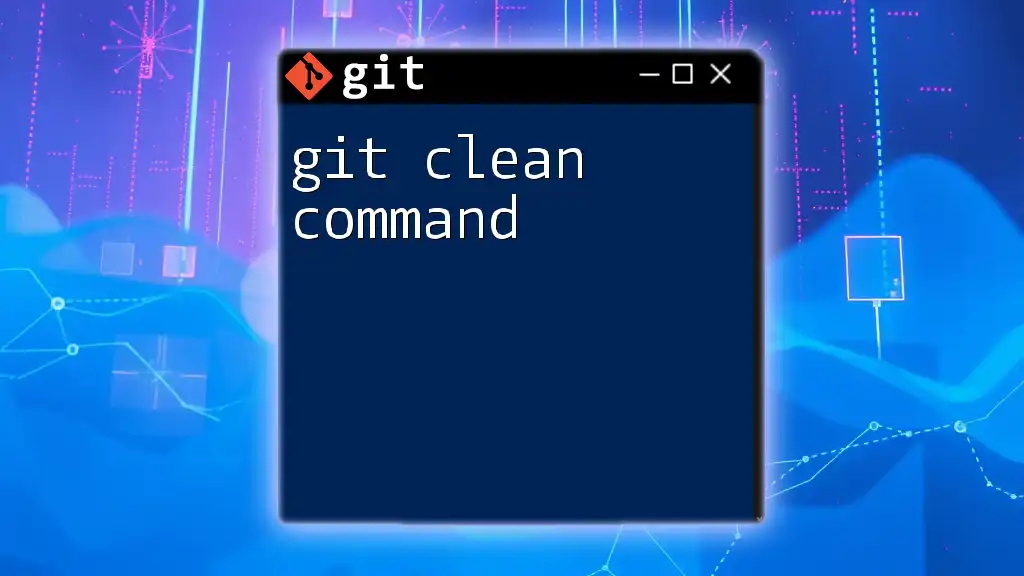
Deleting Branches
Understanding Branch Deletion
In Git, branches allow developers to work on features in isolation. However, once a feature is complete or if a branch is no longer needed, you may want to delete it.
Deleting Local Branches
To delete a local branch safely, you can use the `git branch -d` command:
git branch -d <branch-name>
Example:
git branch -d feature/old-feature
This command deletes the specified branch only if it has already been merged into another branch, ensuring that no work is lost accidentally.
Forcibly Deleting a Branch
If you are sure that you want to delete a branch regardless of its merge status, you can use the `-D` option:
git branch -D <branch-name>
Example:
git branch -D feature/unwanted-feature
Using `-D` can result in the loss of unmerged changes, so it should be used judiciously, especially in collaborative workflows where others may depend on that branch.
Deleting Remote Branches
To delete a branch from a remote repository, you can use the `git push` command with the `--delete` option:
git push origin --delete <branch-name>
Example:
git push origin --delete feature/old-feature
This command informs the remote repository to remove the specified branch. Be aware that deleting a remote branch can impact your team's workflow, as others may still be relying on it.
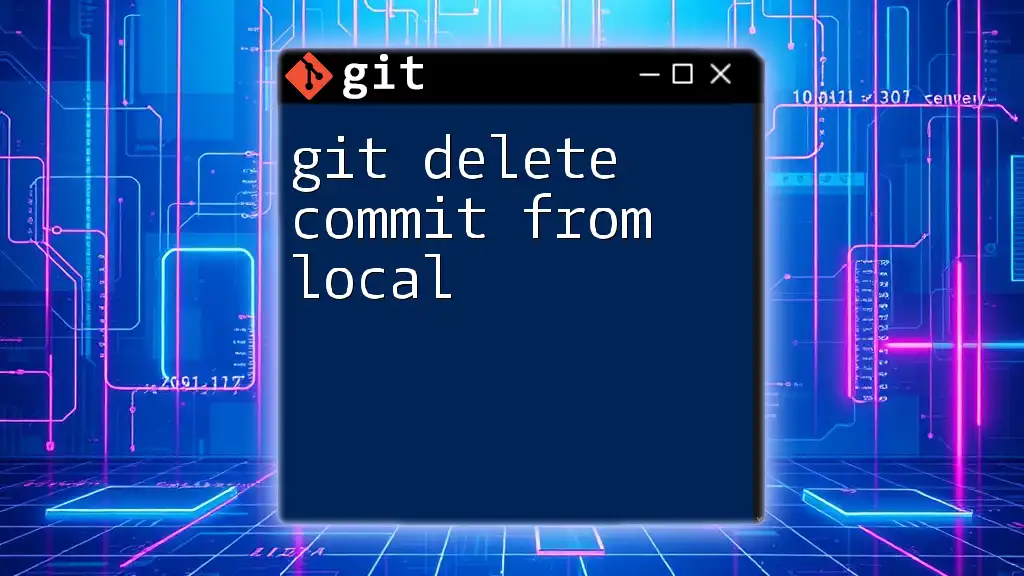
Deleting Commits
Understanding Commit Deletion
Deleting commits is a more complex operation, as it involves rewinding the history of your project. This action can have significant implications, particularly in collaborative environments. Understanding how to do this safely is crucial.
Using `git reset`
The `git reset` command is used to remove commits and can be done in different ways, depending on how much you want to undo.
Soft Reset
To undo a commit while keeping the changes staged, use:
git reset --soft HEAD~1
This resets to the previous commit but leaves your changes in the staging area, enabling you to make adjustments before recommitting.
Hard Reset
A more drastic option is a hard reset:
git reset --hard HEAD~1
Warning: This command will discard all changes in the working directory and staging area that were made in the last commit. Use this option with extreme caution, as it can lead to loss of important data.
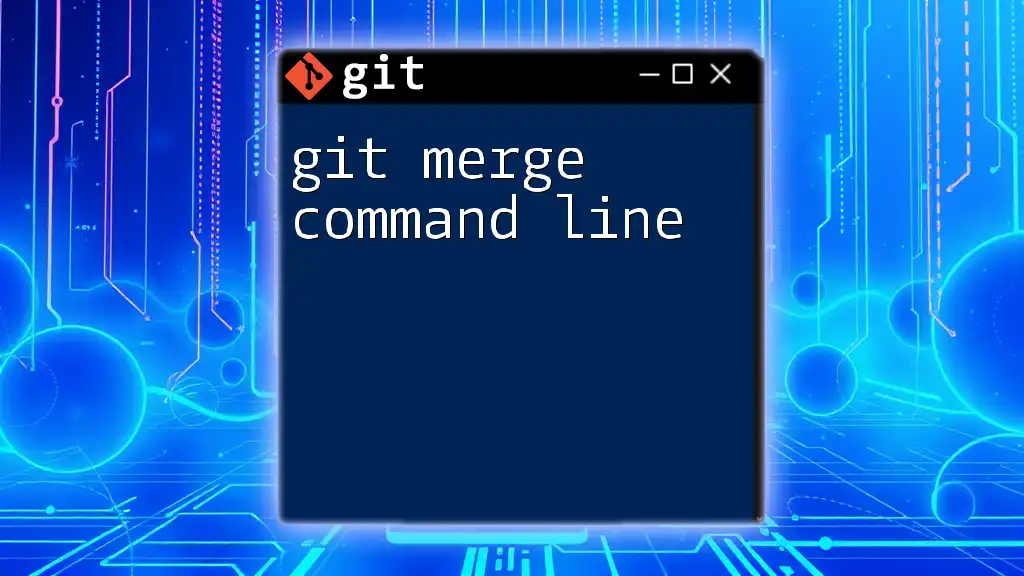
Best Practices When Using Delete Commands
-
Always Backup: Before using any delete commands, particularly on branches or commits, it’s advisable to back up your current state. This way, you can always revert if mistakes occur.
-
Considerations for Collaboration: When working with teams, ensure that everyone is aware of branch deletions and commit resets to avoid confusion and data loss. Communicate significant changes in the repository status.
-
Using Tags Before Deleting: Tagging important commits before deleting branches is a practical way to preserve states. You can always return to a tagged commit if something goes amiss.
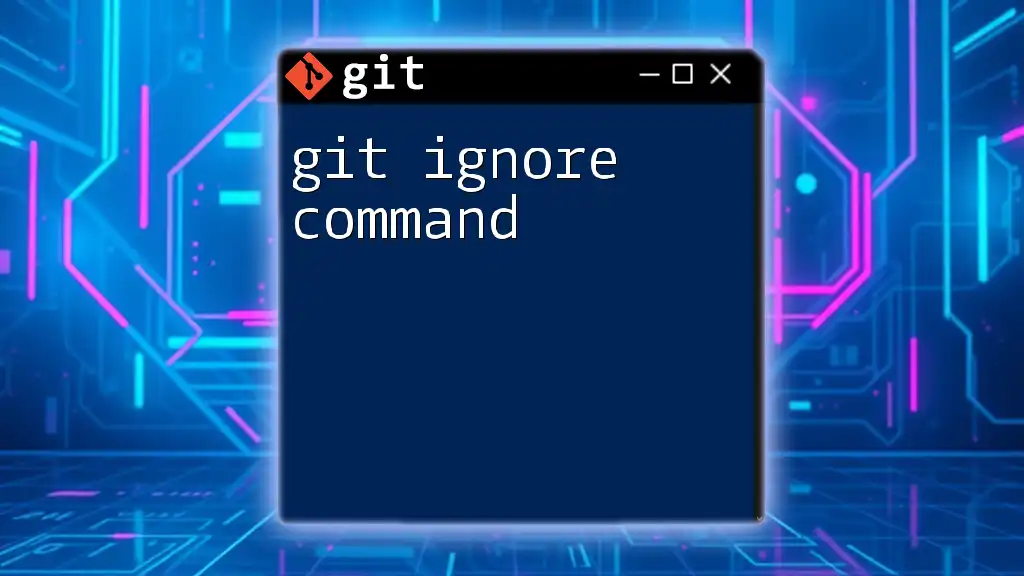
Conclusion
The git delete command plays a crucial role in managing your repository effectively. Understanding how and when to use it ensures that you maintain a clean and efficient workflow. Always practice these commands in a controlled environment before applying them to critical projects, and remember to communicate with your team to avoid unintended disruptions in your collaborative efforts.
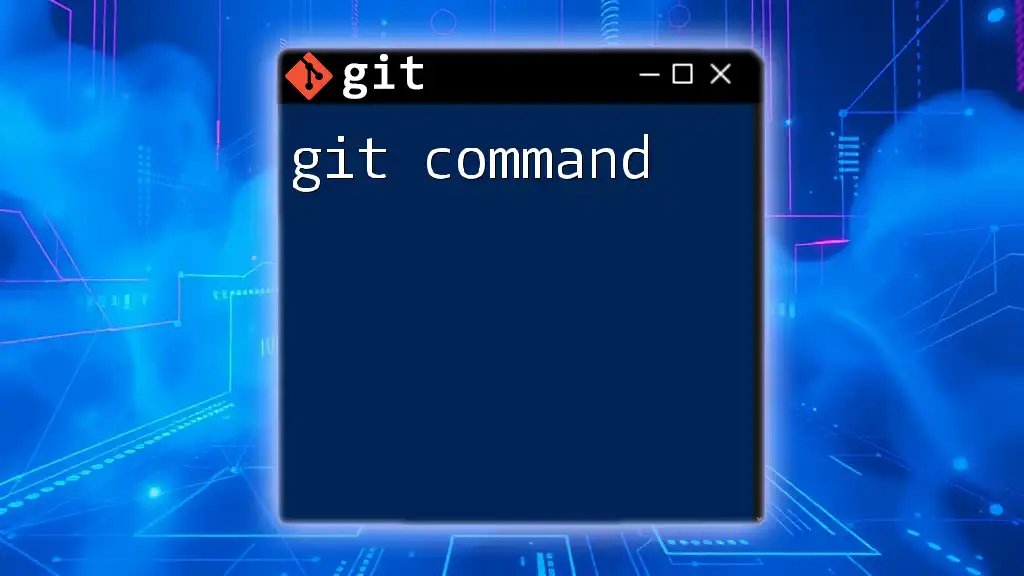
Additional Resources
For further reading and deeper understanding, consider exploring the official Git documentation, which offers comprehensive insights into various Git functionalities. Additionally, numerous online tutorials and guides can enhance your Git proficiency and help you master these commands.