To delete a local branch in Git, you can use the following command, ensuring you aren't currently on the branch you wish to delete:
git branch -d branch_name
Replace `branch_name` with the name of the branch you want to delete.
Understanding Branches in Git
What is a Git Branch?
A Git branch is essentially a separate line of development in your project. By creating branches, developers can work on discrete features, bug fixes, or experiments without affecting the main codebase. This allows for parallel development and facilitates collaboration amongst multiple contributors. When a feature is complete and tested, it can be merged back into the main branch, ensuring that the main code remains stable.
The Importance of Deleting Local Branches
Deleting unused local branches is critical to maintaining a clean and organized repository. By managing your branches effectively, you reduce clutter, making it easier to navigate your project. Additionally, regular pruning of branches can prevent confusion during collaboration, as team members are less likely to encounter outdated branches that may mislead them about the project's status.
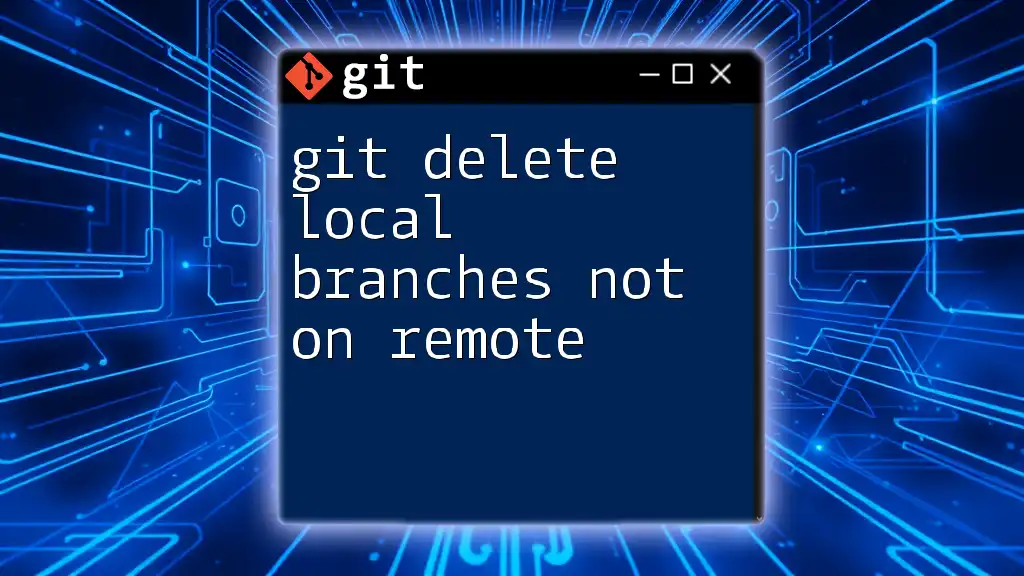
Types of Local Branch Deletion
Understanding Local vs. Remote Branches
It's essential to know the difference between local and remote branches. Local branches exist on your machine and are specific to your local instance of the repository. In contrast, remote branches belong to a shared repository—like those on platforms such as GitHub or GitLab. It's important to assess whether you need to delete a local branch based on its purpose and whether it's already pushed to a remote branch.
Reasons to Delete Local Branches
There are various reasons for deleting local branches:
- Completed Feature Branches: Once a feature has been merged into the main branch, the feature branch can often be safely removed.
- Merged Branches: After merging a bug fix or a feature, it’s good practice to delete the source branch.
- Experimental Changes: Sometimes, branches are created for experiments that do not pan out, making them obsolete.
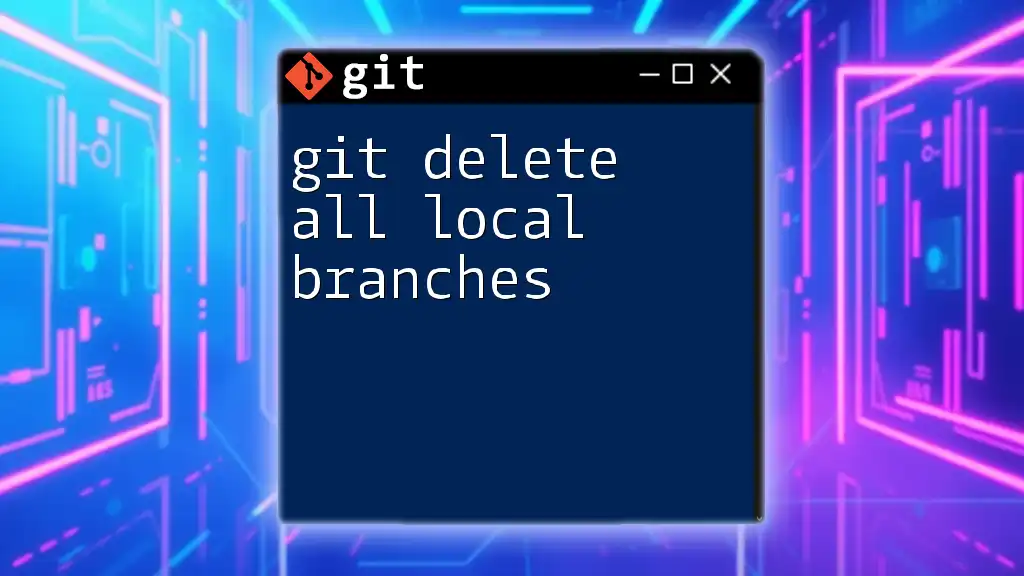
Prerequisites for Deleting Local Branches
Basic Git Commands to Know
Before you start deleting branches, it’s crucial to understand some fundamental Git commands. Familiarize yourself with:
- `git status`: Shows the current state of your working directory and staging area.
- `git branch`: Lists all local branches in your repository.
- `git checkout`: Switches to a different branch in your repository.
Knowing these commands will help guide you in safely deleting local branches without unintended consequences.
Checking Out the Correct Branch
Before deleting any branch, ensure that you are currently on a safe branch—typically the main branch or another branch that is not being deleted. Switching to the safe branch protects you from accidentally deleting the current branch you are working on.
To switch to the main branch, use the following command:
git checkout main
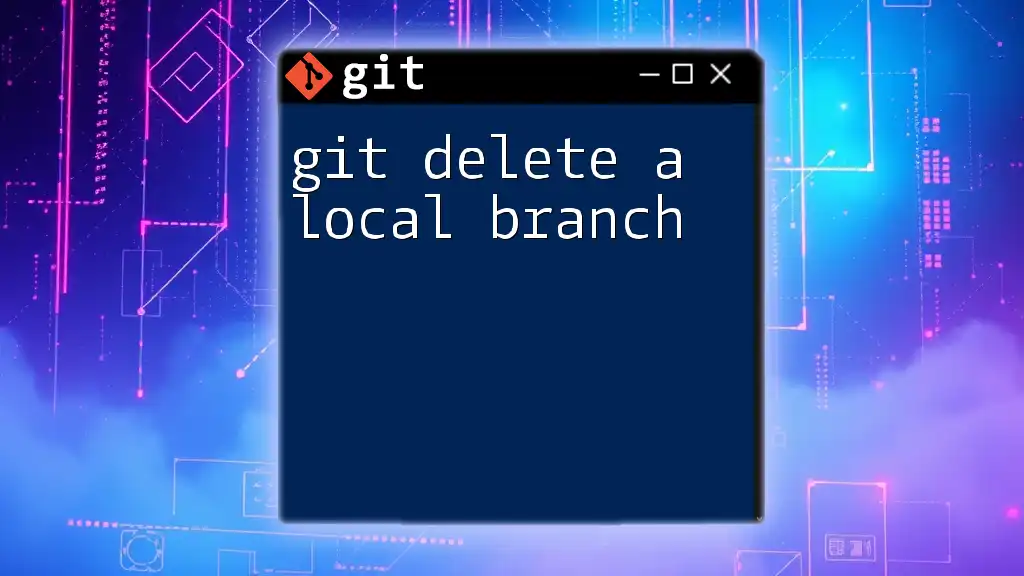
How to Delete Local Branches
Deleting a Local Branch Safely
When you are ready to delete a local branch that has been fully integrated with the main branch, you can use the command:
git branch -d <branch-name>
The `-d` flag stands for "delete," and this command ensures you can only delete branches that have been merged. For example, if you want to delete a branch named `feature-xyz`, you would run:
git branch -d feature-xyz
This command is safe because it prevents the deletion of unmerged branches, alerting you if you try to delete a branch that hasn’t been merged yet.
Forcing Deletion of Local Branches
In cases where you need to delete a branch that hasn’t been merged (for example, if the branch is no longer relevant), you can forcefully remove it using the `-D` flag:
git branch -D <branch-name>
This command bypasses the safety checks and deletes the branch regardless of its merge status. For example:
git branch -D bugfix-123
Use this option with caution—once deleted, restoring that branch can be complex.
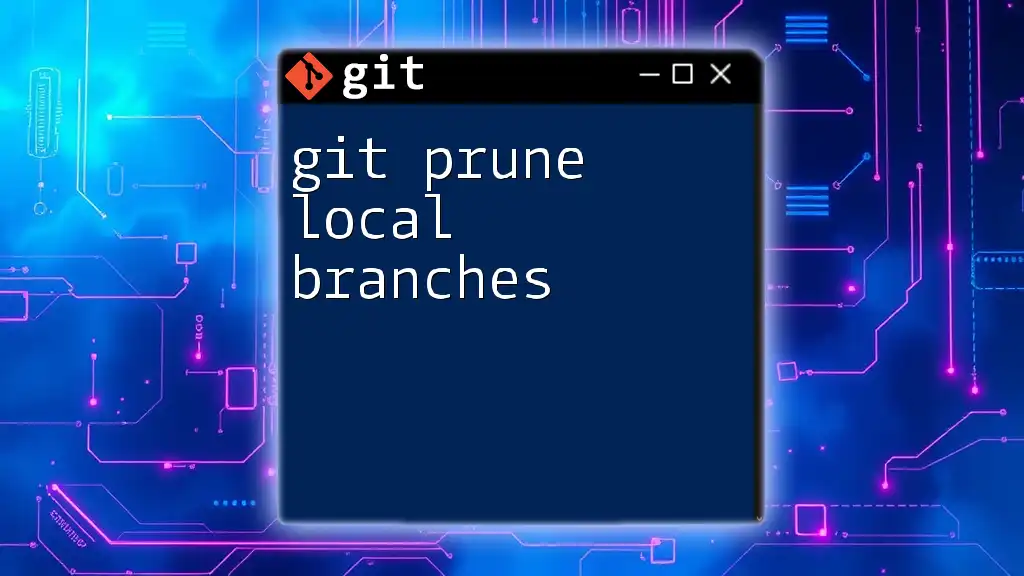
Confirming Local Branch Deletion
Verifying Branch Deletion
After executing the delete command, it’s crucial to confirm that the branch has been removed. You can check the status of your branches using:
git branch
This command lists all remaining local branches in your repository. If the deletion was successful, the deleted branch will no longer appear in this list.
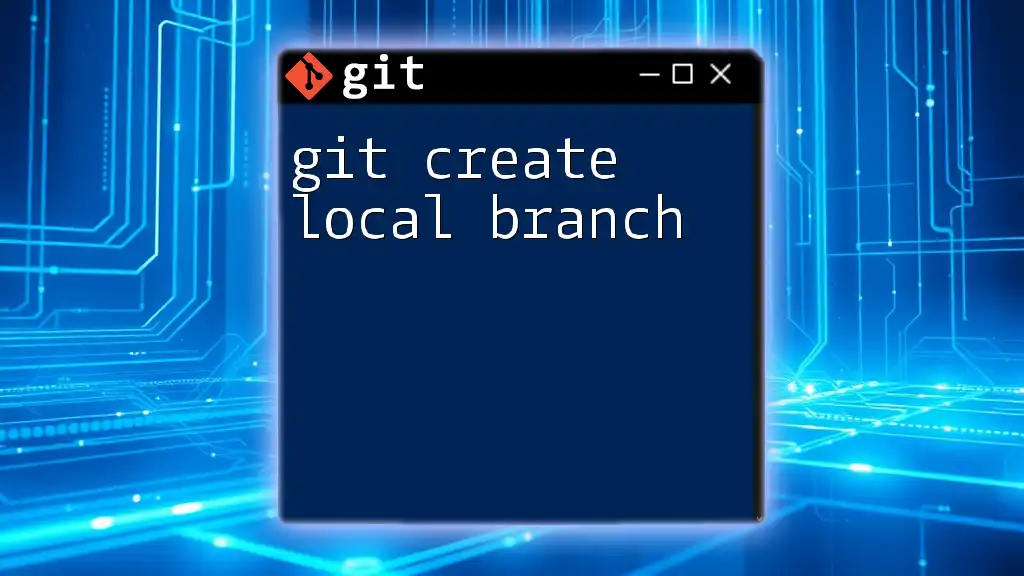
Troubleshooting Common Issues
Error: Branch Deletion Warning
If you encounter a warning when trying to delete a branch, it usually indicates that the branch has unmerged changes. This is a safety measure to ensure you don’t accidentally lose work. To resolve this, assess whether you need the changes from that branch. If you decide to proceed with deletion, you may need to use the force option discussed above.
Undoing Branch Deletion
If you accidentally delete a branch that you need, don’t panic! You can often restore it using `git reflog`, which logs all actions that change the repository head. You can find the commit ID where the branch was last pointing and recreate the branch as follows:
git checkout -b <branch-name> <commit-id>
Replace `<branch-name>` with the name of the deleted branch and `<commit-id>` with the appropriate commit ID found in the reflog.
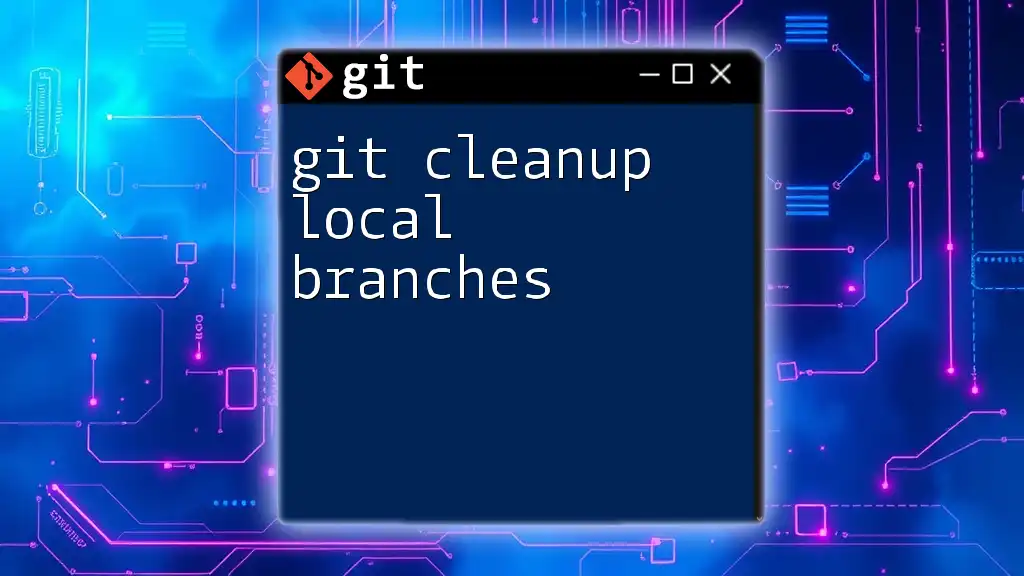
Best Practices for Branch Management
Tips for Clean Branch Management
To maintain a neat repository, consider these best practices:
- Adopt a Naming Convention: Use descriptive branch names, incorporating relevant ticket numbers or feature names.
- Regular Cleanup: Make a habit of reviewing and deleting branches that are no longer needed after merges.
Utilizing Branching Strategies
Employing established branching strategies can significantly enhance your workflow. For example, the Git Flow strategy emphasizes feature and release branches, whereas GitHub Flow focuses more on simplicity and uses a single branch with short-lived feature branches. Choose a strategy that suits the needs of your project and team dynamics.
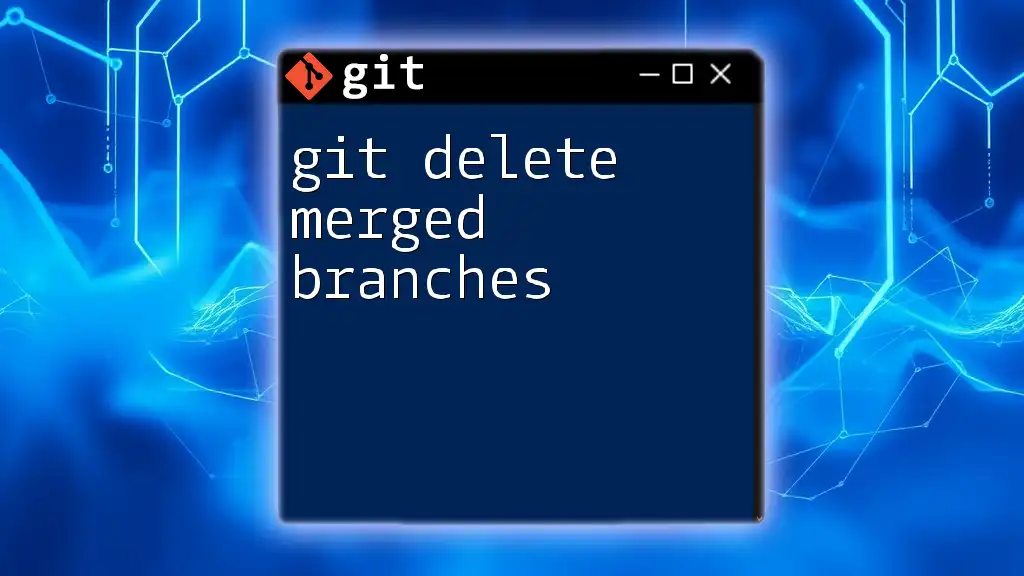
Conclusion
In summary, managing your branches in Git—including the process of deleting local branches—is an integral part of maintaining a clean and efficient workflow. Regularly deleting local branches helps enhance collaboration and project organization. By understanding the commands and processes outlined in this guide, you can confidently manage your Git branches, ensuring your repository remains streamlined and effective.
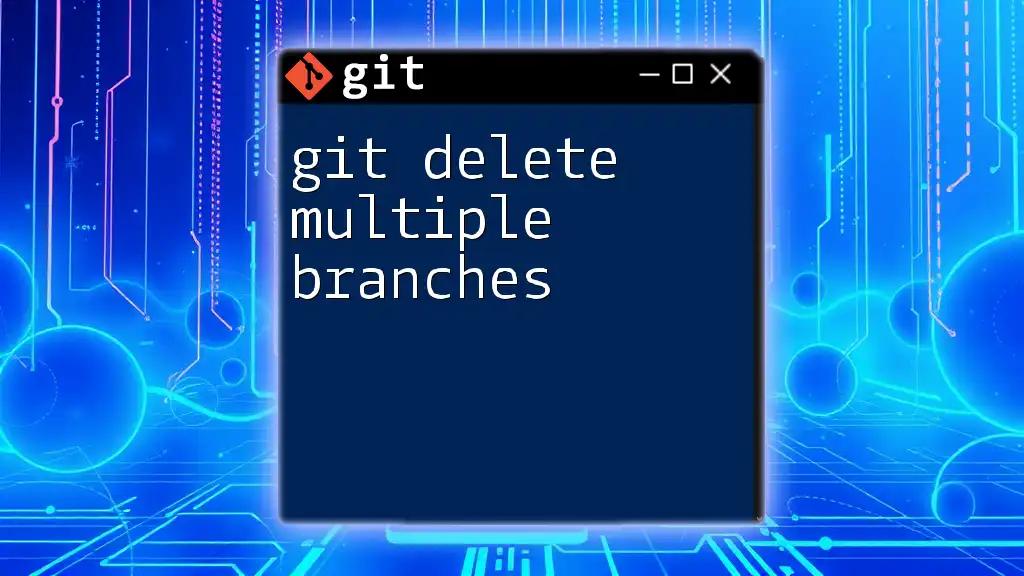
Call to Action
If you found this guide helpful, consider signing up for further tutorials on Git commands to level up your skills. Share your experiences or any questions regarding local branch management in the comments section below!
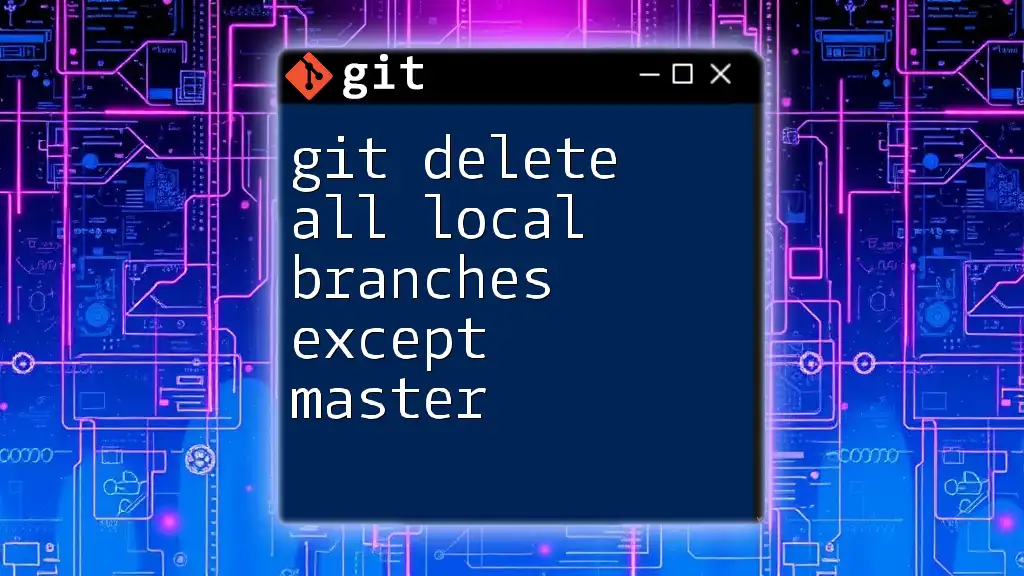
Additional Resources
For those looking to expand their Git knowledge, refer to the official Git documentation for in-depth explanations and additional commands. Explore further reading on Git best practices for a more efficient development process.