To reset a local branch to match its remote counterpart, you can use the following command, which discards any local changes and synchronizes the branch with the remote repository:
git fetch origin && git reset --hard origin/your-branch-name
Replace `your-branch-name` with the name of the branch you want to reset.
Understanding Git Branches
What is a Git Branch?
A Git branch is essentially a pointer to a particular commit in your project’s history. By allowing you to diverge from the main line of development, branches enable you to work on features or fixes in isolation. This feature is invaluable for collaborative development, as it allows multiple developers to work on different aspects of a project without merging changes until they are ready.
Example: In a typical workflow, the default branch is often named `main` or `master`. Developers create feature branches like `feature/login` or `bugfix/typo-fix` to encapsulate individual tasks. This keeps the main branch polished and stable.
Local vs. Remote Branches
Local branches exist on your machine's copy of the repository, while remote branches represent the state of branches on remote servers (like GitHub or GitLab).
- Local Branches: Created and manipulated in your local environment, allowing you to make any changes without affecting others.
- Remote Branches: Updated with the latest commits from your collaborators, providing a snapshot of what the branch looks like on the remote repository.
Understanding this distinction is crucial when you want to perform operations that involve resetting your local branch to align with its remote counterpart.
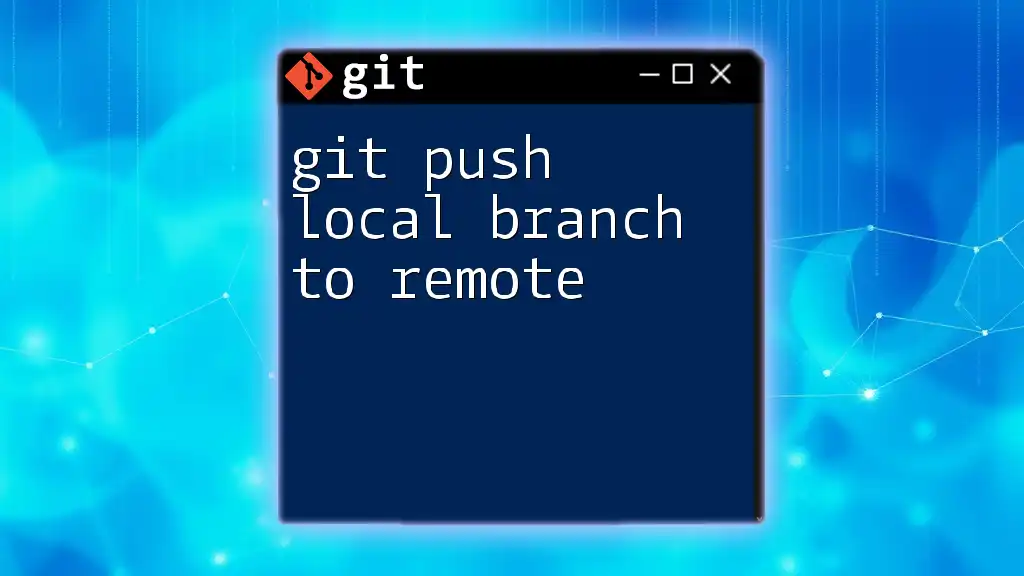
When to Reset Your Local Branch
Use Cases for Resetting
Resetting your local branch can help in several scenarios:
- Reverting Changes After a Flawed Commit: If you’ve made changes that you no longer want, a reset can undo them.
- Syncing a Branch That Has Diverged From the Remote: When your local branch has moved forward while the remote branch has also had new commits, they can diverge, leading to conflicts. Resetting helps realign your local branch to the remote.
Risks of Resetting
Before you proceed with resetting, consider the risks involved. A hard reset can lead to data loss. It is essential to commit any changes you wish to preserve before executing a reset. Always back up your work if you're unsure.
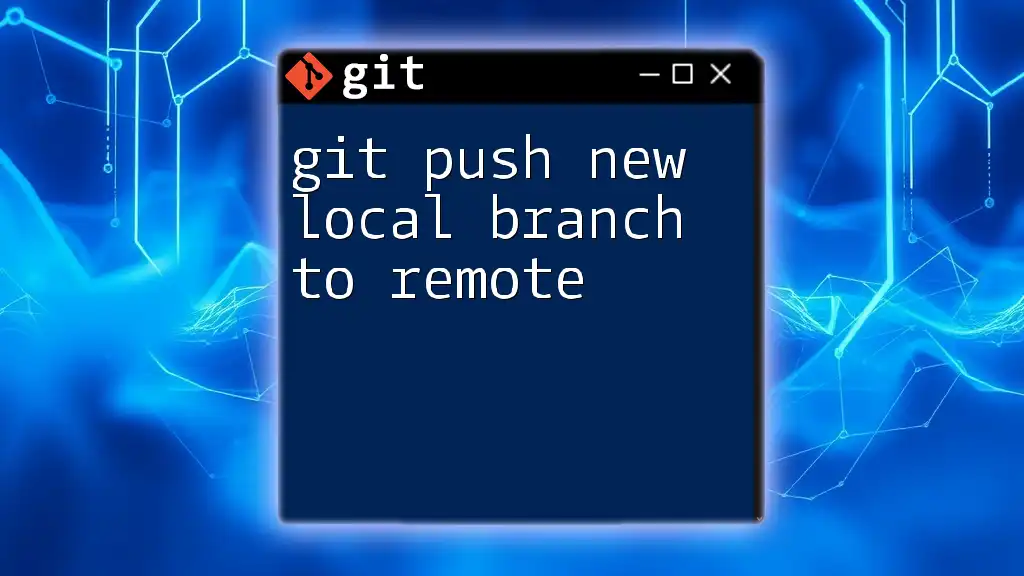
The Git Reset Command
What is `git reset`?
The `git reset` command is a powerful command that alters the staging area and determines the current branch's position in the commit history. The command has different options, including `soft`, `mixed`, and `hard`, each with varying effects on your working directory and staging area.
Types of Git Reset
-
Soft Reset: Moves the HEAD pointer to a previous commit without altering the staging area or working directory. Use this when you want to keep your changes in the staging area.
-
Mixed Reset: This is the default mode when no flags are provided. It moves the HEAD pointer and resets the staging area to the specified commit but doesn't modify your working directory. This is useful for unstaging changes.
-
Hard Reset: This option moves the HEAD pointer and resets both the staging area and working directory to match the specified commit. This will discard all local changes, both staged and unstaged, so it should be used with caution.
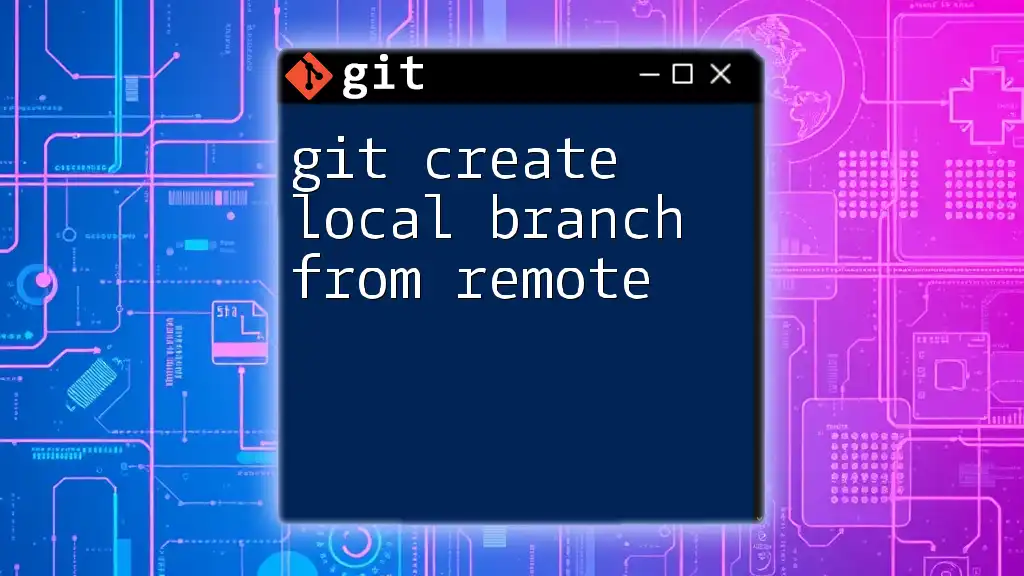
Resetting a Local Branch to Match the Remote
Step-by-Step Instructions
Prerequisites
To begin the process, ensure you are in the correct repository and on the branch you wish to reset. First, update your local tracking of branches by fetching the latest changes from the remote repository:
git fetch origin
Step 1: Identify the Remote Branch
Next, confirm the name of the remote branch you intend to align with your local branch. You can list all remote branches using:
git branch -r
This command displays all the branches available in the remote, ensuring that you're targeting the correct one.
Step 2: Resetting the Local Branch
Once you've confirmed the name of your remote branch, you can use the following command to reset your local branch to match the remote:
git reset --hard origin/branch-name
Here, replace `branch-name` with the actual name of your remote branch (e.g., `main`, `develop`). This command moves your local branch pointer to the state of the specified remote branch, discarding any local changes.
Common Scenarios
Example Scenario 1: Undoing Local Changes
Suppose you've made a series of commits to your local branch that you realize are not needed. After checking the status of your local repository:
git status
You can reset it using the `git reset --hard` command we discussed to revert back to the last commit on the remote branch.
Example Scenario 2: Fixing Diverged Branches
Imagine a situation where you've been actively developing on a local branch that has since diverged due to new commits on the remote. You're faced with merge conflicts when you attempt to pull updates. Instead of resolving these conflicts, employing the reset command can realign your local branch with the remote:
git reset --hard origin/branch-name
By doing this, you discard local changes and adopt the current state of the remote branch directly.
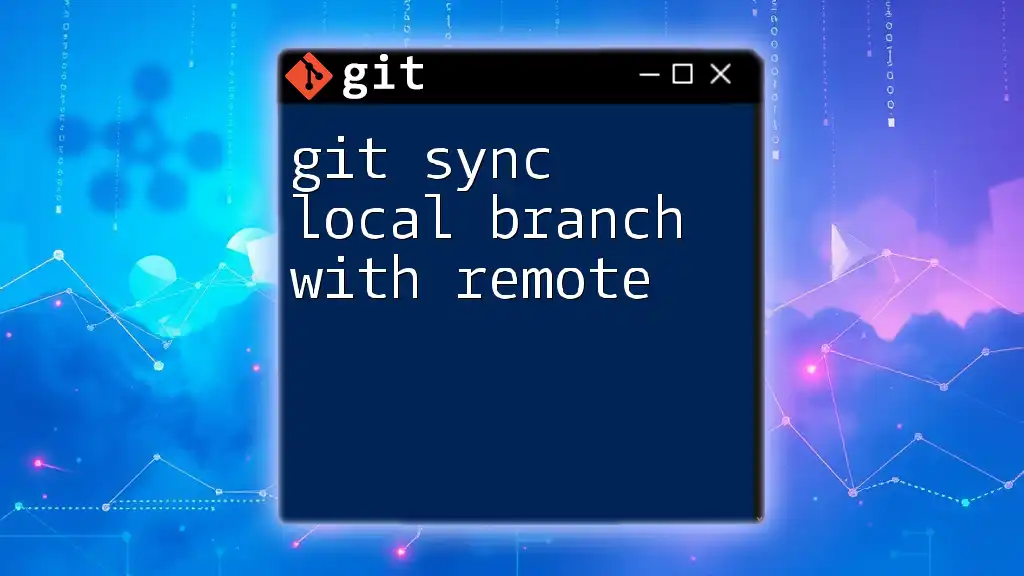
Best Practices for Using `git reset`
Verify Before You Reset
Before resetting, it’s critical to verify your current local branch status to prevent unintentional data loss. You can check your status and review pending changes:
git status
Regularly Push and Pull Changes
To minimize your reliance on reset commands, establish a habit of frequently pulling changes from the remote and pushing your commits. This practice keeps your local branch in sync and reduces divergence.
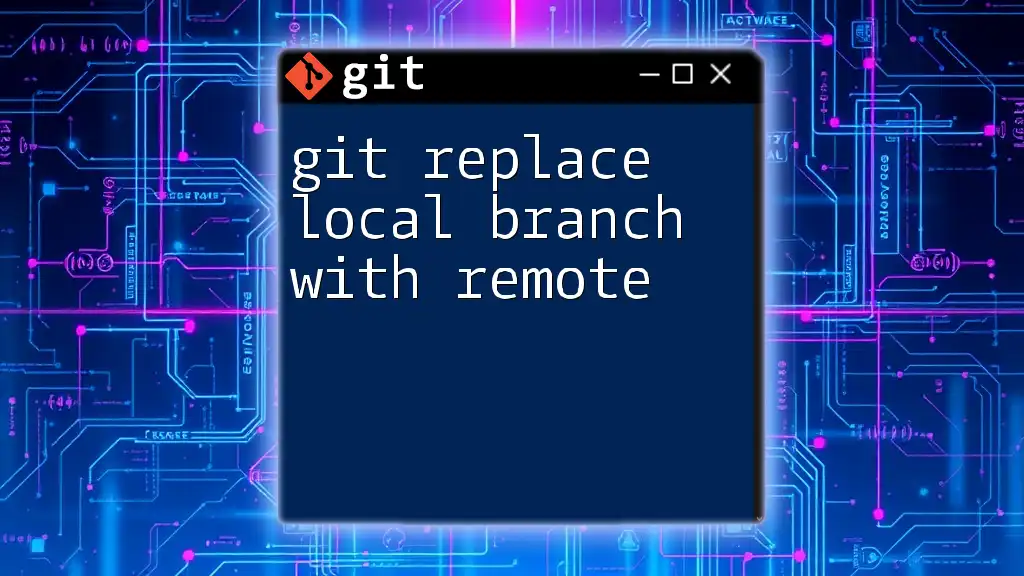
Conclusion
Resetting your local branch to match the remote is a fundamental skill for any Git user. While the `git reset` command is powerful, it must be used with care to avoid critical data loss. Always back up valuable changes, and consider if resetting is the best course of action for your situation.
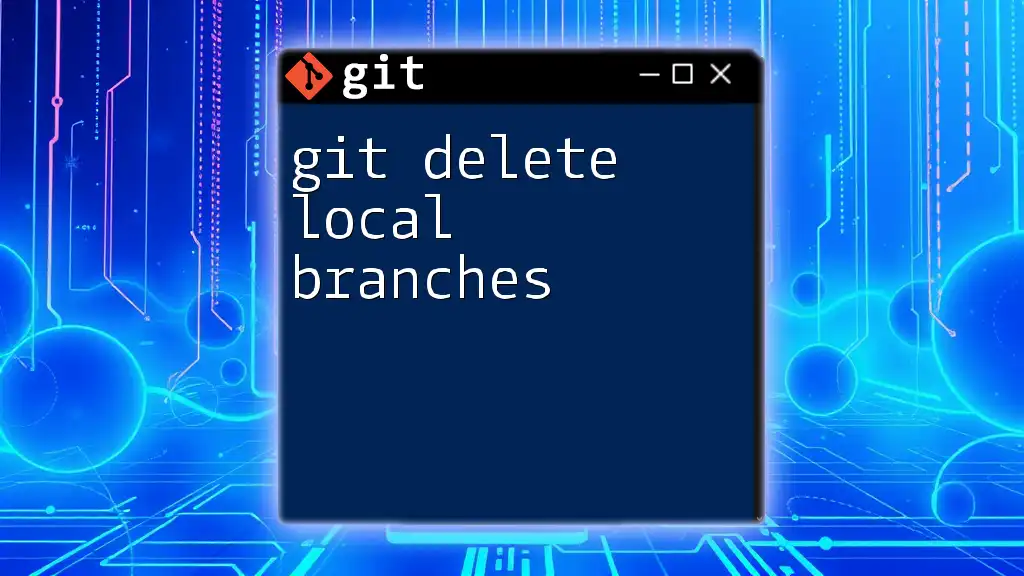
Additional Resources
Further Reading and Tools
For more information, the official Git documentation is invaluable. There are also many GUI Git clients available that can help visualize and manage branches, making the reset process easier.
FAQs
- Will I lose my local commits if I reset? Yes, a hard reset will remove all uncommitted or local commits that are not in the remote branch, so be sure to back up any important changes.
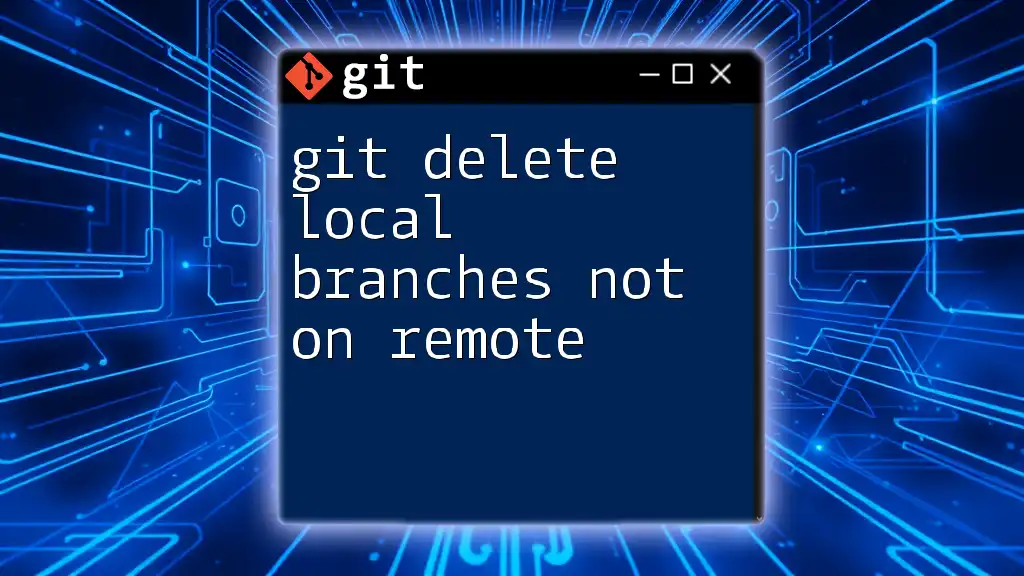
Call to Action
Have you experienced issues with resetting branches? Share your stories or questions in the comments below! Additionally, consider signing up for our service to dive deeper into mastering Git commands and best practices.