To reset your current branch to match another branch, you can use the `git reset` command followed by the branch name you want to reset to.
git reset --hard <branch-name>
Understanding Git Reset
What is Git Reset?
`git reset` is a powerful command in Git that allows you to change the current state of your branch to a previous snapshot. It essentially alters the `HEAD` reference and can impact the staging area and working directory, based on the type of reset you choose.
The Purpose of Using Git Reset
Developers often need to reset their current branch to manage their commit history effectively. Common reasons include:
- Undoing Mistakes: If you’ve made a commit that you want to remove, a reset can bring you back to a previous state.
- Cleaning Up History: Before merging branches, you may want to clean up your commit history for a more coherent narrative in your repository.
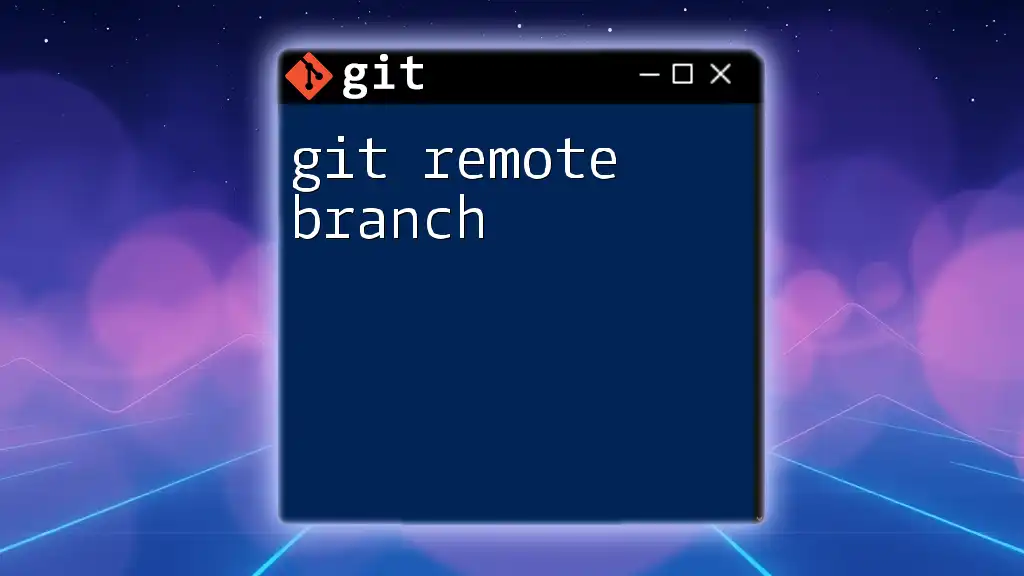
Types of Git Reset
Soft Reset
A soft reset moves the `HEAD` pointer to a specified commit while keeping changes staged in the index. It’s particularly useful when you want to maintain the changes in your working directory for a potential re-commit.
- Example: To perform a soft reset one commit back, you can use:
git reset --soft HEAD~1
- This command will move you back one commit while leaving all your changes available in the staging area.
Mixed Reset (Default)
Mixed reset is the default behavior of `git reset` if you don’t specify a flag. It moves the `HEAD` pointer and clears the staging index while keeping the working directory intact.
- Example: To reset without affecting your working directory, use:
git reset HEAD~1
- After this command, changes will remain in your working directory but will be untracked by Git.
Hard Reset
A hard reset resets the `HEAD`, staging area, and working directory to a specific commit entirely. This means it will remove all changes and commits that are after the target commit. This command should be used with caution, as it can lead to data loss.
- Example: To hard reset to the previous commit:
git reset --hard HEAD~1
- This command wipes out all committed changes and updates your working directory to match the commit you’re resetting to.
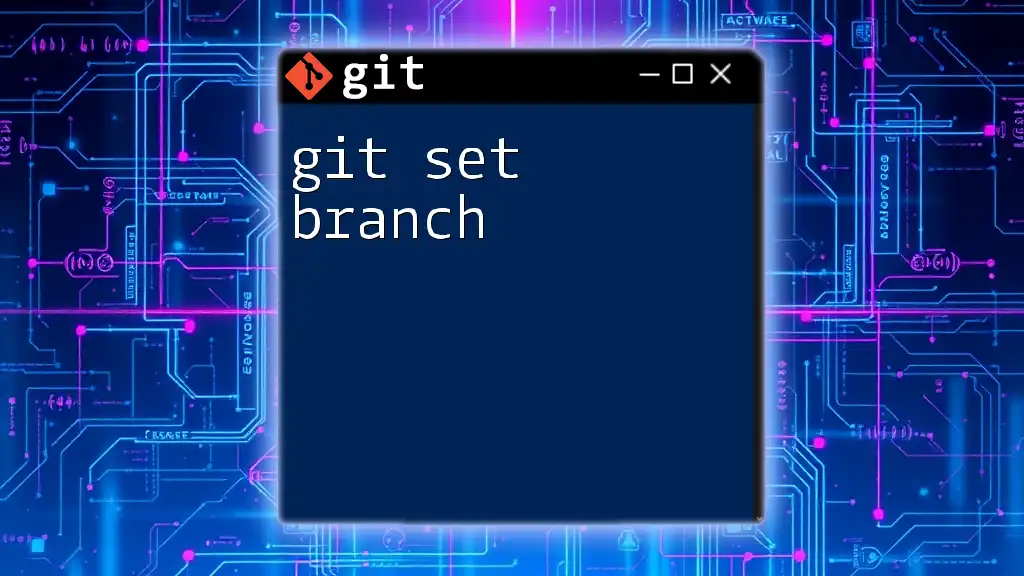
Resetting to a Specific Branch
Why Reset to a Specific Branch?
Resetting to a specific branch can resolve conflicts when you've made commits on your current branch that you don't want to keep. This is particularly useful when trying to revert to a stable version of your project from an experimental feature branch.
Step-by-Step Guide on Resetting to a Branch
Step 1: Checkout the Target Branch
Before you reset, you need to be on the target branch. To do this, use:
git checkout target-branch
This action sets your working directory to the state of `target-branch`.
Step 2: Performing a Soft Reset
If you want to keep the changes but remove the commits from history, you can execute:
git reset --soft branch-name
This will reset your current branch so that the changes in `branch-name` become the staged changes in your working directory.
Step 3: Performing a Mixed Reset
For a reset while keeping your changes in the working directory, run:
git reset branch-name
This will clear the staging area and leave your working directory unchanged, allowing you the ability to selectively stage your changes again.
Step 4: Performing a Hard Reset
If you need to remove all changes and commits after a certain point, use:
git reset --hard branch-name
This will make your local branch exactly match `branch-name`, losing any uncommitted changes.
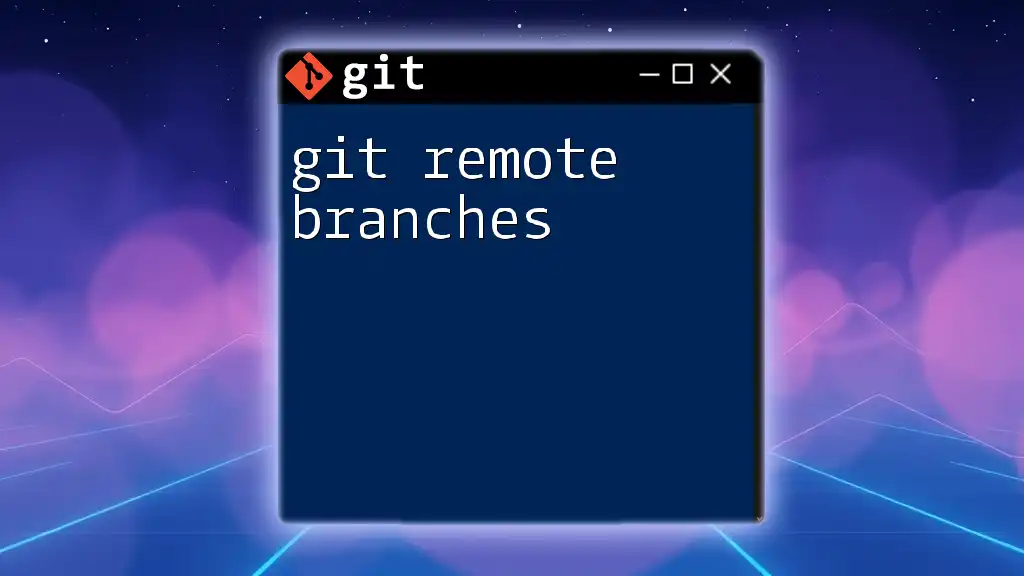
Real-World Examples
Example 1: Reverting to a Stable Release
Imagine you're working in a feature branch and realize that recent commits contain bugs. You can revert to a stable state with the following commands:
- Checkout the stable branch (e.g., `main`):
git checkout main
- Reset to that branch:
git reset --hard main
This will remove all recent changes, bringing you back to the last stable version.
Example 2: Undoing Recent Commits Before a Merge
If you’ve made several commits that are no longer needed before merging a feature branch, you might want to reset to the last clean commit:
- Checkout the feature branch:
git checkout feature-branch
- Reset is performed by:
git reset --soft HEAD~3
This command will remove the last three commits while leaving your changes staged, allowing you to rework or commit them properly.
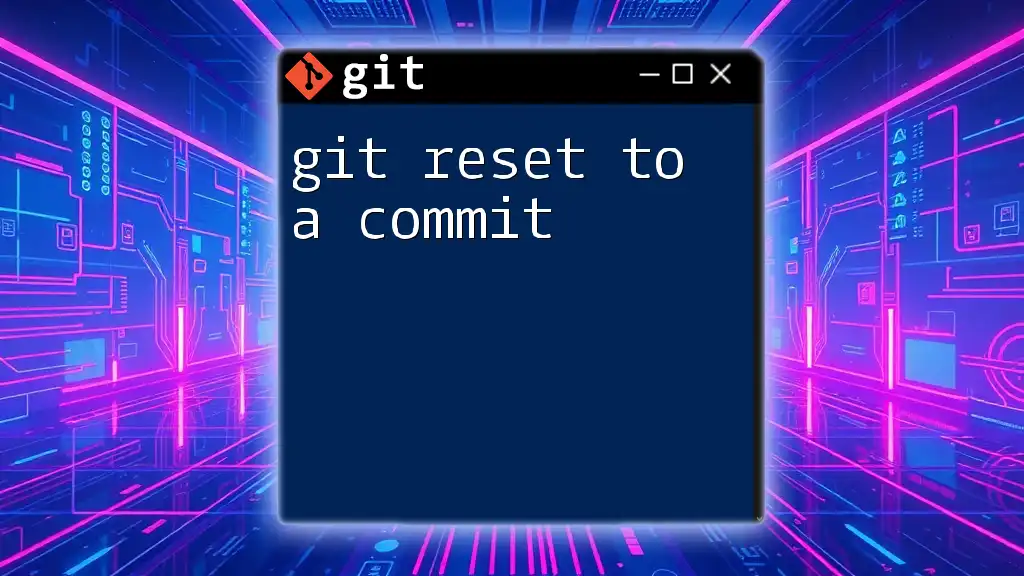
Common Mistakes and How to Avoid Them
Mistake 1: Forgetting to Backup Changes
One of the most frequent mistakes with `git reset` is forgetting to back up changes. Always consider using `git stash` before executing a reset command to safeguard against unintentional data loss.
Mistake 2: Confusing Reset Types
New users often confuse between `--soft`, `--mixed`, and `--hard`. Remember this simple tip:
- Use `--soft` to keep all changes staged.
- Use mixed (or no flag) to keep changes unstaged but still in the working directory.
- Use `--hard` to throw away all changes and revert completely.
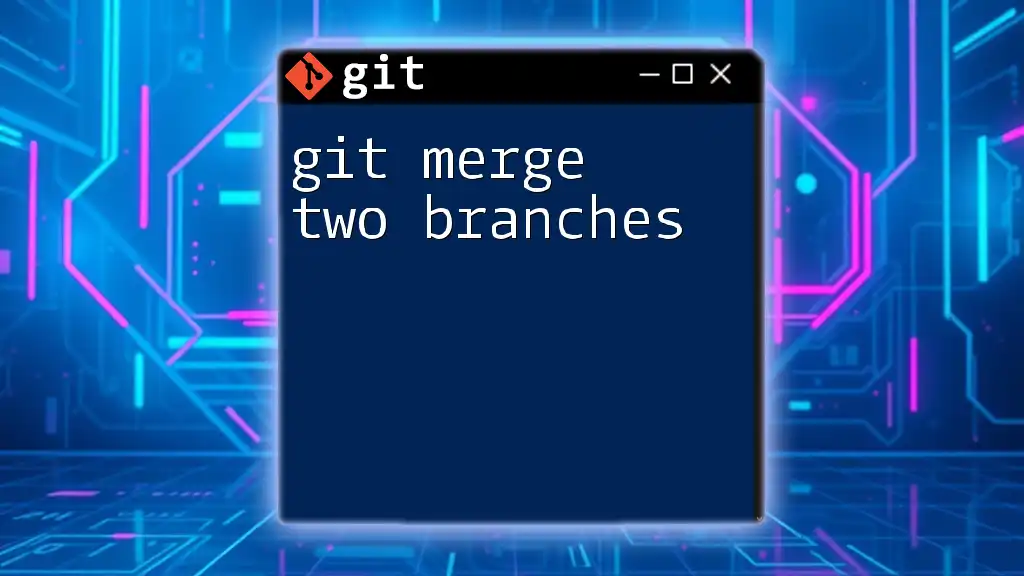
Conclusion
Mastering the `git reset to branch` command is crucial for effective version control in Git. By understanding the implications of each type of reset, you can navigate your repositories with more confidence. Practice using these commands to enhance your Git proficiency, and ensure to back up any crucial changes to avoid unintentional data loss. Embrace your learning journey and dive deeper into the world of Git commands!
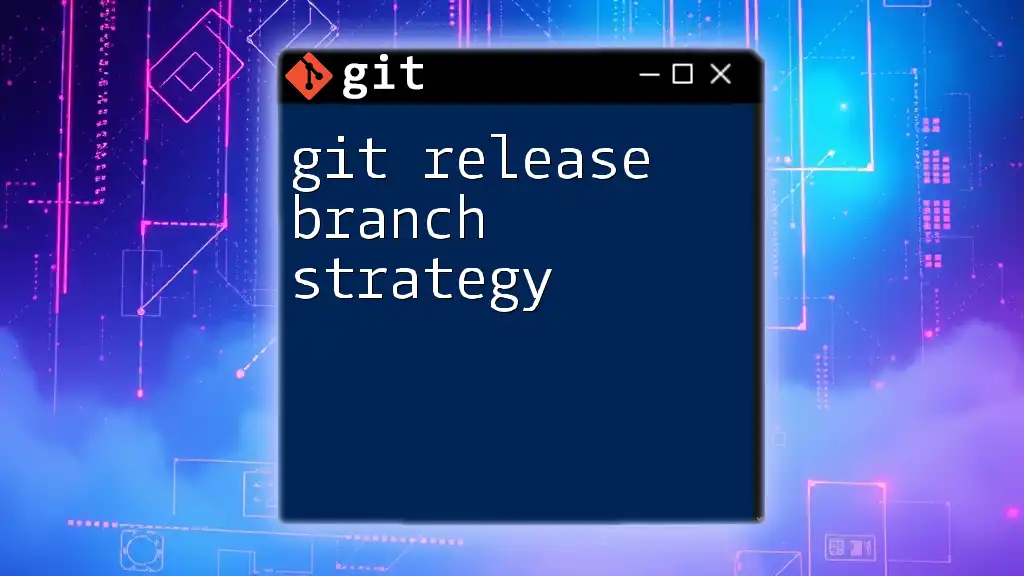
Additional Resources
For further reading and learning, refer to the official [Git documentation](https://git-scm.com/doc), join forums or communities, and consider subscribing to Git tutorials for ongoing education.