The master branch in Git is the default primary branch where the main development occurs, typically used to represent stable code that is ready for production.
git checkout master
What is Git?
Git is a powerful version control system that allows developers to track changes in their code, collaborate with others, and maintain a comprehensive history of their project development. Understanding Git is essential for any developer in today’s fast-paced software environment. Central to this understanding is the concept of branches, which allow for isolated development efforts within a project.
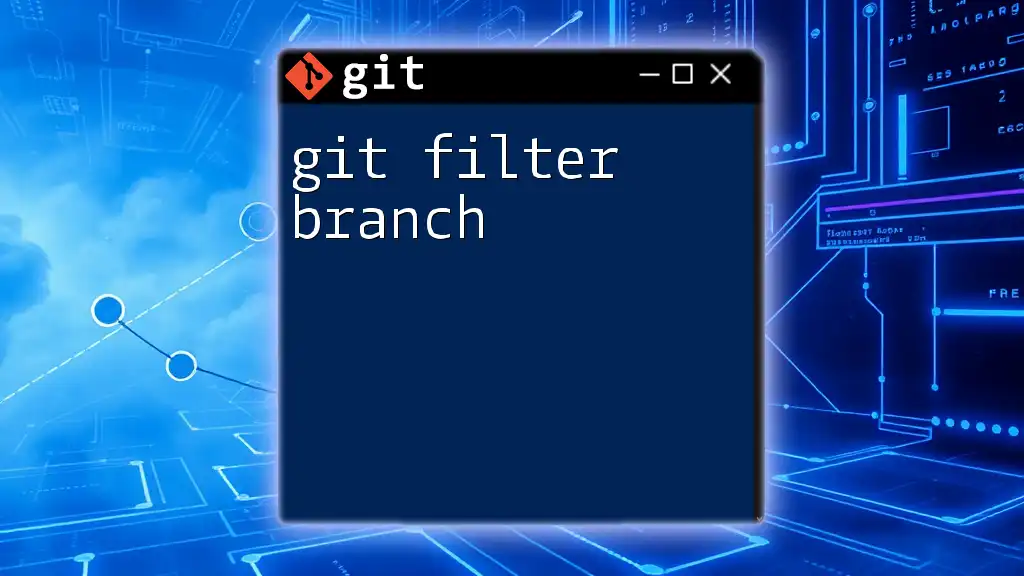
What is the Master Branch?
The master branch is the default branch in Git repositories where the stable version of the project resides. Historically, it has been the primary focus for developers and contains the production-ready code. Over time, some communities have shifted to using the term "main" instead of "master" to foster inclusivity. Nonetheless, whether it's called master or main, this branch holds significant importance in any Git workflow.
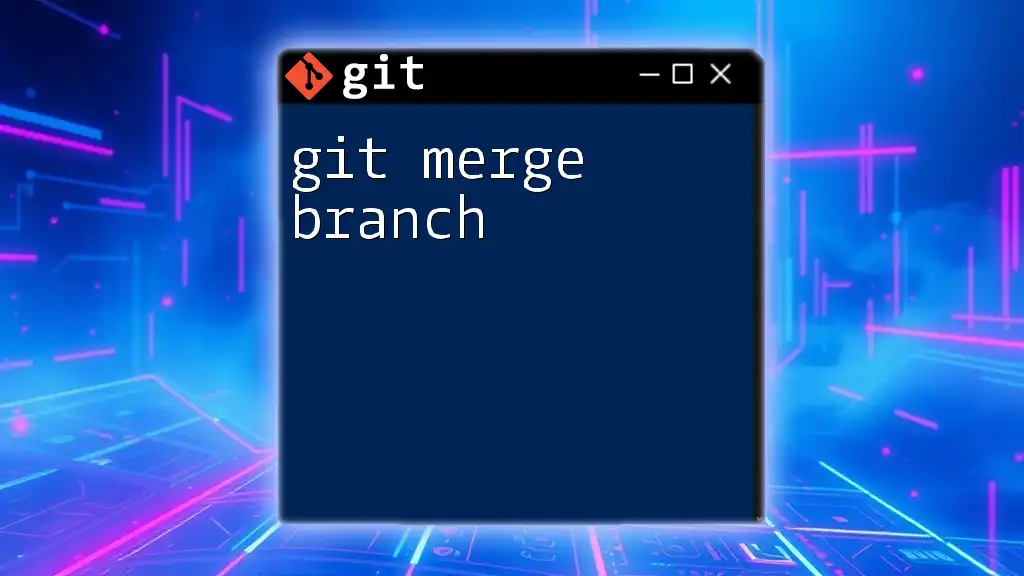
Understanding Branches in Git
What is a Branch?
A branch in Git is simply a lightweight pointer to a specific commit in your project’s history. Think of branches as separate lines of development where you can work on features or fixes without disturbing the main codebase. When you branch away from the master, you can experiment freely without the risk of disrupting the stable code in the master branch.
Importance of the Master Branch
The master branch is typically where your most stable code lives. It serves as the main development line for your project and is essential for collaboration. When more than one developer is working on a project, regular synchronization with the master branch ensures that everyone is working with a unified version of the project, reducing the risk of conflicts.
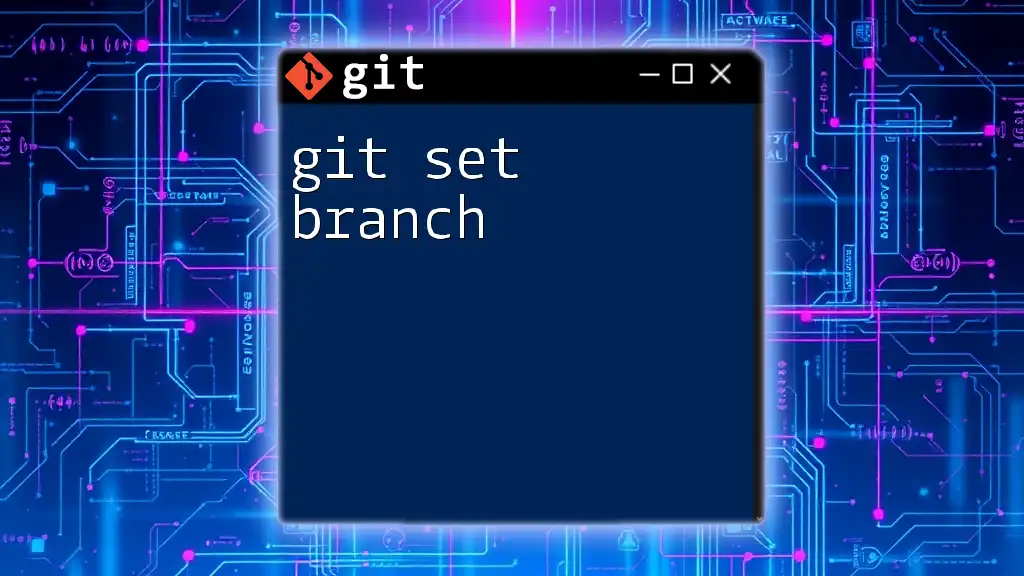
Creating and Working with the Master Branch
Check Out the Master Branch
To begin working with the master branch, you need to switch your working directory to it. You can do this using the following command:
git checkout master
This command updates your working directory to the latest commit on the master branch, and any new changes you make will now reflect in this branch.
Creating a New Master Branch
In some scenarios, you might want to create a new master branch, especially if you are starting a new project or needing a major overhaul of your codebase. You can accomplish this with:
git branch master
This command effectively creates a new branch named "master." It’s crucial to always ensure that you’re working from a stable state of your current branch before creating the new master to avoid carrying over any unstable code.
Switching to the Master Branch
If you need to switch to the master branch at any point, you can do so using:
git switch master
This command is particularly useful when you have multiple branches and need to revert to the main development line. Before switching branches, make sure to commit any changes in your current branch, as Git will not allow you to switch with uncommitted changes.
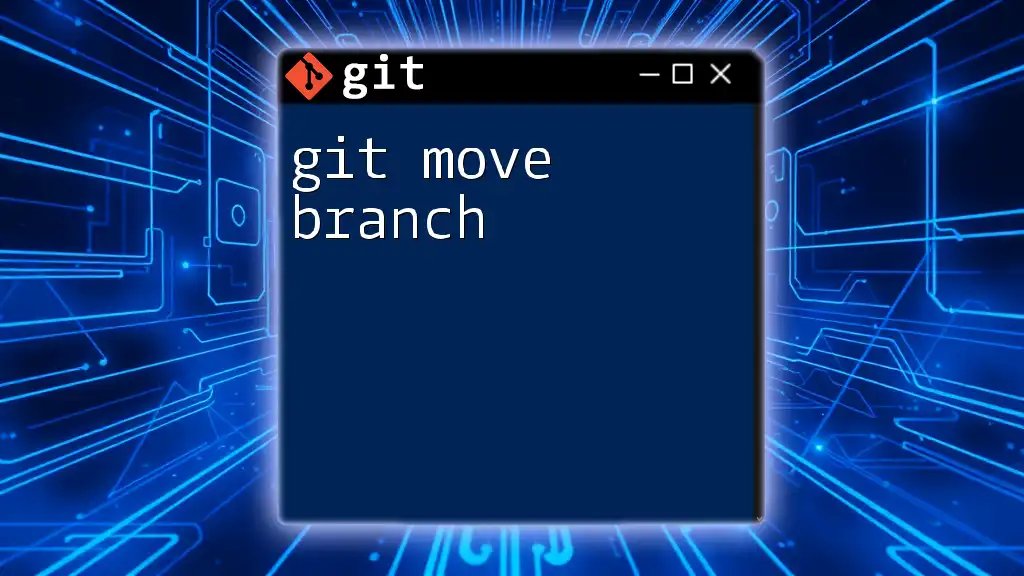
Best Practices for Using the Master Branch
Commit Only Stable Code
A best practice for maintaining the integrity of the master branch is to only commit stable and tested code. The ideal workflow is to develop features or bug fixes in separate branches, thoroughly test them, and only then merge them into the master branch. This approach minimizes the risk of introducing bugs into the production code.
Regularly Sync with Remote
To keep the master branch up-to-date, regularly sync it with the remote repository using:
git pull origin master
This command fetches and merges changes from the remote master branch into your local master branch. Staying updated is crucial to avoid conflicts, especially if multiple team members are working on the same codebase.
Merging Changes to the Master Branch
When your feature branch is ready to be integrated into the master branch, you typically perform a merge. The command to merge a feature branch back into master is:
git merge <branch-name>
This command brings the changes from the specified branch into the master branch. Be sure to resolve any merge conflicts that may arise during this process to maintain a clean codebase.
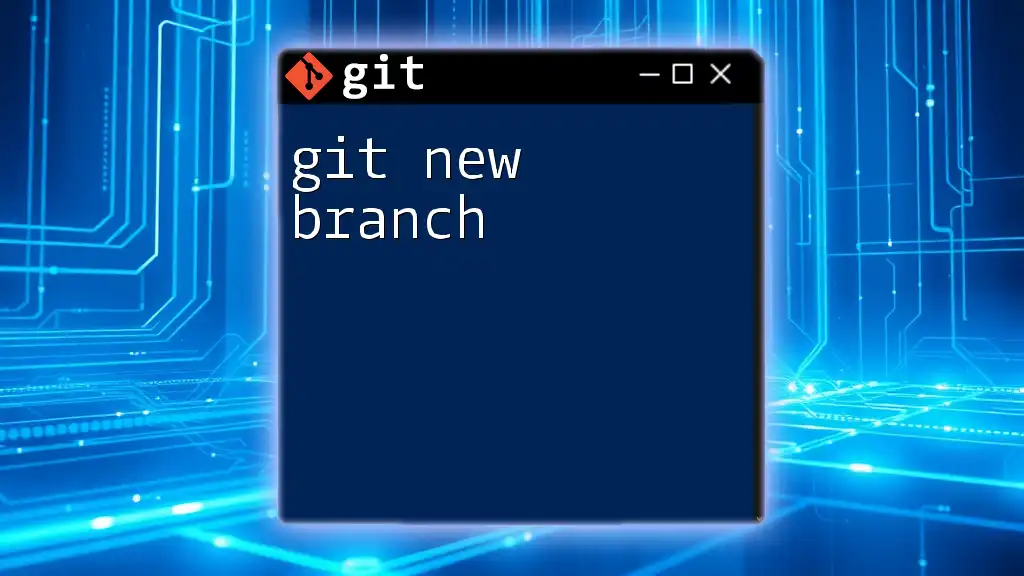
Troubleshooting Common Issues with the Master Branch
Conflicts During Merge
Merge conflicts often occur when changes in the master branch and your feature branch affect the same lines of code. Git will notify you of the conflict. Resolving conflicts involves editing the conflicting files, identifying the parts that need to be corrected, and finalizing the merge with a commit.
Undoing Changes in the Master Branch
In case you need to undo changes made in the master branch, there are two primary commands to consider: `git reset` and `git revert`.
- Git Reset is useful for removing commits permanently:
git reset --hard HEAD~1
This command will reset the state of your master branch to one commit prior. Use it with caution, as it can lead to loss of data.
- Git Revert creates a new commit that undoes the changes made by an existing commit. This is safer, as it preserves the project history:
git revert <commit-hash>
This command is beneficial for keeping the integrity of your commit history intact.
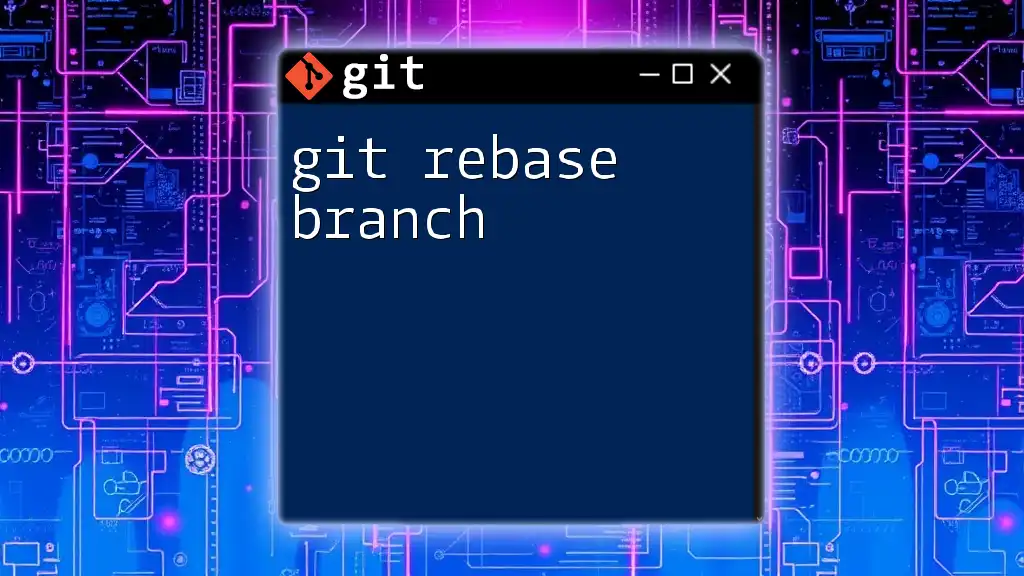
Moving Beyond the Master Branch
Understanding Branching Models
Various branching models exist to help manage workflows efficiently. Popular methods include Git Flow and GitHub Flow. Each model provides a structured approach to development, typically using the master branch as the foundation upon which other branches are built. By understanding how the master branch operates within these models, you can optimize your development process.
Transitioning from Master to Main
In recent years, there has been a notable shift in terminology from "master" to "main" within the Git community. If you decide to rename your branch to "main," you can do so with the following command:
git branch -m master main
This is an important step towards creating an inclusive environment. Moreover, it is vital to update any references to “master” in your continuous integration and deployment scripts to reflect this change.
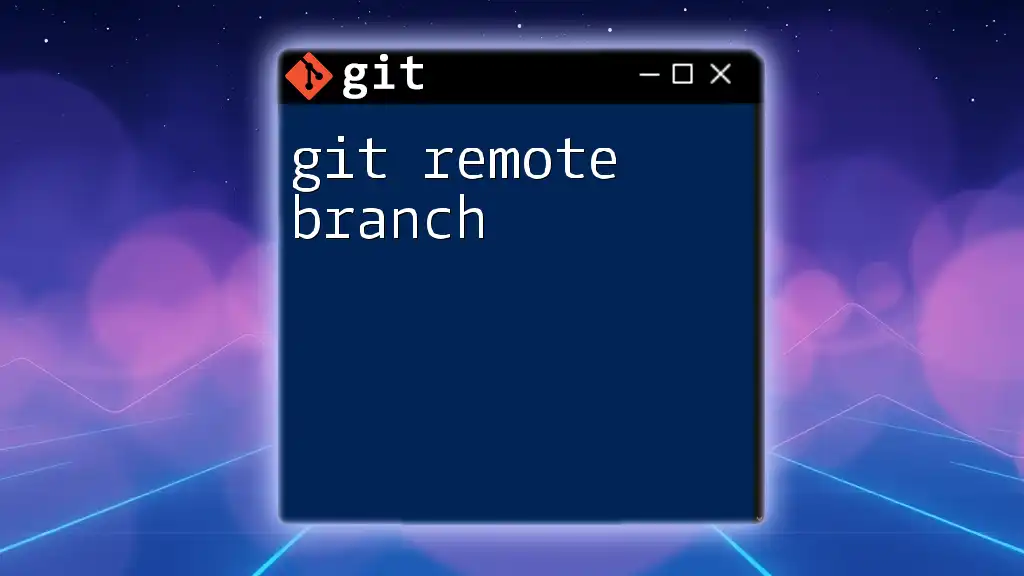
Conclusion
The git master branch is a cornerstone concept in Git workflows. Understanding its importance and adhering to best practices ensures a stable codebase that promotes effective collaboration. Practice the commands and concepts outlined in this guide to solidify your understanding and efficiency in using Git. As you continue to develop your skills, remember that mastering these foundational elements will empower you in your software development journey.
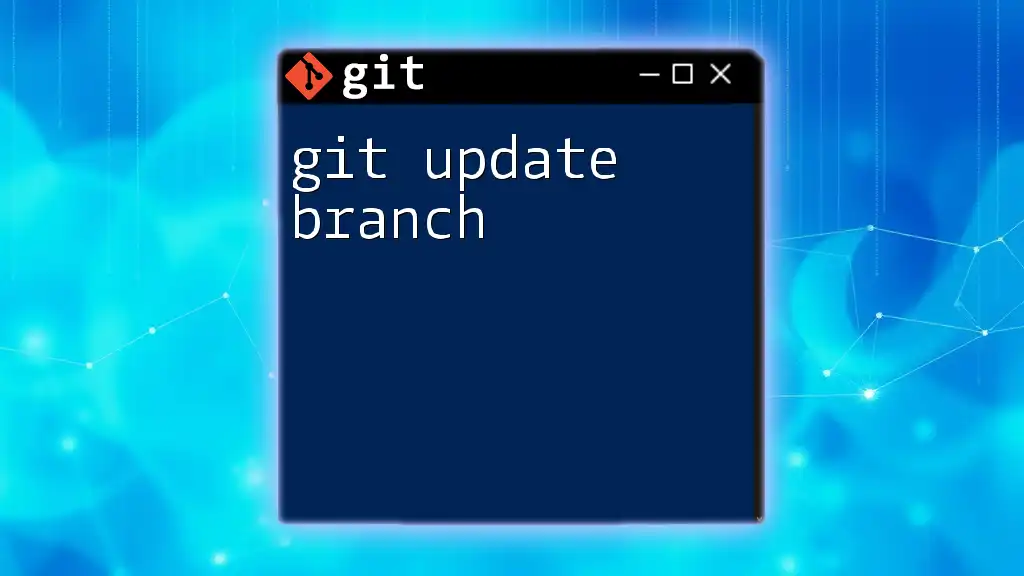
Additional Resources
To improve your Git knowledge further, consider exploring the official Git documentation. There are numerous enriching resources, including books, online courses, and communities where you can enhance your understanding of Git and its intricacies. If you're looking to deepen your skills even more, feel free to check out our upcoming classes that focus on Git commands and best practices.