The `git filter-branch` command allows you to rewrite Git history by applying specified filters to existing commits, enabling you to make bulk changes across branches.
Here’s a basic usage example:
git filter-branch --env-filter '
OLD_EMAIL="old@example.com"
CORRECT_NAME="New Name"
CORRECT_EMAIL="new@example.com"
if [ "$GIT_COMMITTER_EMAIL" = "$OLD_EMAIL" ]
then
export GIT_COMMITTER_NAME="$CORRECT_NAME"
export GIT_COMMITTER_EMAIL="$CORRECT_EMAIL"
fi
' -- --all
What is Git Filter Branch?
Git filter branch is a powerful command used in Git to rewrite the history of a repository. It allows users to modify commits—an essential feature when you need to clean up a repository by removing sensitive information, correcting author details, or reorganizing a project's structure.
Use Cases
-
Removing Sensitive Data: If you accidentally committed confidential data (like API keys or passwords), using `git filter branch` to purge that data from the entire history is crucial.
-
Changing Author Information: If you need to update the author’s email address on several commits, this command grants you the ability to retroactively correct these details.
-
Splitting a Subdirectory: When you need to isolate a specific part of a repository into its separate project, `git filter branch` can help you extract that subdirectory while preserving its history.

How Git Filter Branch Works
Basic Mechanics
The command rewrites commits in the history of a repository, creating a new set of commits with altered histories based on the specified filters. This process can significantly transform how your project's timeline looks, which is powerful but should be approached cautiously.
Command Syntax
The basic syntax for the `git filter branch` command is structured as follows:
git filter-branch [options] <filter> -- <ref>
This means you can apply different types of filters with various options on specified references.
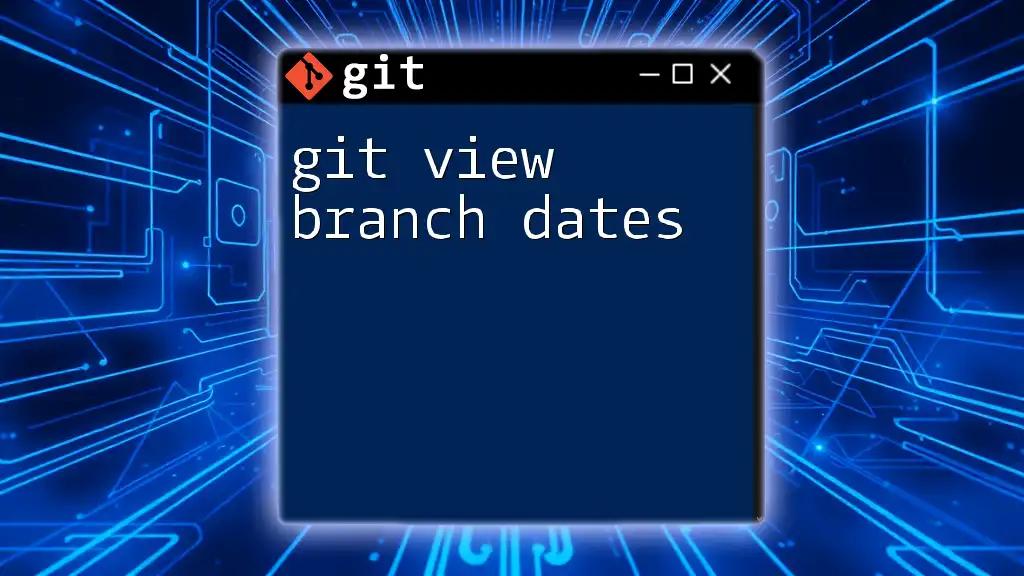
Commonly Used Options
`--env-filter`
This option modifies the environment variables for commits, allowing you to change the author names and emails directly. For example, if you mistakenly used a wrong email address, you can correct it like this:
git filter-branch --env-filter 'GIT_AUTHOR_EMAIL="new@example.com"; GIT_COMMITTER_EMAIL="new@example.com"' HEAD
This command updates both the author and committer emails for every commit in the current branch.
`--tree-filter`
With `--tree-filter`, you can run arbitrary commands against the entire working tree for each commit. This is useful for files you want to delete across all commits. For instance, removing a sensitive file named `secrets.txt` can be achieved as follows:
git filter-branch --tree-filter 'rm -f secrets.txt' HEAD
`--index-filter`
If speed is a concern, `--index-filter` is a faster alternative because it executes commands on the index rather than checking out files into a working directory. Here’s how to remove a file from the history using this option:
git filter-branch --index-filter 'git rm --cached --ignore-unmatch filename.txt' HEAD
This command can execute much more quickly, making it ideal for larger repositories.
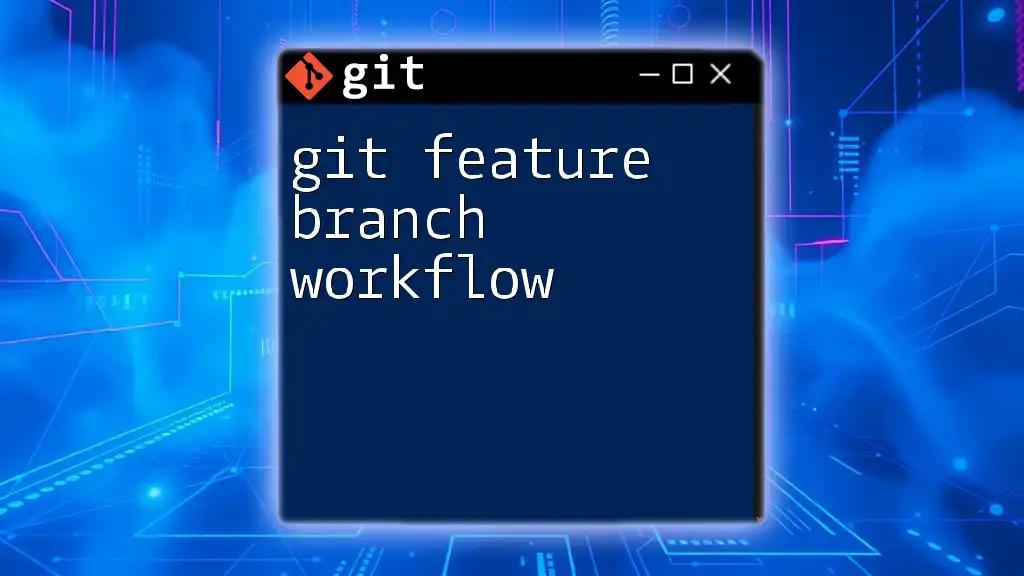
Step-by-Step Guide to Using Git Filter Branch
Step 1: Backup Your Repository
Before manipulating history, always make a backup of your repository. This precaution saves you from irreversible changes. Here’s how you can back up your repository:
git clone --mirror original-repo.git backup-repo.git
Step 2: Run the Filter Branch Command
Once you have your backup, it’s time to run the filter branch command. For example, if you want to remove all instances of `secrets.txt`, you can execute:
git filter-branch --tree-filter 'rm -f secrets.txt' HEAD
Understanding the command: This command will step through each commit in the current branch, executing the `rm -f secrets.txt` command. If `secrets.txt` is found in any commit, it will be removed.
Step 3: Verification
After running the filter branch command, it’s crucial to verify the changes. You can check your commit history to ensure that the changes have been applied correctly:
git log
Look through the commit messages and files to confirm that removals or changes took place as intended.
Step 4: Cleanup
After using `git filter branch`, you'll need to clean up any references to the original history to avoid confusion. Execute the following command to do so:
rm -rf .git/refs/original/ && git reflog expire --expire=now --all && git gc --prune=now --aggressive
This command removes old references and garbage collects and prunes your repository, creating a cleaner history.
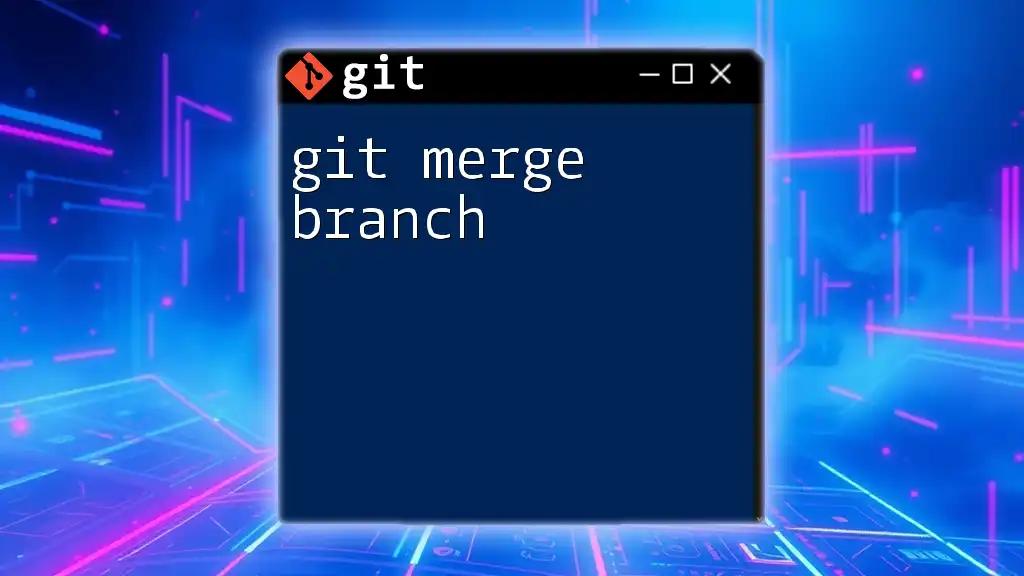
Alternatives to Git Filter Branch
Git Rebase
While `git filter branch` is invaluable for history rewriting, `git rebase` offers a different approach for linearizing commit histories. It is best used when you want to rearrange, delete, or combine commits rather than altering earlier entries. This approach is more effective for projects still in development.
BFG Repo-Cleaner
An alternative specifically designed for removing large files and sensitive data from Git history is BFG Repo-Cleaner. This tool is not only easier to use but significantly faster than `git filter branch`, especially in larger repositories. Choose BFG when you need a straightforward way to clean up without extensive scripting.
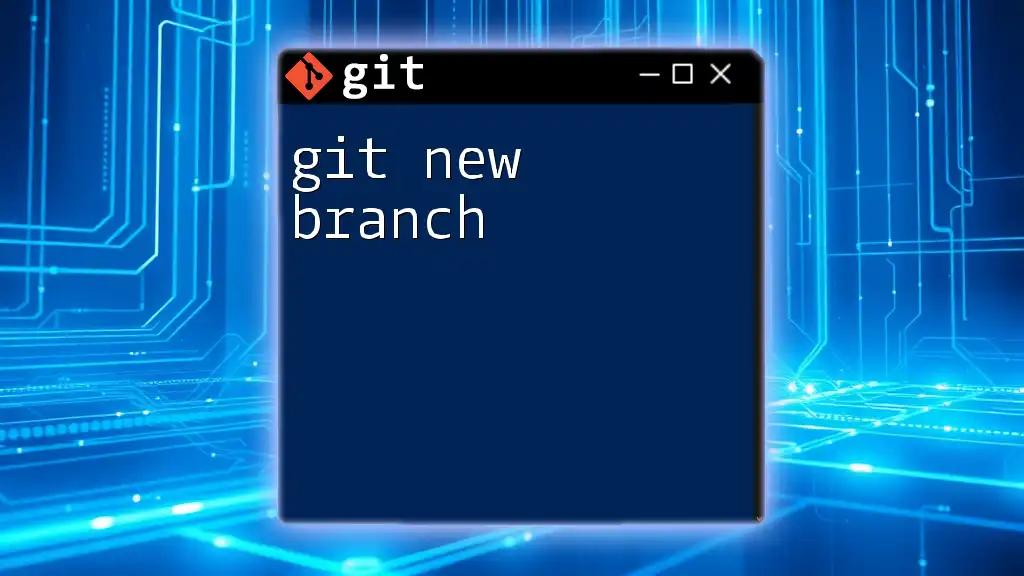
Conclusion
The git filter branch command provides incredible flexibility for managing a repository's history. However, it should be used with caution since it rewrites history. Understanding when and how to use this tool will help you maintain a cleaner project timeline while keeping the integrity of your code intact.
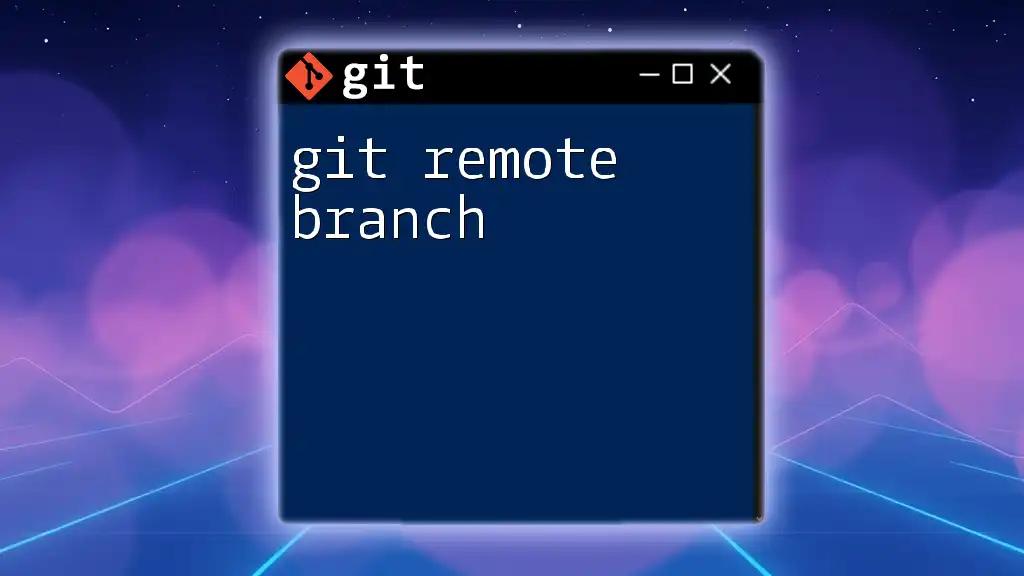
Additional Resources
For continued learning, refer to the official Git documentation for comprehensive details on the `git filter branch` command and its options. Many online resources, including tutorials and videos, are also available to deepen your understanding of this powerful tool.
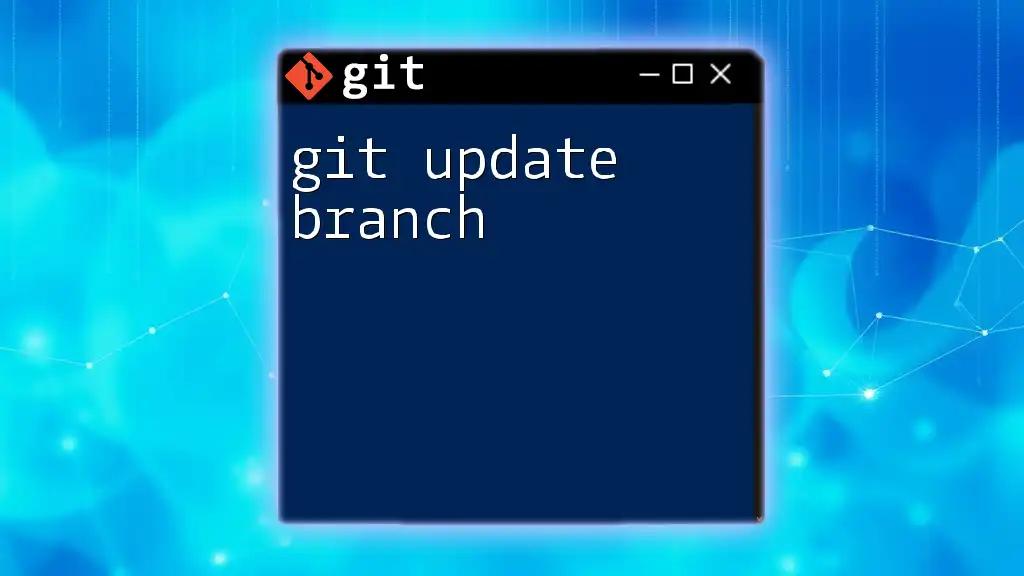
FAQs
What is the main difference between `git filter branch` and `git rebase`?
The primary distinction lies in usage. `git rebase` is suited for reshaping the commits you have yet to push, while `git filter branch` rewrites the history of commits that may already be shared or pushed to a remote.
Can `git filter branch` be undone?
Since `git filter branch` rewrites history, reverting changes can be complex. This reinforces the necessity of backups before making any history-altering commands.
When should `git filter branch` be avoided?
Be cautious with `git filter branch` on publicly shared repositories, as altering commit history can lead to confusion among collaborators. Use it mainly for private or non-distributed repositories where you control the entire history.
By following this guide, you should now have a comprehensive grasp of git filter branch, its applications, and best practices for safely using it in your project workflows.