Git branches allow you to create separate lines of development within a repository, enabling multiple features or experiments to be developed in isolation from each other. Here's a simple command to create and switch to a new branch called "feature-branch":
git checkout -b feature-branch
Understanding Branches
What is a Branch?
In Git, a branch is essentially a pointer to one of the commits in your repository's history. Each branch represents an independent line of development, allowing you to work on multiple features, fixes, or experiments simultaneously without impacting the main codebase.
This capability is crucial in collaborative environments where several developers might be adding new features or addressing bugs at the same time. By creating branches, you can isolate your work, ensuring that the codebase remains stable while you make changes.
Why Use Branches?
Using branches in your projects offers several advantages:
- Enhanced Collaboration: Different team members can work on separate branches without interfering with one another’s work.
- Easier Bug Fixes and Feature Implementation: You can create branches specifically for bug fixes or to implement new features without risking the integrity of the main branch (usually `main` or `master`).
- Safe Experimentation: Branches provide a safe environment for experimenting with new ideas or refactoring code. If an experiment fails, you can simply delete the branch without affecting the main development line.
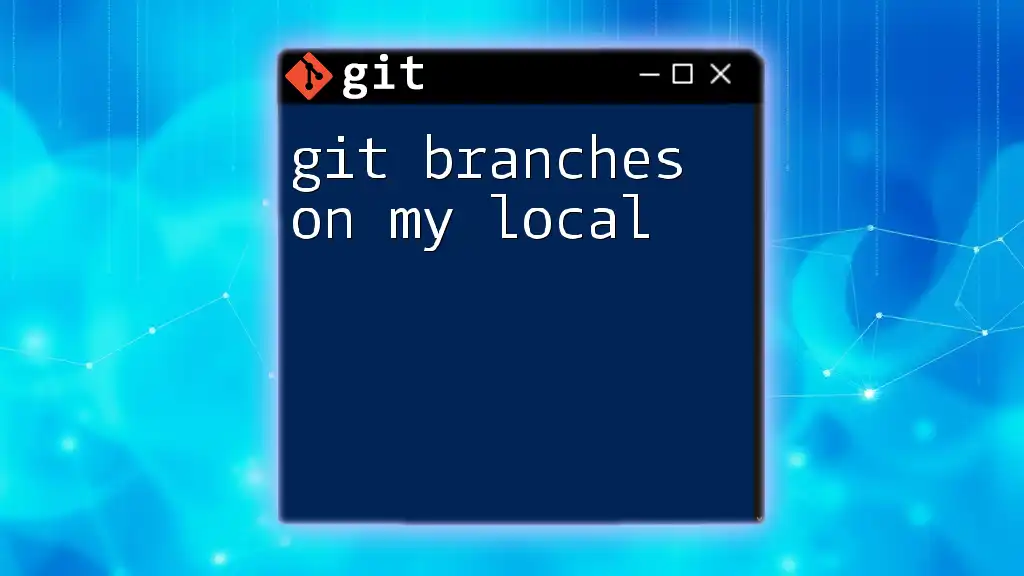
Basic Branching Commands
Creating a Branch
To create a new branch, use the following command:
git branch <branch-name>
For example, if you want to create a branch for a login form feature, you would execute:
git branch feature/login-form
This command creates a new branch called `feature/login-form`. However, keep in mind that simply creating a branch does not switch to it; you will remain on your current branch until you explicitly check out the new one.
Switching Branches
To switch to a different branch, you can use one of the following commands:
-
Checkout Command:
git checkout <branch-name>
-
Switch Command: (preferred in newer Git versions)
git switch <branch-name>
For example, to switch to the `feature/login-form` branch:
git checkout feature/login-form
or
git switch feature/login-form
While both commands achieve the same result, `git switch` is more intuitive and specifically designed for branch switching.
Listing Branches
To view all branches in your repository, use the command:
git branch
This will display a list of all branches, highlighting the one you are currently on. The output will look something like this:
* main
feature/login-form
bugfix/fix-button
The asterisk (*) indicates you are currently on the `main` branch.
Deleting a Branch
If you need to remove a branch that is no longer necessary, you can do so with:
git branch -d <branch-name>
For example, to delete the `feature/login-form` branch, run:
git branch -d feature/login-form
It’s important to note that Git will prevent you from deleting a branch if it contains changes that haven't been merged into the main branch. If you are sure you want to delete a branch regardless, you can force delete it with:
git branch -D <branch-name>
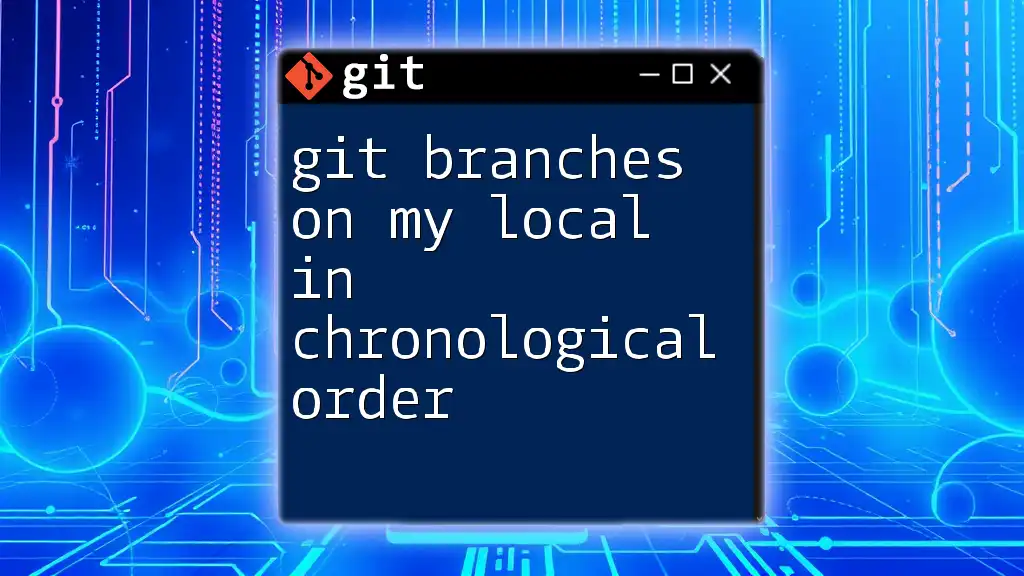
Advanced Branching Concepts
Merging Branches
What is Merging?
Merging is the process of incorporating changes from one branch into another. This is a vital part of collaborative development where code contributions from various branches need to come together into the main branch.
Performing a Merge
To merge changes from another branch (e.g., `feature/login-form`) into your current branch (e.g., `main`), first switch to the target branch:
git checkout main
Then execute the merge command:
git merge feature/login-form
This command will combine the changes from the `feature/login-form` branch into the `main` branch. If the merge is straightforward, Git will do this automatically. However, if there are conflicting changes, you will need to resolve them manually.
Resolving Merge Conflicts
A merge conflict occurs when changes made in different branches affect the same lines in a file. When this happens, Git cannot automatically merge the branches.
When a conflict arises, Git will notify you. You can identify conflicts by looking for sections like this in your files:
<<<<<<< HEAD
Current change in main
=======
Change in feature/login-form
>>>>>>> feature/login-form
To resolve the conflict, you must edit the file to select the desired changes, then save it. After resolving conflicts in all affected files, mark them as resolved using:
git add <resolved-file>
Finally, complete the merge with:
git commit
Rebasing
Rebasing is an alternative to merging and involves moving or combining a sequence of commits to a new base commit. This can create a cleaner project history.
To rebase your feature branch onto `main`, execute the following commands:
git checkout feature/login-form
git rebase main
During rebasing, Git will replay your changes over the latest commit in `main`. If conflicts occur, resolve them as described previously. After resolving, continue the rebase with:
git rebase --continue
The key distinction between merging and rebasing is that merging creates a merge commit, whereas rebasing keeps your history linear, making it easier to navigate.
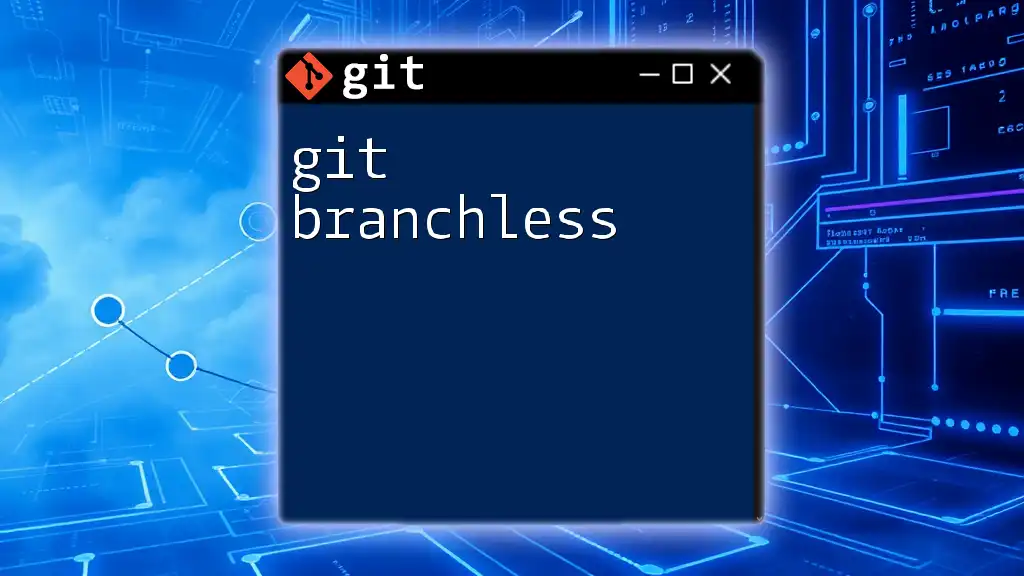
Branching Strategies
Feature Branching
Feature branching is a workflow where each new feature is developed within its own branch. This allows developers to focus on one feature at a time, minimizing disturbances in the main branch.
Once the feature is complete and tested, it can be merged back into the main development line. This practice encourages disciplined development and improves collaboration.
Git Flow
Git Flow is a popular branching model that structures your workflow around specific branches:
- `main`: A stable branch for production-ready releases.
- `develop`: An integration branch for features and fixes.
- `feature/`: Dedicated branches for new features.
- `release/`: For preparing new releases.
- `hotfix/`: For urgent fixes based directly off the production code.
This structured approach simplifies release management and facilitates continuous development cycles.
Trunk Based Development
Trunk Based Development emphasizes collaboration and rapid integration by having developers work on a single main branch (`trunk`). Developers create short-lived branches for experimental or isolated work, merging them into the trunk regularly.
This methodology minimizes integration challenges and promotes velocity in the development process, making it well-suited for agile teams.
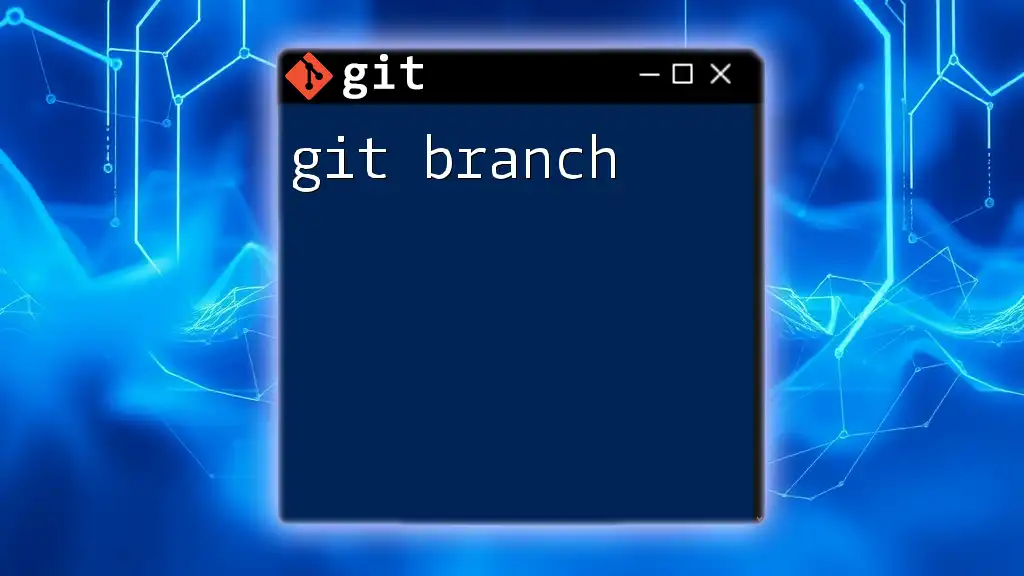
Best Practices for Branching
Naming Conventions
Clear branch names are essential for effective collaboration. Adopt a consistent naming convention to enhance readability and understanding. Common patterns include:
- Feature branches: `feature/<feature-name>`
- Bug fix branches: `bugfix/<fix-description>`
- Hotfix branches: `hotfix/<issue>`
Descriptive names help team members understand the purpose of a branch quickly.
Keeping Branches Up to Date
Regularly integrating changes from the `main` or `develop` branches into your feature branches is critical. This prevents large merge conflicts later on and keeps your branch aligned with the latest updates. Use the following commands:
git fetch origin
git merge origin/main # or 'git rebase origin/main'
Limiting Branch Lifespan
To maintain a clean repository, strive to keep your branches short-lived. This encourages frequent integration of completed features or fixes into the main branch, reducing the risk of conflicts and simplifying project management.
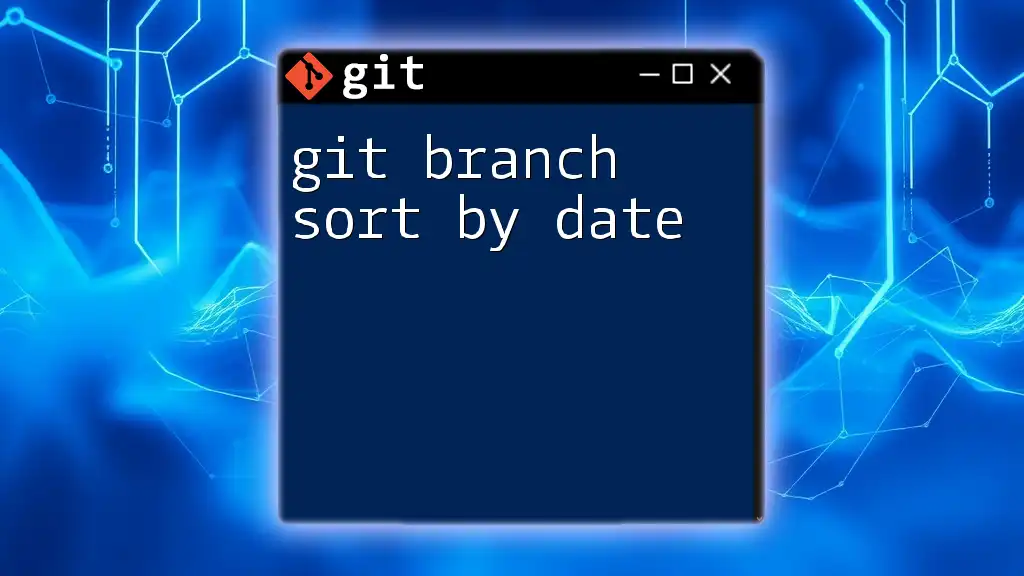
Conclusion
Understanding git branches is vital for effective version control and collaboration in software development. By mastering the key commands, branching strategies, and best practices, you can enhance your workflow and contribute more effectively to your projects. As you implement branching into your development process, remember to follow the outlined practices to maximize efficiency and minimize disruptions. Embrace the power of Git for a more streamlined and productive coding experience.
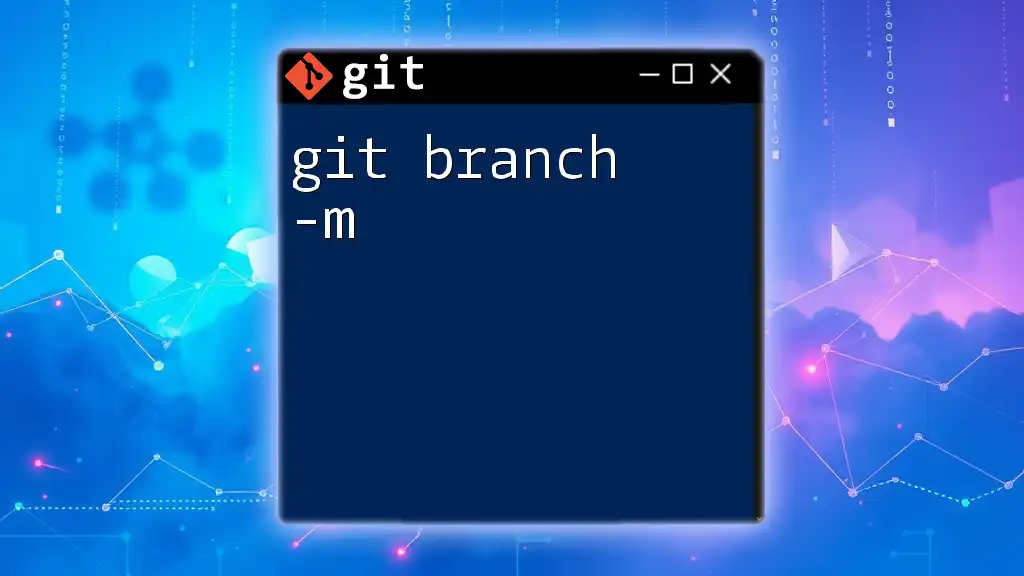
Additional Resources
For further reading, check the official [Git documentation](https://git-scm.com/doc). Additionally, consider exploring tools like SourceTree and GitKraken for graphical interfaces that simplify branch management. You might also look for tutorials that delve into version control systems and best practices in Git for deeper insights.