The `git branch -u` command sets the upstream branch for the current branch to track remote branches easily, allowing you to use simple commands like `git push` and `git pull` without specifying the remote branch each time.
git branch -u origin/feature-branch
Understanding `git branch`
What is a Git Branch?
A branch in Git is a lightweight, movable pointer to a commit. When you create a new branch, you are effectively creating a copy of the working environment that allows you to develop features or fix bugs without impacting the main codebase.
Why Use Branches?
Branches are crucial in collaborative projects as they allow multiple developers to work in parallel without interfering with each other's progress. They facilitate code reviews, experimentation, and streamlined collaboration, ultimately leading to a more organized and efficient development process.
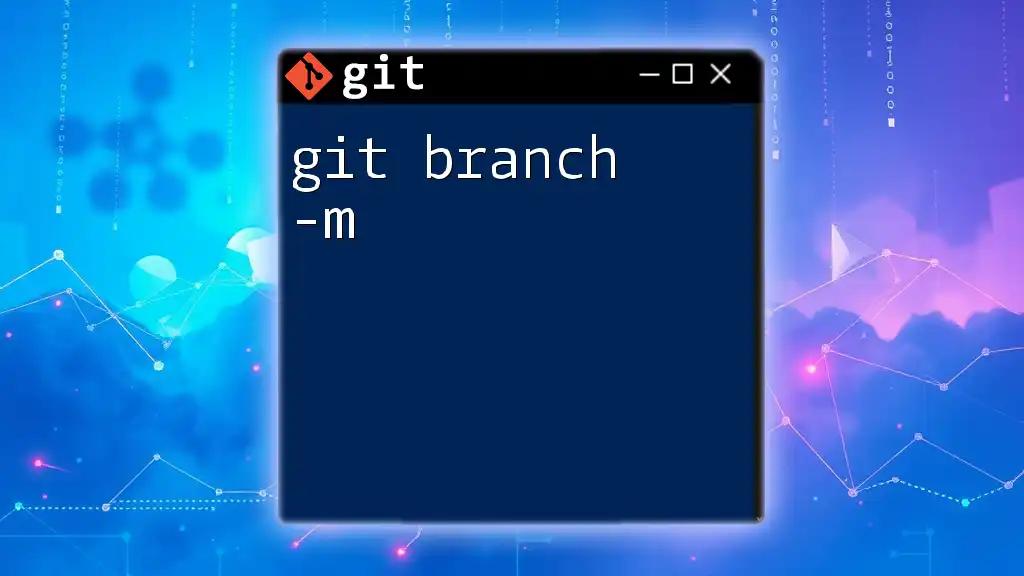
`git branch -u` Command Overview
Definition of `git branch -u`
The `git branch -u` command, also known as `git branch --set-upstream-to`, is used to set the upstream branch for your current local branch. This upstream branch serves as a reference point for Git when performing operations that synchronize your local work with the remote repository.
Importance of Upstream Branches
An upstream branch is the remote branch that your local branch is tracking. It plays a vital role in workflows as it allows you to pull updates from the remote and push your changes back seamlessly. Having a well-defined upstream branch helps avoid confusion, especially when collaborating with others, by ensuring that all parties are on the same page regarding where changes are being pushed.
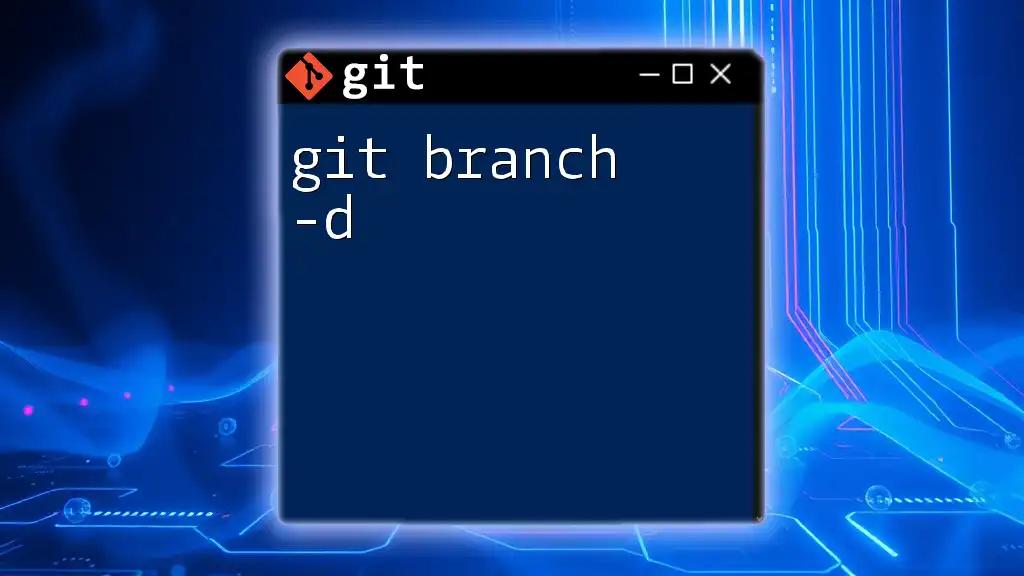
Syntax and Usage
Basic Syntax
The syntax for using the `git branch -u` command is as follows:
git branch -u <remote>/<branch>
Here, `<remote>` represents the name of your remote repository (commonly `origin`), and `<branch>` is the branch you wish to track.
Example Scenario
Imagine you are working on a local branch called `feature`. To set its upstream branch to the `feature` branch on the remote repository, you would execute:
git branch -u origin/feature
This command tells Git to link your local `feature` branch with the `origin/feature` remote branch, enabling you to easily sync changes.
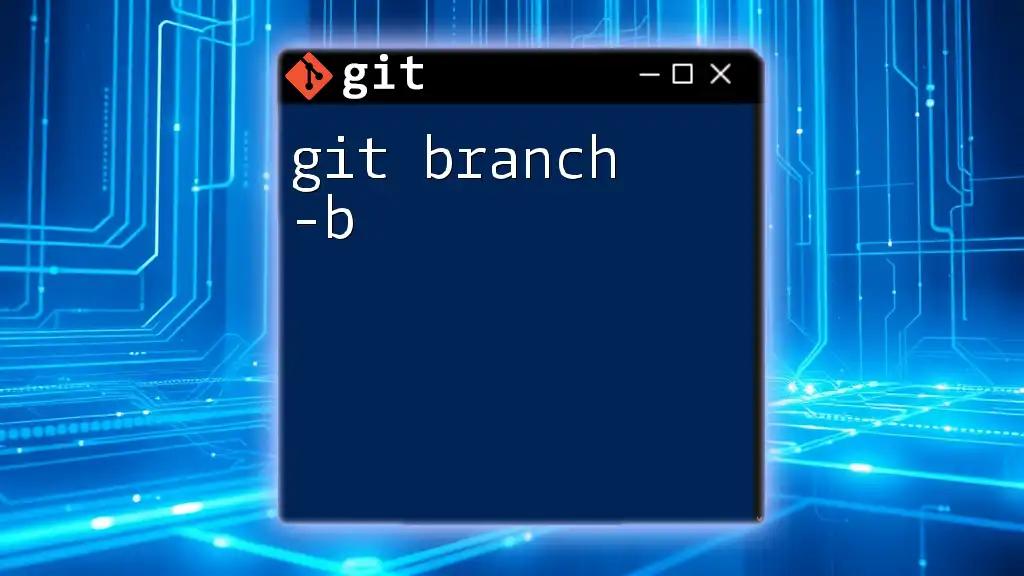
How to Set Up an Upstream Branch
Steps to Follow
To set up an upstream branch, follow these straightforward steps:
-
Ensure you're on the branch you wish to connect to an upstream. You can switch branches using:
git checkout feature
-
Use the `-u` option to specify the upstream branch:
git branch -u origin/feature
Verifying Upstream Branches
To verify that your current branch is correctly tracking its upstream, you can run:
git status
The output will show information regarding the branch you are on and its tracking status, confirming that it is linked to the intended upstream branch.
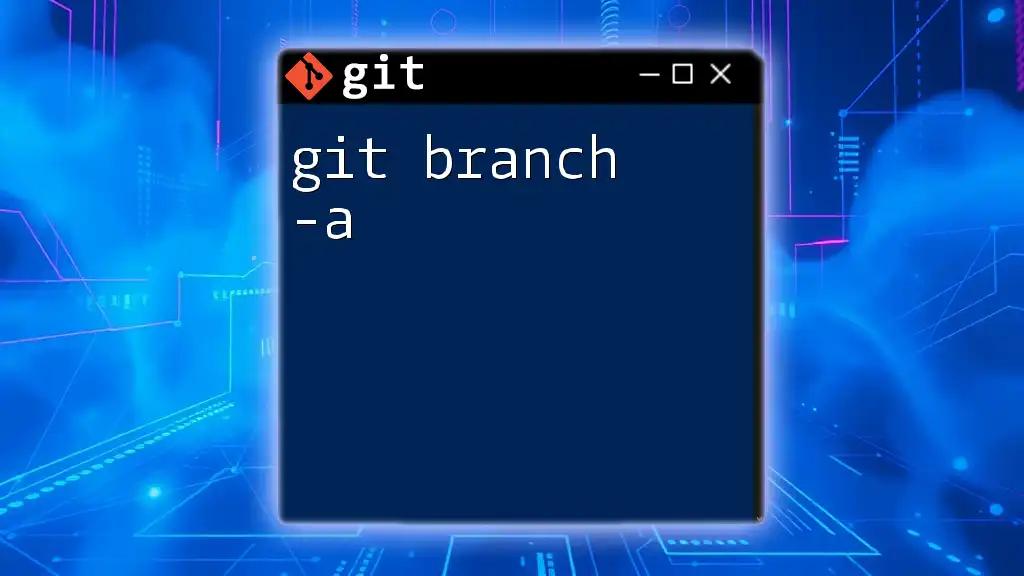
Common Use Cases
Scenario 1: Creating a New Local Branch
When you create a new local branch, it's beneficial to set its upstream branch immediately. Here’s how to do this:
git checkout -b new-feature
git push -u origin new-feature
The `git push -u` command sets the upstream automatically with the first push, linking the remote branch to your new local branch.
Scenario 2: Switching Between Branches
When frequently switching between branches, you might want to ensure that each branch has a correctly set upstream. To switch to an existing feature branch and update its upstream:
git checkout existing-feature
git branch -u origin/existing-feature
This practice helps maintain clarity regarding where your changes are being directed.
Scenario 3: Working with Multiple Remotes
In situations where you have multiple remotes set up (e.g., `origin` and `upstream`), you can specify the remote explicitly when setting an upstream branch:
git branch -u remote-name/branch-name
This command allows you to define exactly where you want to push your commits, which is particularly useful in collaborative environments or open-source projects.
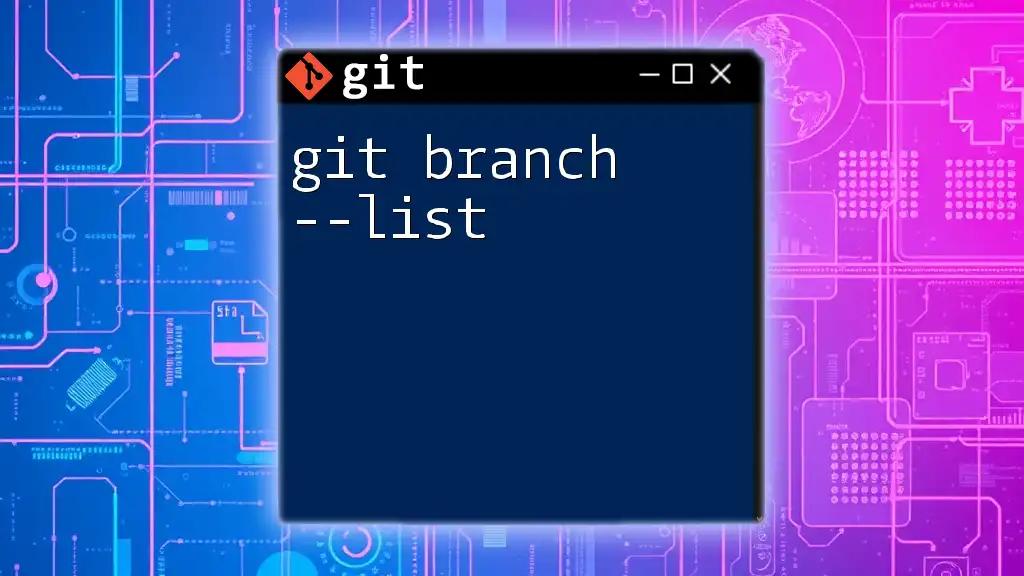
Troubleshooting Common Issues
Issue 1: Error Messages
You may encounter some common error messages when using `git branch -u`, especially if the branch name you specified does not exist. For instance, an error like `fatal: Unknown upstream` indicates that the branch you referenced does not exist on the specified remote. Double-check the branch name and remote spelling to resolve this.
Issue 2: Detached HEAD State
If you find yourself in a detached HEAD state, it means that you are not on a branch that corresponds to a commit. This can happen if you check out a specific commit or tag. To resolve this issue, either create a new branch from this state or check out an existing branch using:
git checkout branch-name
After that, you can set up the upstream branch as previously described.
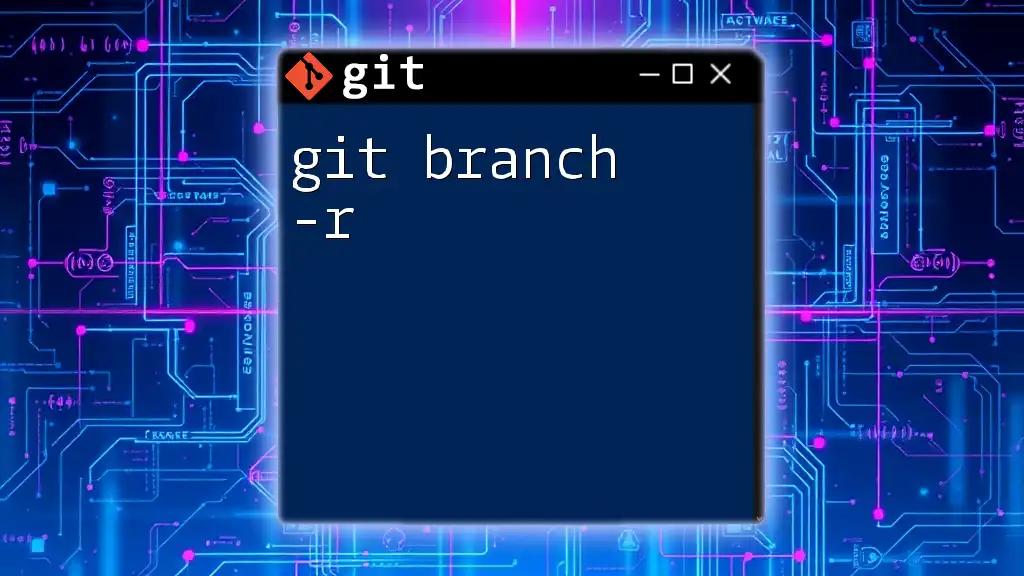
Best Practices for Using `git branch -u`
Regularly Update Upstream Branches
Keeping your upstream branches updated is essential for effective collaboration. Regularly fetch and merge changes from the upstream repository to minimize conflicts:
git fetch origin
git merge origin/branch-name
Maintain Clear Naming Conventions
Utilizing clear and descriptive names for branches can significantly reduce confusion. For instance, instead of vague names like `temp`, consider using `feature/login-page` or `bugfix/issue-123`.
Use Descriptive Commit Messages
Whenever you commit changes to your branch, use descriptive commit messages that indicate the purpose of the changes. This practice not only helps you recall your work later but also aids your collaborators in understanding the context of your changes.
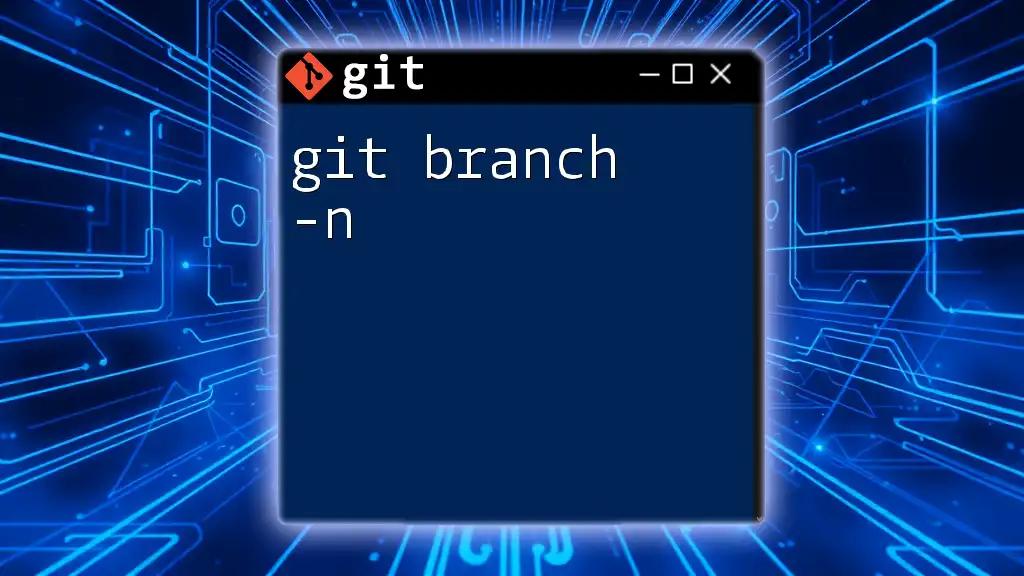
Conclusion
The `git branch -u` command is a powerful tool for managing branch relationships in Git. By setting upstream branches, you can streamline your workflow, enhance collaboration, and maintain clarity in your development processes. Practicing using this command will significantly enhance your Git skills and confidence.
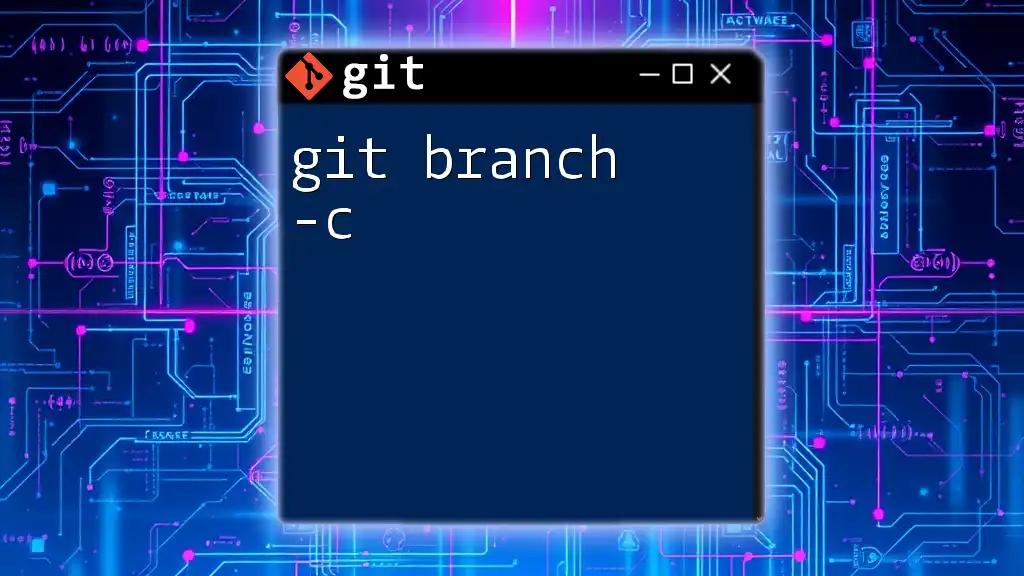
Additional Resources
Recommended Readings
To dive deeper into Git, refer to the [official Git documentation](https://git-scm.com/doc) for more comprehensive guides and tutorials.
FAQs
As you begin to use the `git branch -u` command, it’s common to have questions. Don't hesitate to seek clarification on concepts you're unsure about! Exploring community forums or GitHub discussions can also provide valuable insights.