The `git branch --list` command displays a list of all the branches in your repository, highlighting the current branch with an asterisk.
git branch --list
What is `git branch --list`?
The command `git branch --list` is an essential tool in Git that allows users to view all the branches in a repository. It serves as a way to get a snapshot of the current state of your branches, helping you navigate through your project efficiently. By using this command, you can keep track of which branches exist and assist with management in collaborative settings.
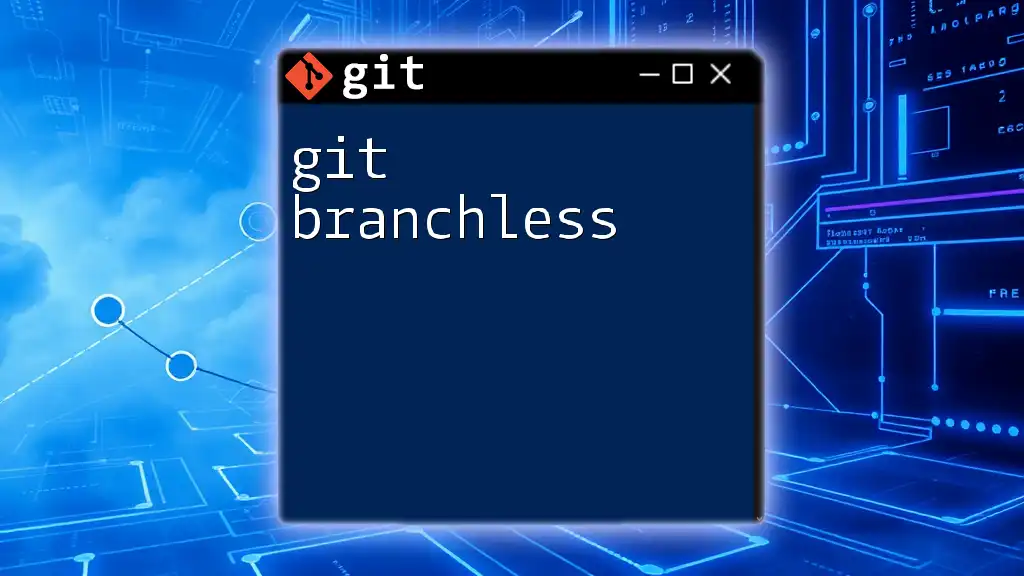
Understanding Branches in Git
What is a Git Branch?
A branch in Git represents an independent line of development. It's a fundamental part of the version control system that allows you to isolate changes, work on features without affecting the main codebase, and ultimately help with collaboration among multiple developers. Branches keep your different workflows organized, enabling seamless integration once the features are complete.
Types of Branches
Default Branch: The main branch where the stable project code resides is typically called `main` or `master`. This is where releases and production code usually live.
Feature Branches: When working on new features, developers often create feature branches that allow for isolated development. Once the feature is complete, it can be merged back into the main branch.
Release Branches: Used in preparing for a new release, these branches allow teams to finalize a feature before it goes live, often with critical fixes or necessary changes.
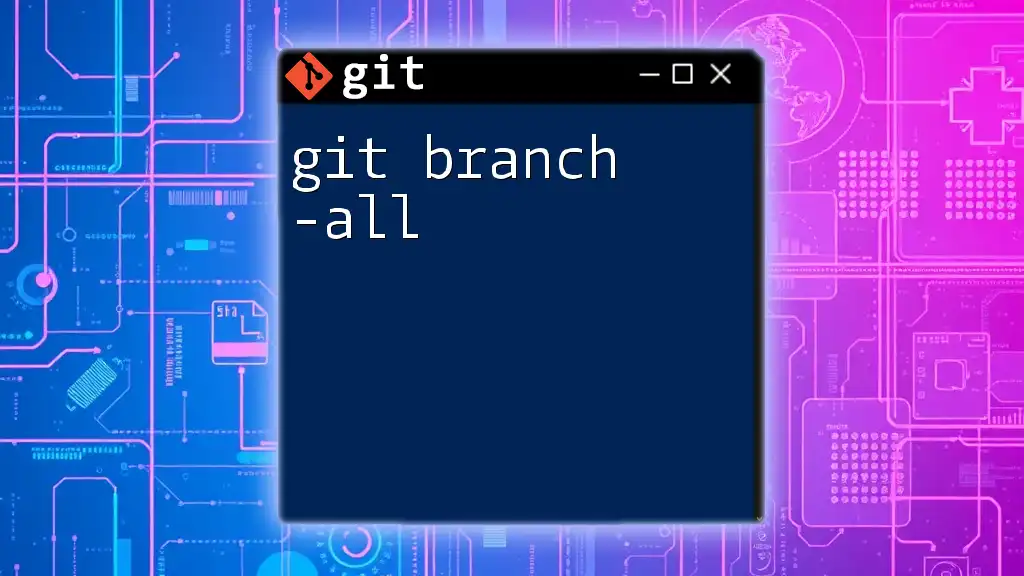
Using `git branch --list`
Syntax of the Command
The basic syntax of the command is as follows:
git branch --list [pattern]
The `[pattern]` is optional and allows you to filter the branches you wish to see, using wildcards as necessary.
Examples of the Command
Listing All Local Branches
To list all your local branches, simply run:
git branch --list
The output will display all branches in the repository, with an asterisk (*) next to the current branch you are on. This helps you quickly see which branch you are working within the context of your code.
Filtering Branches Using Patterns
Sometimes, you may need to focus on specific branches, particularly in large repositories. The following command lists only feature branches:
git branch --list "feature/*"
In this example, the wildcard (*) acts as a placeholder for any characters that follow "feature/", allowing you to find branches associated with specific features or topics.
Comparing with Other Branch Listing Commands
`git branch`
Using `git branch` alone will give you a list of all local branches, which is almost identical to `git branch --list` but without the option to filter by pattern. It is a more general command but serves the same purpose of displaying current branches.
`git branch -a`
To view both local and remote branches, you can run:
git branch -a
This command is especially useful for keeping track of branches that other team members are working on, providing a broader perspective of the project’s development landscape.
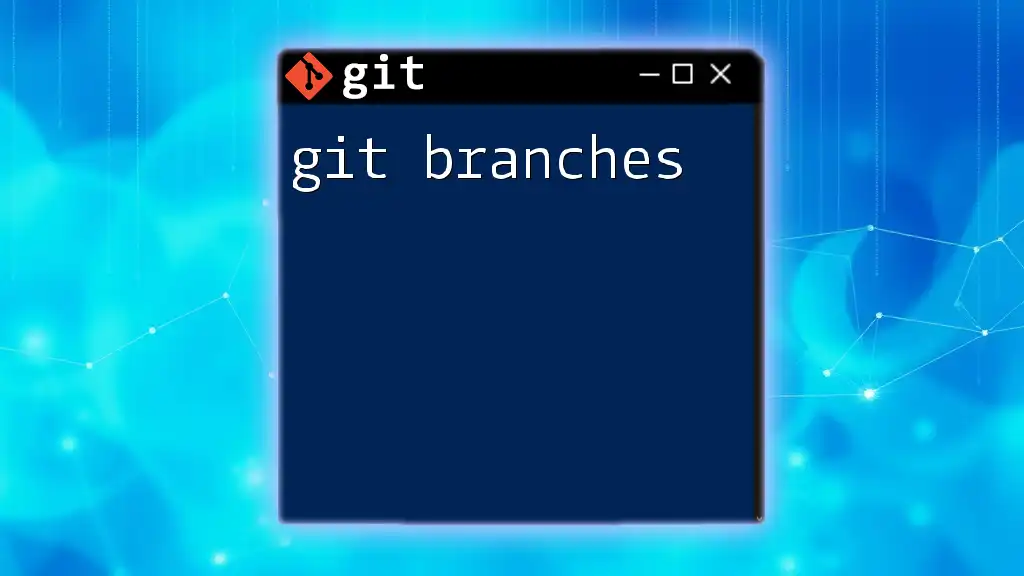
Additional Options and Flags
Using `--merged` and `--no-merged`
These additional flags enhance the `git branch --list` command by showing you merged or unmerged branches.
- Listing Merged Branches: To see branches that have been merged into your current branch, use:
git branch --list --merged
This is useful for identifying branches that can be safely deleted after merger.
- Listing Unmerged Branches: Conversely, if you want to see branches that have not yet been merged, you can run:
git branch --list --no-merged
This helps you identify branches that may still hold important changes that need to be integrated.
Short-Hand Command
For those who favor brevity, `git br --list` can be employed as a shorthand to achieve the same result. This can speed up your workflow, especially for frequent use.
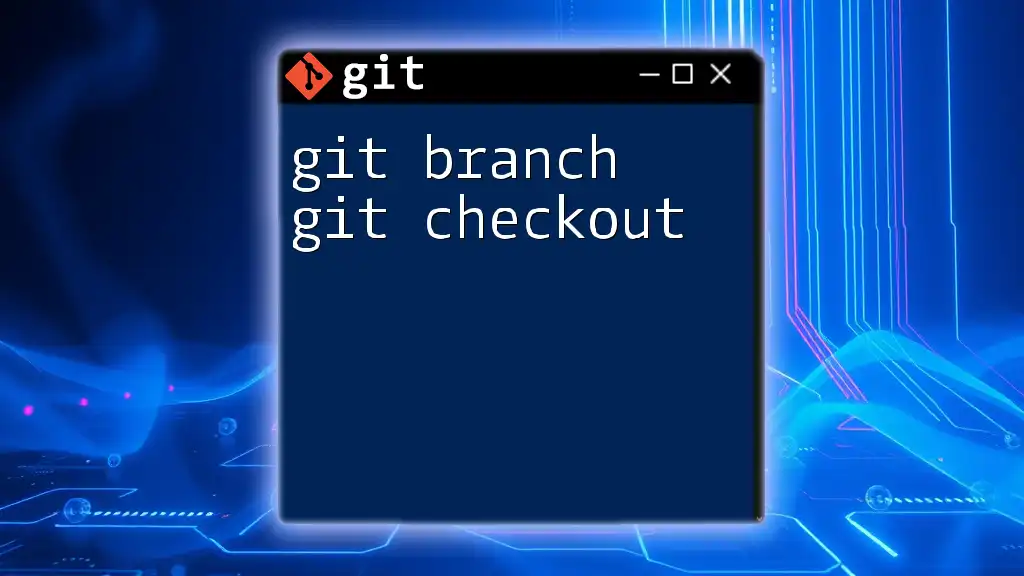
Common Use Cases for `git branch --list`
-
Listing branches to check for completion: Before finalizing a feature or merging, knowing the status of branches helps in planning integration strategies.
-
Pre-checking before merging: It provides insights into existing work, reducing the chance of multiple developers working on similar branches or inadvertently merging incomplete features.
-
Supporting code reviews with branch visibility: Clear branch management supports better collaboration and code reviews, ensuring all team members are aware of ongoing work.
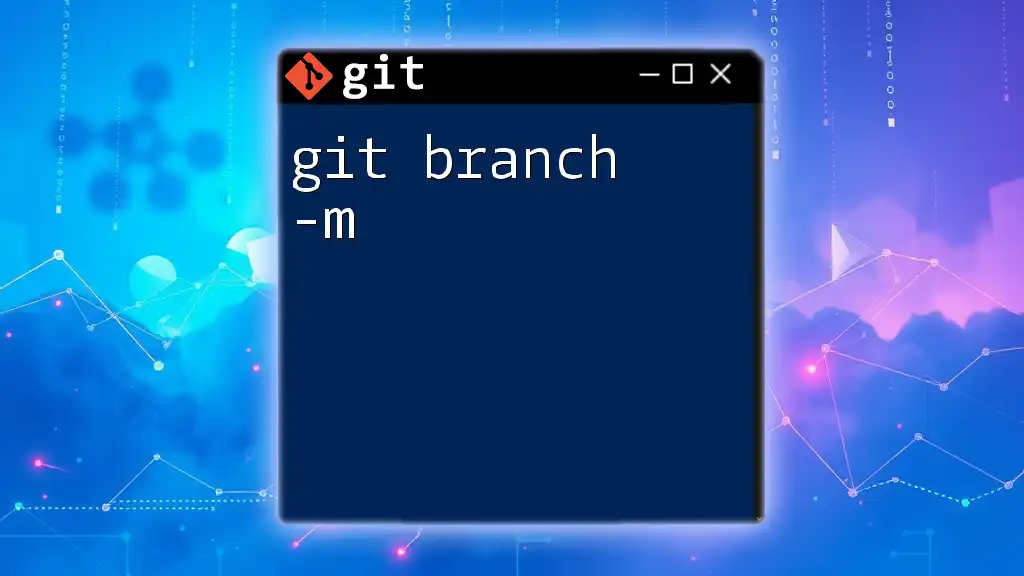
Best Practices for Branch Management
Naming Conventions for Branches
Establishing meaningful and consistent naming conventions for branches is crucial for clarity. A good branch name can convey its purpose at a glance. For instance, using prefixes like `feature/`, `bugfix/`, or `hotfix/` can help categorize your branches.
Regularly Cleaning Up Old Branches
Often, old branches accumulate in a repository, which can lead to confusion. Regularly reviewing and removing stale branches is essential. To delete a branch after you confirm it's no longer needed, you can use:
git branch -d branch-name
This command ensures your list remains manageable and organized.
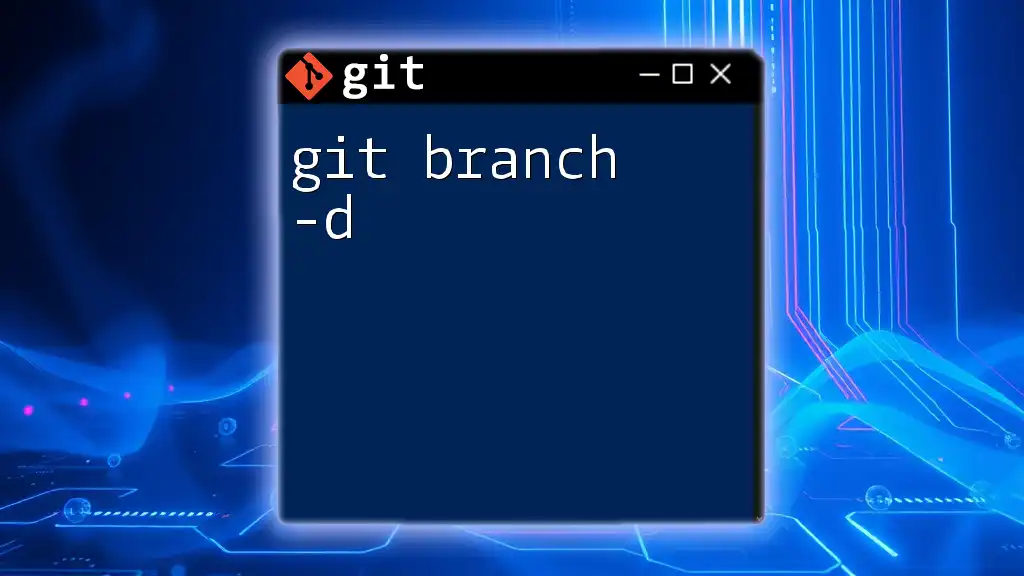
Conclusion
The `git branch --list` command is a powerful and essential tool for any developer working within Git. By effectively managing branches and utilizing the insights this command provides, you can enhance your productivity and maintain a cleaner codebase. Make it a practice to familiarize yourself with this command and the broader implications of effective branch management—your future self will thank you.
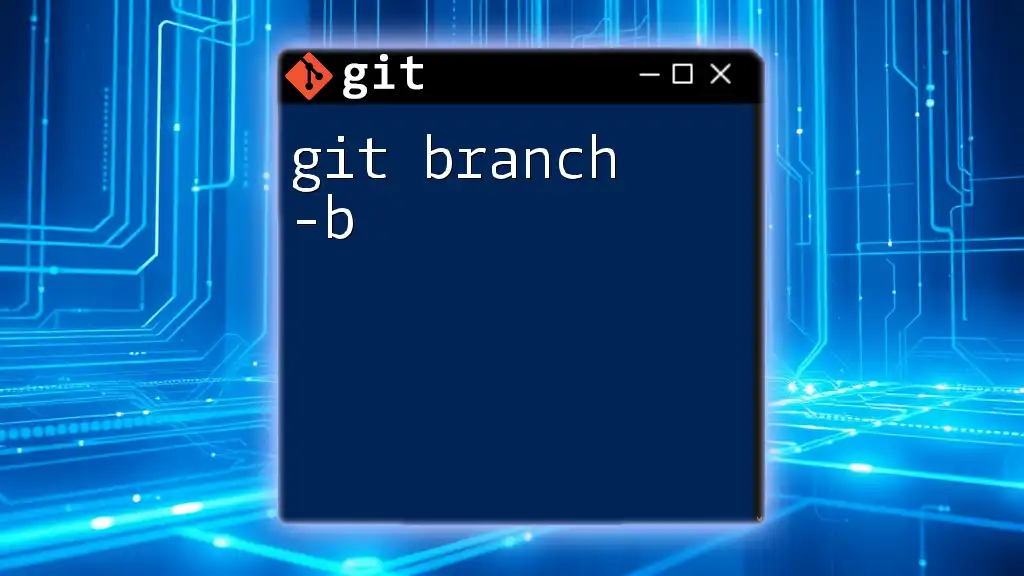
Additional Resources
For further reading, check out the official Git documentation and look for online Git tutorials that can deepen your understanding of these concepts. Tools for managing your Git workflow can also assist you with visualizing branches and enhancing your productivity.
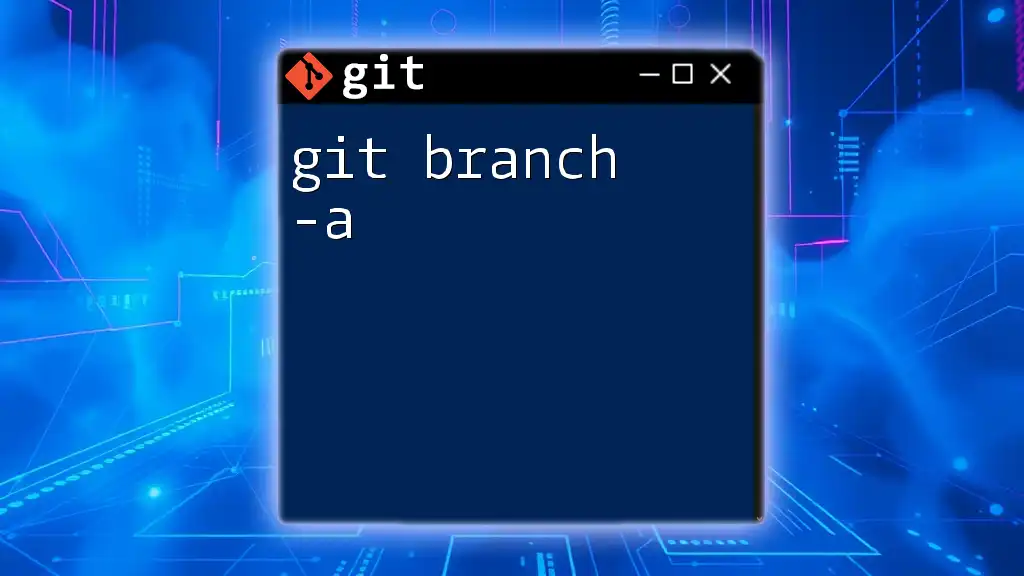
FAQs
What happens if I don't see my branch listed?
It could mean that the branch is either deleted, out of scope due to merging, or you are not currently on the correct repository or remote.
Can I list remote branches using `git branch --list`?
No, this command lists only local branches. To see remote branches, you can use `git branch -a`.
How do I create a new branch after listing?
To create a new branch, simply use the command:
git checkout -b new-branch-name
This allows for instantaneous creation and switching to the new branch.