The `git branch` command is used to create, list, or delete branches, while `git checkout` is used to switch between branches or restore working tree files.
Here’s how you can use these commands:
# Create a new branch called "feature-branch" and switch to it
git checkout -b feature-branch
# List all branches
git branch
# Switch back to the main branch
git checkout main
Understanding Git Branches
What is a Git Branch?
A Git branch is essentially a separate line of development in your project. It allows you to diverge from the main line of development and work on changes independently. Think of it as a way to isolate your work from the rest of the project until you are ready to merge it back.
Unlike traditional version control systems that handle file versions linearly, Git's branching model allows for multiple simultaneous lines of development. This flexibility is one of the greatest strengths of Git, enabling parallel work on different features, bug fixes, or experimental changes without affecting the main codebase.
How Branching Works
When you create a branch in Git, you are creating a pointer to a specific commit. From this point, you can make changes and commit them to this new branch without altering the original branch's history. This creates a visual tree structure, where branches can diverge, converge, and even be abandoned—all while keeping your main project history clean.
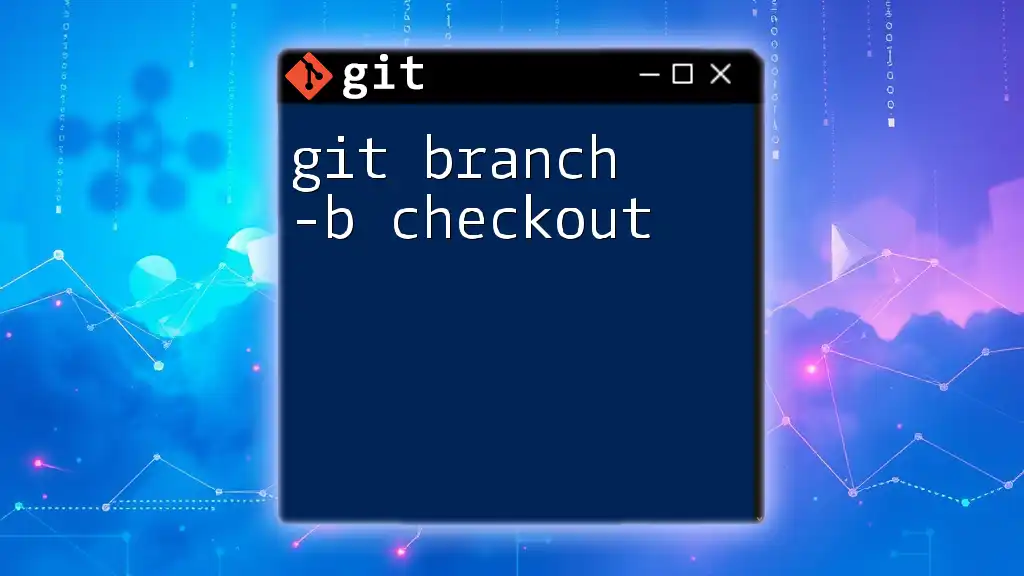
Creating a New Branch
Command: `git branch [branch-name]`
To create a new branch, you can use the command:
git branch feature-xyz
This command generates a new branch named feature-xyz based on your current branch's snapshot. However, it's important to note that creating a branch alone does not switch to it; you remain on the current branch unless you explicitly change.
Creating branches helps in organizing your work, allowing you to tackle different tasks or features in isolation. Make sure to choose a descriptive branch name that clearly indicates the purpose, such as "feature-login", "bugfix-header-issue", or "experiment-new-ui".
Best Practices for Naming Branches
Having a standardized naming convention is crucial. Consider the following best practices for naming your branches:
- Prefix branch names by their purpose, such as `feature/`, `bugfix/`, or `hotfix/`.
- Include a short description of the changes or issue being addressed.
- Maintain consistency across your team to enhance collaboration and readability.
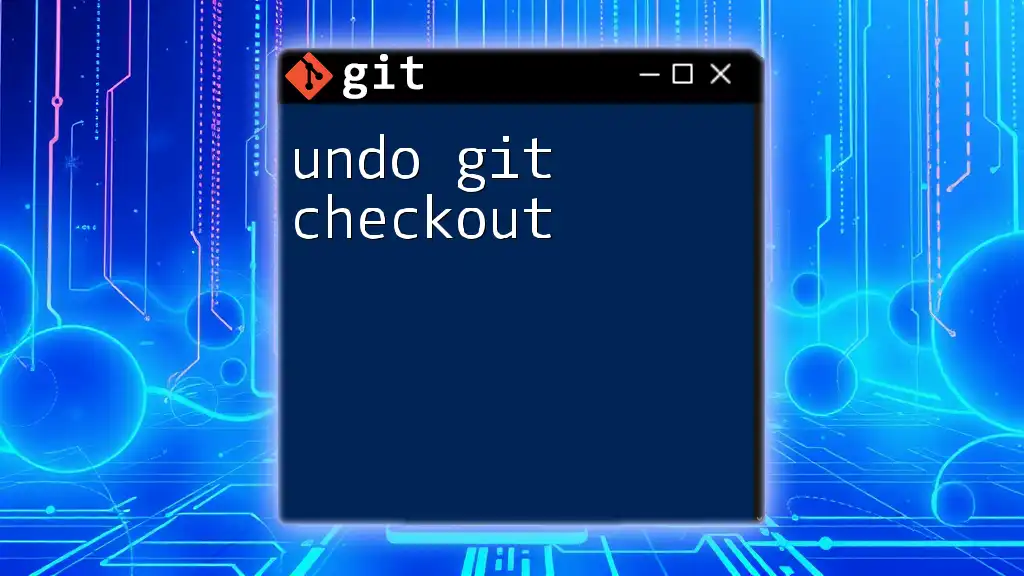
Switching Between Branches
Command: `git checkout [branch-name]`
To switch to a different branch, you can use the command:
git checkout feature-xyz
This command tells Git to update your working directory to the latest commit in the feature-xyz branch. If the command executes successfully, you can start working on this branch immediately.
However, before switching between branches, ensure that you have saved all your changes. If there are uncommitted changes in the current branch that could conflict with the target branch, Git will prevent the switch to avoid potential data loss.
Alternative Command: `git switch`
Introduced in newer versions of Git, the `git switch` command offers a more intuitive way to change branches. You can simply use:
git switch feature-xyz
Unlike `git checkout`, `git switch` is focused solely on changing branches, minimizing confusion with other functionalities. It can improve workflow speed and efficiency.
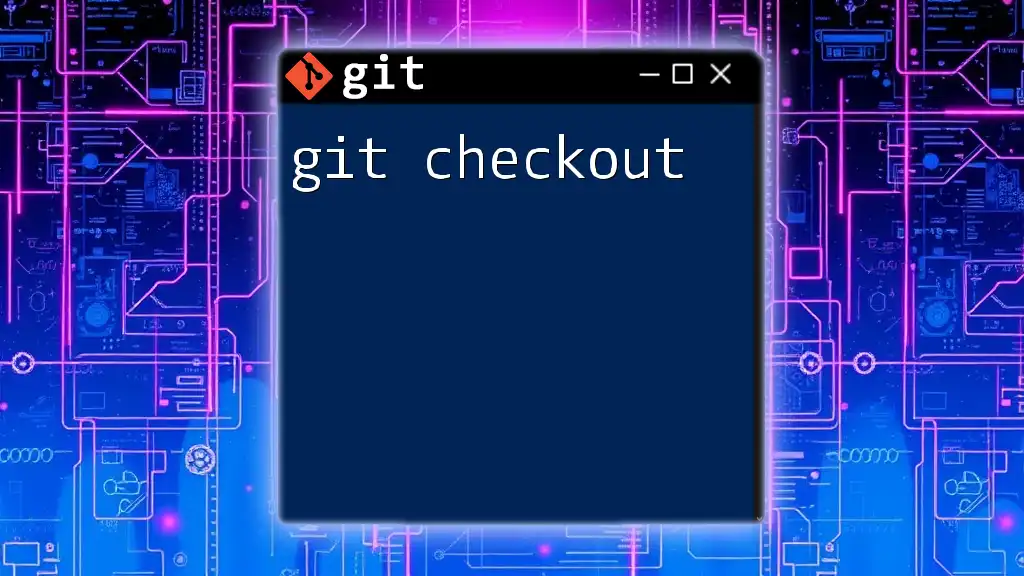
Working with Multiple Branches
Viewing All Branches
To get an overview of all branches in your project, use:
git branch
This command lists both your local branches and highlights the branch that you are currently on. Understanding the structure of your branches helps in effective project management.
Deleting a Branch
Sometimes, branches become obsolete or after merging, they may no longer be needed. To safely delete a branch, you can use:
git branch -d feature-xyz
This command deletes the branch feature-xyz only if it has been fully merged into its upstream branch. If the branch contains unmerged changes, Git will raise a warning. Use `-D` to forcefully delete it, but this should be done with caution, as it can lead to loss of work.
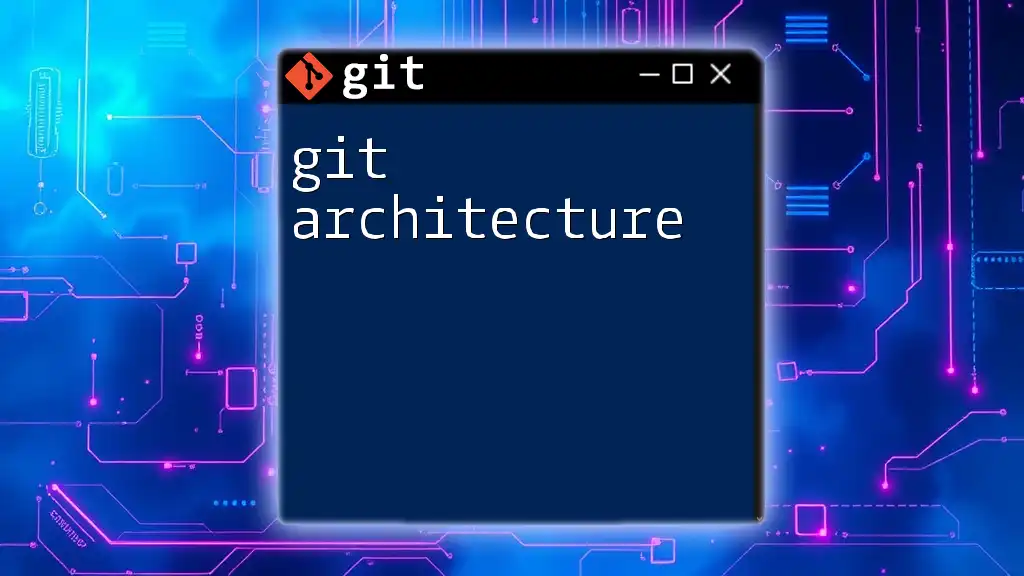
Merging Branches
Overview of the Merge Process
Merging is the process of integrating work from different branches. When a feature branch is complete, it should be merged back into the main branch (often called main or master) to ensure that the latest changes become part of the project.
Command: `git merge [branch-name]`
To merge a branch back into your main branch, first switch to the main branch:
git checkout main
Then, execute the merge command:
git merge feature-xyz
This command integrates the changes made in feature-xyz into the main branch. If there are no conflicts, the merge will complete automatically, creating a new commit that reflects the combined work.
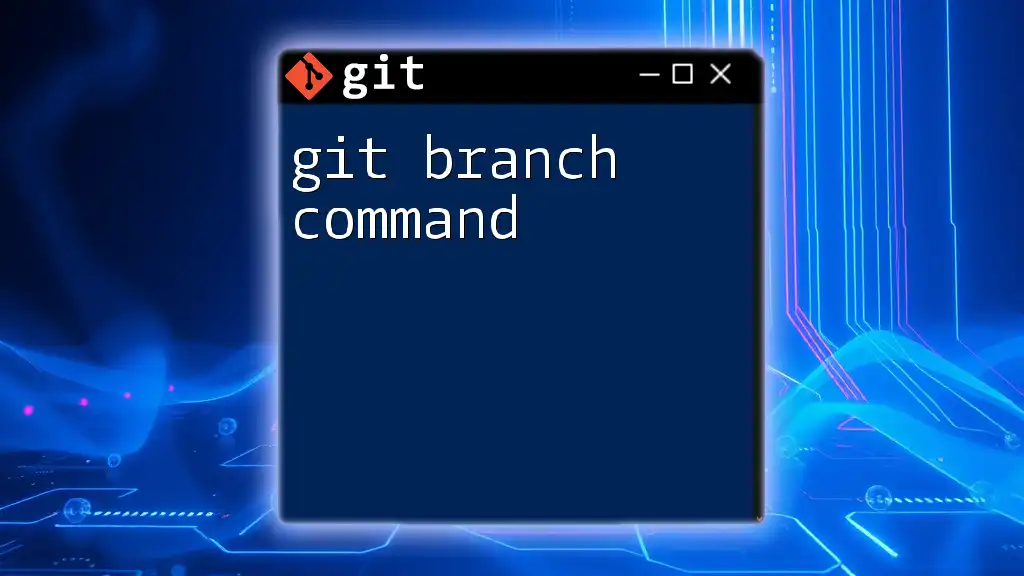
Troubleshooting Common Issues
Conflicts During Merge
Merge conflicts happen when two branches have made incompatible changes to the same part of the code. Git will alert you of a conflict, which must be resolved manually. Follow these steps to resolve:
- Open the files that have conflicts.
- Locate the conflict markers (e.g. `<<<<<<< HEAD`, `=======`, `>>>>>>> feature-xyz`).
- Make the necessary changes to integrate both sets of changes.
- Once resolved, add the changes and complete the merge:
git add conflicted-file.txt
git commit
Switching Branches with Uncommitted Changes
When you try to switch branches while having uncommitted changes, Git may refuse to do so. To manage this risk, consider the following solutions:
- If you want to keep your changes and switch branches, use git stash to temporarily remove your changes:
git stash
git checkout feature-xyz
git stash pop
This command sequence stashes uncommitted changes, switches branches, and then re-applies the changes back into the working directory, allowing you to continue your work seamlessly.
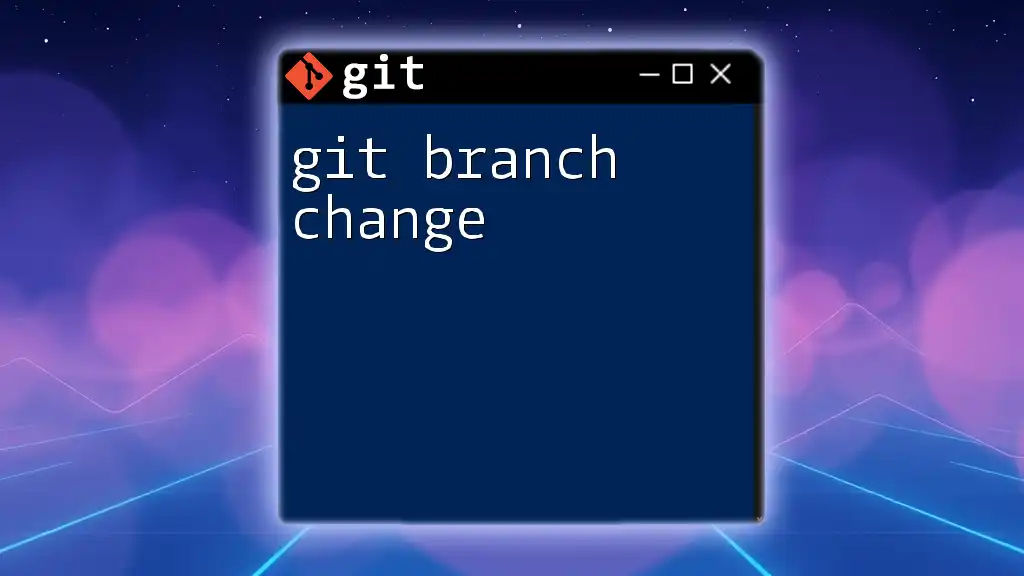
Conclusion
In this guide, we explored the essential commands and practices surrounding git branch and git checkout. By mastering branching and switching techniques, you empower your collaborative workflows and enhance project management. Implement these practices to increase your proficiency with Git and streamline your development processes.