The `git checkout -b` command is used to create a new branch and switch to it in a single step.
git checkout -b new-branch-name
Understanding Git Command Basics
What is Git?
Git is a powerful version control system designed to help developers track changes in their code over time. Its decentralized nature makes it perfect for collaborative projects, allowing multiple developers to work simultaneously without losing track of changes. Git is crucial for maintaining the integrity of your codebase, ensuring that everyone can contribute effectively.
The Role of Git Commands
Git commands are the core functionalities that allow you to interact with your repositories. These commands are executed in a command-line interface (CLI) where you can manage branches, commits, merges, and much more. Proper knowledge of these commands empowers developers to handle version control intuitively and efficiently.
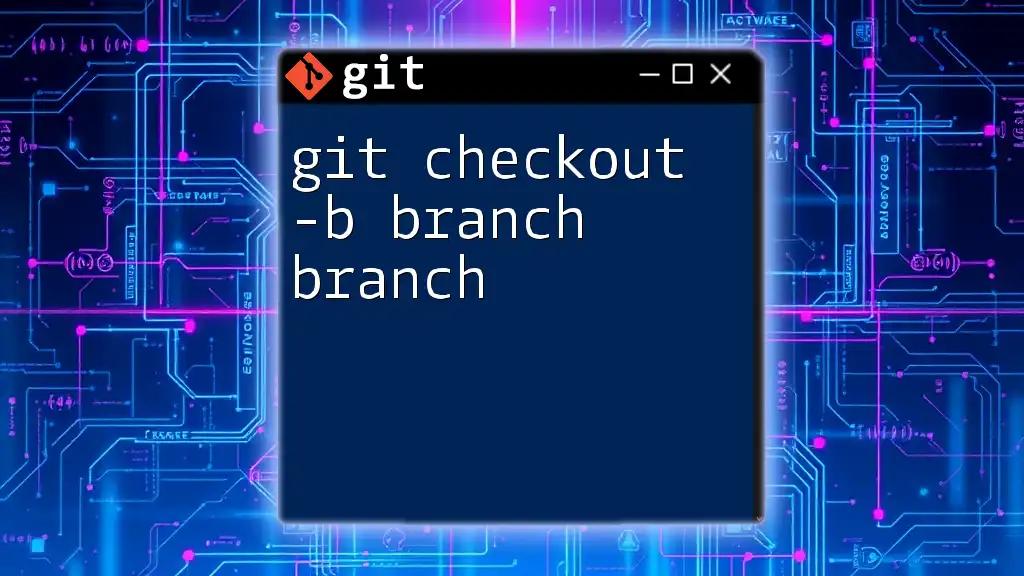
Introduction to `git checkout`
What is `git checkout`?
The `git checkout` command is a versatile tool in Git, primarily used to switch between branches or to restore working tree files. This command allows you to navigate your project's history or revert files back to a previous state. It’s important to distinguish between `git checkout` and the newer `git switch` command, which is designed specifically for switching branches.
When to Use `git checkout`
You should consider using `git checkout` when you need to switch to a different branch to work on separate features or fixes. It is also essential when you want to restore files in your working directory to their last committed state. Understanding these scenarios will enhance your collaboration and help maintain a clean project history.
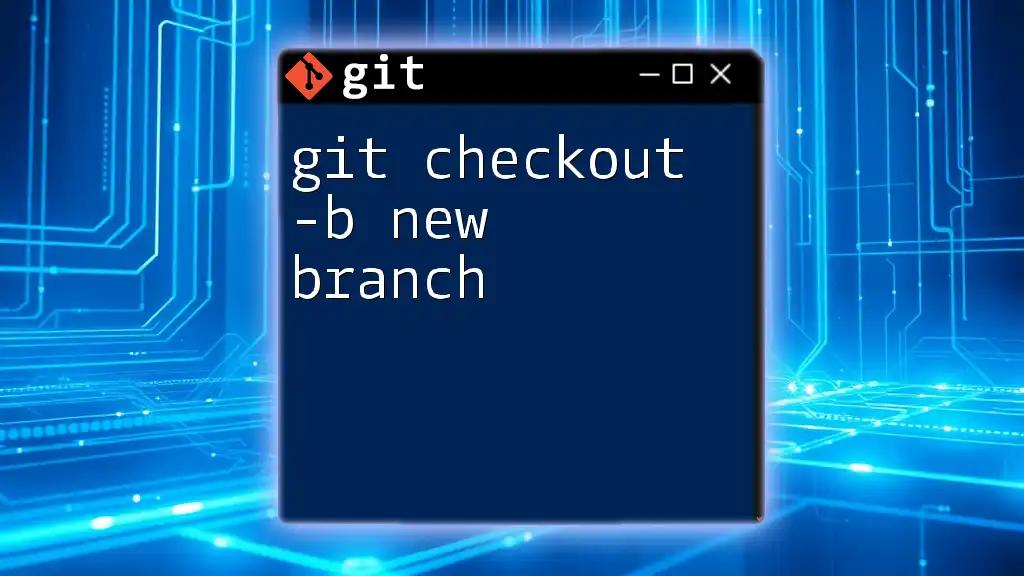
The Functionality of `git checkout -b`
What Does `git checkout -b` Do?
The `-b` flag in the `git checkout` command is a shortcut for creating a new branch while simultaneously checking it out. Instead of using two separate commands, you can streamline the process into one. This command is particularly helpful when initiating new features, as it saves time and reduces the likelihood of errors.
Syntax of `git checkout -b`
The basic syntax for the command is as follows:
git checkout -b <branch_name>
Here, `<branch_name>` should be replaced with your desired name for the new branch. This combination is efficient and commonly used by developers to manage branches effectively.
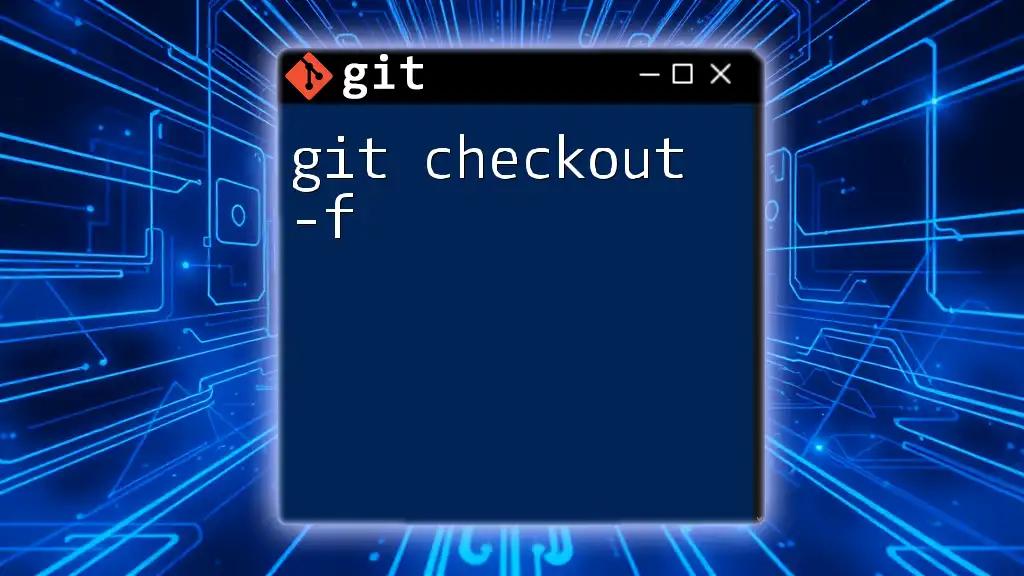
Creating a New Branch Using `git checkout -b`
Step-by-Step Process
To create and switch to a new branch using the `git checkout -b` command, follow these simple steps:
-
Ensure you are in the correct repository: Before executing the command, navigate to your repository with:
cd path/to/your/repo
-
Create and switch to a new branch: Once in your repository, execute the following command to create and switch to a new feature branch:
git checkout -b feature/new-feature
This command accomplishes two actions: it creates a branch named `feature/new-feature` and switches your workspace to that branch. Under the hood, Git automatically sets the new branch to track changes, allowing you to start working immediately.
Best Practices for Branch Naming
Naming conventions are crucial in maintaining a clean workflow in Git. When creating new branches, consider using descriptive names that indicate the purpose of the branch—this is particularly beneficial in team environments. For instance, prefixes like `feature/`, `bugfix/`, or `hotfix/` can make it clear what the branch's intent is. Adopting these naming barriers helps avoid conflicts and confusion among team members.
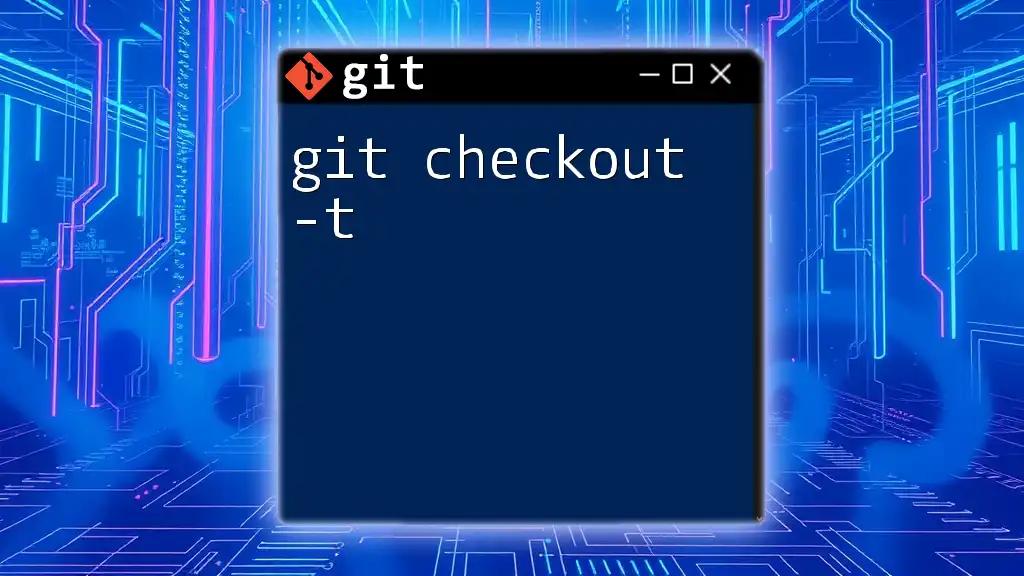
Common Scenarios for Using `git checkout -b`
Working on a New Feature
When you’re assigned to develop a new feature, starting with a new branch is essential. By running:
git checkout -b feature/login-system
you create a dedicated workspace for your feature. This approach helps maintain a clean project history and allows for easier merging once your feature is complete. You can experiment with changes without affecting the primary codebase, ensuring a smoother integration process.
Fixing Bugs
Whenever a bug is identified, it’s best practice to create a dedicated branch for the fix. Running:
git checkout -b bugfix/issue-214
allows you to address the specific issue without disrupting the main branch. You can make necessary corrections, test extensively, and merge back into the main codebase once you’ve resolved the problem. This strategy enhances collaboration by keeping the main branch stable.
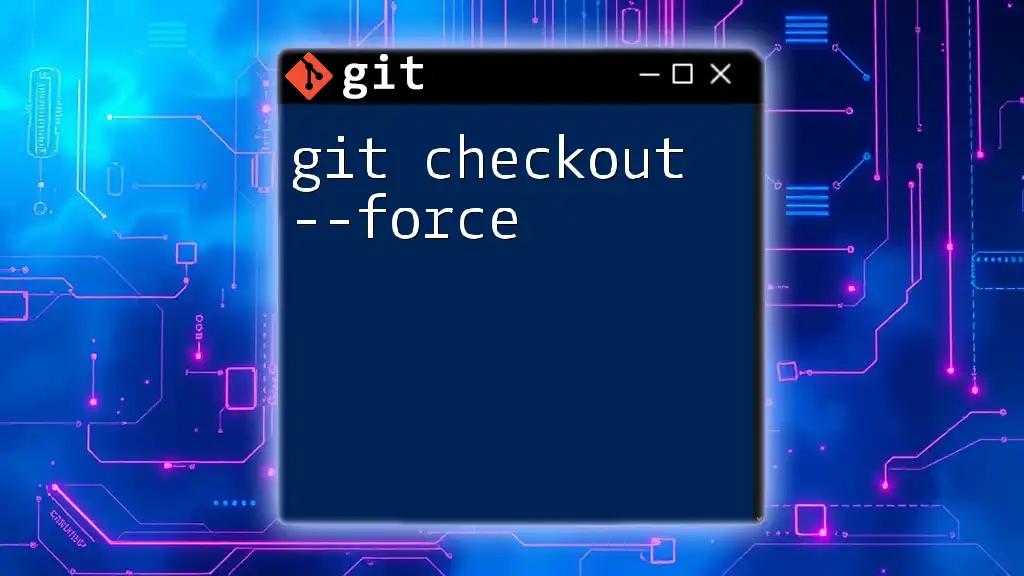
Potential Issues and Troubleshooting
Conflicts and Errors
Sometimes, while attempting to create a new branch, you might encounter errors. A common mistake is trying to create a branch with an already existing name. Git will return a message indicating the conflict, and you’ll need to choose a different name for your new branch. Be mindful of your existing branches by checking with:
git branch
Checking Existing Branches
Understanding your branch structure before creating new branches is vital. You can list all existing branches using:
git branch
This command will display all branch names, helping you select unique identifiers for new ones and avoiding conflicts.
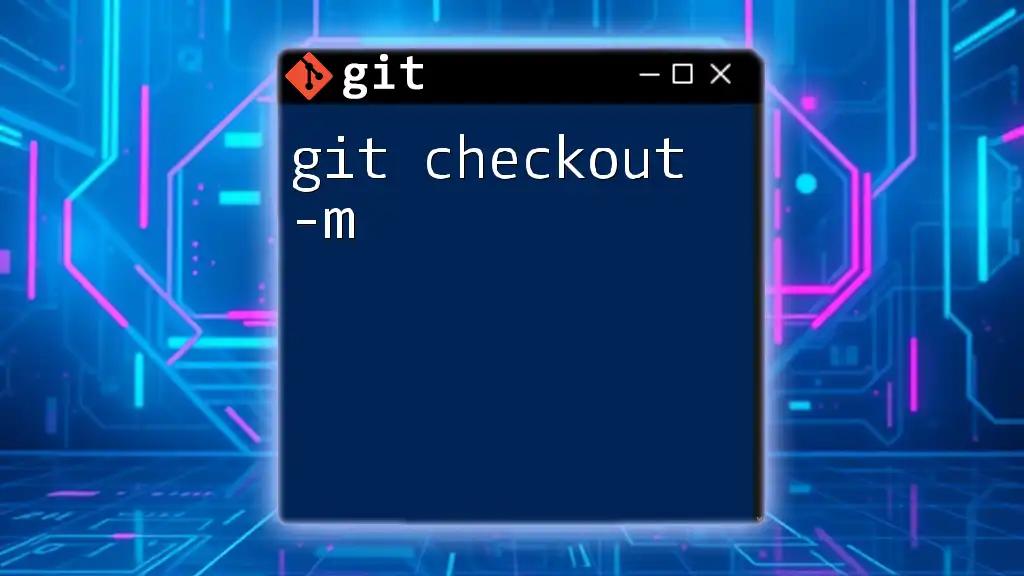
Alternatives to `git checkout -b`
Introducing `git switch`
Git has introduced the `git switch` command as an alternative for ease of use. This command is dedicated to switching branches, enhancing clarity when executing branch-related actions. For instance, you can create and switch to a new branch using:
git switch -b <branch_name>
This alternative is more intuitive for users new to Git, focusing solely on switch functionalities.
Other Branching Techniques
Sometimes you may want to create a branch without immediately switching to it. You can do this using the following command:
git branch <branch_name>
This command creates the branch but keeps you on your current branch. For those who prefer graphical interfaces, various GUI tools are available that simplify branch management, providing a visual representation of your branches and commits.
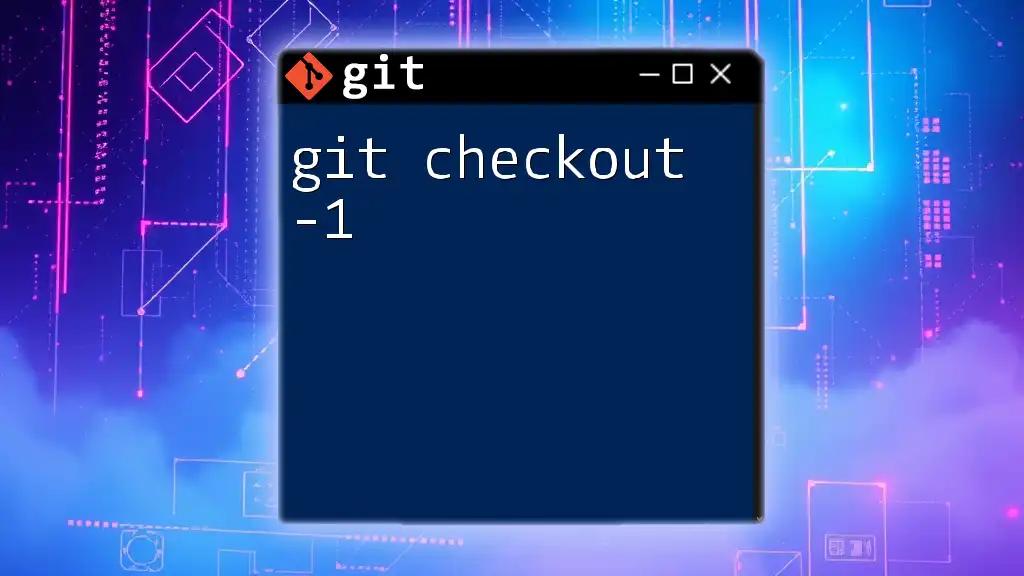
Conclusion
Recap of `git checkout -b`
The `git checkout -b` command is an effective way to create and switch branches in your Git workflow. Understanding how to use this command efficiently will enhance your development process, making it easier to manage features and bug fixes in a collaborative climate.
Call to Action
To deepen your Git knowledge, continue exploring additional commands and best practices. By mastering these tools, you will empower yourself and your team, ensuring seamless collaboration and robust version control in your projects.