The "git checkout error" typically occurs when trying to switch branches or restore files that do not exist in the repository or are incorrectly specified.
Here’s an example of how you might encounter this error:
git checkout non-existent-branch
Understanding Git Checkout
What is Git Checkout?
The `git checkout` command is a fundamental part of using Git for version control. It is primarily used to switch between different branches in your repository or restore files to a previous version. This powerful command allows you to navigate the various states of your project, giving you the flexibility to work on multiple features or bug fixes simultaneously.
Common Use Cases for Git Checkout
There are several typical scenarios where you would use `git checkout`:
- Switching between branches: When you want to work on a different feature or fix a bug, you might switch from the current branch to another branch by using this command.
- Creating a new branch: The `git checkout -b <branch-name>` command is used to create a new branch and switch to it at the same time, allowing for quick feature development.
- Restoring a file: You can revert changes to a file in your working directory to match a specific commit, enabling you to recover from mistakes or unwanted changes.
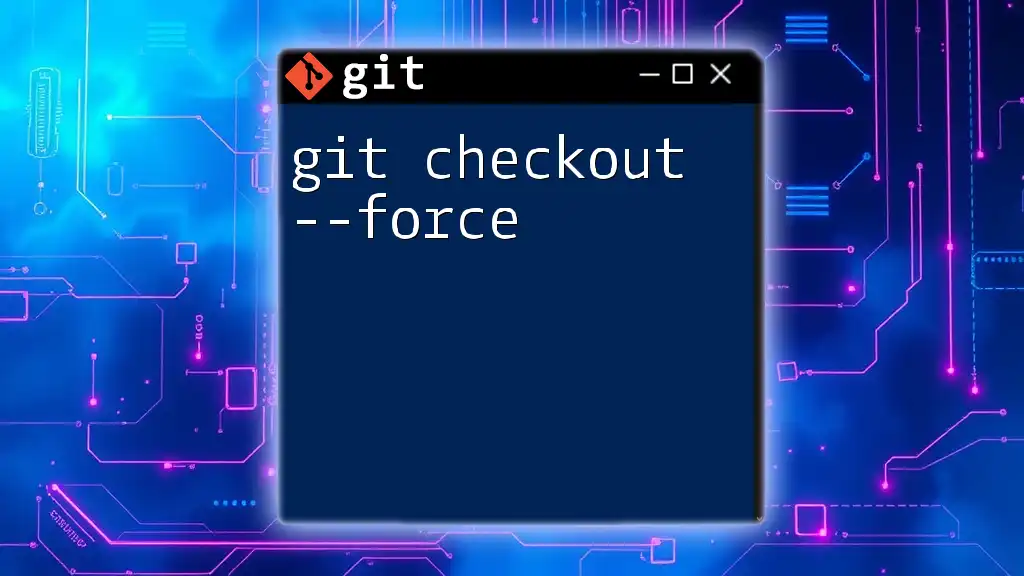
Overview of Checkout Errors
Why Do Checkout Errors Occur?
Errors that arise when using `git checkout` are commonly due to conflicting states in your repository. These errors often occur when you try to switch branches or restore files while there are uncommitted changes in your working directory, or when you reference a branch or file that does not exist. Understanding these potential pitfalls can help you troubleshoot more effectively.
Types of Checkout Errors
Several errors can interrupt your use of `git checkout`. Here are a few common ones:
- Pathspec Error: This occurs when Git cannot find the branch or file you've specified. The error message might read: "error: pathspec '...' did not match any file(s) known to git."
- Uncommitted Changes Error: You might encounter this message: "error: Your local changes to the following files would be overwritten by checkout." This indicates that your working directory has changes that haven’t been committed, which would conflict with the switch.
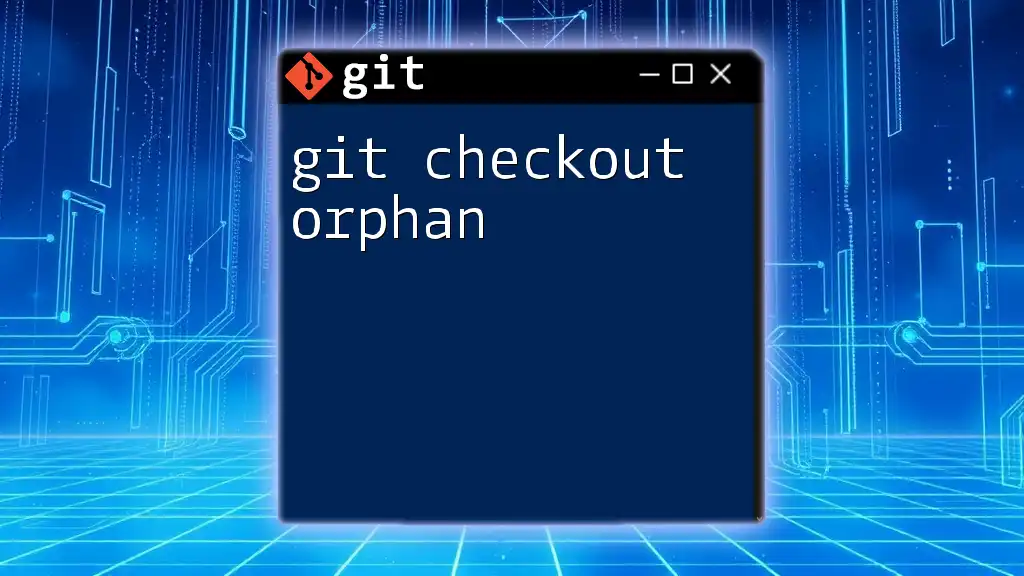
Diagnosing Git Checkout Errors
Identifying the Source of the Error
When you encounter a checkout error, the first step is to pinpoint the source. One effective way is to run the following command:
git status
This command will show you the current state of your branch, any uncommitted changes, and helpful hints about what you can do next. Make sure your working directory is clean before attempting to switch branches or restore files, as uncommitted changes can lead to further complications.
Common Error Messages Explained
Pathspec Error
When faced with a pathspec error, you might have mistyped a branch name or are trying to check out a branch that doesn’t exist. For example, if you attempt to run:
git checkout non-existent-branch
You would see the following error message.
error: pathspec 'non-existent-branch' did not match any file(s) known to git.
Uncommitted Changes Error
If you see an uncommitted changes error, it indicates that you have modified files that conflict with the operation you are attempting. This can occur if you're trying to check out another branch without committing or stashing your changes.
For instance, if you execute:
git checkout another-branch
And have changes that conflict, you will receive the message:
error: Your local changes to the following files would be overwritten by checkout.
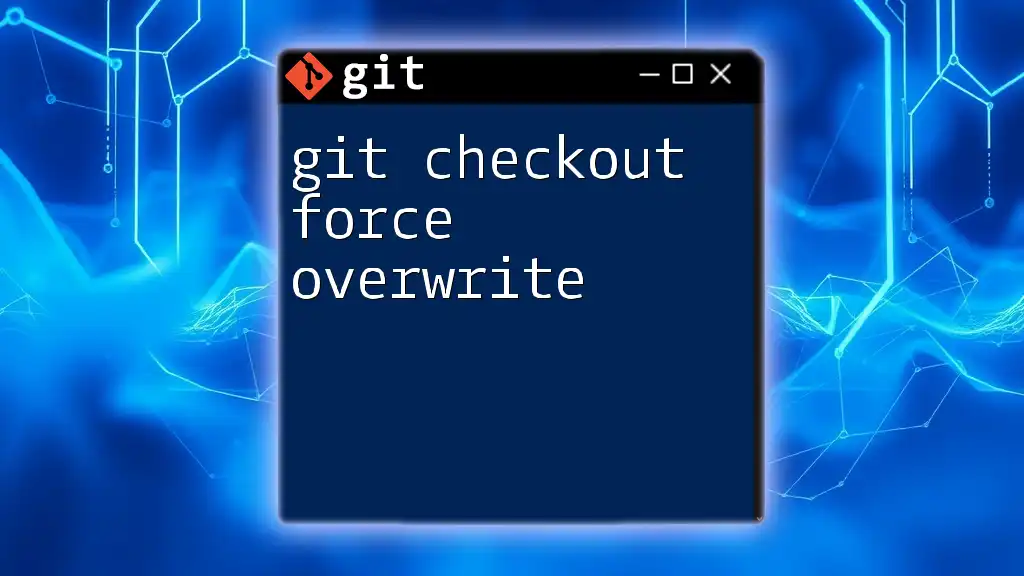
Fixing Git Checkout Errors
Resolving Pathspec Errors
Check for Typos
A simple yet common cause of the pathspec error is a typo in your command. To verify a branch name, you can list all available branches:
git branch -a
By ensuring that you have the correct branch name, you can avoid pathspec errors.
Ensure the Branch Exists
Before checking out a branch, always confirm its existence. If you suspect that it might not be available, use the `git branch -a` command to list all branches, including remote ones. This will help you pinpoint any discrepancies.
Resolving Uncommitted Changes Errors
Committing Changes
If you have changes that you want to preserve, commit them before switching branches. This is done by running:
git add .
git commit -m "Your commit message here"
After committing, you can safely check out another branch.
Stashing Changes
If you want to temporarily set aside changes without committing, you can stash them. Use the following command:
git stash
After stashing, you can switch branches without the risk of losing your changes. To apply your stashed changes later, use:
git stash apply
Other Potential Fixes
Using Force Checkout
In some cases, you may need to force a checkout, especially when you are certain that you want to discard any uncommitted changes. The command is as follows:
git checkout -f branch-name
However, exercise caution when using this option, as it will throw away all local changes in your working directory. Always ensure you don't need those changes before performing a forced checkout.
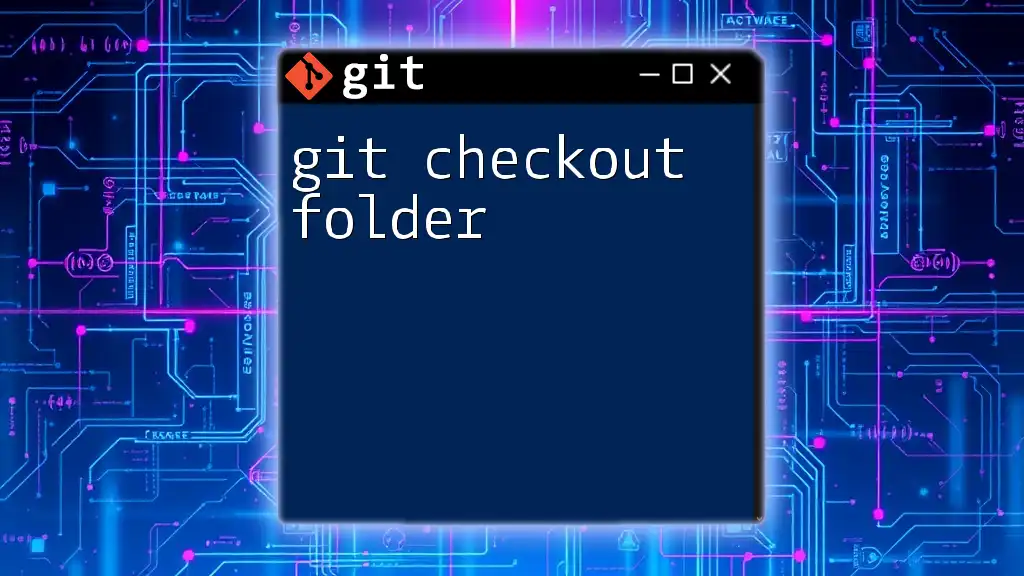
Best Practices for Avoiding Checkout Errors
Regularly Commit Your Changes
Frequent commits can help maintain a clean working directory and reduce the likelihood of encountering checkout errors. Aim to commit early and often, especially when significant changes are made.
Use Branch Naming Conventions
Establishing clear and consistent branch naming conventions can help reduce confusion. For example, using prefixes such as `feature/` for new features or `bugfix/` for bug fixes clarifies the purpose of each branch.
Maintain a Clean Workspace
Before switching branches or restoring files, run `git status` to check for uncommitted changes. Keeping your workspace organized can save time and prevent errors.
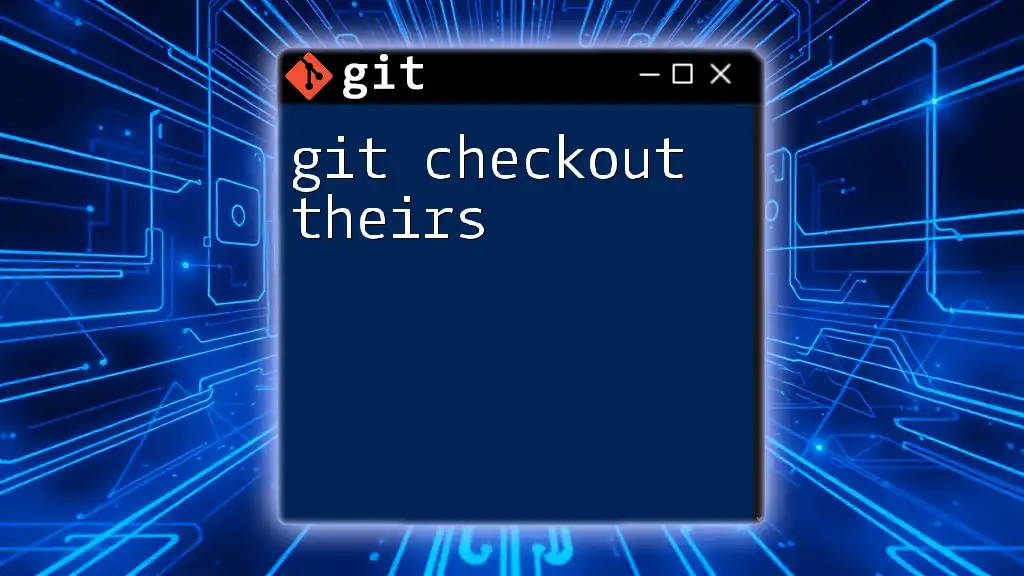
Conclusion
In summary, understanding the git checkout error is essential for efficient version control. By diagnosing errors promptly and implementing best practices, you can navigate your repositories effectively and minimize disruptions in your workflow. Remember that mastering Git requires practice, and by continuously learning and applying these skills, you can become proficient in handling Git commands with confidence.
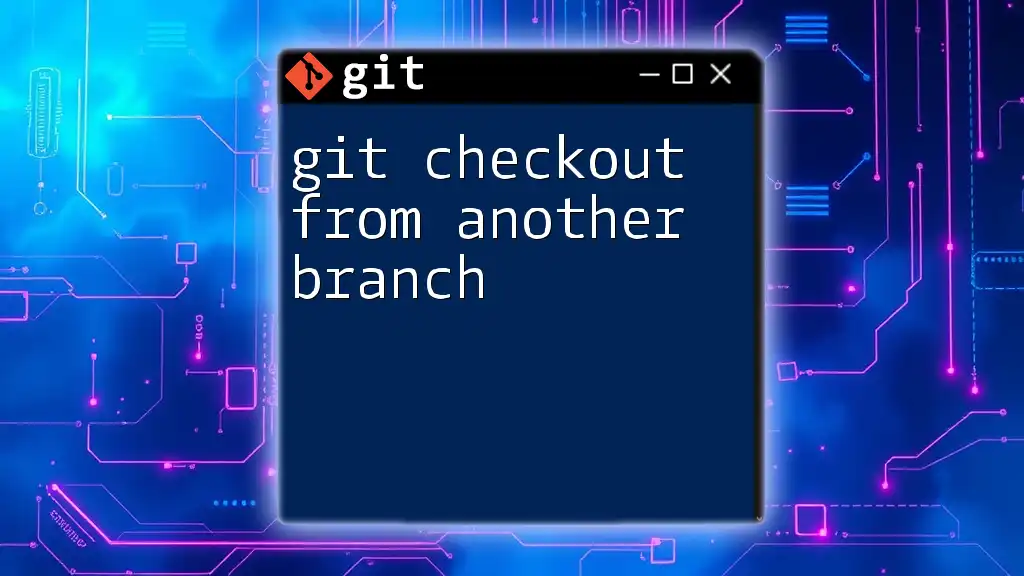
Additional Resources
Recommended Reading
For further knowledge, explore the official Git documentation and reputable blogs focusing on Git and version control.
Tools and Plugins
Consider using tools like Git GUIs or plugins for IDEs that provide visual interfaces, helping you manage branches and resolve conflicts more easily.