The `git checkout -b branch-name` command is used to create a new branch and immediately switch to it in your Git repository.
git checkout -b new-feature-branch
What is Git Branching?
Branching in Git allows developers to create separate lines of development within a project. This technique is crucial for implementing features, fixing bugs, or testing new ideas without affecting the main codebase. By creating branches, developers can isolate changes and collaborate more effectively, leading to a smoother integration process.
In Git, the primary branch is often called `main` (or `master` in older repositories), while feature branches, like `feature/login-form`, are created to work on specific tasks. This ensures that the main code remains stable while new features are being developed.
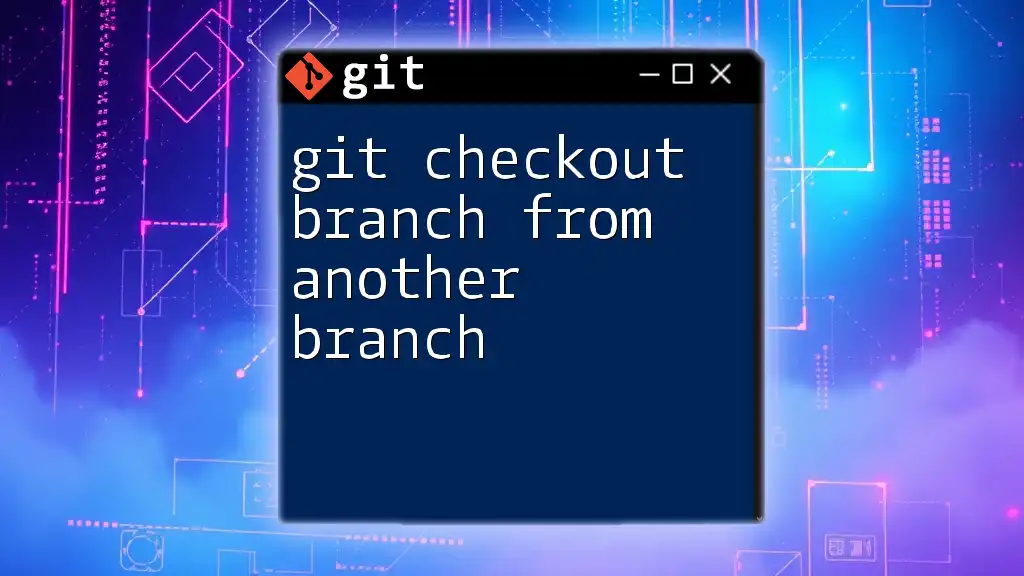
Understanding the `git checkout` Command
The `git checkout` command serves multiple purposes in the Git ecosystem. Its primary function is to switch between different branches or restore working tree files. Understanding this command is essential for effective version control.
The basic syntax for switching branches is:
git checkout <branch-name>
This command will change your working directory to the specified branch. If changes have been made to tracked files, Git will warn you about uncommitted changes.
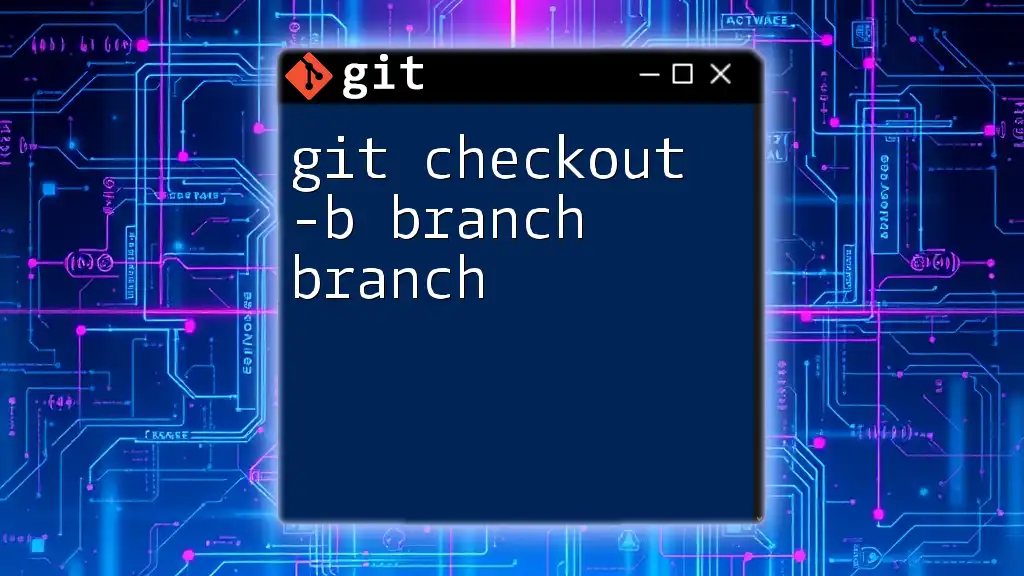
What Does the `-b` Option Do?
The `-b` flag in the `git checkout` command indicates that you want to create a new branch and then switch to it immediately. This eliminates the need for two separate commands: one to create the branch and another to switch to it.
When you use the `-b` option, you streamline your workflow, making the command not only efficient but also easy to remember.
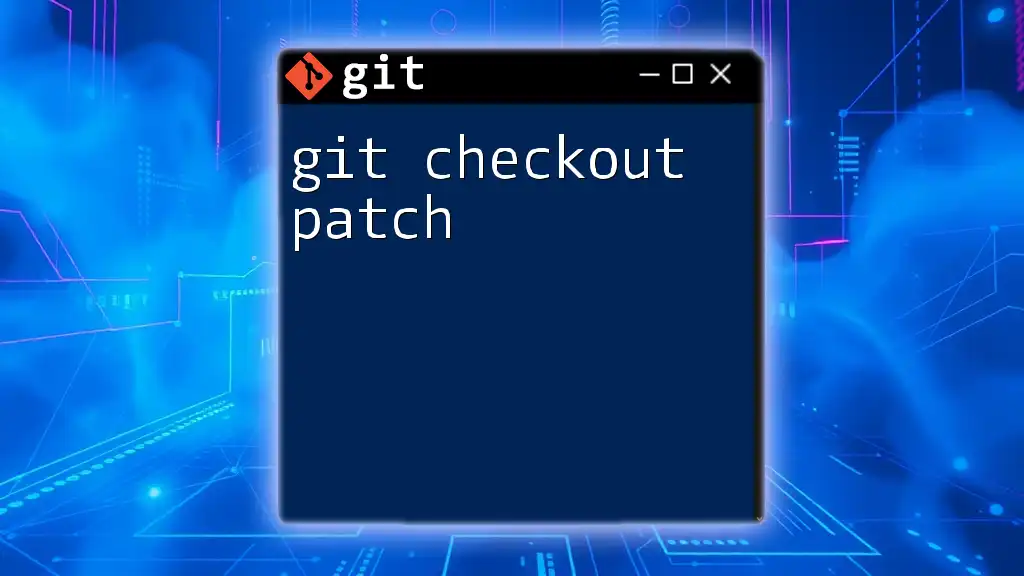
Using `git checkout -b` Command
Basic Syntax
To create and switch to a new branch, the syntax is:
git checkout -b <new-branch-name>
Step-by-Step Guide
Step 1: Ensure Your Repository is Up-to-Date
Before creating a new branch, it's crucial to make sure your local repository is clean and up-to-date. Use the following command to check the current status:
git status
If you have uncommitted changes, consider either committing or stashing those changes to avoid conflicts.
Step 2: Create and Switch to the New Branch
Once the repository is clean, you can create a new branch and switch to it using:
git checkout -b feature/new-feature
In this example, the branch `feature/new-feature` is created, and your working directory is switched to this new branch, allowing you to start making changes related to that feature immediately.
Example Scenario
Imagine you're working on a web application and need to implement a new user authentication feature. Instead of working directly on the `main` branch, you can create a dedicated branch for this feature. Here’s how you would do it:
git checkout -b feature/login-form
After executing this command, you make the necessary changes to your codebase related to the login form. Once the feature is complete, you would typically stage your changes, commit them, and prepare for a merge back into the main branch.
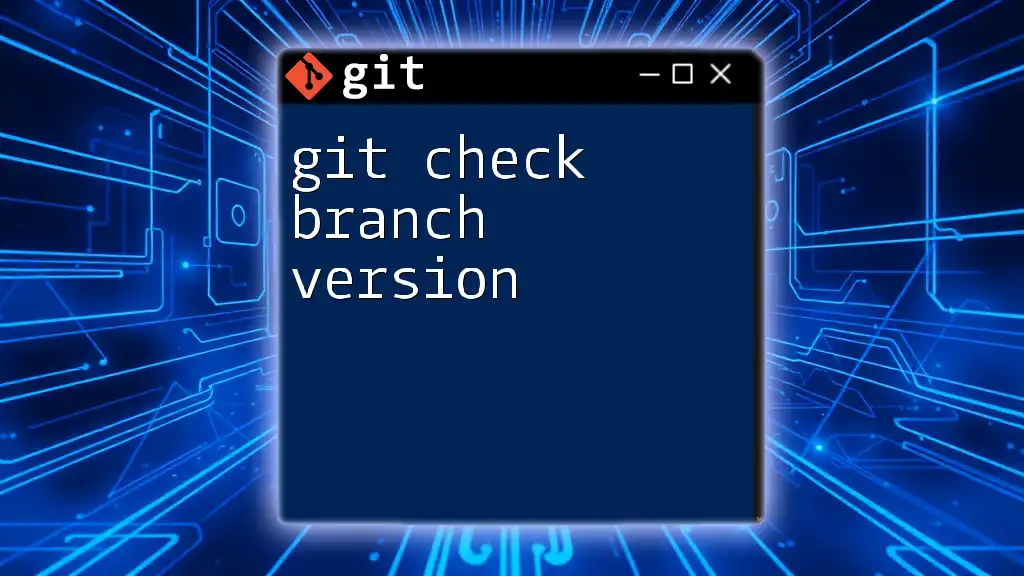
Best Practices for Using `git checkout -b`
Naming Conventions
It’s essential to use descriptive names for your branches. A good branch name conveys the purpose of the branch and makes it easier for collaborators to understand its intent. Following conventions such as `feature/`, `bugfix/`, or `hotfix/` can add clarity.
Regularly Review Branches
Keeping your repository neat means regularly reviewing and cleaning up unused branches. To list all your branches, you can use:
git branch
If you find stale branches that are no longer needed, remove them using:
git branch -d <branch-name> # Deletes the local branch
git push origin --delete <branch-name> # Deletes the remote branch
This habit ensures your workspace remains organized and minimizes confusion during collaborative endeavors.
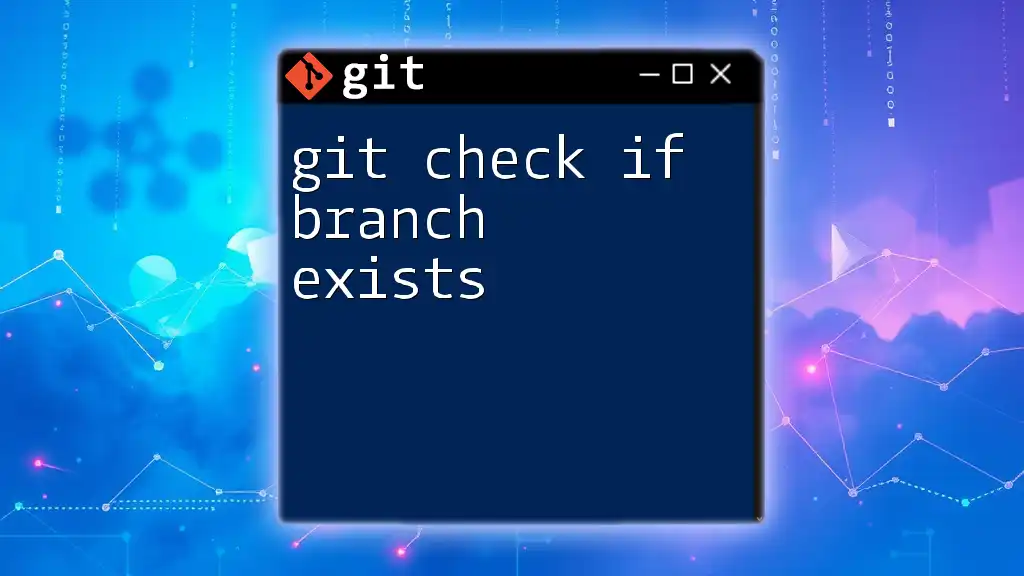
Common Errors and Troubleshooting
Error: Branch Already Exists
One common pitfall when using `git checkout -b` is attempting to create a branch that already exists. In this case, Git will return an error message informing you that the branch cannot be created. To resolve this, you can simply switch to the existing branch using:
git checkout <existing-branch-name>
Error: Dirty Working Directory
Another frequent issue arises when your working directory contains uncommitted changes. If you attempt to switch branches with uncommitted changes, Git will alert you. To handle this, you can either commit your changes or stash them with:
git stash
This temporarily saves your changes, allowing you to switch branches freely.
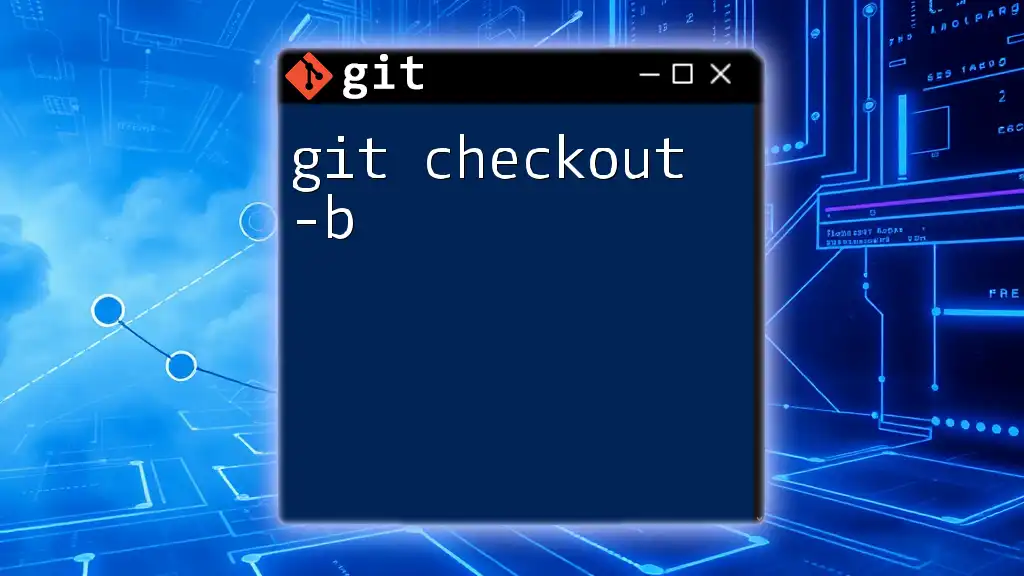
Conclusion
The `git checkout -b` command is an invaluable tool for developers, streamlining the process of creating and switching to new branches. By mastering this command, you enhance your Git skills, promote better collaboration, and streamline your development workflow. Remember to follow best practices for naming branches and cleaning up unused ones to maintain an organized repository.
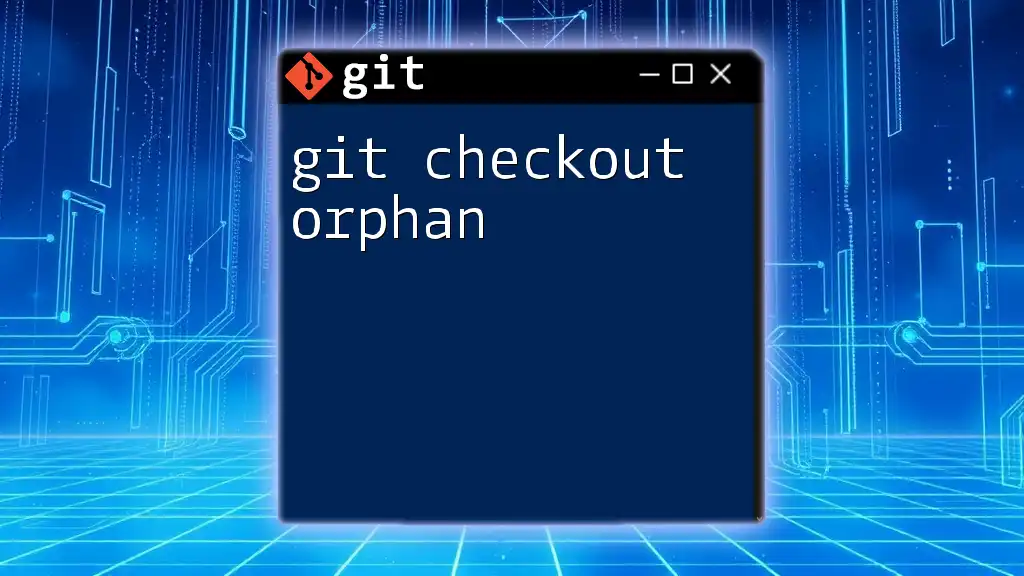
Additional Resources
For further learning, the official Git documentation provides in-depth information about branching and other Git commands. Additionally, consider exploring branching strategies and workflows, such as Git Flow or GitHub Flow, to enhance your version control practices.
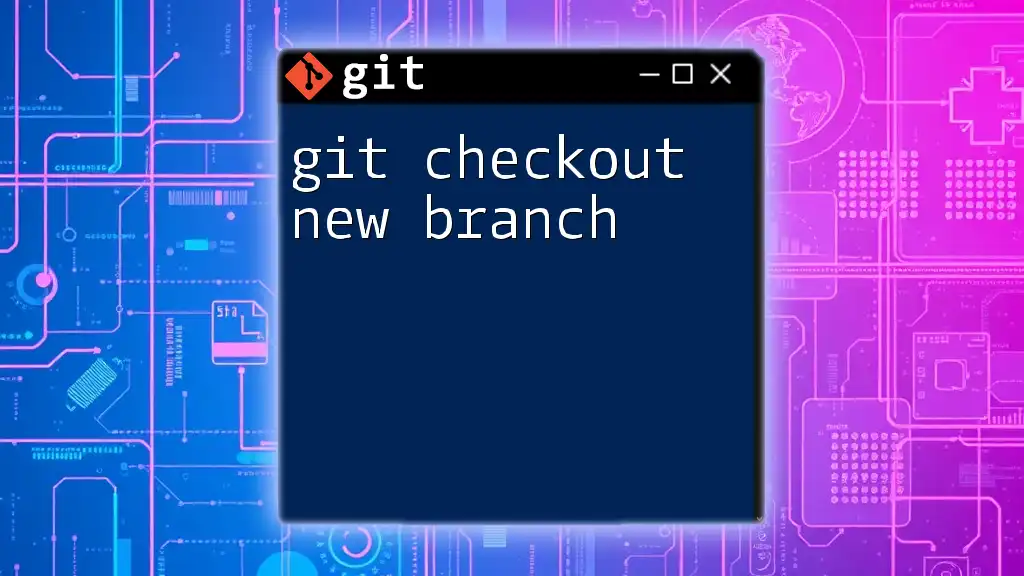
Call to Action
Stay tuned for more insightful articles and tutorials aimed at simplifying your Git experience. For anyone looking to get started quickly, consider downloading our free Git cheat sheet of commands!