To switch to a branch in Git from another branch, you can use the command `git checkout` followed by the target branch name.
Here's the code snippet:
git checkout target-branch
What is a Git Branch?
Definition of a Branch
A branch in Git represents an independent line of development. This allows multiple developers to work on different features simultaneously without affecting the main codebase. Branching is a powerful feature in Git that promotes isolation for changes being made, enabling safer development and testing.
Types of Branches in Git
Branches can be categorized into several types:
- Main Branch: This is typically the primary branch known as `main` or `master`. It contains the stable version of the project.
- Feature Branches: These branches are used for developing new features, allowing developers to work independently on specific tasks without disrupting the workflow of others.
- Development Branches: Temporary branches created for testing or integration purposes, often merged back into the main or feature branches.
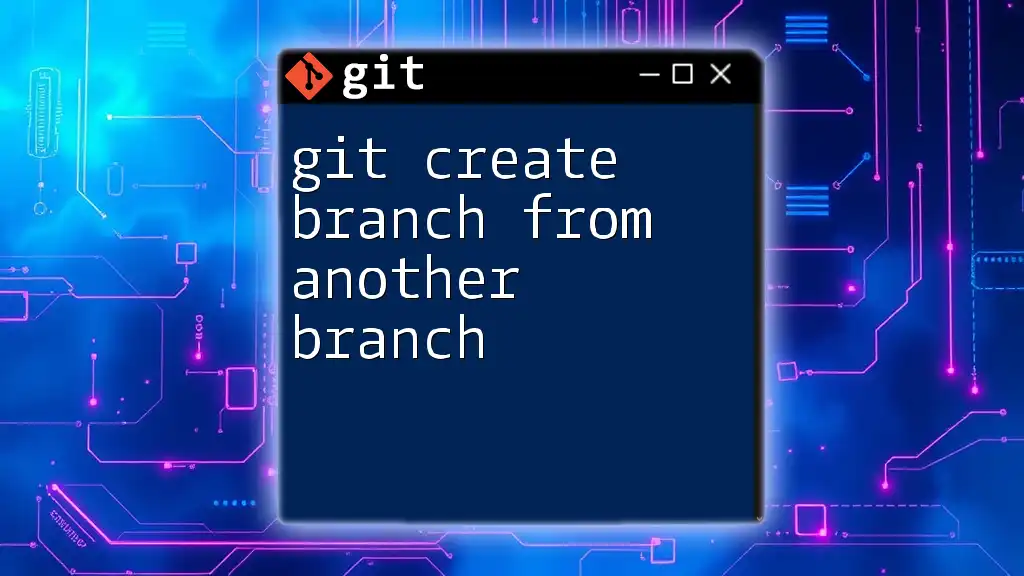
Overview of `git checkout`
What Does `git checkout` Do?
The `git checkout` command plays a key role in navigating between branches and restoring files in your project. Its functionality allows a user to switch to a different branch, retrieve changes from another branch, or revert files to a previous state.
The Purpose of Checking Out a Branch
When you use `git checkout`, you are essentially telling Git which branch you want to work on. This is crucial for ensuring that you’re making changes in the context of the right development line. Working on different branches allows teams to manage features, fixes, and experiments independently, thus reducing the likelihood of affecting ongoing work.
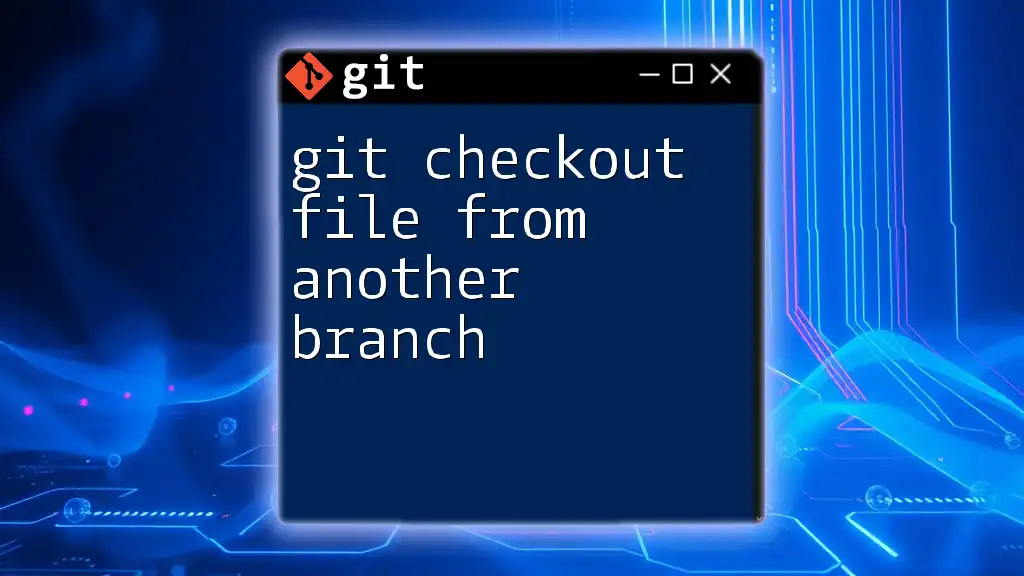
Checking Out a Branch from Another Branch
The Basic Command Structure
To switch to a different branch, the basic syntax used is:
git checkout <branch_name>
This command lets you transition to the branch specified, updating your working directory to reflect the state of that branch.
Checking out a New Branch from Another Branch
Steps to Checkout a New Branch
If you want to create a new branch based on another branch and switch to it simultaneously, you can use:
git checkout -b feature/my-feature other-branch
In this command:
- `-b` flag is critical; it denotes that you want to create a new branch.
- `feature/my-feature` is the name of the new branch, and `other-branch` is the branch you’re basing it on.
This operation will seamlessly create a new branch and allow you to start working on it without affecting the original branch.
Switching Between Existing Branches
To switch to an already existing branch, simply execute:
git checkout existing-branch
This command will take you straight to the specified branch, updating your working directory to its latest commit.
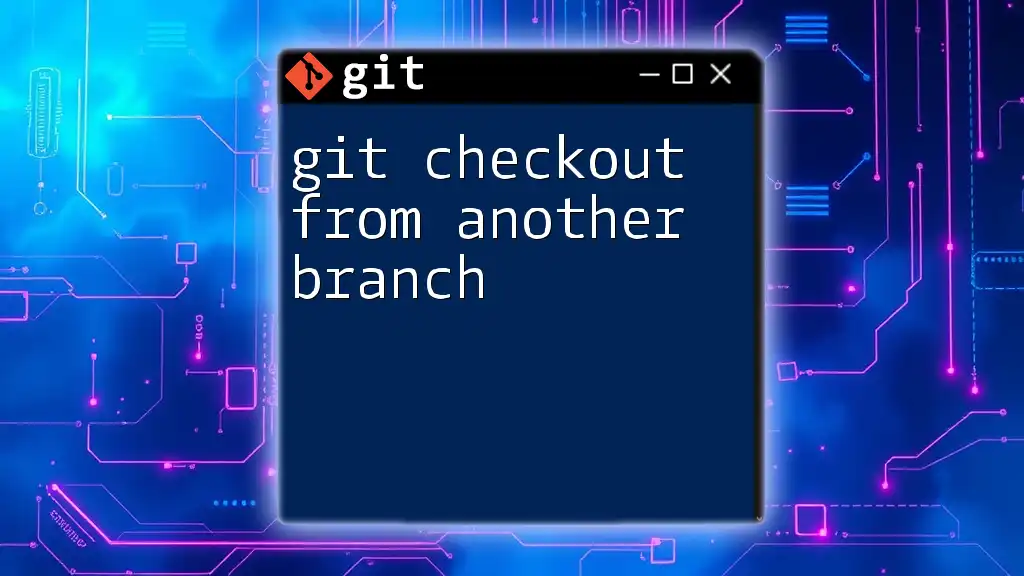
Best Practices for Branch Management
Using Descriptive Branch Names
Clear and descriptive branch names are vital for collaboration. They provide insight into the feature or fix being developed. Instead of generic names like `feature1`, opt for something meaningful such as `feature/add-user-authentication`.
Keeping Branches Up to Date
To ensure that your Feature Branch has the latest changes from the Main Branch, regularly sync them by following these steps:
git checkout main
git pull origin main
git checkout feature/my-feature
git merge main
Executing the commands above allows you to:
- Switch to the main branch.
- Pull the latest changes from the remote repository.
- Switch back to your feature branch and merge in any new updates.
Avoiding Conflicts
Branching makes collaboration smoother, but it can also lead to conflicts. To minimize these issues, communicate with team members to synchronize efforts and regularly merge the main branch into your feature branch.
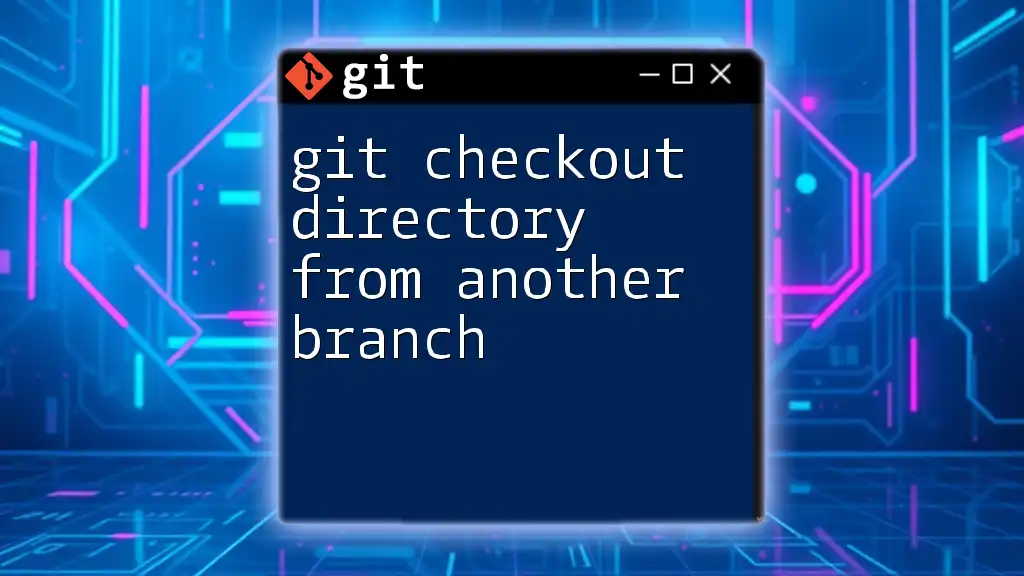
Common Issues and Troubleshooting
Errors when Checking Out Branches
It's common to encounter error messages during branch checkout, such as "Your path is dirty," which means you have uncommitted changes in your working directory. This is an important situation to address before attempting to switch branches.
Dealing with Uncommitted Changes
Sometimes, you need to switch branches without committing changes. In such cases, you can stash changes temporarily:
git stash
This command saves your changes aside and allows you to switch branches freely. Later, you can apply the stashed changes using:
git stash apply
This approach keeps your workflow moving smoothly without losing any modifications.
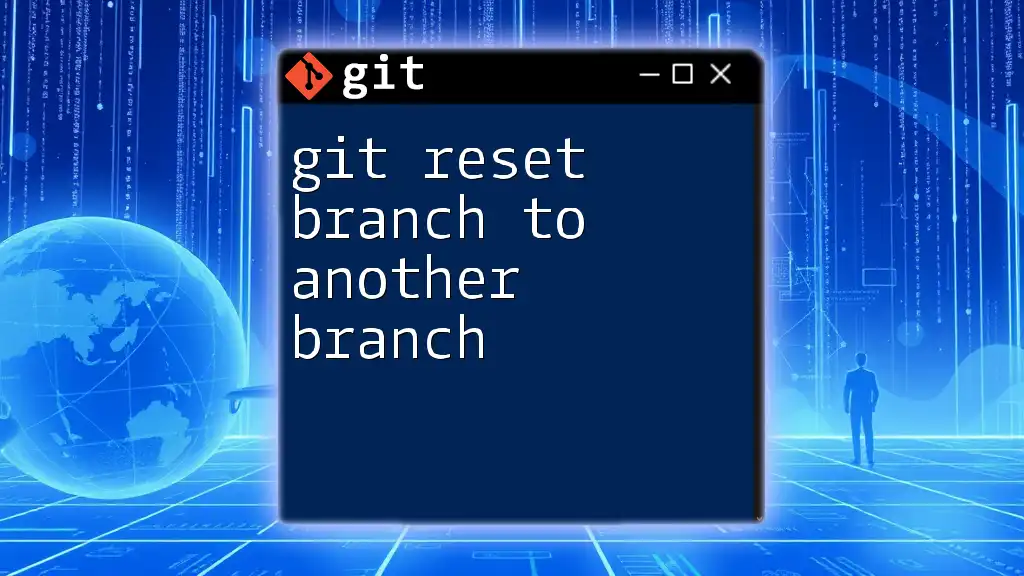
Conclusion
Understanding how to git checkout branch from another branch is a fundamental skill in version control that enhances collaboration and productivity. By mastering branch management techniques, developers can effectively isolate features, integrate updates, and minimize conflicts. Practice the commands and concepts discussed, and you'll be well on your way to Git proficiency. For those eager to dive deeper, many resources and guides await your exploration.