To create a new branch from another existing branch in Git, first switch to the desired base branch and then use the `git checkout -b` command followed by the new branch name.
git checkout existing-branch
git checkout -b new-branch
Understanding Branches in Git
What is a Git Branch?
A branch in Git can be thought of as a separate line of development. It allows you to isolate changes, making it easier to experiment with new features or fix bugs without affecting the main codebase. By maintaining separate branches for different tasks, teams can work concurrently on features and enhancements without interference, which is crucial in collaborative software development.
Why Create a Branch from Another Branch?
Creating a new branch from an existing one is particularly advantageous when you want to build on top of features that are still in progress or to base your new work off a specific point in your project history. This practice supports:
- Feature Development: You might want to implement a new feature that depends on the work already done in another branch.
- Bug Fixes: If you're developing a bug-fix branch, starting from a feature branch lets you target specific changes while keeping your bug fixes well-organized.
- Experimentation: Creating branches for experimental changes allows for a seamless exploration of new ideas without disrupting the primary codebase.
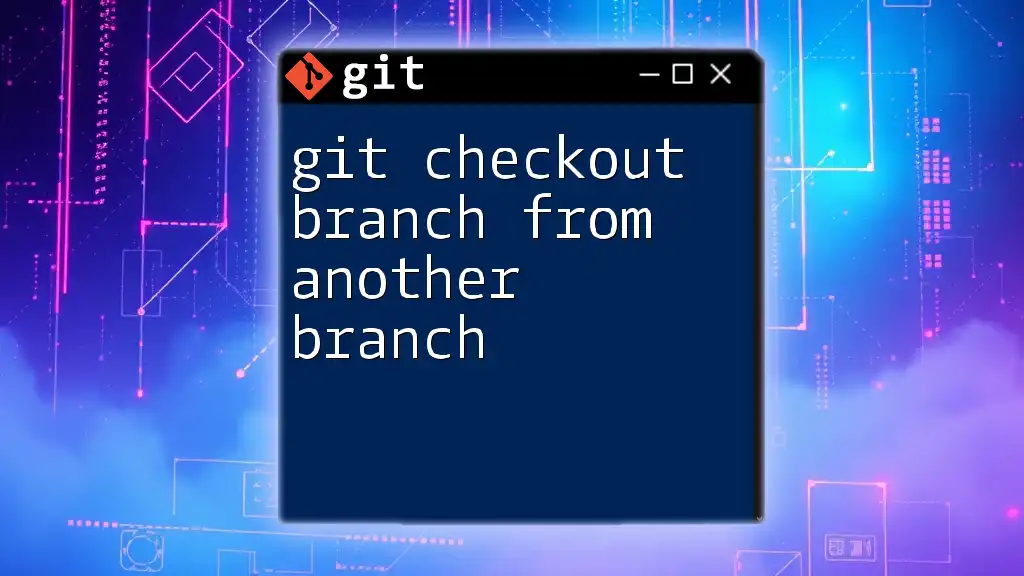
Preparing to Create a New Branch
Navigating to Your Repository
Before creating a new branch, ensure you are in the appropriate project directory. You can navigate there using:
cd /path/to/your/repository
Checking the Current Branch
It's crucial to check which branch you are currently on before creating a new branch. This ensures you're basing your new work on the correct branch. You can see your current branch with the following command:
git branch
In the output, the current branch will be highlighted with an asterisk (*), making it clear which branch you are working in.
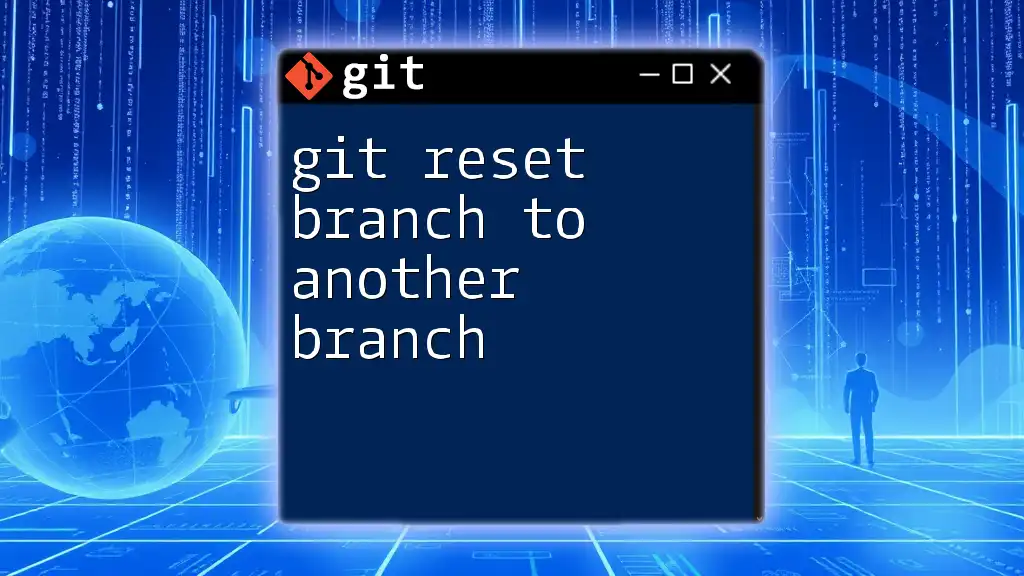
Creating a New Branch from Another Branch
Step-by-Step Process
Step 1: Switch to the Base Branch
The first step in creating a new branch from another branch is to check out the branch that will serve as the foundation. This is essential to ensure your new branch contains all the changes from the base branch. You can switch to the desired branch using:
git checkout base-branch-name
For example, if your base branch is `main`, you would run:
git checkout main
Step 2: Create the New Branch
Once you're on the base branch, you can create your new branch. The command to do this is:
git checkout -b new-branch-name
This command accomplishes two tasks: it creates a new branch and immediately switches to that branch. For instance, if you're creating a feature branch called `feature/new-feature`, you would enter:
git checkout -b feature/new-feature
Alternative Method: Using Git Commands
Alternatively, you can create a new branch using the `git branch` command. This method separates the creation of the branch from the action of checking it out:
git branch new-branch-name base-branch-name
This approach can be particularly useful if you’d like to create multiple branches at once without checking them out immediately. For example:
git branch feature/new-feature main
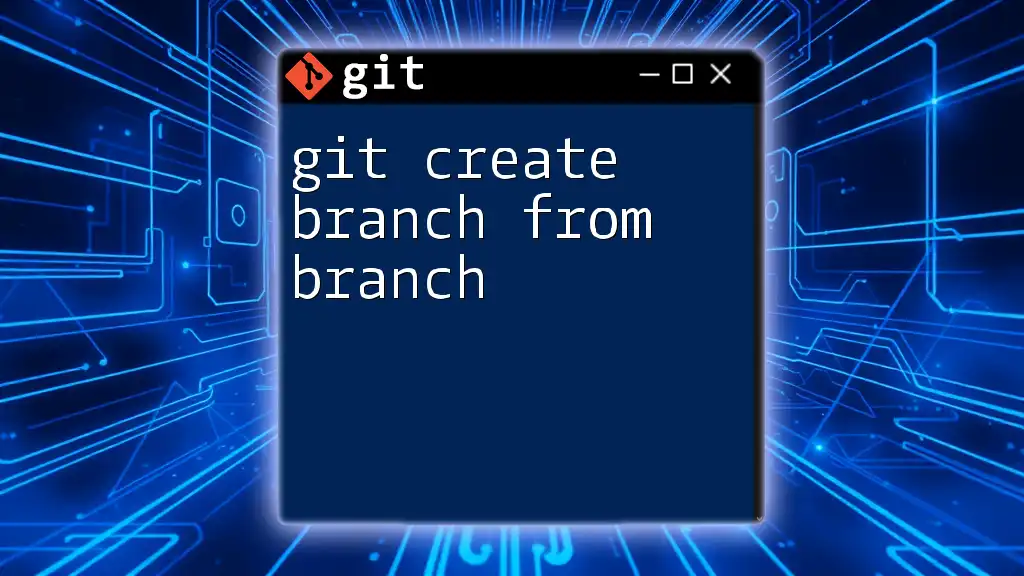
Working with the New Branch
Making Changes in the New Branch
Once your new branch is created, you can start making changes. It’s always a best practice to stage your changes and commit them with clear messaging. Here’s how to do this:
git add .
git commit -m "Add new feature implementation"
Proper commit messages describe the purpose of changes, which is crucial for maintaining the integrity and clarity of your project history.
Pushing the New Branch to Remote
After making your changes, pushing the new branch to your remote repository allows others to access your work. Use the following command to push the newly created branch:
git push origin new-branch-name
Pushing the branch facilitates collaboration, ensuring that teammates can review or build upon your changes.
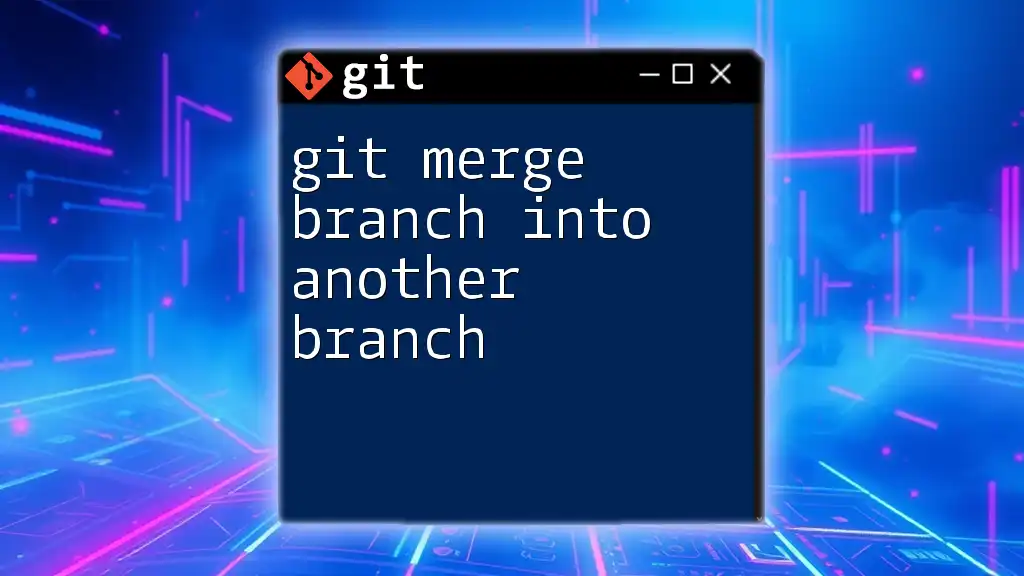
Merging the New Branch Back
Preparing for a Merge
Once development on your new branch is complete, you’ll likely want to merge your changes back into the base branch. This helps integrate your feature or fix into the main project.
Merge Command
To merge your changes, switch back to the base branch and use the merge command. The commands are:
git checkout base-branch-name
git merge new-branch-name
Merging may result in conflicts if changes in the two branches overlap. Be prepared to resolve these conflicts manually if they occur.
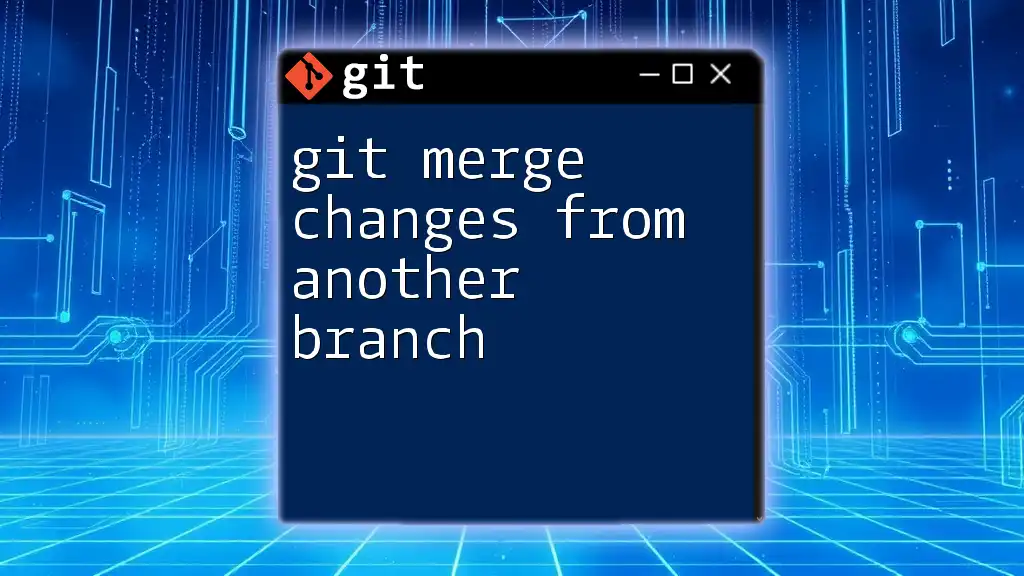
Best Practices When Creating Branches
Naming Conventions
Establishing clear and descriptive branch naming conventions is key to maintaining an understandable project structure. Follow these suggestions:
- Use Prefixes: For example, use `feature/` for new features, `bugfix/` for bug fixes, etc.
- Descriptive Names: Consider including the issue or work item number in the branch name, like `feature/123-implement-login`.
Keeping Branches Up to Date
Keeping branches up to date with the latest changes from the main branch helps prevent complex merge conflicts. Regularly merge the main branch into your feature branch with:
git merge main
This practice ensures your feature branch stays relevant and functional as corrections or additions occur in the main codebase.
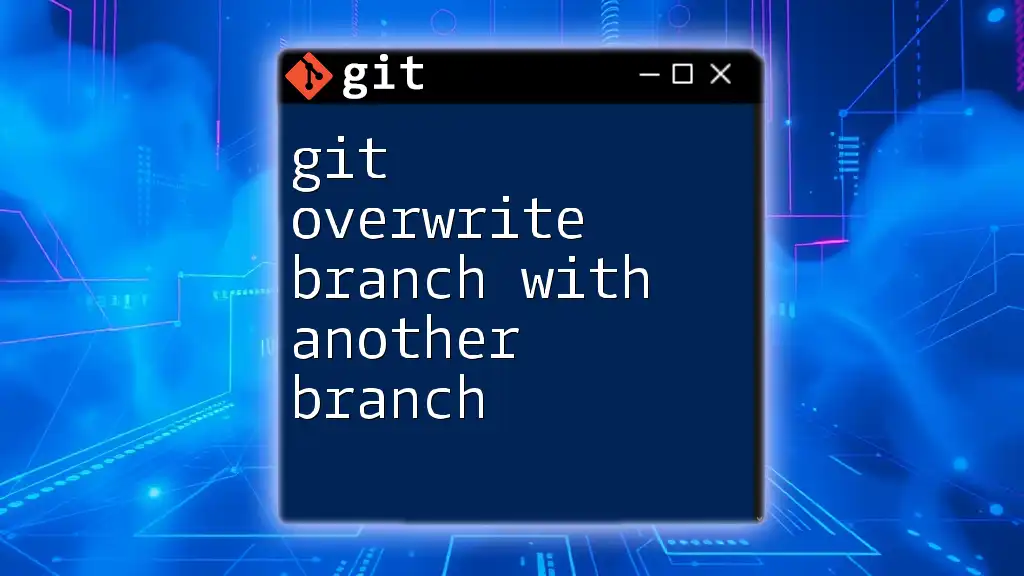
Conclusion
Creating a branch from another branch is a fundamental practice in Git that enhances your workflow and project management capabilities. By isolating changes, you can effectively collaborate and develop robust features without risking the stability of the main codebase. Consistent practice and application of these branching techniques will solidify your understanding of Git and improve your productivity in version control.
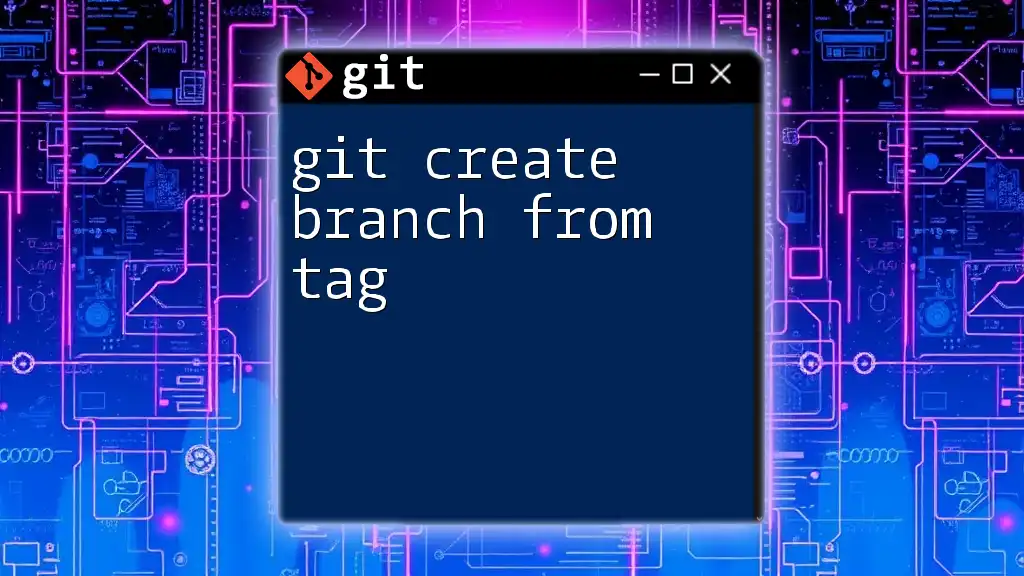
Additional Resources
Explore the official Git documentation for further understanding, and consider additional readings to complement your knowledge about branching strategies and best practices in Git. Stay tuned for more insights and tips as you continue on your journey to mastering Git commands!