To create a new branch from an existing branch in Git, you can use the following command while checking out the existing branch first.
git checkout existing-branch
git checkout -b new-branch
Understanding Git Branching
What is a Git Branch?
In Git, a branch is essentially a pointer to a specific commit in your repository's history. You can think of it as a separate line of development that allows you to work on features, fixes, or experiments independently of the main codebase. This capability is crucial for maintaining a clean and organized project history.
Why Use Branches?
Using branches in development offers numerous advantages, including:
- Isolated Development: Each branch allows you to work on features or fixes without affecting the main codebase, providing a layer of safety during the development process.
- Experimentation without Risk: Branches enable developers to experiment freely. If the experiment doesn't work out, you can simply delete the branch without impacting the main project.
- Facilitating Collaboration: Multiple developers can work on different branches simultaneously, allowing for efficient collaboration without conflicts.
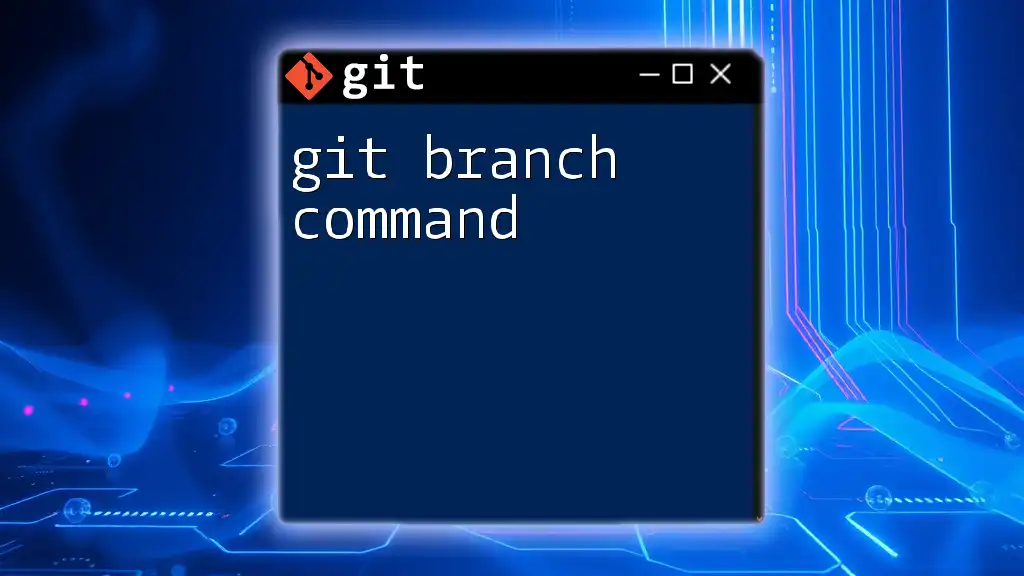
Creating a Branch from Another Branch
The Basics of Branch Creation
To create a new branch in Git, you typically use the `git branch` command followed by the name of the new branch you want to create. The general syntax is:
git branch <new-branch-name>
Creating a Branch from a Specific Branch
You can create a new branch from an existing one by first checking out the existing branch and then creating a new one based on it. Here’s how you can do it:
- First, switch to the existing branch from which you want to branch out:
git checkout <existing-branch-name>
- Next, create the new branch:
git branch <new-branch-name>
In this process, the new branch will start at the same commit as the existing branch, thus capturing the context and history at that point.
Checking Out to the New Branch
Once you've created your new branch, you'll want to switch to it to start making changes. This can be accomplished using either the `git checkout` or `git switch` commands:
git checkout <new-branch-name>
Alternatively, you can use the simpler `git switch` command:
git switch <new-branch-name>
This command immediately transitions your working directory to the new branch, making it ready for development.
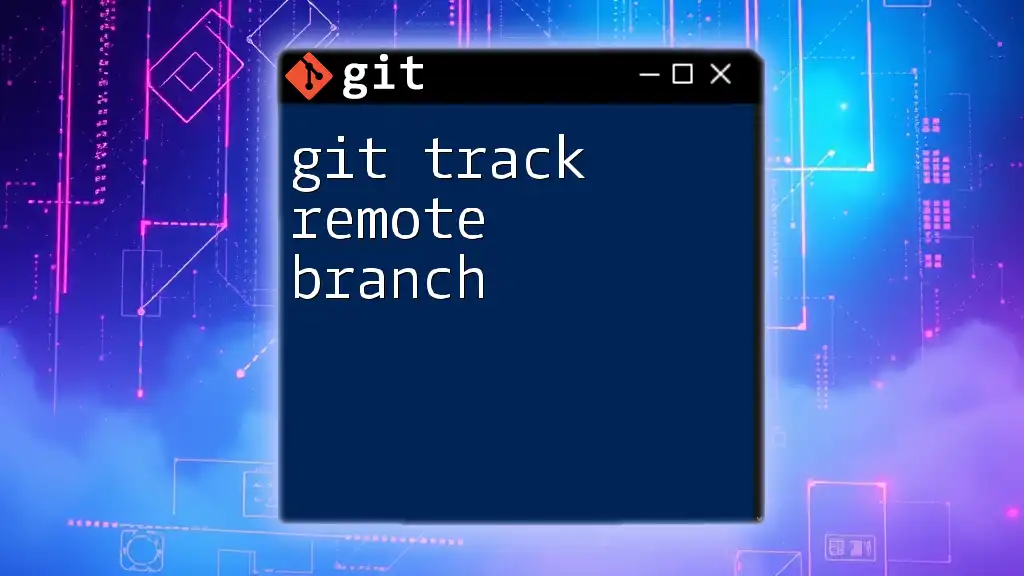
Advanced Techniques
Creating and Checking Out in One Command
A more expedient approach to create and switch to a new branch is to combine both commands into one. This can be done using the following syntax:
git checkout -b <new-branch-name> <existing-branch-name>
This command not only creates a new branch from the specified existing branch but also checks it out immediately. This method saves you time and reduces the number of commands you need to type.
Tracking Remote Branches
In collaborative projects, you frequently need to create local branches that track corresponding remote branches. This helps you synchronize changes between your local repository and the team's remote repository. You can achieve this by using the following command:
git checkout -b <new-branch-name> origin/<remote-branch-name>
By tracking a remote branch, any changes you make can be easily pushed to the team, and you can also pull updates from the remote side to keep your local changes current.
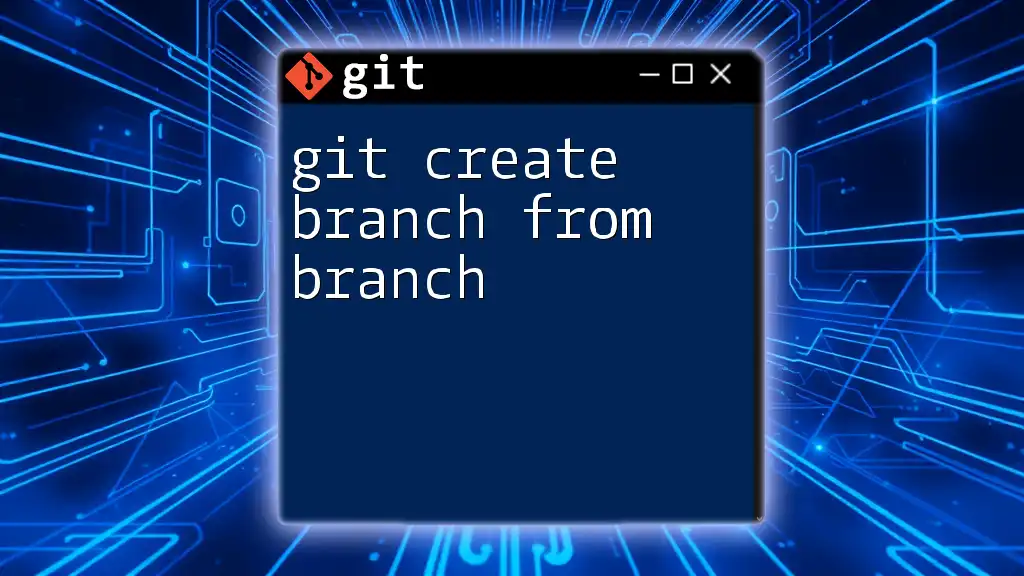
Workflow Scenarios
Feature Development
Suppose you're working on a new feature and want to branch off from the main branch (`main`). You would run:
git checkout main
git checkout -b feature/new-awesome-feature
This command creates a new branch specifically dedicated to the feature, allowing focused development without messing with the main code.
Bug Fixing
Let’s say you need to address a bug found in the `release` branch. To create a bug fix branch from the release branch, use:
git checkout release
git checkout -b fix/urgent-bug
This keeps your bug fixing isolated, and once completed, you can merge back into the release branch while ensuring that the main code remains stable.
Experimentation with Experimental Features
Creating an experimental branch is perfect for trying out concepts that might not yet be finalized. Doing it from `develop` or `main` branches is quite straightforward:
git checkout develop
git checkout -b experiment/new-idea
This branch serves as a playground where you can freely experiment with new ideas. If it doesn’t pan out, simply delete the branch without any consequences to your main codebase.
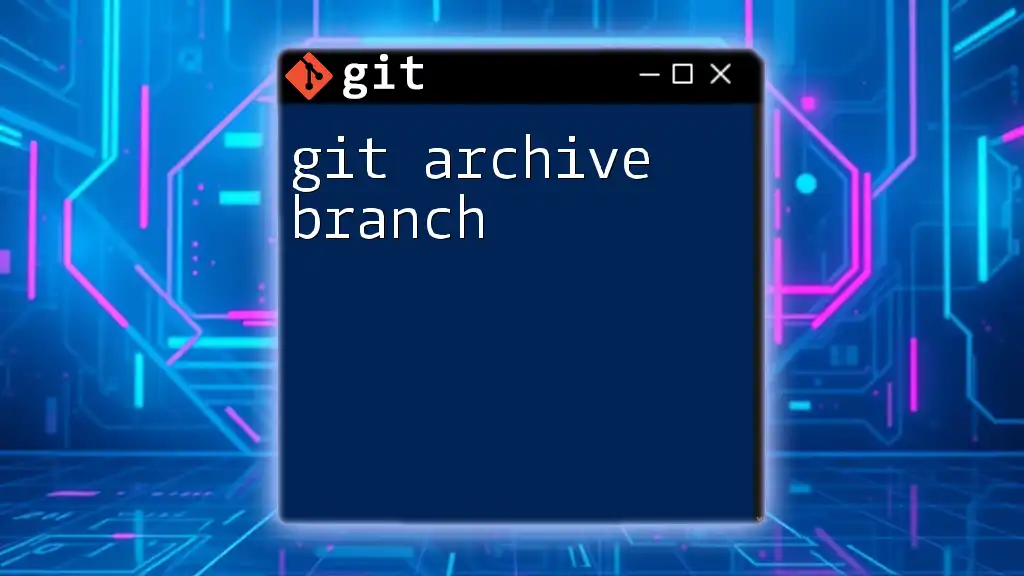
Common Pitfalls and Best Practices
Avoiding Confusion with Branch Names
Clear and descriptive branch names are crucial to maintaining an organized codebase. Use naming conventions that reflect the purpose, such as:
- `feature/` for new features
- `fix/` for bug fixes
- `experiment/` for experimental work
This clarity will make it easier for you and your team members to understand the purpose of each branch at a glance.
Keeping Your Branches Updated
Regularly updating your branches is essential to avoid conflicts and ensure you’re working with the latest code. Consider merging or rebasing your branches periodically. For merging, you may use:
git checkout <branch>
git merge <target-branch>
This practice minimizes the headache of merge conflicts by keeping your branches in sync with the main project.
Deleting Branches After Merging
Once you've completed work on a branch and merged it back into the main codebase, it's best practice to delete the branch to keep your repository clean. You can delete a branch using:
git branch -d <branch-name>
This not only declutters your project but also ensures that outdated branches do not lead to confusion in the development workflow.
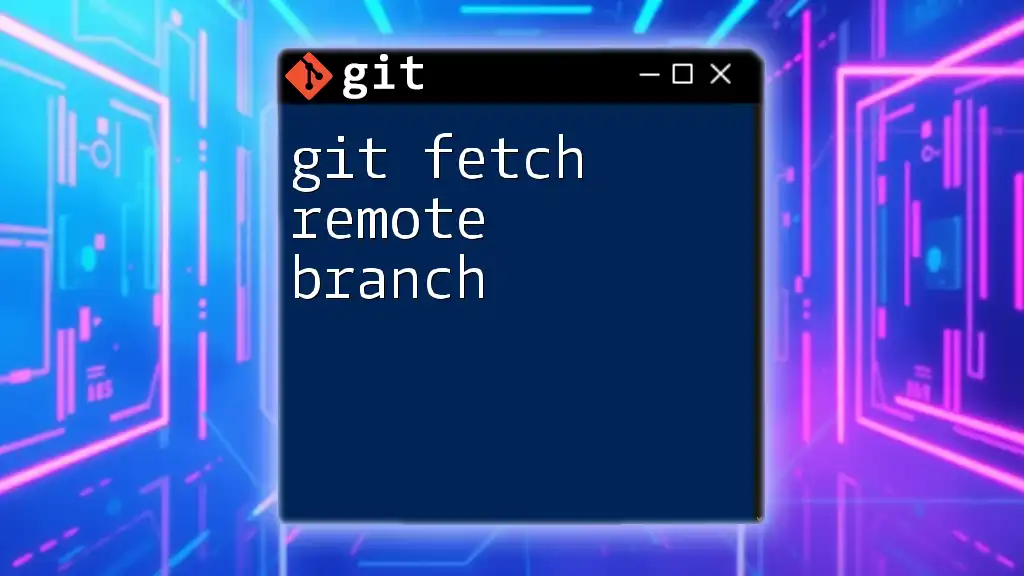
Conclusion
The ability to create a git branch from a branch offers powerful flexibility in your development workflow, allowing you to manage features, fixes, and experiments effectively. By mastering branching techniques and best practices, you can maintain a streamlined and efficient development process that fosters collaboration and innovation. As you explore Git further, consider joining our training sessions for hands-on experience and deeper insights into advanced Git functionalities!
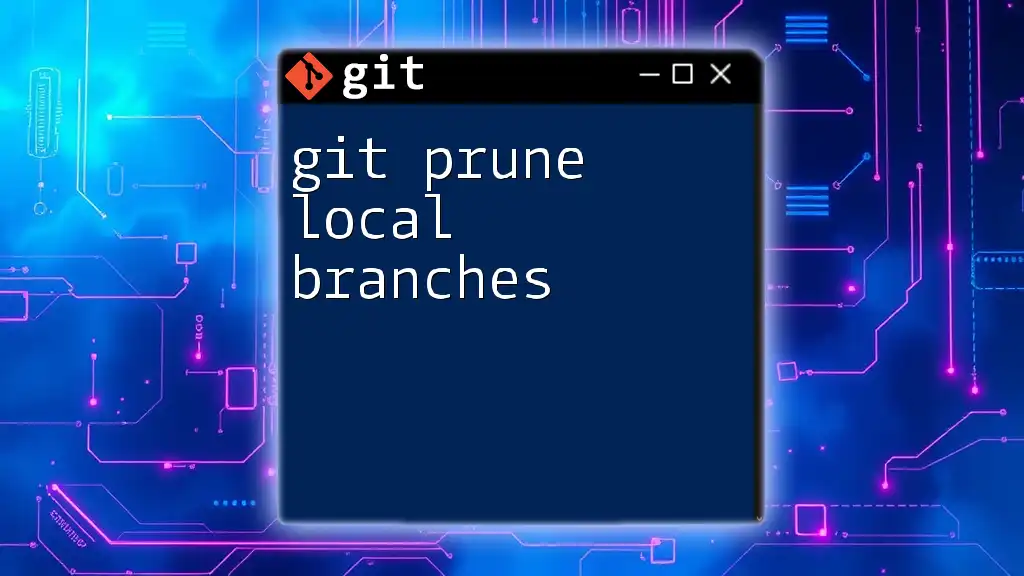
Additional Resources
To deepen your understanding of Git and branching, check out the official Git documentation and various online resources available. There are numerous books and courses that can guide your journey further. Don't hesitate to take advantage of the extensive resources available to enhance your skills!