To pull changes from another branch in Git, you first need to switch to your current branch and then use the `git pull` command followed by the branch name from which you want to fetch and merge updates.
git checkout your-current-branch
git pull origin target-branch
Understanding Git Branches
What is a Git Branch?
A branch in Git is essentially a pointer to a specific commit in your repository's history. It allows you to work on features or fixes in isolation from the stable code located in the main branch, often referred to as `main` or `master`. When you create a branch, you can experiment without disrupting the primary codebase.
Branches play a crucial role in version control, facilitating collaboration among team members. When multiple developers work on a project, each can create their own branches to develop features. This way, everyone's work remains separate until it's ready to be integrated.
Example: A simple visualization of branch creation might look like this:
* 3df69a3 - Feature A (feature-branch)
| * 2b4234e - Initial commit (main)
This shows `feature-branch` working on a commit while the `main` branch remains unchanged.
Typical Branching Workflow
In a typical Git workflow, developers create a new branch from `main` whenever they start working on a new feature or bug fix. They can make as many changes as needed in this branch. Once their work is ready and tested, they can merge it back into the `main` branch, ensuring that the project remains stable.
Importance: Keeping branches updated is vital, especially in collaborative environments. This ensures that new changes from the `main` branch are incorporated into feature branches, reducing future merge conflicts.
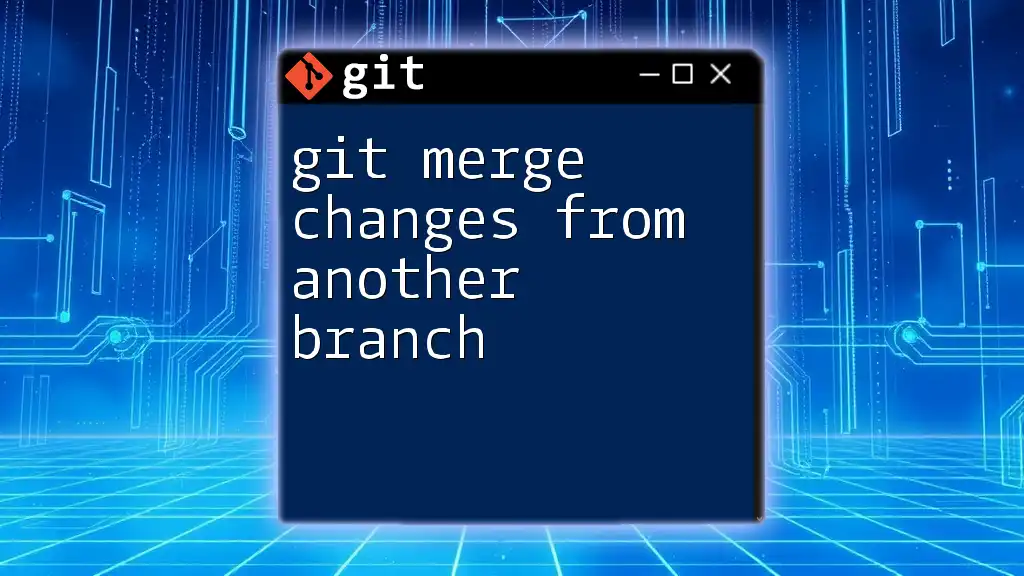
The Basics of the `git pull` Command
What Does `git pull` Do?
The `git pull` command is used to fetch and download content from a remote repository and directly integrate it into your current branch. Essentially, it combines two commands: `git fetch` and `git merge`. When you run `git pull`, Git first fetches updates from the specified branch and then merges them into the branch you are currently on.
Common Use Cases for `git pull`
Developers generally utilize `git pull` when they need to:
- Update their local repository with the latest changes made by others.
- Collaborate with team members, ensuring everyone's contributions are reflected in their working copies.
- Sync feature branches with the main branch so they can incorporate the latest changes before merging.
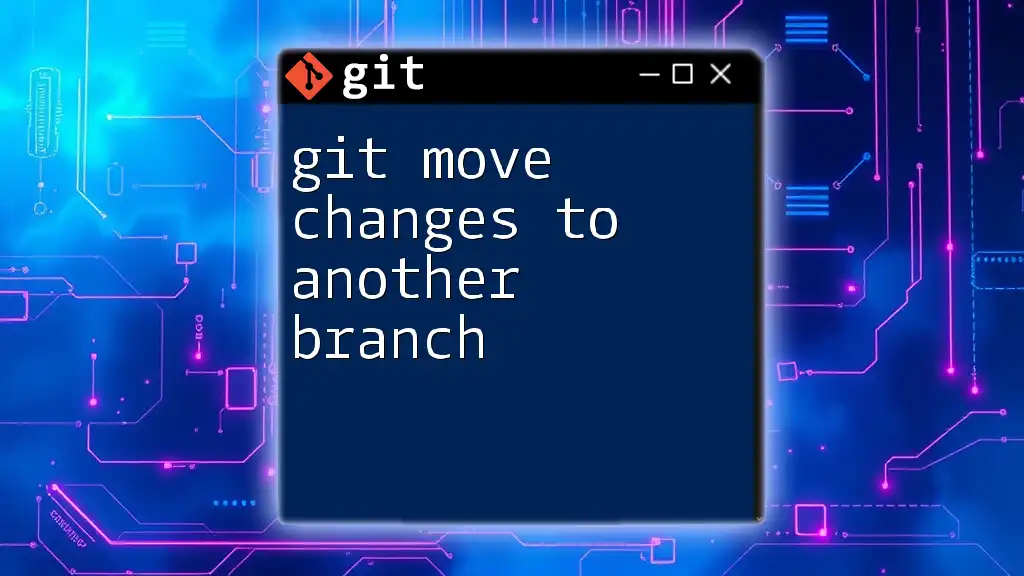
Pulling Changes from Another Branch
The `git pull` Syntax
The general syntax of the `git pull` command is as follows:
git pull <remote> <branch>
Here, `<remote>` specifies the remote repository (often `origin`), and `<branch>` represents the name of the branch you wish to pull.
Pulling Changes from a Specific Branch
To pull changes from a specific branch, simply use the following command:
git pull origin feature-branch
In this command:
- `origin` refers to the default name for your remote repository.
- `feature-branch` is the name of the branch from which you want to pull changes.
This command fetches the latest changes from the `feature-branch` into your current branch. If there are no conflicts, the changes will be merged automatically.
Handling Conflicts During a Pull
What are Merge Conflicts?
Merge conflicts arise when changes in the branch being pulled contradict the changes in your current branch. For instance, if both branches modify the same line of code but with different values, Git doesn’t know which version to keep, resulting in a conflict.
Example: Suppose you have the following scenario leading to a conflict:
- You change line 10 in your current branch to display "Hello World!"
- Simultaneously, someone else changes line 10 in `feature-branch` to display "Greetings, Universe!"
When you attempt to pull the changes from `feature-branch`, Git will indicate a conflict and stop the merging process.
Step-by-Step Resolution Guide
Step 1: If a conflict arises, you will see notifications in your terminal about the conflicting files.
Step 2: Use `git status` to identify which files are in conflict. This command gives you a clear view of the status of your repository, highlighting any issues.
Step 3: Open the conflicting files, and you will see sections marked with conflict markers:
<<<<<<< HEAD
Your changes
=======
Changes from feature-branch
>>>>>>> feature-branch
Edit the file to resolve the conflict, choosing the desired code, or combining both changes as appropriate.
Step 4: After resolving the conflicts, use `git add` to stage the changes:
git add <conflicted-file>
Finally, finalize the merge with:
git commit
This ensures that the merge is recorded, and your branch now reflects both sets of changes.
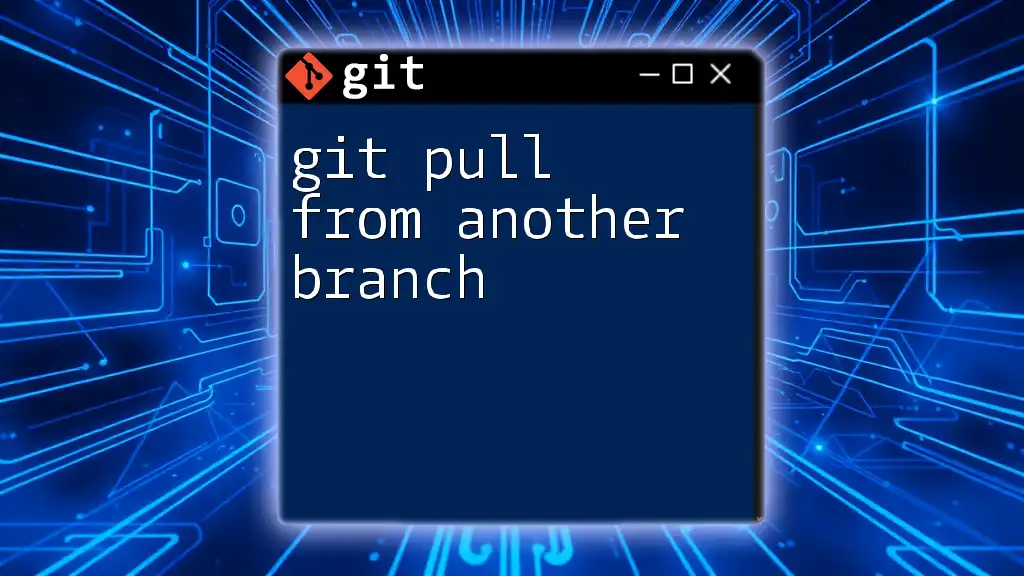
Best Practices for Pulling from Another Branch
Regular Pulls to Maintain Sync
To effectively manage your branches and reduce the likelihood of conflicts, it’s essential to pull changes frequently. Regular updates help to keep your local branches aligned with remote branches, thereby facilitating smoother integration when you are ready to merge your changes.
Pull Requests vs. Direct Pulls
While `git pull` is a straightforward way to bring in changes, pull requests are a more collaborative approach, often utilized in team environments. A pull request allows you to propose your changes and request reviews before merging. It's an excellent way to ensure that all team members are on the same page and can inspect changes before they affect the main codebase.
Keeping Your Own Branches Updated
A good practice before executing `git pull` is to run `git fetch`. This command downloads changes from the remote branch without merging them, letting you inspect what changes will be applied:
git fetch origin
Once you're aware of the incoming changes, you can perform `git pull` with greater confidence.
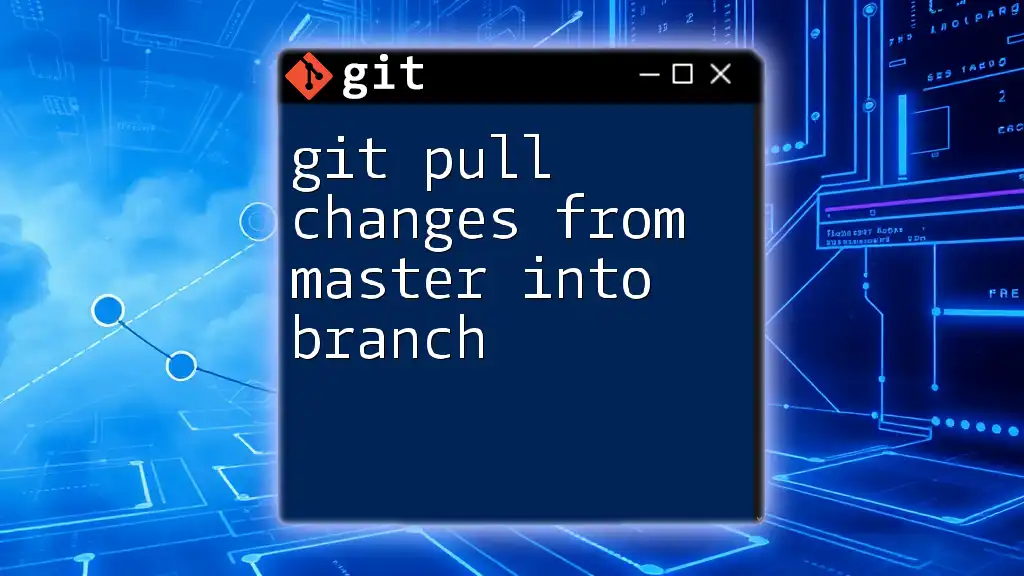
Conclusion
Pulling changes from another branch in Git is a fundamental skill every developer must master. It enhances collaboration and ensures everyone’s contributions are accurately represented in the project. Frequent practice with these commands will solidify your understanding, making your workflow more efficient and effective. Embrace the power of Git and continue to explore its commands and techniques to become a proficient developer!
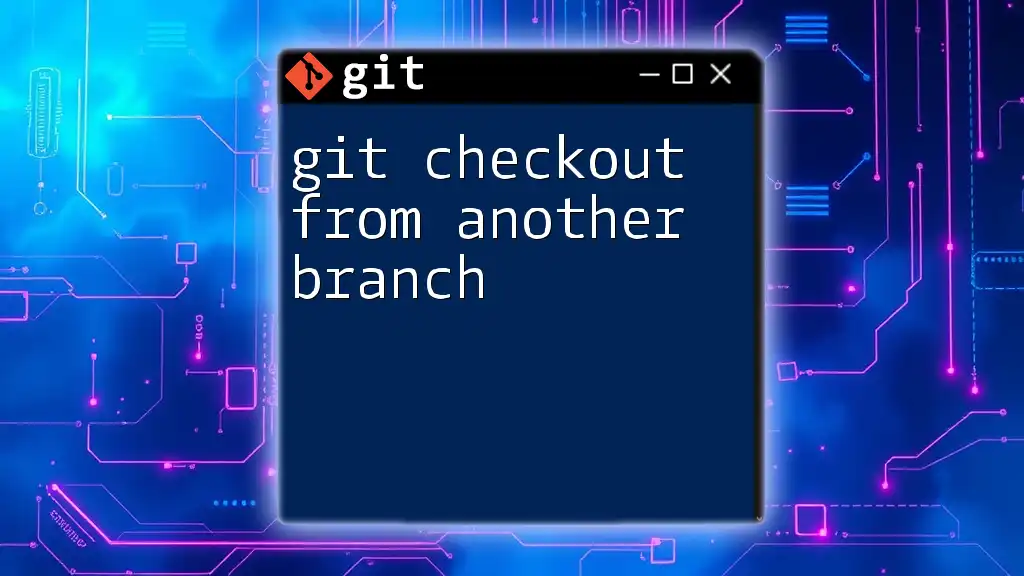
Additional Resources
For further learning, consult the official Git documentation, which provides comprehensive descriptions of commands and their uses. There are also numerous tutorials and courses available online, dedicated to honing your Git skills for better collaboration and productivity.